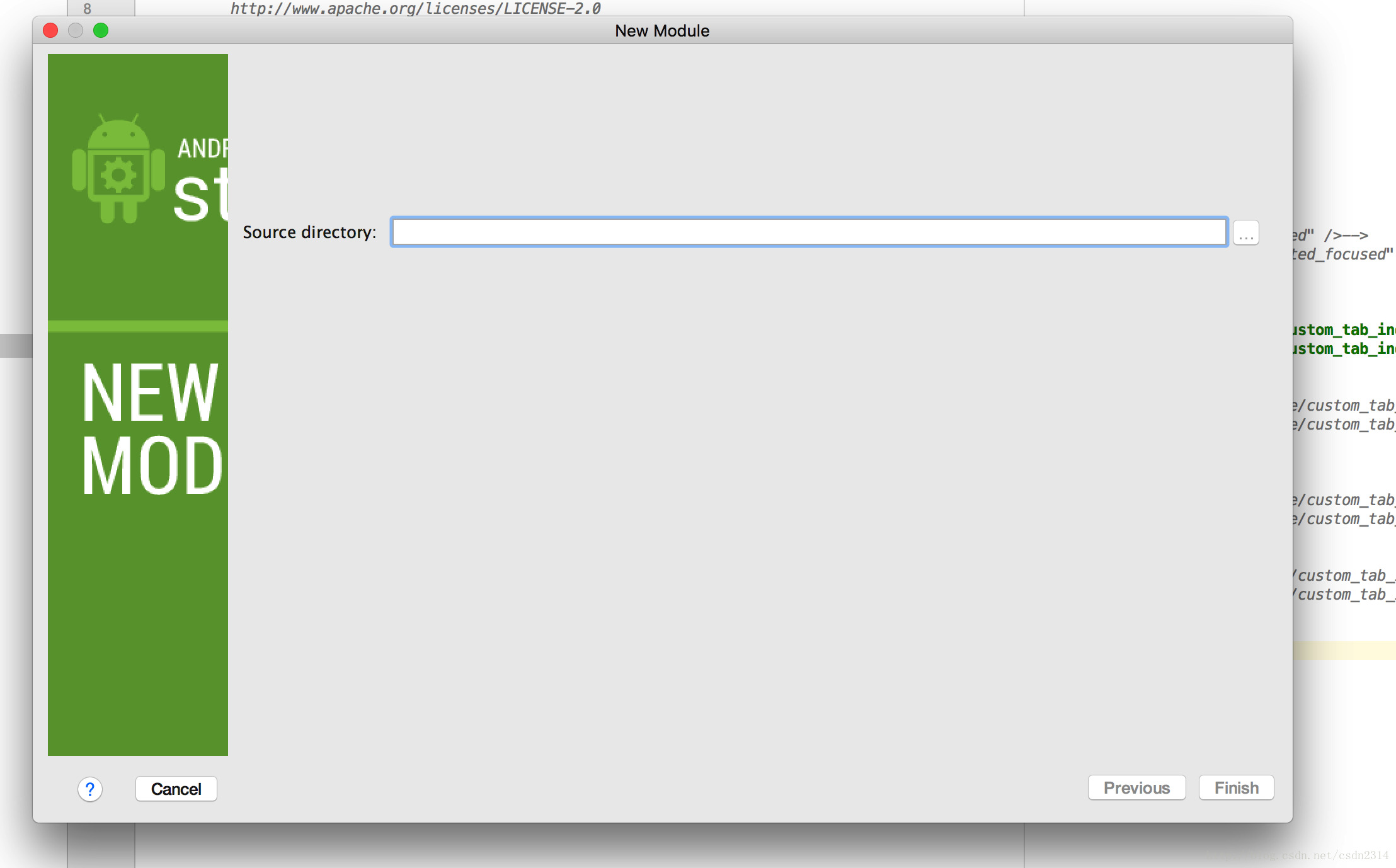
Introduction
Creating a seamless user experience in Android app development is crucial. One key component in achieving this is the ViewPager
, which allows users to flip through pages of data. To make the ViewPager
more intuitive and user-friendly, indicators are often added to show which page is currently active. This article explores ViewPagerIndicator
, its various implementations, usage, and customization options.
