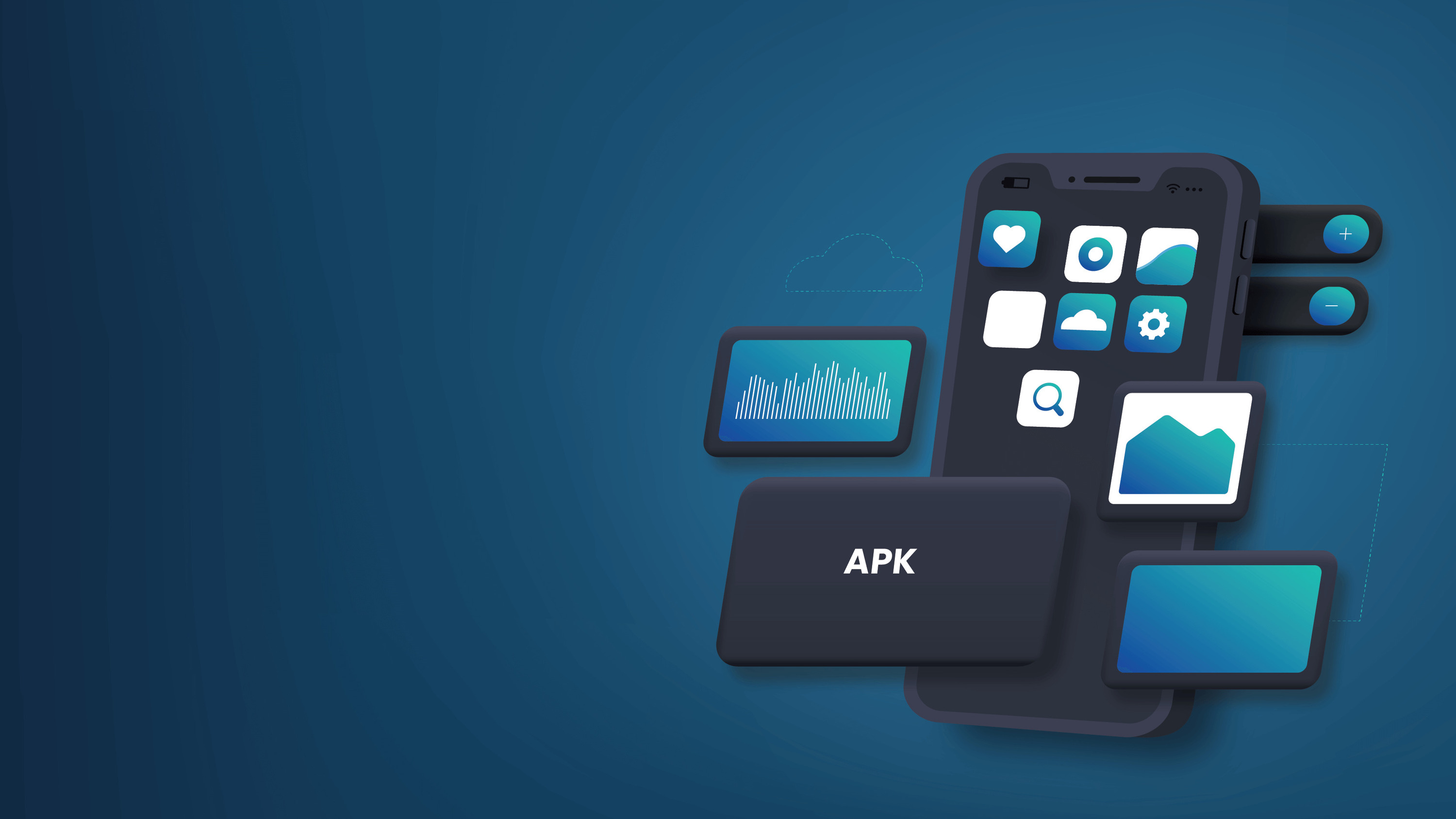
Introduction
Android Studio is the primary integrated development environment (IDE) for developing Android applications. It provides a comprehensive set of tools and features to help developers build, run, and debug their apps. One of the essential steps in the development process is building an APK (Android Package Kit) for testing and releasing your app. This guide will walk you through the process of building an APK in Android Studio, covering both debug and release builds.
Key Takeaways:
- Building APKs and AABs in Android Studio is simple: APKs are direct install files, while AABs are modular and optimized for different devices, making apps smaller and faster to download.
- Troubleshooting build issues and using best practices like versioning and code shrinking can make your app development smoother, faster, and more efficient.
Setting Up Your Project
Before building an APK, set up your project in Android Studio. Here’s how:
Create a New Project
- Open Android Studio.
