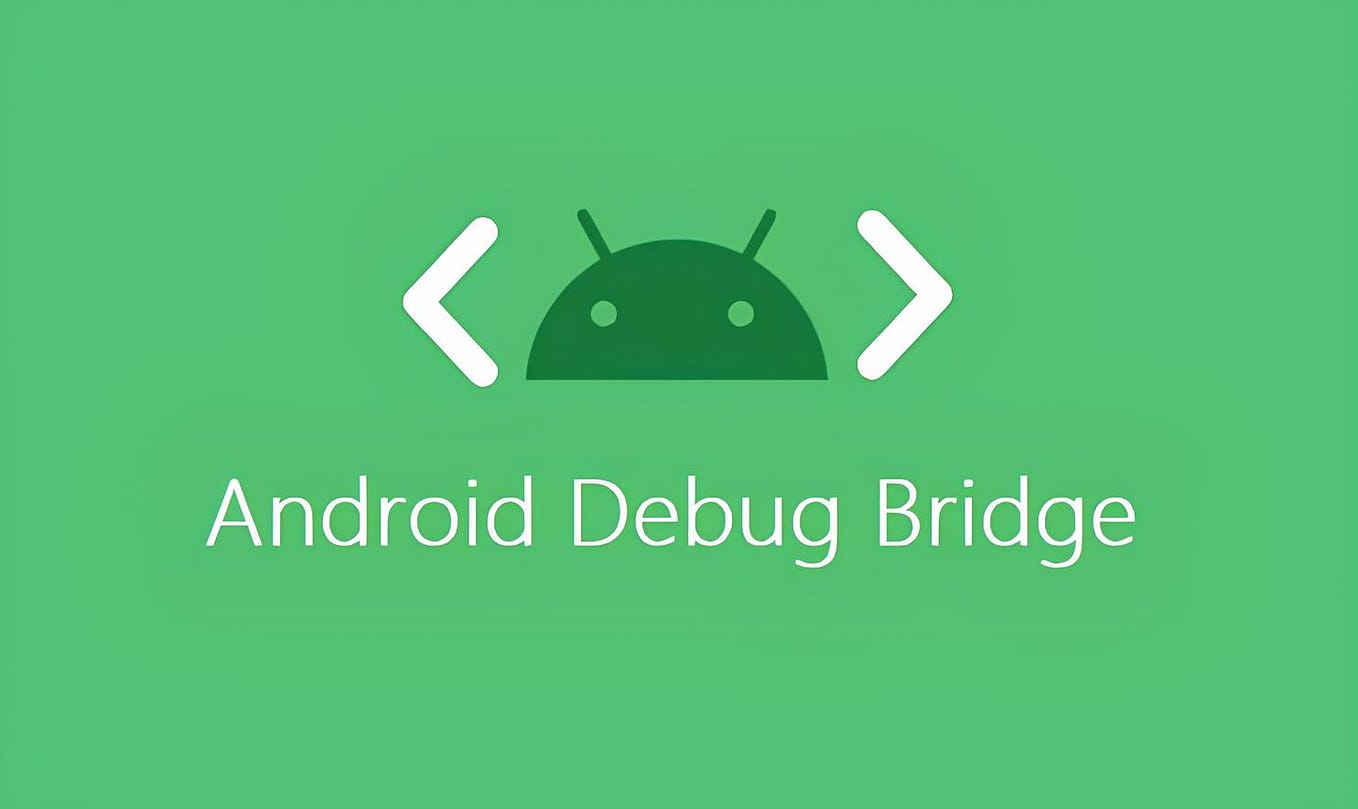
Introduction to ADB
What is ADB?
Android Debug Bridge (ADB) is a command-line tool that lets you communicate with an Android device. Think of it as a bridge between your computer and your Android phone or tablet. With ADB, you can run commands on your device, install and debug apps, and even access the device's file system. It's a must-have tool for developers and tech enthusiasts who want to get the most out of their Android devices.
Why Master ADB on Windows?