Key Takeaways:
- ViewPagerIndicator makes apps cooler by showing dots or lines that help users know which page they're on, making navigation easy and fun.
- Setting up ViewPagerIndicator is simple: add it to your project, connect it with your ViewPager, and customize it to match your app's style.
What is ViewPagerIndicator?
The ViewPagerIndicator
library enhances the functionality of the ViewPager
by adding visual indicators that help users navigate through pages. These indicators can take various forms, such as dots, tabs, or custom designs. The library is particularly useful for providing a clear visual cue about the current page, making it easier for users to understand their position within the ViewPager
.
History and Evolution
The concept of ViewPagerIndicator
has been around for several years, with various libraries emerging to address different needs. One of the earliest and most popular libraries is Jake Wharton's ViewPagerIndicator
, which was widely used in the past but has since been less maintained. Another notable library is Material-ViewPagerIndicator
, designed to work seamlessly with the Android Support Library v4's ViewPager
widget and incorporates Material Design ink animations.
Material-ViewPagerIndicator
Material-ViewPagerIndicator is a modern and easy-to-use library that integrates well with the Android Support Library v4's ViewPager
. It provides a dot-based page indicator with Material Design ink animations, making it visually appealing and intuitive for users.
Features
- Material Design Ink Animations: Includes Material Design ink animations, enhancing the visual appeal of the page indicators.
- Easy to Use: Simple to integrate into your project, requiring minimal code changes.
- Customizable: Appearance of the indicators, such as their color, size, and spacing, can be customized.
Usage
To use Material-ViewPagerIndicator in your project, follow these steps:
-
Add Dependency:
- Add the following dependency to your
build.gradle
file:
gradle
implementation 'com.github.ronaldsmartin:Material-ViewPagerIndicator:x.y.z'
Replace
x.y.z
with the latest release version number. - Add the following dependency to your
-
XML Layout:
- Add the
ViewPagerIndicator
as a child view of yourViewPager
in the XML layout file:
xml
<androidx.viewpager.widget.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignWithParentIfMissing="true">
<com.itsronald.widget.ViewPagerIndicator
android:id="@+id/view_pager_indicator"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom|center_horizontal"
android:gravity="center_vertical"/>
</androidx.viewpager.widget.ViewPager>
- Add the
-
Programmatic Addition:
- Alternatively, add the
ViewPagerIndicator
programmatically:
java
import androidx.viewpager.widget.ViewPager;
import android.view.Gravity;
import android.view.ViewGroup.LayoutParams;
import com.itsronald.widget.ViewPagerIndicator;
// …
final ViewPager viewPager = …;
final ViewPager.LayoutParams layoutParams = new ViewPager.LayoutParams();
layoutParams.width = LayoutParams.MATCH_PARENT;
layoutParams.height = LayoutParams.WRAP_CONTENT;
layoutParams.gravity = Gravity.BOTTOM;final ViewPagerIndicator viewPagerIndicator = new ViewPagerIndicator(context);
viewPager.addView(viewPagerIndicator, layoutParams); - Alternatively, add the
vejei/ViewPagerIndicator
Another popular library for ViewPagerIndicator
is vejei/ViewPagerIndicator, which supports both ViewPager
and ViewPager2
. This library provides a flexible way to customize the indicators and includes various animation modes.
Features
- Support for ViewPager and ViewPager2: Supports both
ViewPager
and the newerViewPager2
, making it versatile for different versions of the Android Support Library. - Customizable Animations: Includes different animation modes, such as slide and fade, which can be set using the
setAnimationMode
method. - Flexible Configuration: Allows you to use the item count of the
ViewPager
automatically or manually set it using thesetItemCount
method.
