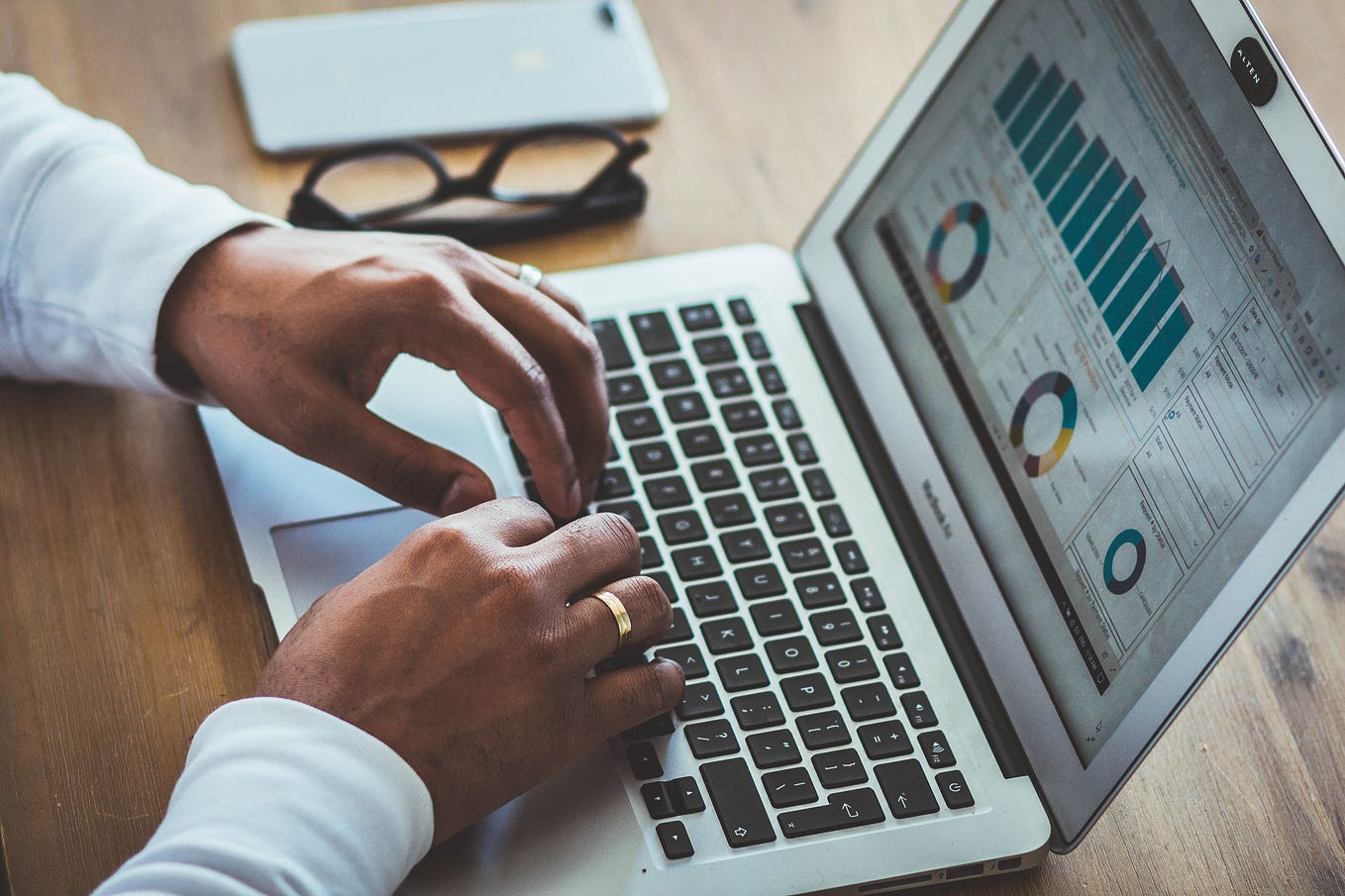
Understanding the Android Design Guidelines
Creating a successful Android app starts with adhering to the Android Design Guidelines. These guidelines offer a framework for designing visually pleasing and intuitive user interfaces (UIs).