- Click on
Start a new Android Studio project
. - Choose the type of project you want to create (e.g., Empty Activity, App Module, etc.).
- Fill in the necessary details such as project name, package name, and location.
- Click
Next
and thenFinish
.
Configure Your Build
By default, Android Studio sets up your project with two build variants: debug and release. Manage these build variants by going to Build
> Select Build Variant
or using the Build Variants
panel.
Building a Debug APK
Building a debug APK is useful for immediate testing and debugging of your app. Follow these steps:
Open Command Line
Navigate to the root of your project directory.
Invoke Gradle Task
To build a debug APK, invoke the assembleDebug
task using Gradle:
bash
gradlew assembleDebug
This command creates an APK named module_name-debug.apk
in the project_name/module_name/build/outputs/apk/
directory. The file is already signed with the debug key and aligned with zipalign
, so you can immediately install it on a device.
Install Debug APK
To build and immediately install the APK on a running emulator or connected device, use:
bash
gradlew installDebug
The "Debug" part in the task names is just a camel-case version of the build variant name, so it can be replaced with whichever build type or variant you want to assemble or install.
Run Tasks
To see all the build and install tasks available for each variant (including uninstall tasks), run:
bash
gradlew tasks
Building a Release APK
Building a release APK is necessary for sharing or uploading your app to the Google Play Store. Follow these steps:
Open Build Menu
Go to Build
> Generate Signed Bundle / APK...
.
Select APK or AAB
In the window that appears, select whether you would like to generate an App Bundle (required for the Google Play Store) or an APK.
Complete Keystore Information
Complete the Keystore information by either choosing an existing one or creating a new one. Once done, click Next
.
Create Release Destination Folder
Select the release destination folder and click Create
.
Locate Bundle/APK
After a successful build, click Locate
in the pop-up window that appears. This will open up a Finder window with the location of your Bundle/APK.
Sign Your App
If building an APK for release, sign your app with a release key before uploading it to the Play Console. For more information about app signing, see Sign Your App.
Advanced Build Features
Android Studio provides several advanced build features useful for more complex projects.
Change Build Variant
By default, Android Studio builds the debug version of your app when you click Run
. To change the build variant, follow these steps:
- Go to
Build
>Select Build Variant
. - Alternatively, use
View
>Tool Windows
>Build Variants
. - Click on the
Build Variants
tab on the tool window bar.
Switch Between Variants
For projects without native/C++ code, the Build Variants
panel has two columns: Module
and Active Build Variant
. Click on the Active Build Variant
cell for a module and choose the desired variant from the list. For projects with native/C++ code, there are three columns: Module
, Active Build Variant
, and Active ABI
. Change either column by clicking on it and selecting from the list. The IDE will sync your project automatically after changing either column.
Deploying Your App
Once you have built your APK, deploy it to either an emulator or a physical device.
Deploying to Emulator
To use the Android Emulator, create an Android Virtual Device (AVD) using Android Studio. Here’s how:
-
Create an AVD using Android Studio by going to
Tools
>Android
>AVD Manager
. -
Start the emulator by specifying your AVD:
bash
android_sdk/tools/emulator -avd avd_nameIf unsure of the AVD name, execute:
bash
android_sdk/tools/emulator -list-avds -
Install your app using either one of the Gradle install tasks mentioned earlier or the
adb
tool:
bash
adb install path/to/your_app.apkAll APKs you build are saved in
project_name/module_name/build/outputs/apk/
.
Deploying to Physical Device
Before running your app on a physical device, enable USB debugging
on your device. Here’s how:
-
Enable USB Debugging:
- Go to
Settings
>Developer options
. - On Android 4.2 and newer,
Developer options
is hidden by default. To make it available, go toSettings
>About phone
, tapBuild number
seven times, and then return to the previous screen to findDeveloper options
.
- Go to
-
Connect your device via USB.
-
Install your app using either the Gradle install tasks mentioned earlier or the
adb
tool:
bash
adb -d install path/to/your_app.apkAll APKs you build are saved in
project_name/module_name/build/outputs/apk/
.
Troubleshooting Common Issues
Here are some common issues you might encounter while building and deploying your APK:
Errors During Build
If errors occur during the build process, Android Studio will warn you about any issues with the target device configuration. Iconography and stylistic changes differentiate between errors (device selections that result in a broken configuration) and warnings (device selections that might result in unexpected behavior but are still runnable).
Signing Issues
If building a release APK, ensure that you've signed your app with a release key. For more information about app signing, see Sign Your App.
Gradle Tasks Not Found
If issues with Gradle tasks not being found occur, ensure that you've correctly set up your Gradle wrapper in your project directory. Check this by navigating to your project directory and running:
bash
gradlew tasks
This should list all available tasks for your project.
