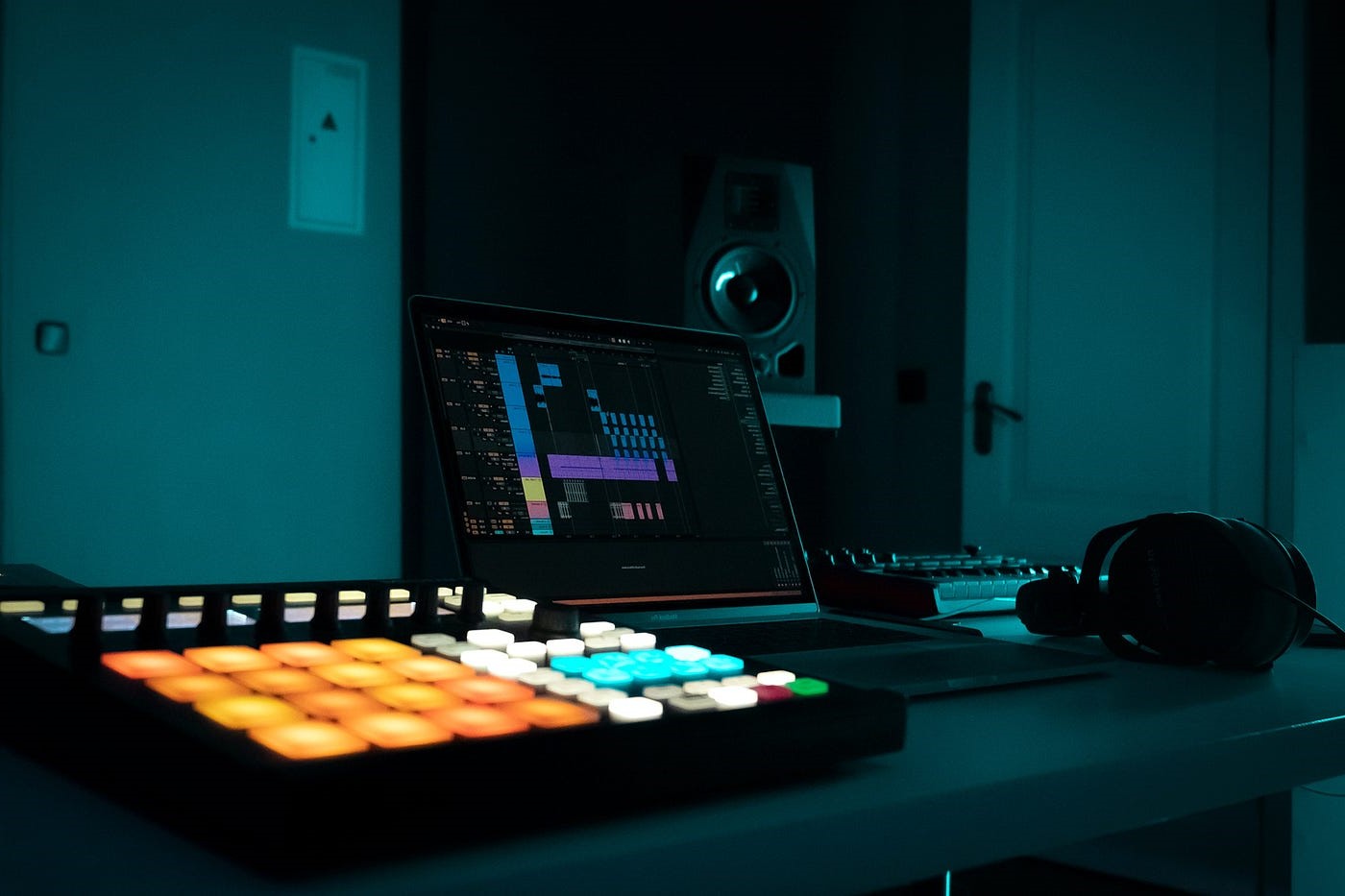
Introduction to Kotlin for Android Development
Why Kotlin for Android?
Kotlin has become a favorite for Android developers for several reasons. First off, it's concise. You can accomplish more with fewer lines of code compared to Java, which means less room for errors and easier maintenance. Kotlin is also safe. Its null safety feature helps prevent the dreaded NullPointerException, making your apps more reliable. Plus, Kotlin is interoperable with Java, so you can use both languages in the same project without any issues. This makes it easier to gradually migrate existing Java codebases to Kotlin.