Mastering ADB on Windows can be a game-changer for developers and power users. For developers, it simplifies the process of testing and debugging apps. You can quickly install apps, run tests, and gather logs without touching your device. For power users, ADB offers a way to customize and control your device beyond the standard settings. You can remove bloatware, back up data, and even root your device. Integrating ADB with Windows makes these tasks more efficient and straightforward.
Key Takeaways:
- Mastering ADB on Windows lets you control and customize your Android device, making tasks like installing apps, transferring files, and debugging much easier and more efficient.
- Using ADB with Android Studio helps developers test and debug apps quickly, while power users can remove unwanted apps, back up data, and even root their devices for more control.
Setting Up ADB on Windows
Download and Install Android Studio
First, you need to download and install Android Studio, which includes ADB. Head over to the Android Studio website and click on the download button. Once the download is complete, open the installer and follow the on-screen instructions. The installation process might take a while, so be patient. After installation, launch Android Studio to complete the initial setup.
Installing ADB Command-Line Tools
After setting up Android Studio, you need to install the ADB command-line tools. Open Android Studio and go to the SDK Manager. You can find it under the "Configure" menu on the welcome screen or in the "Tools" menu if you have a project open. In the SDK Manager, look for the "SDK Tools" tab. Check the box next to "Android SDK Platform-Tools" and click "Apply" to install ADB.
Configuring Environment Variables
To use ADB from any command prompt, you need to set up environment variables. First, find the path where the SDK Platform-Tools are installed. Usually, it's in the "sdk" folder inside the Android Studio installation directory. Copy this path. Next, right-click on "This PC" or "My Computer" on your desktop and select "Properties." Click on "Advanced system settings" and then "Environment Variables." In the "System variables" section, find the "Path" variable and click "Edit." Add the path you copied earlier and click "OK" to save the changes.
Basic ADB Commands
Connecting Your Device
To connect your Android device to your Windows computer using ADB, you need a USB cable. First, enable Developer Options on your device by going to "Settings," then "About phone," and tapping "Build number" seven times. In Developer Options, enable "USB debugging." Connect your device to your computer via USB. Open a command prompt and type adb devices
. If everything is set up correctly, you should see your device listed.
Common Commands
Once your device is connected, you can start using basic ADB commands. The adb devices
command lists all connected devices. The adb install
command lets you install an APK file on your device. For example, adb install myapp.apk
installs the app named "myapp.apk." The adb push
command transfers files from your computer to your device. For instance, adb push myfile.txt /sdcard/myfile.txt
copies "myfile.txt" to the device's SD card. These commands are just the tip of the iceberg, but they are essential for getting started with ADB.
Advanced ADB Usage
Wireless ADB Debugging
Wireless ADB debugging lets you connect your Android device to your computer without a USB cable. First, connect your device via USB and enable Developer Options. Then, toggle on Wireless Debugging. Use the command adb tcpip 5555
to switch ADB to listen over TCP/IP. Disconnect the USB cable and find your device's IP address in the same Developer Options menu. Finally, connect wirelessly using adb connect [device_ip]:5555
.
Port Forwarding
Port forwarding with ADB allows you to redirect network traffic from your computer to your Android device. This is useful for testing network applications. Use the command adb forward tcp:[local_port] tcp:[remote_port]
. For instance, adb forward tcp:8080 tcp:8080
forwards traffic from port 8080 on your computer to port 8080 on your device.
File Transfer
Transferring files between your device and computer is straightforward with ADB. To push a file from your computer to your device, use adb push [source] [destination]
. For example, adb push myfile.txt /sdcard/myfile.txt
copies myfile.txt
to your device's SD card. To pull a file from your device to your computer, use adb pull [source] [destination]
. For instance, adb pull /sdcard/myfile.txt myfile.txt
copies the file from your device to your computer.
Troubleshooting ADB Issues
Connection Problems
ADB connection problems can be frustrating. Ensure USB debugging is enabled on your device. Use a good-quality USB cable and try different USB ports. Restarting both your device and computer can help. If issues persist, use adb kill-server
followed by adb start-server
to restart the ADB server.