Usage
To use vejei/ViewPagerIndicator in your project, follow these steps:
-
Add Dependency:
- Add the following dependency to your
build.gradle
file:
gradle
implementation 'io.github.vejei.viewpagerindicator:viewpagerindicator:1.0.0-alpha.1'
- Add the following dependency to your
-
XML Layout:
-
Add the
Indicator
to your layout file:
xml
<androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android"
xmlns:tools="http://schemas.android.com/tools"
android:layout_width="match_parent"
android:layout_height="match_parent"
tools:context=".MainActivity"><androidx.viewpager.widget.ViewPager
android:id="@+id/view_pager2"
android:layout_width="match_parent"
android:layout_height="match_parent"/><io.github.vejei.viewpagerindicator.CircleIndicator
android:id="@+id/circle_indicator"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:layout_constraintBottom_toBottomOf="@id/view_pager2"
app:layout_constraintEnd_toEndOf="@id/view_pager2"
app:layout_constraintStart_toStartOf="@id/view_pager2"/>
</androidx.constraintlayout.widget.ConstraintLayout>
-
-
Programmatic Addition:
- Alternatively, add the
Indicator
programmatically:
java
import androidx.viewpager.widget.ViewPager;
import io.github.vejei.viewpagerindicator.CircleIndicator;
// …
final ViewPager viewPager2 = view.findViewById(R.id.view_pager2);
final CircleIndicator circleIndicator = view.findViewById(R.id.circle_indicator);// Set the ViewPager for the indicator
circleIndicator.setWithViewPager2(viewPager2, false);// Set the item count of the ViewPager (optional)
circleIndicator.setItemCount(activity.data.length);// Set the animation mode for the indicator (optional)
circleIndicator.setAnimationMode(CircleIndicator.AnimationMode.SLIDE); - Alternatively, add the
Implementing ViewPagerIndicator
Implementing a ViewPagerIndicator
in your Android app involves several steps. Here’s a detailed guide on how to do it:
Step 1: Create a New Project
First, create a new project in Android Studio. For this example, use an Empty Activity with Java as the programming language.
Step 2: Add Required Dependency
Navigate to your build.gradle
file and add the required dependency for the ViewPagerIndicator
library you want to use. For example, if you are using Material-ViewPagerIndicator, add:
gradle
implementation 'com.github.ronaldsmartin:Material-ViewPagerIndicator:x.y.z'
Replace x.y.z
with the latest release version number.
Step 3: Design Your Layout
Create a layout file where you will add both the ViewPager
and the ViewPagerIndicator
. For Material-ViewPagerIndicator, your layout file might look like this:
xml
<androidx.viewpager.widget.ViewPager
android:id="@+id/view_pager"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:layout_alignWithParentIfMissing="true">
<!-- Add as a direct child of your ViewPager -->
<com.itsronald.widget.ViewPagerIndicator
android:id="@+id/view_pager_indicator"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:layout_gravity="bottom|center_horizontal"
android:gravity="center_vertical"/>
</androidx.viewpager.widget.ViewPager>
Step 4: Create a PagerAdapter
Create a PagerAdapter
class that will supply pages to the ViewPager
. For example, if you are displaying images, your adapter might look like this:
java
package com.example.viewpagerwithdotsindicator;
import android.content.Context;
import android.media.Image;
import android.view.LayoutInflater;
import android.view.View;
import android.view.ViewGroup;
import android.widget.ImageView;
import androidx.annotation.NonNull;
import androidx.viewpager.widget.PagerAdapter;
public class ViewAdapter extends PagerAdapter {
private Context context;
private LayoutInflater layoutInflater;
private Integer[] images = {R.drawable.one, R.drawable.two, R.drawable.three, R.drawable.four, R.drawable.five};
public ViewAdapter(Context context) {
this.context = context;
}
@Override
public int getCount() {
return images.length;
}
@Override
public boolean isViewFromObject(@NonNull View view, @NonNull Object object) {
return view == object;
}
@NonNull
@Override
public Object instantiateItem(@NonNull ViewGroup container, int position) {
layoutInflater = (LayoutInflater) context.getSystemService(Context.LAYOUT_INFLATER_SERVICE);
View view = layoutInflater.inflate(R.layout.item, null);
ImageView imageView = view.findViewById(R.id.image_view);
imageView.setImageResource(images[position]);
ViewPager viewPager = (ViewPager) container;
viewPager.addView(view, 0);
