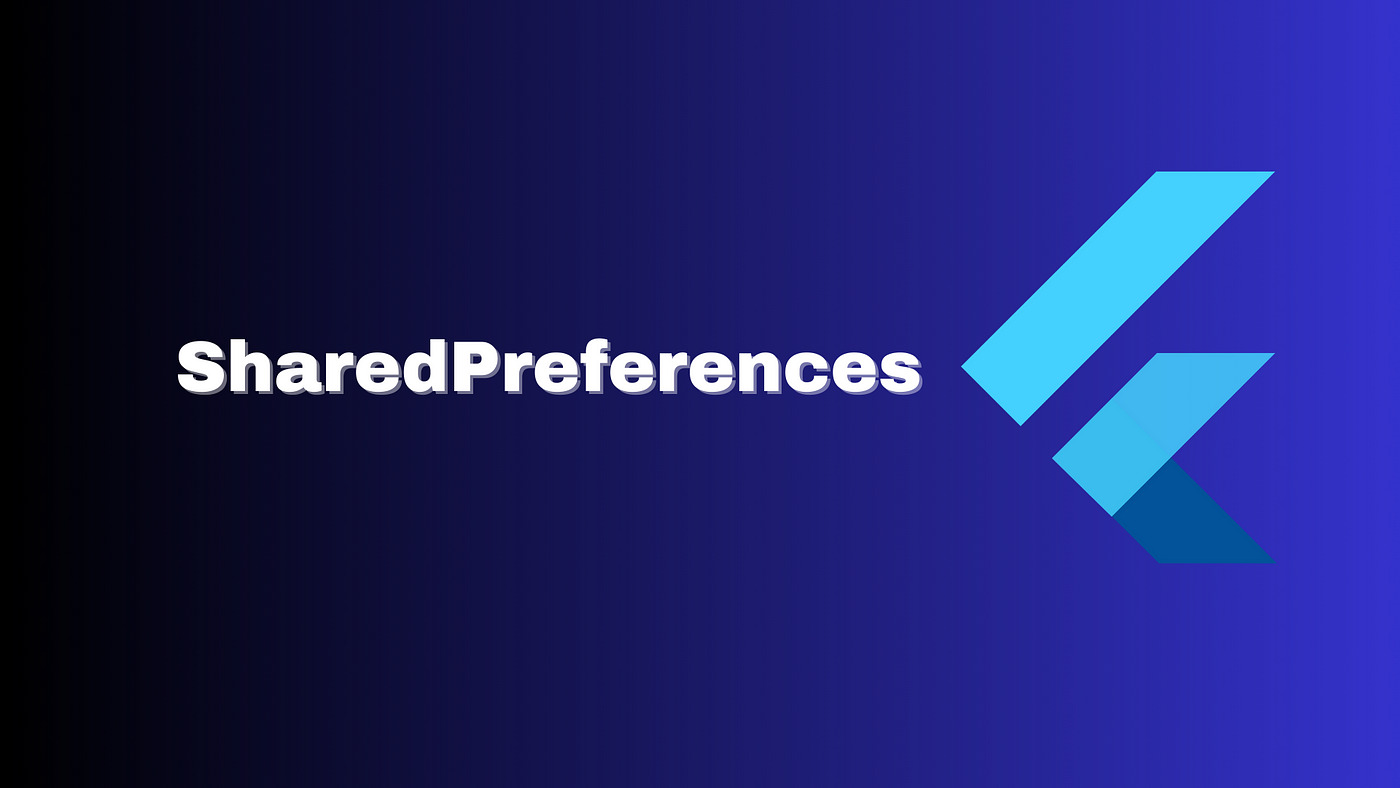
Introduction to SharedPreferences
SharedPreferences is a fundamental component in Android development, used to store and retrieve small amounts of data in the form of key-value pairs. This data persistence mechanism is crucial for managing user preferences, settings, and other application-specific configurations.
Key Features of SharedPreferences
- Key-Value Pairs: Stores data in the form of key-value pairs, making it easy to manage and retrieve specific pieces of information.
- Persistence: Data stored in SharedPreferences persists even when the application is closed, making it a reliable choice for long-term storage.
- Security: By default, SharedPreferences files are private to the application, ensuring that sensitive data is not accessible to other applications.
- Synchronization: Modifications to the preferences are committed asynchronously by default, which helps avoid blocking the main thread during lifecycle transitions.
Accessing SharedPreferences
To access SharedPreferences in an Android application, you can use one of two primary methods:
Using getSharedPreferences()
This method allows you to create a new shared preference file or access an existing one by specifying the name of the file and the mode.
java
SharedPreferences pref = getApplicationContext().getSharedPreferences("MyPref", 0); // 0 – for private mode
The getSharedPreferences()
method is versatile and can be called from any context in your app. It is particularly useful when you need multiple shared preference files identified by name.