Device Not Recognized
If your device isn't recognized by ADB, check if the correct USB drivers are installed. On Windows, you might need to install OEM-specific drivers. Use adb devices
to see if your device appears. If not, try switching USB modes on your device (e.g., from charging to file transfer).
ADB Server Issues
Sometimes, the ADB server itself causes problems. To fix this, stop and restart the server using adb kill-server
and adb start-server
. If the issue persists, check for conflicting software that might be using the same ports as ADB. Disabling such software temporarily can resolve the conflict.
Integrating ADB with Development Tools
Using ADB with Android Studio
Android Studio and ADB go hand in hand. To start, make sure your Android device is connected to your computer. Open Android Studio, and it should automatically detect your device. If it doesn't, you might need to enable USB debugging on your device. Head to the Developer Options in your device's settings and toggle on USB debugging.
Once connected, you can use ADB commands directly within Android Studio's terminal. This makes debugging and testing apps a breeze. For instance, you can install your app on the device using adb install
or check the list of connected devices with adb devices
. This integration streamlines the development process, allowing you to focus more on coding and less on setup.
ADB and Command-Line Interfaces
ADB isn't just limited to Android Studio. It works seamlessly with other command-line tools and scripts. For example, you can write shell scripts to automate repetitive tasks like installing multiple APKs or pulling logs from your device.
To use ADB in a command-line interface, open your terminal or command prompt. Navigate to the directory where ADB is installed, or add ADB to your system's PATH for easier access. From there, you can run any ADB command just like you would in Android Studio. This flexibility makes ADB a powerful tool for developers who prefer working in a command-line environment.
Security and Best Practices
Securing ADB Connections
When using ADB, especially over Wi-Fi, security becomes a concern. Always ensure that your device is connected to a trusted network. Avoid using public Wi-Fi for ADB connections. To secure your ADB connection, use strong passwords and consider setting up a VPN.
Another good practice is to disable USB debugging when not in use. This prevents unauthorized access to your device. You can also use ADB over a secure shell (SSH) to add an extra layer of security. These steps help protect your device and data from potential threats.
Maintaining Device Security
While ADB is a powerful tool, it can also be a security risk if not used properly. Always be cautious about the commands you run, especially those that modify system files or settings. Only use ADB with devices you trust and avoid connecting to unknown devices.
Regularly update your device's software to patch any security vulnerabilities. Also, consider using a secure lock screen method, like a PIN or fingerprint, to prevent unauthorized access. By following these best practices, you can enjoy the benefits of ADB while keeping your device secure.
Additional ADB Features
Taking Screenshots and Recording Videos
ADB makes capturing screenshots and recording screen activity straightforward. To take a screenshot, connect your device and run adb shell screencap /sdcard/screenshot.png
. Then, pull the screenshot to your computer with adb pull /sdcard/screenshot.png
.
For recording videos, use adb shell screenrecord /sdcard/video.mp4
. This command starts recording your device's screen. To stop recording, press Ctrl+C. After recording, use adb pull /sdcard/video.mp4
to transfer the video to your computer. These features are handy for creating tutorials or documenting issues.
Managing Apps and Packages
ADB offers advanced commands for managing apps and packages. To list all installed packages, use adb shell pm list packages
. If you want to uninstall an app, run `adb uninstall
. You can also disable or enable apps with
adb shell pm disable
and
adb shell pm enable
These commands give you fine-grained control over the apps on your device. They are particularly useful for developers testing different versions of their apps or users who want to remove bloatware.
Using Dumpsys for System Information
Dumpsys is a powerful ADB command that provides detailed system information. To use it, run adb shell dumpsys
. This command outputs a lot of data, so you might want to filter it. For example, adb shell dumpsys battery
gives you information about the battery status.
Dumpsys can help diagnose issues by providing insights into various system components. Whether you're troubleshooting performance problems or checking system health, Dumpsys is an invaluable tool for getting detailed information about your device.