return view;
}
@Override
public void destroyItem(@NonNull ViewGroup container, int position, @NonNull Object object) {
ViewPager viewPager = (ViewPager) container;
View view = (View) object;
viewPager.removeView(view);
}
}
Step 5: Set Up the ViewPager
In your activity's onCreate
method, set up the ViewPager
and add the adapter:
java
package com.example.viewpagerwithdotsindicator;
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.PagerAdapter;
import androidx.viewpager.widget.ViewPager;
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize the ViewPager
viewPager = findViewById(R.id.view_pager);
// Create and set the adapter for the ViewPager
ViewAdapter viewAdapter = new ViewAdapter(this);
viewPager.setAdapter(viewAdapter);
}
}
Step 6: Add the ViewPagerIndicator
Finally, add the ViewPagerIndicator
either programmatically or through XML. For example, using Material-ViewPagerIndicator:
java
package com.example.viewpagerwithdotsindicator;
import androidx.appcompat.app.AppCompatActivity;
import androidx.viewpager.widget.ViewPager;
public class MainActivity extends AppCompatActivity {
private ViewPager viewPager;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize the ViewPager
viewPager = findViewById(R.id.view_pager);
// Create and set the adapter for the ViewPager
ViewAdapter viewAdapter = new ViewAdapter(this);
viewPager.setAdapter(viewAdapter);
// Add the ViewPagerIndicator programmatically
final ViewPager.LayoutParams layoutParams = new ViewPager.LayoutParams();
layoutParams.width = LayoutParams.MATCH_PARENT;
layoutParams.height = LayoutParams.WRAP_CONTENT;
layoutParams.gravity = Gravity.BOTTOM;
final ViewPagerIndicator viewPagerIndicator = new ViewPagerIndicator(context);
viewPager.addView(viewPagerIndicator, layoutParams);
}
}
Customizing the ViewPagerIndicator
The ViewPagerIndicator
can be customized to fit your app's design and style. Here are some common customization options:
Material-ViewPagerIndicator Customization
Material-ViewPagerIndicator provides several customization options through its constructor and setter methods. Here are some key customization options:
- Color: Change the color of the dots using the
setDotColor
method. - Size: Adjust the size of the dots using the
setDotSize
method. - Spacing: Modify the spacing between dots using the
setDotSpacing
method. - Animation Mode: Alter the animation mode using the
setAnimationMode
method.
Here is an example of how you might customize Material-ViewPagerIndicator:
java
// Get a reference to your ViewPagerIndicator
final ViewPagerIndicator viewPagerIndicator = findViewById(R.id.view_pager_indicator);
// Set custom colors for selected and unselected dots
viewPagerIndicator.setDotColor(Color.RED);
viewPagerIndicator.setSelectedDotColor(Color.GREEN);
// Set custom size for dots
viewPagerIndicator.setDotSize(20);
// Set custom spacing between dots
viewPagerIndicator.setDotSpacing(8);
// Set custom animation mode
viewPagerIndicator.setAnimationMode(ViewPagerIndicator.AnimationMode.SLIDE);
vejei/ViewPagerIndicator Customization
vejei/ViewPagerIndicator also provides several customization options. Here are some key customization options:
- Animation Mode: Change the animation mode using the
setAnimationMode
method. - Item Count: Set the item count manually using the
setItemCount
method. - Indicator Type: Change the type of indicator (e.g., circle or line) using different classes like
CircleIndicator
orLineIndicator
.
Here is an example of how you might customize vejei/ViewPagerIndicator:
java
// Get a reference to your CircleIndicator
final CircleIndicator circleIndicator = findViewById(R.id.circle_indicator);
// Set custom animation mode
circleIndicator.setAnimationMode(CircleIndicator.AnimationMode.SLIDE);
// Set custom item count manually (optional)
circleIndicator.setItemCount(5);
// Set custom colors for selected and unselected dots (optional)
circleIndicator.setSelectedDotColor(Color.GREEN);
circleIndicator.setUnselectedDotColor(Color.RED);
Feature Overview
ViewPagerIndicator enhances user navigation in Android apps by providing visual indicators for different pages. These indicators help users know their current position within a series of pages. Key functionalities include customizable styles, smooth animations, and easy integration with ViewPager. It supports various indicator types like dots, lines, and tabs, making it versatile for different app designs.