Final Thoughts
Building an APK in Android Studio is a crucial step in the development process. Whether testing your app in debug mode or preparing it for release, understanding how to build and deploy your APK is essential. By following the steps outlined in this guide, you'll be able to manage your build variants, deploy to both emulators and physical devices, and troubleshoot common issues that may arise during the process. With these tools at your disposal, you'll be well-equipped to handle any challenges that come up as you develop and distribute your Android applications.
Feature Overview
Android Studio's Build APK feature compiles your app's code, resources, and assets into an APK file. This file can be installed on Android devices. Key functionalities include debugging, signing, and optimizing the APK. It also supports multiple build variants for different versions of your app.
Necessary Requirements and Compatibility
To ensure your device supports the feature, check these requirements and compatibility details:
Operating System: Your device must run Android 5.0 (Lollipop) or higher. Older versions won't support the latest features.
Processor: A 64-bit ARM or x86 processor is necessary. Devices with 32-bit processors may face performance issues.
RAM: At least 2GB of RAM is required. For smoother performance, 4GB or more is recommended.
Storage: Ensure you have at least 500MB of free space for the app and its data. More space might be needed for additional features or updates.
Screen Resolution: A minimum resolution of 720p (1280x720) is required. Higher resolutions like 1080p (1920x1080) will provide a better experience.
Internet Connection: A stable Wi-Fi or 4G/5G connection is essential for downloading updates and accessing online features.
Bluetooth: If the feature involves connecting to other devices, Bluetooth 4.0 or higher is needed.
Sensors: Some features may require specific sensors like GPS, accelerometer, or gyroscope. Check your device specifications.
Permissions: Ensure the app has the necessary permissions like location, storage, and camera access.
Meeting these requirements will help you enjoy all the features without any hiccups.
Initial Setup Steps
- Open Android Studio: Launch the program on your computer.
- Open Your Project: Click on "File" then "Open" and select your project.
- Build Menu: Navigate to the top menu bar and click on "Build."
- Build APK: Select "Build Bundle(s) / APK(s)" then "Build APK(s)."
- Wait for Build: Let Android Studio compile your project. This might take a few minutes.
- Locate APK: Once done, a notification will appear. Click "locate" to find your APK file.
- Transfer APK: Move the APK file to your Android device using a USB cable or any file transfer method.
- Install APK: On your device, open the file manager, find the APK, and tap to install. You might need to enable "Install from unknown sources" in your settings.
- Run App: After installation, open the app from your device's app drawer.
Done! Your app is now installed and ready to use.
Effective Usage Tips
Keep your code clean. Use meaningful variable names and consistent formatting. This makes debugging easier.
Use Gradle effectively. Customize your build.gradle file to manage dependencies and build configurations. Avoid hardcoding values; use buildConfigField instead.
Enable ProGuard. It helps shrink and obfuscate your code, making your APK smaller and harder to reverse-engineer.
Test on multiple devices. Emulators are good, but real devices are better. Check performance and UI consistency across different screen sizes and Android versions.
Use Android Profiler. Monitor CPU, memory, and network usage to identify bottlenecks and optimize performance.
Keep libraries updated. Outdated libraries can introduce security vulnerabilities and bugs. Regularly check for updates.
Minimize permissions. Only request permissions your app truly needs. This improves user trust and reduces potential security risks.
Optimize images. Use WebP format for better compression without losing quality. Compress large images to reduce APK size.
Enable Instant Run. It speeds up the build process by deploying only the changes, not the entire APK.
Use version control. Tools like Git help track changes and collaborate with others. Always commit your work regularly.
Automate testing. Use JUnit and Espresso for unit and UI tests. This ensures your app remains stable as you add features.
Monitor crash reports. Tools like Firebase Crashlytics help identify and fix crashes quickly.
Document your code. Good documentation helps others understand your code and makes future maintenance easier.
Backup your keystore. Losing your keystore means you can't update your app. Store it securely.
Stay updated. Follow Android development blogs and forums to keep up with new features and best practices.
Troubleshooting Common Problems
Problem: Slow Emulator Performance
Close unnecessary applications running in the background. Allocate more RAM to the emulator. Use a physical device for testing if possible. Update Android Studio and the emulator to the latest versions.
Problem: Gradle Build Fails
Check for syntax errors in the build.gradle file. Ensure all dependencies are correctly listed. Clear the Gradle cache by running ./gradlew clean
. Sync the project with Gradle files.
Problem: APK Not Installing on Device
Enable USB debugging on the device. Ensure the device is recognized by running adb devices
. Check for sufficient storage space on the device. Verify the APK is signed if installing on a non-debuggable device.
Problem: Missing SDK Components
Open the SDK Manager in Android Studio. Ensure all required SDK components are installed. Update outdated SDK components. Restart Android Studio after making changes.