Google's Endorsement
Google's backing of Kotlin for Android development is a big deal. In 2017, Google announced Kotlin as an official language for Android development. This endorsement means that Kotlin is well-supported and integrated into the Android ecosystem. Google has even made many of its own libraries Kotlin-friendly, ensuring that developers have a smooth experience. This support from Google has led to a growing community and a wealth of resources, making it easier for new developers to get started.
Kotlin vs. Java
When comparing Kotlin to Java, several differences stand out. Syntax is one of the most noticeable. Kotlin's syntax is more modern and expressive, which can make the code easier to read and write. For example, Kotlin reduces boilerplate code, meaning you write less to achieve the same functionality. In terms of performance, Kotlin is just as fast as Java, as it runs on the Java Virtual Machine (JVM). However, developer productivity is where Kotlin really shines. Features like type inference, extension functions, and coroutines make development faster and more enjoyable.
Key Takeaways:
- Kotlin makes Android app development easier and safer with fewer lines of code, preventing common errors like crashes from null values.
- Google's support for Kotlin means lots of helpful tools and resources, making it a great choice for building Android apps.
Setting Up Your Development Environment
Installing Android Studio
To start developing with Kotlin, you'll need to install Android Studio. First, go to the Android Studio website and download the installer for your operating system. Run the installer and follow the prompts to complete the installation. Once installed, open Android Studio and follow the setup wizard to configure your development environment. This includes downloading the necessary SDK components and setting up an emulator for testing your apps.
Configuring Kotlin in Android Studio
After installing Android Studio, you need to enable Kotlin support. Open Android Studio and create a new project. During the project setup, you'll see an option to include Kotlin support. Make sure to check this box. If you're adding Kotlin to an existing project, go to File > New > Kotlin File/Class
and follow the prompts to configure Kotlin in your project. Android Studio will automatically download the necessary Kotlin plugins and set up your project to use Kotlin.
Creating Your First Kotlin Project
Creating a new Kotlin project in Android Studio is straightforward. Open Android Studio and select Start a new Android Studio project
. Choose a project template, like Empty Activity
, and click Next
. Fill in the project details, such as the name and save location, and make sure the Include Kotlin support
box is checked. Click Finish
to create your project. Android Studio will generate the necessary files and folders, and you'll be ready to start coding in Kotlin.
Core Kotlin Features for Android Development
Null Safety
Kotlin's null safety feature is a game-changer for Android developers. In many programming languages, null pointer exceptions are a common headache. Kotlin tackles this issue head-on by distinguishing between nullable and non-nullable types.
For instance, if you declare a variable as var name: String
, it can't be null. If you want it to be nullable, you'd write var name: String?
. This simple addition of a question mark helps prevent many runtime errors. Kotlin forces you to handle null cases explicitly, making your code more robust and less prone to crashes.
Extension Functions
Extension functions allow you to add new functionality to existing classes without modifying their source code. This feature can make your code cleaner and more readable.
Imagine you want to add a function to the String
class to capitalize the first letter of a string. In Kotlin, you can do this easily:
kotlin
fun String.capitalizeFirstLetter(): String {
return this.substring(0, 1).uppercase() + this.substring(1)
}
Now, you can call capitalizeFirstLetter
on any string object. This makes your code more intuitive and easier to maintain.
Coroutines for Asynchronous Programming
Handling asynchronous tasks in Android can be tricky, but coroutines simplify this process. Coroutines allow you to write asynchronous code in a sequential manner, making it easier to read and maintain.
To start a coroutine, you use the launch
function from the kotlinx.coroutines
library:
kotlin
import kotlinx.coroutines.*
fun main() = runBlocking {
launch {
delay(1000L)
println("Hello, Coroutines!")
}
println("Start")
}
In this example, "Start" prints first, then after a one-second delay, "Hello, Coroutines!" prints. Coroutines help you manage background tasks like network requests or database operations without blocking the main thread.
Building a Basic Android App with Kotlin
Designing the User Interface
Creating a user interface (UI) in Android involves using XML files to define the layout. Here's a simple example of an XML layout file:
xml
<TextView
android:id="@+id/textView"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Hello, World!" />
<Button
android:id="@+id/button"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Click Me" />
In your Kotlin activity file, you can reference these UI elements and set up event listeners.
Handling User Input
Managing user input in Kotlin is straightforward. Suppose you have a button and a text view in your layout. You can set up an OnClickListener
for the button to change the text view's text:
kotlin
button.setOnClickListener {
textView.text = "Button Clicked!"
}
This snippet changes the text of textView
when button
is clicked. Handling user input becomes intuitive with Kotlin's concise syntax.