Using getPreferences()
This method is specifically designed for activities and allows you to use only one shared preference file for the activity. Since it retrieves a default shared preference file that belongs to the activity, you do not need to supply a name.
java
SharedPreferences sharedPref = getActivity().getPreferences(Context.MODE_PRIVATE);
Using getPreferences()
is convenient when you only need a single preference file for your activity.
Best Practices for Using SharedPreferences
Avoiding Key Duplication
One common pitfall when using SharedPreferences is key duplication. If multiple developers are working on the same project and define SharedPreferences with the same name or insert equivalent key-value pairs, it can lead to conflicts and make it difficult to manage the data.
To avoid this issue, use a SharedPreferences singleton that uses string keys or enum keys. Enum keys are particularly useful as they enforce stricter control and ensure that all keys are in the same place, making it easier to manage and maintain.
Using Unique File Names
When naming your shared preference files, it is essential to use a name that is uniquely identifiable to your app. A good practice is to prefix the file name with your application ID. For example:
java
"com.example.myapp.PREFERENCE_FILE_KEY"
This helps prevent conflicts with other applications or libraries that might use the same preference names.
Accessing SharedPreferences Off the UI Thread
Accessing SharedPreferences should be done off the UI thread to avoid blocking the main thread. This is particularly important when using commit()
or apply()
methods, as these operations can pause your UI rendering if performed on the main thread.
Using apply()
vs commit()
Both apply()
and commit()
can be used to save changes to SharedPreferences. However, there are differences in how they handle data persistence:
apply()
: Writes the updates to disk asynchronously. It is generally recommended for use in the main thread because it does not block the UI rendering.commit()
: Writes the data to disk synchronously. It should be avoided on the main thread as it can pause your UI rendering.
Writing to SharedPreferences
To write data to SharedPreferences, create a SharedPreferences.Editor
object by calling edit()
on your SharedPreferences
.
java
SharedPreferences.Editor editor = pref.edit();
editor.putString("key", "value");
editor.commit(); // or editor.apply()
Use various methods like putInt()
, putString()
, etc., to pass the keys and values. After making the necessary changes, commit or apply them using either commit()
or apply()
.
Reading from SharedPreferences
To retrieve values from SharedPreferences, call methods like getInt()
, getString()
, etc., providing the key for the value you want.
java
String value = pref.getString("key", "");
int intValue = pref.getInt("key", 0);
These methods return the stored value or a default value if the key is not found.
Example Implementation
Let's assume you want to create an application that stores user-specific settings like name and email. Here’s an example implementation:
java
public class MainActivity extends AppCompatActivity {
private static final String MY_PREFS_FILENAME = "MyPrefsFile";
private static final String NAME_KEY = "nameKey";
private static final String EMAIL_KEY = "emailKey";
private SharedPreferences sharedpreferences;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
sharedpreferences = getSharedPreferences(MY_PREFS_FILENAME, Context.MODE_PRIVATE);
// Initialize UI components
name = findViewById(R.id.etName);
email = findViewById(R.id.etEmail);
// Load saved preferences
if (sharedpreferences.contains(NAME_KEY)) {
name.setText(sharedpreferences.getString(NAME_KEY, ""));
}
if (sharedpreferences.contains(EMAIL_KEY)) {
email.setText(sharedpreferences.getString(EMAIL_KEY, ""));
}
}
public void Save(View view) {
String n = name.getText().toString();
String e = email.getText().toString();
SharedPreferences.Editor editor = sharedpreferences.edit();
editor.putString(NAME_KEY, n);
editor.putString(EMAIL_KEY, e);
editor.commit(); // or editor.apply()
}
public void clear(View view) {
name.setText("");
email.setText("");
}
public void Get(View view) {
// Load saved preferences
if (sharedpreferences.contains(NAME_KEY)) {
name.setText(sharedpreferences.getString(NAME_KEY, ""));
}
if (sharedpreferences.contains(EMAIL_KEY)) {
email.setText(sharedpreferences.getString(EMAIL_KEY, ""));
}
}
}
In this example, getSharedPreferences()
is used to access the shared preferences file and store user-specific settings like name and email. The Save
method updates these settings using a SharedPreferences.Editor
, while the Get
method retrieves and displays the saved values.
Additional Considerations
- Security: While SharedPreferences provides a level of security by default, it is not recommended for storing sensitive data like user security or privacy settings. For such cases, consider using more secure data storage options like Room or DataStore.
- Performance: Be mindful of the number of operations performed on SharedPreferences, especially when using
commit()
orapply()
. These operations can lead to blocking the main thread during lifecycle transitions, potentially causing ANR errors.
By mastering SharedPreferences, you can ensure that your Android applications are robust, efficient, and user-friendly, providing a seamless experience for your users.