Requirements and Compatibility
To use ViewPagerIndicator on your Android device, ensure your device meets these requirements:
- Android Version: Your device must run Android 4.0 (Ice Cream Sandwich) or higher. Devices with older versions won't support this feature.
- RAM: At least 1GB of RAM is necessary for smooth operation. Less memory might cause lag or crashes.
- Storage: Ensure you have at least 50MB of free storage. This space is needed for the app and its dependencies.
- Screen Resolution: A minimum screen resolution of 800x480 pixels is required. Lower resolutions may not display the indicators correctly.
- Processor: A device with a dual-core processor or better is recommended. Single-core processors may struggle with performance.
- Google Play Services: Ensure your device has the latest version of Google Play Services installed. This ensures compatibility with various libraries.
- Permissions: The app will need permissions to access the internet, read/write storage, and possibly location services. Make sure to grant these permissions for full functionality.
Check these requirements to ensure your device can support ViewPagerIndicator without any issues. If your device meets all these criteria, you should be good to go!
Setting Up ViewPagerIndicator
Open Android Studio and load your project.
Navigate to the
build.gradle
file (Module: app).Add the dependency for ViewPagerIndicator: groovy implementation 'com.viewpagerindicator:library:2.4.1'
Sync your project by clicking "Sync Now" in the top right corner.
Open your layout file (e.g.,
activity_main.xml
).Add the ViewPager and ViewPagerIndicator to your layout: xml <androidx.viewpager.widget.ViewPager android:id="@+id/viewPager" android:layout_width="match_parent" android:layout_height="match_parent" /> <com.viewpagerindicator.CirclePageIndicator android:id="@+id/indicator" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" />
Open your Activity file (e.g.,
MainActivity.java
orMainActivity.kt
).Initialize the ViewPager and set its adapter: java ViewPager viewPager = findViewById(R.id.viewPager); MyPagerAdapter adapter = new MyPagerAdapter(getSupportFragmentManager()); viewPager.setAdapter(adapter);
Initialize the ViewPagerIndicator and link it to the ViewPager: java CirclePageIndicator indicator = findViewById(R.id.indicator); indicator.setViewPager(viewPager);
Run your app to see the ViewPager with the indicator working.
Done!
Effective Use of ViewPagerIndicator
Use Fragments: When implementing ViewPagerIndicator, fragments make managing different pages easier. Each fragment can represent a distinct screen or section.
Keep it Simple: Avoid overloading each page with too much content. Simple, focused pages improve user experience.
Customize Indicators: Tailor the indicator style to match your app's theme. Change colors, shapes, or animations to fit your design.
Smooth Transitions: Ensure smooth animations between pages. Users appreciate fluid transitions.
Lazy Loading: Load content only when needed. This saves memory and speeds up the app.
Test on Multiple Devices: Check how the ViewPagerIndicator looks and performs on various screen sizes and orientations. Consistency is key.
User Feedback: Provide visual feedback when users interact with the ViewPager. Highlight the current page or use progress indicators.
Accessibility: Make sure your ViewPagerIndicator is accessible. Use descriptive labels for screen readers.
Optimize Performance: Keep an eye on performance. Optimize images and other resources to prevent lag.
Documentation: Keep your code well-documented. Future you or other developers will appreciate clear comments and structure.
Troubleshooting Common Issues
Common issues with ViewPagerIndicator include incorrect setup, missing dependencies, and layout problems.
Incorrect Setup: Ensure the ViewPager and ViewPagerIndicator are correctly linked. Use
viewPager.setAdapter(adapter)
andindicator.setViewPager(viewPager)
.Missing Dependencies: Add the necessary library in your
build.gradle
file: gradle implementation 'com.github.JakeWharton:ViewPagerIndicator:2.4.1'Layout Problems: Check XML layout for proper placement. Example: xml <androidx.viewpager.widget.ViewPager android:id="@+id/viewPager" android:layout_width="match_parent" android:layout_height="match_parent"/> <com.viewpagerindicator.CirclePageIndicator android:id="@+id/indicator" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="bottom"/>
Adapter Issues: Ensure the adapter provides the correct number of items and handles data properly. Use
notifyDataSetChanged()
if data changes.Compatibility: Verify compatibility with your Android version and other libraries. Update dependencies if needed.