Layout Basics
- Use rounded corners for UI buttons
- Avoid using two icons in a single button
- Ensure text is clear and readable
UI Component Functionality
- Buttons should be easily clickable
- Design text fields for specific purposes (e.g., numeric values, passwords)
Consistency in Design
- Achieve a consistent look recognizable to any Android user
- Minimize learning curves to improve retention rates
Keeping Up with the Latest Android Trends
Staying updated with the latest trends is crucial for maintaining a competitive edge.
SDK Updates
- New APIs, libraries, and tools improve app performance, security, and functionality
- Recent updates include features like Jetpack Compose for simplified UI development
Best Practices for SDK Integration
- Thoroughly test new features to avoid bugs or performance issues
- Document codebase changes for future maintenance
Asking for and Listening to User Feedback
User feedback provides insights into what users like and dislike about the app.
Methods for Gathering Feedback
- In-App Pop-Ups: Prompt users for reviews or feedback directly within the app
- Market Research: Use questionnaires, surveys, and focus groups
- Incentives: Offer discounts or exclusive content to encourage feedback
Acting on Feedback
- Incorporate user feedback into the development process
- Show users that their input is valued by making improvements based on their suggestions
Selecting the Best Development Method
Choosing the right development method depends on the project's requirements and goals.
Native App Development
- Full control over app performance and features
- Requires separate development for each platform
Hybrid App Development
- Uses web technologies for apps that run on multiple platforms
- Faster and more cost-effective but may compromise performance
Web-Based App Development
- Ideal for apps that do not require native functionality
- Offers a more universal reach across different devices
Maintaining High-Quality Code
Quality code is essential for Android app development.
Using Proguard
- Deletes unusable code and reduces APK size
- Improves app performance by reducing data load
Keeping Code Organized
- Separate different functionalities into modules or classes
- Avoid deep hierarchies in the code structure
Interacting with Users
- Design text fields for specific jobs (e.g., numeric values, passwords)
- Provide hints for passwords to enhance user experience
Working with Designers
Effective collaboration between developers and designers is crucial.
Understanding Design Elements
- Balance creativity with functionality
- Understand the effects of layout additives and design elements
Using Mood Boards
- Organize thoughts and track design elements
- Reduce the chance of making many changes later on
Early Planning
- Plan design elements early to avoid last-minute changes
Optimizing for Performance
Performance optimization is critical in Android app development.
Using the Right View Group
- Avoid deep hierarchies in the code
- Use a single-level order to keep things simple
Gradle Settings
- Increase memory allocation and enable data storage in memory for subsequent builds
- Recommended settings:
gradle.jvmargs=-Xmx2048m
,gradle.daemon=true
,gradle.configureondemand=true
Modularizing the App
- Run tasks from multiple projects simultaneously
- Useful for complex apps
Utilizing ADB for Development
ADB (Android Debug Bridge) is a command-line tool for communicating with the Android operating system.
Adding/Removing Files/Data
- Add or remove files/data from Android devices
- Useful for testing and debugging purposes
Hidden Data Management
- Manage hidden data quickly on your computer
Conducting In-Depth Research
Research is essential before starting any app development project.
Market Research
- Determine if there is a need for your proposed app
- Ask people directly about their needs and show mockups
Learning from Similar Apps
- Understand what features are missing in similar apps
- Differentiate your app from existing ones
Designing a Strategic Plan
A strategic plan helps understand how your app will differ from others.
Understanding Target Audience
- Determine if there is a need for an app of that kind in your target audience’s eyes
- Avoid developing another app if similar services or products already exist
Choosing Development Method
- Decide on native or cross-platform development based on your strategic plan
By following these tips and techniques, developers can create successful and user-friendly apps that meet the evolving needs of the market. Whether you're a beginner or an experienced developer, understanding these best practices will help navigate the challenges of Android development and produce high-quality applications that users will love.