Wrapping It All Up
Technology offers endless possibilities, and mastering tools like ADB can supercharge your Android device experience. Whether you're a developer debugging apps or a power user customizing your phone, ADB provides powerful commands that make life easier. From installing apps to transferring files and even securing your device, ADB proves invaluable. Plus, integrating it with tools like Android Studio streamlines workflows and saves time. Remember to follow best practices for security to keep your device safe. By diving into these features, you unlock a whole new level of tech-savviness. Happy hacking!
Understanding Android ADB on Windows
Android ADB, or Android Debug Bridge, acts like a Swiss Army knife for your Android device. It lets you communicate with your phone or tablet from a computer. You can install apps, transfer files, and even debug your device. With ADB, you can run shell commands, capture screenshots, and record screen activity. It also helps in unlocking bootloaders and flashing custom ROMs. Essentially, ADB gives you full control over your Android device from your computer.
Necessary Tools and System Compatibility
To ensure your device supports Android ADB on Windows, check these requirements:
- Operating System: Your computer must run Windows 7 or later. Older versions won't work.
- Android Version: Your phone or tablet needs Android 4.0 (Ice Cream Sandwich) or newer. Devices with older versions won't be compatible.
- USB Drivers: Install the OEM USB drivers specific to your device. Without these, your computer won't recognize your phone.
- Developer Options: Enable Developer Options on your Android device. Go to Settings > About Phone and tap Build Number seven times.
- USB Debugging: Within Developer Options, turn on USB Debugging. This allows your computer to communicate with your device.
- USB Cable: Use a high-quality USB cable. Faulty or low-quality cables can cause connection issues.
- ADB Tools: Download and install the ADB tools from the Android SDK Platform Tools. Ensure you have the latest version for compatibility.
- Permissions: When you connect your device, grant USB Debugging permissions on your phone. This step is crucial for establishing a connection.
By meeting these requirements, your device should support Android ADB on Windows seamlessly.
Getting Started with Android ADB on Windows
- Download the Android SDK Platform Tools from the official Android website.
- Extract the downloaded ZIP file to a location on your computer.
- Open the extracted folder and find the "platform-tools" directory.
- Right-click on "This PC" or "My Computer" and select "Properties."
- Click on "Advanced system settings" on the left.
- Select the "Environment Variables" button.
- Under "System variables," find and select the "Path" variable, then click "Edit."
- Click "New" and paste the path to the "platform-tools" directory.
- Click "OK" to close all dialog boxes.
- Connect your Android device to your computer using a USB cable.
- Enable USB debugging on your Android device by going to "Settings" > "About phone" > tap "Build number" seven times to unlock developer options, then go to "Developer options" and turn on "USB debugging."
- Open Command Prompt on your computer.
- Type
adb devices
and press Enter. - Verify your device appears in the list. If prompted on your device, allow USB debugging.
Done!
Tips for Effective Use of Android ADB
Install ADB: Download the Android SDK Platform Tools from the official Android website. Extract the files to a folder on your computer.
Enable Developer Options: On your Android device, go to Settings > About Phone. Tap Build Number seven times to unlock Developer Options. Then, go to Settings > Developer Options and enable USB Debugging.
Connect Device: Plug your Android device into your computer using a USB cable. Open a command prompt or terminal window on your computer. Navigate to the folder where you extracted the Platform Tools.
Verify Connection: Type adb devices
in the command prompt. If your device is listed, you're good to go. If not, make sure your USB drivers are installed correctly.
Install Apps: Use `adb install
to install apps directly from your computer. Replace
Uninstall Apps: Remove unwanted apps with `adb uninstall
. Replace
Transfer Files: Use adb push <local_path> <remote_path>
to transfer files from your computer to your device. For the opposite, use adb pull <remote_path> <local_path>
.
Screen Recording: Record your screen with adb shell screenrecord /sdcard/demo.mp4
. Stop recording by pressing Ctrl+C.