Connecting to a Backend
Making network requests in Kotlin is often done using libraries like Retrofit. Here's a basic example of how to set up Retrofit to make a GET request:
kotlin
interface ApiService {
@GET("endpoint")
suspend fun getData(): Response
}
val retrofit = Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build()
val service = retrofit.create(ApiService::class.java)
GlobalScope.launch {
val response = service.getData()
if (response.isSuccessful) {
val data = response.body()
// Handle the data
}
}
This code sets up a Retrofit instance, defines an API service, and makes a network request. Using coroutines, you can handle the response asynchronously without blocking the main thread.
Advanced Kotlin Techniques for Android
Using Kotlin Libraries
Kotlin has a bunch of libraries that make Android development easier and more fun. Koin, Retrofit, and Room are some of the most popular ones. Koin helps with dependency injection, making your code cleaner and more modular. Retrofit simplifies network requests, letting you fetch data from the internet without much hassle. Room handles local database management, so you can store data on the device efficiently.
Dependency Injection with Koin
Dependency injection might sound fancy, but it just means providing objects that a class needs, rather than letting the class create them itself. Koin makes this super easy in Kotlin. You define your dependencies in a module, and Koin takes care of the rest. This approach keeps your code neat and testable, as you can easily swap out dependencies when needed.
Database Management with Room
Room is a powerful library for managing local databases in Android apps. It abstracts away much of the boilerplate code needed for database operations. To use Room, you define your database schema using annotations, and Room generates the necessary code for you. This makes database interactions straightforward and less error-prone.
Testing and Debugging Kotlin Code
Unit Testing with JUnit
JUnit is a framework for writing and running tests in Kotlin. Unit tests check if individual parts of your code work as expected. To write a unit test, you create a test class and annotate methods with @Test
. Running these tests helps catch bugs early, ensuring your code behaves correctly.
UI Testing with Espresso
Espresso is a tool for testing your app's user interface. It simulates user interactions, like tapping buttons or entering text, to verify that your app responds correctly. Writing UI tests with Espresso involves creating test cases that mimic real user behavior, helping ensure a smooth user experience.
Debugging Tips and Tricks
Debugging is an essential skill for any developer. In Android Studio, you can set breakpoints in your code to pause execution and inspect variables. The Logcat tool shows real-time logs from your app, helping you track down issues. Additionally, using the debugger to step through your code line by line can reveal hidden bugs and logic errors.
Wrapping Up Kotlin for Android Development
Kotlin's rise in Android development isn't just hype; it's a game-changer for developers. With concise syntax, null safety, and Java interoperability, Kotlin makes coding both fun and efficient. Google's endorsement has cemented its place in the Android ecosystem, offering robust support and resources. Setting up your environment in Android Studio is a breeze, and features like extension functions and coroutines make complex tasks simpler. Advanced techniques using libraries like Koin and Room streamline dependency injection and database management. Whether you're handling user input or debugging, Kotlin's got your back, making it a must-learn language for anyone diving into Android development.
Understanding the Basics
This feature simplifies Android app development by using Kotlin. It offers concise syntax, null safety, and coroutines for asynchronous programming. Developers can write cleaner code, reduce boilerplate, and improve app performance. The feature integrates seamlessly with existing Java code, allowing gradual adoption. It also supports extension functions, making code more readable and maintainable.
What You Need to Get Started
To ensure your device supports the feature, check these requirements:
- Operating System: Your device must run Android 5.0 (Lollipop) or higher. Older versions won't support the latest features.
- RAM: At least 2GB of RAM is necessary for smooth performance. Less RAM might cause lag or crashes.
- Storage: Ensure you have at least 500MB of free storage. This space is needed for app installation and updates.
- Processor: A Quad-core processor or better is recommended. Slower processors may struggle with complex tasks.
- Screen Resolution: A minimum resolution of 720p (1280x720) ensures the app displays correctly. Lower resolutions might distort the interface.
- Google Play Services: Your device must have Google Play Services installed and updated. This is crucial for app functionality.
- Internet Connection: A stable Wi-Fi or mobile data connection is required for downloading and using online features.
- Bluetooth: If the feature involves connectivity, ensure your device supports Bluetooth 4.0 or higher.
- GPS: For location-based features, your device needs a functional GPS module.
- Camera: If the app uses camera functions, a 5MP camera or better is recommended for clear images.
Check these specs to confirm compatibility.
Getting Everything Ready
Install Android Studio: Download from the official website. Follow the installation steps.
Open Android Studio: Launch the program after installation.
Start a New Project: Click on "Start a new Android Studio project."