Custom Indicators: If using custom indicators, ensure drawable resources are correctly referenced and sized.
Performance: For large datasets, consider using
FragmentStatePagerAdapter
instead ofFragmentPagerAdapter
to save memory.Debugging: Use log statements to check if methods like
getCount()
andinstantiateItem()
are called as expected.
By following these steps, most ViewPagerIndicator issues can be resolved efficiently.
Security and Privacy Tips
When using ViewPagerIndicator in Android Studio, user data handling becomes crucial. Always ensure data encryption during transmission and storage. Avoid storing sensitive information directly on the device. Use Android's built-in security features like KeyStore for managing cryptographic keys. Regularly update your app to patch security vulnerabilities.
For maintaining privacy, limit permissions requested by the app. Only ask for what's necessary. Implement user consent mechanisms for data collection. Use anonymous identifiers instead of personal data whenever possible. Regularly review and comply with privacy policies and regulations like GDPR or CCPA.
Comparing Alternatives
Pros of ViewPagerIndicator:
- Easy integration with Android Studio
- Customizable indicators
- Smooth transitions between pages
- Supports various indicator styles
Cons of ViewPagerIndicator:
- Limited to Android platform
- Requires additional setup
- May not support all custom designs
Alternatives:
iOS Page Control:
- Built-in support for iOS
- Simple to use
- Limited customization options
React Native Pager View:
- Cross-platform support
- Easy to implement
- Requires React Native knowledge
Flutter PageView:
- Cross-platform compatibility
- Highly customizable
- Requires Flutter framework knowledge
Final Thoughts on ViewPagerIndicator
ViewPagerIndicator simplifies navigation in Android apps. It enhances user experience by providing clear, intuitive indicators for swiping between pages. Integrating it into your project is straightforward, requiring minimal code changes. Customization options allow you to match the indicator's appearance with your app's design, ensuring a seamless look and feel.
Performance remains smooth, even with multiple pages, making it suitable for various applications. Developers benefit from its flexibility and ease of use, reducing development time and effort.
Incorporating ViewPagerIndicator can significantly improve your app's navigation, making it more user-friendly and visually appealing. Whether you're building a new app or updating an existing one, this tool is a valuable addition to your development toolkit. Give it a try and see the difference it makes in your app's usability and overall user satisfaction.
How do I integrate ViewPagerIndicator in Android Studio?
Add the ViewPagerIndicator library to your project by including it in your build.gradle
file. Then, set up your ViewPager and link it with the ViewPagerIndicator.
What dependencies are needed for ViewPagerIndicator?
You need to include the ViewPagerIndicator library in your build.gradle
file. Typically, it looks something like implementation 'com.viewpagerindicator:library:2.4.1'
.
Can I customize the ViewPagerIndicator?
Yes, you can customize the appearance and behavior of the ViewPagerIndicator. You can change colors, sizes, and even the type of indicator (like circles, lines, etc.) through XML attributes or programmatically.
How do I link ViewPager with ViewPagerIndicator?
First, set up your ViewPager with a PagerAdapter. Then, initialize your ViewPagerIndicator and call setViewPager()
on it, passing your ViewPager as the parameter.
Is ViewPagerIndicator compatible with ViewPager2?
ViewPagerIndicator was initially designed for the original ViewPager. For ViewPager2, you might need to look for updated libraries or workarounds, as direct support may not be available.
What are some common issues with ViewPagerIndicator?
Common issues include incorrect linking with ViewPager, customization problems, and compatibility issues with newer Android versions or ViewPager2. Always check for library updates and community forums for solutions.
Can I use ViewPagerIndicator with fragments?
Absolutely! You can use ViewPagerIndicator with fragments by setting up your ViewPager with a FragmentPagerAdapter or FragmentStatePagerAdapter. Then, link it with the ViewPagerIndicator as usual.