Reboot Device: Restart your device using adb reboot
. For recovery mode, use adb reboot recovery
.
Logcat: View system logs with adb logcat
. This helps in debugging apps.
Wireless ADB: Connect wirelessly by first connecting via USB, then typing adb tcpip 5555
followed by adb connect <device_ip_address>
. Replace <device_ip_address>
with your device's IP.
Backup and Restore: Backup your device with adb backup -apk -shared -all -f backup.ab
. Restore using adb restore backup.ab
.
Factory Reset: Perform a factory reset with adb shell am broadcast -a android.intent.action.MASTER_CLEAR
.
Shell Commands: Access the device's shell with adb shell
. This allows you to run various Linux commands directly on your device.
Stay Updated: Always keep your ADB tools updated to avoid compatibility issues.
Troubleshooting Frequent Problems
Problem: ADB device not recognized. Solution: Ensure USB debugging is enabled on the phone. Check the USB cable for damage. Try a different USB port. Update or reinstall ADB drivers.
Problem: ADB command not found. Solution: Add ADB to the system PATH. Open Environment Variables, find PATH, and add the directory where ADB is installed.
Problem: Device unauthorized. Solution: Revoke USB debugging authorizations on the phone. Reconnect the device and accept the RSA key fingerprint prompt.
Problem: ADB server is out of date.
Solution: Kill the ADB server using adb kill-server
. Restart it with adb start-server
. Ensure the latest version of ADB is installed.
Problem: ADB connection reset. Solution: Disable and re-enable USB debugging on the phone. Restart both the computer and the device. Check for conflicting software that might interfere with ADB.
Problem: ADB over Wi-Fi not working.
Solution: Ensure both the computer and device are on the same network. Use adb tcpip 5555
to switch to TCP mode. Connect using the device's IP address with adb connect [device IP]:5555
.
Problem: Slow ADB transfer speeds. Solution: Use a high-quality USB cable. Connect to a USB 3.0 port. Disable any unnecessary background processes on the computer.
Problem: ADB shell commands not executing. Solution: Verify the device is rooted if required for the command. Check for typos or syntax errors in the command. Ensure the device is properly connected and recognized by ADB.
Problem: ADB push/pull not working. Solution: Confirm the file paths are correct. Ensure the device has sufficient storage space. Check for permission issues on the device.
Problem: ADB logcat not displaying logs. Solution: Verify the device is connected and recognized. Ensure USB debugging is enabled. Use the correct filters to display the desired logs.
Keeping Your Data Safe
Using Android ADB on Windows requires careful attention to security and privacy. When connecting your device, ensure USB debugging is only enabled when necessary. This feature grants deep access to your device, so disable it when not in use. Always use trusted computers to avoid unauthorized access.
User data handled through ADB commands can be sensitive. Avoid executing commands from unknown sources. Regularly update your ADB tools to patch any vulnerabilities. Use strong passwords and enable encryption on your device to protect data.
For additional privacy, consider using a VPN when transferring data over networks. Be mindful of the permissions granted to apps, especially those requiring root access. Regularly review and manage app permissions to minimize data exposure.
Comparing Other Tools to Android ADB
Pros of Android ADB:
- Flexibility: Allows deep access to Android devices for debugging and development.
- Control: Offers command-line control over device functions.
- Customization: Enables installation of custom ROMs and apps not available on the Play Store.
- Backup: Facilitates full device backups and restores.
Cons of Android ADB:
- Complexity: Requires technical knowledge to use effectively.
- Security Risks: Potential for misuse if unauthorized access is gained.
- Compatibility Issues: May not work seamlessly with all devices or Windows versions.
Alternatives:
Apple iOS Xcode:
- Pros: Integrated development environment for iOS apps, user-friendly interface, strong community support.
- Cons: Only available on macOS, limited to Apple devices.
Samsung Smart Switch:
- Pros: Easy data transfer between Samsung devices, user-friendly, supports multiple data types.
- Cons: Limited to Samsung devices, fewer customization options.