Choose a Template: Select "Empty Activity" and hit "Next."
Name Your Project: Enter a name, like "MyFirstApp." Set the save location.
Select Language: Choose Kotlin from the dropdown menu.
Set Minimum API Level: Pick the lowest Android version you want to support.
Finish Setup: Click "Finish" to create the project.
Wait for Gradle Build: Let Android Studio set up your project. This might take a few minutes.
Open MainActivity.kt: Navigate to
app > java > your.package.name > MainActivity.kt
.Edit Layout: Go to
app > res > layout > activity_main.xml
. Add UI elements like buttons or text views.Write Code: In
MainActivity.kt
, add code to handle user interactions.Run Your App: Click the green play button. Choose an emulator or connected device.
Test Your App: Interact with your app on the emulator or device.
Debug if Needed: Use the logcat window to find and fix issues.
Save and Commit: Save your work. Use version control like Git to commit changes.
Congratulations! You've set up your first Android app with Kotlin.
Tips for Best Results
Start simple: Begin with basic apps to grasp Kotlin syntax. Create a "Hello World" app to understand the structure.
Use Android Studio: This IDE is tailored for Android development. It offers code completion, debugging tools, and emulators.
Leverage Kotlin's features: Utilize null safety to avoid null pointer exceptions. Implement data classes for storing data without boilerplate code.
Follow MVVM architecture: Model-View-ViewModel separates concerns, making code more manageable. ViewModel handles UI-related data, Model manages data logic, and View displays data.
Utilize Coroutines: For asynchronous programming, coroutines simplify code. They help manage background tasks without blocking the main thread.
Test your code: Write unit tests using JUnit and Espresso for UI tests. Testing ensures your app works as expected.
Optimize performance: Use profiling tools in Android Studio to monitor memory usage and CPU performance. Optimize layouts and reduce unnecessary operations.
Stay updated: Follow Android development blogs and forums. Google's Android Developer Blog and Stack Overflow are great resources.
Use libraries: Libraries like Retrofit for networking, Room for database management, and Glide for image loading can save time and effort.
Keep UI/UX in mind: Design intuitive and responsive interfaces. Follow Material Design guidelines for a consistent look and feel.
Document your code: Write clear comments and documentation. This helps others understand your code and makes maintenance easier.
Backup your work: Use version control systems like Git. Platforms like GitHub or Bitbucket keep your code safe and allow collaboration.
Seek feedback: Share your app with others for testing. User feedback can highlight areas for improvement you might have missed.
Troubleshooting Tips
App Crashes on Launch: Check for any missing permissions in the manifest file. Ensure all necessary libraries are included. Use logcat to identify errors.
Slow Performance: Optimize your code by avoiding unnecessary operations on the main thread. Use background threads for heavy tasks. Implement caching where possible.
UI Not Updating: Verify that UI updates are happening on the main thread. Use
runOnUiThread
orHandler
for UI changes.Memory Leaks: Use tools like Android Profiler to detect memory leaks. Avoid holding references to activities or views in long-lived objects.
Network Issues: Ensure proper permissions for internet access are set. Use libraries like Retrofit for efficient network calls. Implement retry logic for failed requests.
Inconsistent Layouts: Use ConstraintLayout for more flexible and responsive designs. Test on multiple screen sizes and orientations.
App Not Installing: Check for conflicting package names. Ensure the device has enough storage. Verify that the APK is signed correctly.
Database Errors: Use Room for database management. Ensure proper migrations are in place when altering database schemas.
Push Notifications Not Working: Verify Firebase setup and ensure the device has internet access. Check for correct API keys and permissions.
Battery Drain: Optimize background services and limit their usage. Use JobScheduler or WorkManager for background tasks. Reduce the frequency of location updates.
Keeping Your Data Safe
When using this feature, user data is collected and stored securely. Encryption ensures that sensitive information remains safe from unauthorized access. To maintain privacy, avoid sharing personal details unnecessarily. Regularly update your device and apps to patch any security vulnerabilities. Use strong passwords and enable two-factor authentication for added protection. Be cautious of phishing attempts and only download apps from trusted sources. Adjust privacy settings to control what information is shared. Always log out from shared devices to prevent unauthorized access.
Other Options to Consider
Pros of Android App Development with Kotlin:
- Interoperability: Works seamlessly with Java.
- Concise Syntax: Reduces boilerplate code.
- Null Safety: Helps avoid null pointer exceptions.
- Coroutines: Simplifies asynchronous programming.
- Community Support: Strong backing from Google and developers.
Cons of Android App Development with Kotlin:
- Learning Curve: Can be steep for beginners.