Problem: Layout Rendering Issues
Ensure all layout files are correctly formatted. Check for missing or incorrect resource references. Update the Android Studio layout editor. Use the "Invalidate Caches / Restart" option in Android Studio.
Problem: Code Changes Not Reflecting
Clean the project by selecting "Build" > "Clean Project." Rebuild the project by selecting "Build" > "Rebuild Project." Ensure the correct build variant is selected. Restart Android Studio if the issue persists.
Problem: Debugger Not Working
Ensure the device is in developer mode with USB debugging enabled. Check for correct breakpoints in the code. Restart the debugging session. Update Android Studio and the Android SDK tools.
Problem: Out of Memory Errors
Increase the heap size in the gradle.properties file. Optimize code to use less memory. Use memory profiling tools to identify memory leaks. Close other memory-intensive applications running on the system.
Problem: Version Compatibility Issues
Check the minimum SDK version in the build.gradle file. Ensure the device meets the minimum SDK requirements. Update libraries to versions compatible with the target SDK. Test on multiple devices with different Android versions.
Problem: Network Connection Issues
Ensure the device has a stable internet connection. Check for correct network permissions in the AndroidManifest.xml file. Use a proxy or VPN if network restrictions exist. Test network requests using a physical device.
Privacy and Security Tips
When using Android Studio to build an APK, user data handling becomes crucial. Always encrypt sensitive information to keep it safe from prying eyes. Use proguard to obfuscate code, making it harder for hackers to reverse-engineer your app. Implement network security configurations to ensure data transmitted over the internet remains secure. Regularly update dependencies to patch any vulnerabilities. Avoid storing personal data directly on the device; instead, use secure storage solutions like SharedPreferences with encryption or SQLite databases with encryption. Enable permissions only when absolutely necessary and always ask for user consent before accessing sensitive information.
Comparing Alternatives
Pros of Android Studio:
- Integrated Development Environment (IDE): Combines code editing, debugging, and testing tools.
- Emulator: Simulates various devices for testing.
- Gradle Build System: Automates tasks like building, testing, and deploying.
- Intelligent Code Editor: Offers code completion, refactoring, and analysis.
- Support for Multiple Languages: Java, Kotlin, and C++.
Cons of Android Studio:
- Resource-Intensive: Requires significant memory and processing power.
- Complex Setup: Can be challenging for beginners.
- Slow Performance: May lag on older machines.
- Frequent Updates: Requires regular updates, which can disrupt workflow.
Alternatives:
- Xcode (for iOS): Offers a robust IDE for Apple devices, with features like Swift programming, Interface Builder, and a powerful simulator.
- Visual Studio (for cross-platform): Supports multiple languages and platforms, including Android, iOS, and Windows, with features like IntelliSense and integrated debugging.
- Eclipse with ADT Plugin: An older but still viable option for Android development, offering a less resource-intensive environment.
- React Native: Allows building mobile apps using JavaScript and React, providing a single codebase for both Android and iOS.
- Flutter: Uses Dart language to create natively compiled applications for mobile, web, and desktop from a single codebase.
Final Thoughts
Building an APK in Android Studio isn't rocket science. Open your project, head to the Build menu, and select Build Bundle(s) / APK(s). Choose Build APK(s), and let Android Studio do its thing. Once done, a notification will pop up. Click it to locate your APK. This file is ready for testing or distribution. Remember, keeping your SDK and tools updated ensures smoother builds. If errors pop up, check your Gradle files and dependencies. With practice, this process becomes second nature. Happy coding!
What's the difference between a build bundle and an APK in Android Studio?
App bundles are for publishing only and can't be installed directly on devices. APKs are installable files that run on Android devices. App bundles need processing by a distributor to become APKs.
Why should I use a split APK instead of a regular APK?
Split APKs let users download smaller, modular APKs tailored to their device. This avoids downloading a large, universal APK with all assets, architectures, and languages.
How do I generate an APK in Android Studio?
Click on "Build" in the menu, then select "Build Bundle(s) / APK(s)" and choose "Build APK(s)." Android Studio will compile your project into an APK file.
Can I test an app bundle on my device?
No, app bundles can't be installed directly on devices. You need to generate APKs from the bundle to test on an actual device.
What are the benefits of using app bundles?
App bundles optimize app delivery by creating APKs specific to a user's device. This reduces download size and improves installation speed.
How do I convert an app bundle to APKs?
Use the "bundletool" command-line tool provided by Google. It converts app bundles into device-specific APKs for testing or distribution.
Are there any downsides to using app bundles?
App bundles require extra steps for testing and distribution. They also depend on Google Play or other distributors to generate the final APKs.