Huawei HiSuite:
- Pros: Simplifies data management for Huawei devices, easy backup and restore.
- Cons: Limited to Huawei devices, fewer advanced features.
Microsoft Windows Device Portal:
- Pros: Web-based interface for managing Windows devices, supports IoT devices.
- Cons: Limited to Windows devices, less control compared to ADB.
Problem: ADB device not recognized. Solution: Ensure USB debugging is enabled on the phone. Check the USB cable for damage. Try a different USB port. Update or reinstall ADB drivers.
Problem: ADB command not found. Solution: Add ADB to the system PATH. Open Environment Variables, find PATH, and add the directory where ADB is installed.
Problem: Device unauthorized. Solution: Revoke USB debugging authorizations on the phone. Reconnect the device and accept the RSA key fingerprint prompt.
Problem: ADB server is out of date.
Solution: Kill the ADB server using adb kill-server
. Restart it with adb start-server
. Ensure the latest version of ADB is installed.
Problem: ADB connection reset. Solution: Disable and re-enable USB debugging on the phone. Restart both the computer and the device. Check for conflicting software that might interfere with ADB.
Problem: ADB over Wi-Fi not working.
Solution: Ensure both the computer and device are on the same network. Use adb tcpip 5555
to switch to TCP mode. Connect using the device's IP address with adb connect [device IP]:5555
.
Problem: Slow ADB transfer speeds. Solution: Use a high-quality USB cable. Connect to a USB 3.0 port. Disable any unnecessary background processes on the computer.
Problem: ADB shell commands not executing. Solution: Verify the device is rooted if required for the command. Check for typos or syntax errors in the command. Ensure the device is properly connected and recognized by ADB.
Problem: ADB push/pull not working. Solution: Confirm the file paths are correct. Ensure the device has sufficient storage space. Check for permission issues on the device.
Problem: ADB logcat not displaying logs. Solution: Verify the device is connected and recognized. Ensure USB debugging is enabled. Use the correct filters to display the desired logs.
Mastering Android ADB on Windows
Mastering Android ADB on Windows opens up a world of possibilities for developers and tech enthusiasts. With ADB, you can debug apps, transfer files, and even control your device from your computer. It's a powerful tool that, once understood, can significantly enhance your productivity and capabilities.
Remember to install the necessary drivers and set up your environment correctly. Familiarize yourself with basic commands like adb devices
, adb push
, and adb pull
. These commands will become second nature with practice.
Don't forget to enable USB debugging on your Android device. Without this, ADB won't recognize your device. Keep your software updated to avoid compatibility issues.
In short, ADB is an essential tool for anyone serious about Android development or customization. Dive in, experiment, and you'll soon see how much it can do.
What is the difference between Logcat and Dumpsys?
Logcat is mainly used by app developers to view logs and debug apps. Dumpsys is designed to get state info about system processes and often maintains some history of activities.
How do I get ADB to work on Windows?
First, download and install the latest Android Studio release. After installation, click the More Actions button and select SDK Manager. In the SDK Manager, go to SDK Tools and install Android SDK Command-Line tools.
What does the command adb tcpip 5555 do?
This command enables TCP/IP mode on your Android device, making it listen on port 5555 for ADB connections.
How do I enable ADB over TCP mode on my Android phone?
Connect your Android device to your computer via USB. Run the command adb tcpip 5555 to enable TCP/IP mode. After that, disconnect the USB cable.
Why would I use ADB over TCP/IP instead of USB?
Using ADB over TCP/IP allows for wireless debugging, which can be more convenient than being tethered to a USB cable, especially when testing apps on multiple devices.
What are the basic steps to install ADB on a Windows machine?
Download Android Studio and install it. Open SDK Manager from the More Actions dropdown. In SDK Tools, select and install Android SDK Command-Line tools.
Can I use ADB without installing Android Studio?
Yes, you can download the SDK Platform Tools from the official Android developer website. Extract the tools and add the path to your system's environment variables.