- Compilation Speed: Sometimes slower than Java.
- Limited Resources: Fewer tutorials compared to Java.
- Tooling Issues: Occasional bugs in IDE support.
- Library Support: Some libraries still Java-centric.
Alternatives:
Java:
- Pros: Mature, extensive resources, robust libraries.
- Cons: Verbose syntax, prone to null pointer exceptions.
Flutter (Dart):
- Pros: Cross-platform, fast development, rich UI components.
- Cons: Larger app size, less mature than native solutions.
React Native (JavaScript):
- Pros: Cross-platform, large community, reusable code.
- Cons: Performance issues, complex debugging.
Swift (for iOS):
- Pros: Modern syntax, fast performance, strong Apple support.
- Cons: Limited to iOS, smaller community compared to Android.
Xamarin (C#):
- Pros: Cross-platform, strong Microsoft support, native performance.
- Cons: Larger app size, less popular than other frameworks.
App Crashes on Launch: Check for any missing permissions in the manifest file. Ensure all necessary libraries are included. Use logcat to identify errors.
Slow Performance: Optimize your code by avoiding unnecessary operations on the main thread. Use background threads for heavy tasks. Implement caching where possible.
UI Not Updating: Verify that UI updates are happening on the main thread. Use
runOnUiThread
orHandler
for UI changes.Memory Leaks: Use tools like Android Profiler to detect memory leaks. Avoid holding references to activities or views in long-lived objects.
Network Issues: Ensure proper permissions for internet access are set. Use libraries like Retrofit for efficient network calls. Implement retry logic for failed requests.
Inconsistent Layouts: Use ConstraintLayout for more flexible and responsive designs. Test on multiple screen sizes and orientations.
App Not Installing: Check for conflicting package names. Ensure the device has enough storage. Verify that the APK is signed correctly.
Database Errors: Use Room for database management. Ensure proper migrations are in place when altering database schemas.
Push Notifications Not Working: Verify Firebase setup and ensure the device has internet access. Check for correct API keys and permissions.
Battery Drain: Optimize background services and limit their usage. Use JobScheduler or WorkManager for background tasks. Reduce the frequency of location updates.
Android App Development with Kotlin
Kotlin makes Android app development smoother and more efficient. Its concise syntax reduces boilerplate code, making your apps cleaner and easier to maintain. Plus, Kotlin's interoperability with Java means you can gradually migrate existing projects without a complete overhaul. The language's null safety features help prevent common runtime errors, boosting your app's reliability. With a growing community and strong support from Google, Kotlin is becoming the go-to choice for Android developers. Dive into Kotlin, and you'll find yourself building robust, high-performance apps in no time. Whether you're a seasoned developer or just starting, Kotlin offers tools and features that simplify the development process. Embrace Kotlin for your next project, and experience the benefits firsthand.
Is Kotlin the best for Android app development?
Kotlin is an ideal language for Android app development with a long list of features for creating great apps. Its compatibility with Java and its interoperability are two main benefits for mobile app developers. The language also offers many features and libraries, enabling developers to build powerful apps quickly.
Can I make Android apps using Kotlin?
Develop Android apps with Kotlin. Write better Android apps faster with Kotlin. Kotlin is a modern statically typed programming language used by over 60% of professional Android developers that helps boost productivity, developer satisfaction, and code safety.
Should I learn Kotlin or Flutter for Android development?
Flutter appeals to developers who want to target multiple platforms with a single codebase. Kotlin is preferred by those who are developing exclusively for Android but want a more modern and expressive language than Java.
Is Kotlin now Google's preferred language for Android app development?
At Google I/O 2019, we announced that Android development will be increasingly Kotlin-first, and we've stood by that commitment. Kotlin is an expressive and concise programming language that reduces common code errors and easily integrates into existing apps.
What are the benefits of using Kotlin over Java for Android development?
Kotlin offers several advantages over Java, including null safety, extension functions, and more concise syntax. These features help reduce boilerplate code and minimize errors, making development faster and more enjoyable.
How easy is it to switch from Java to Kotlin for Android development?
Switching from Java to Kotlin is relatively straightforward. Kotlin is fully interoperable with Java, so you can gradually introduce Kotlin into your existing projects. Plus, there are many resources and tools available to help you make the transition smoothly.
Are there any good resources for learning Kotlin for Android development?
Absolutely! There are plenty of online courses, tutorials, and documentation available to help you learn Kotlin. Websites like Kotlinlang.org, Udacity, and Coursera offer comprehensive guides and courses tailored for Android development.