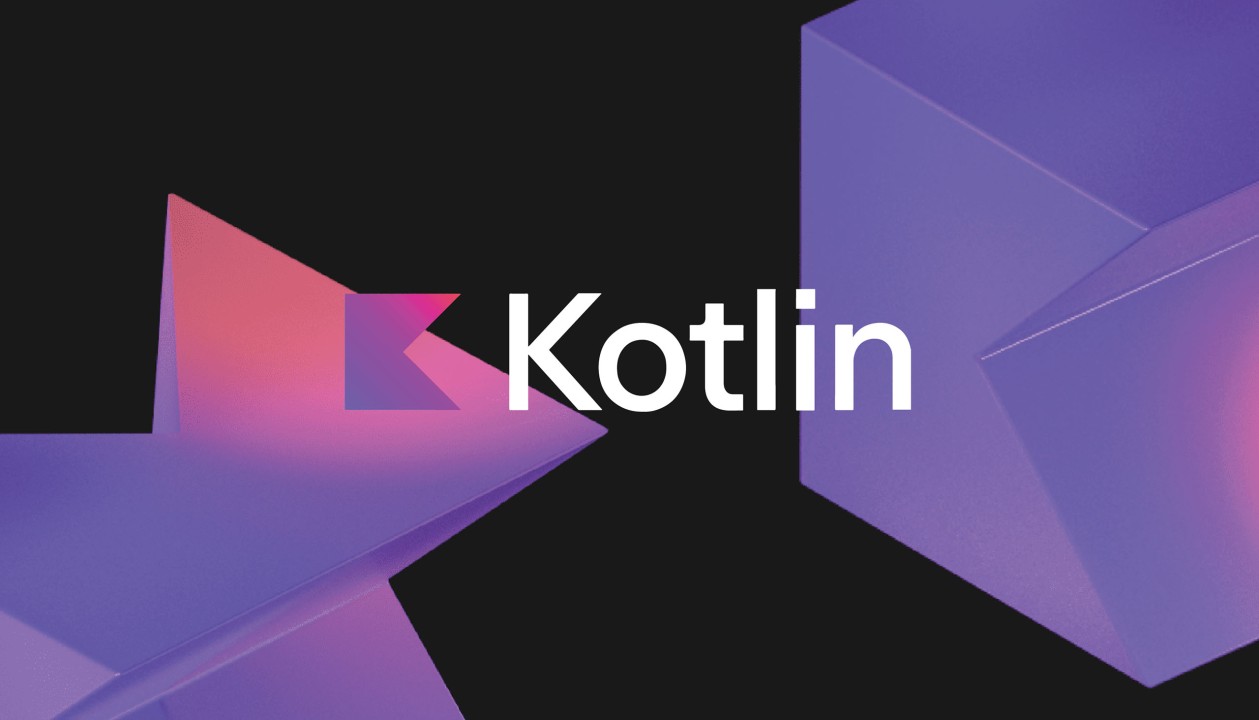
Introduction to Kotlin 2.0
Kotlin, a modern programming language developed by JetBrains, has been gaining significant traction in the software development community. Its concise syntax, null safety features, and multiplatform capabilities make it an attractive choice for developers. The latest version, Kotlin 2.0, brings numerous enhancements and improvements that further solidify its position as a top-tier programming language.
Key Features and Updates
K2 Compiler
The K2 compiler is a major component of Kotlin 2.0, designed to provide faster compilation times and better performance compared to its predecessor. Available in both Beta and Stable modes, it offers developers flexibility based on their specific needs.
Performance Improvements
- Faster Compilation: Optimized for speed, the K2 compiler allows quicker code compilation, especially useful during rapid iteration phases.
- Better Optimization: Advanced techniques reduce the size of compiled code and improve execution speed.
- Stability Enhancements: Stabilizes language features, reducing runtime errors and improving overall application stability.
Multiplatform Support
Kotlin has always been known for its multiplatform capabilities, allowing code to run on JVM, Native, JavaScript, and WebAssembly. Kotlin 2.0 enhances this support with better integration with various frameworks and libraries.
Multiplatform Integration
- Seamless Integration: Works seamlessly with frameworks like Gradle and other build tools, simplifying dependency and configuration management.
- Unified Standard Library: More stable and universal across platforms, reducing the need for platform-specific code and improving code reusability.
- Compose Multiplatform: Updated to support iOS in Beta and Web in Alpha, enabling the creation of UI components that run on multiple platforms.
Gradle Improvements
Gradle, a popular build tool, sees several enhancements in Kotlin 2.0, making it easier to manage complex projects.
New Gradle DSL
A new Gradle DSL for compiler options in multiplatform projects offers a more concise and expressive way to configure compiler settings, reducing build configuration complexity.
New Compose Compiler Gradle Plugin
This plugin simplifies the integration of Compose with Gradle, automatically configuring the Compose compiler version based on the current Kotlin version, eliminating manual configuration.
Bumping Minimum Supported Versions
Kotlin 2.0 bumps the minimum supported versions of Gradle and the Android Gradle plugin, ensuring developers can leverage the latest features and improvements while maintaining compatibility with older versions.
New Attribute for JVM and Android-Published Libraries
A new attribute distinguishes JVM and Android-published libraries, improving dependency handling and ensuring correct libraries are used based on the target platform.
Improved Dependency Handling
Enhanced support for CInteropProcess in Kotlin/Native improves integration with native libraries, making it easier to use native code in Kotlin applications.
Visibility Changes in Gradle
Visibility changes improve the organization and management of build configurations, reducing clutter and simplifying navigation through complex build scripts.
New Directory for Kotlin Data in Gradle Projects
A new directory helps organize data-related files, making it easier to manage and maintain large-scale projects.
Kotlin/Native Compiler Downloaded When Needed
The Kotlin/Native compiler is now downloaded as needed, reducing initial setup time for new projects and allowing developers to start working quickly.
Deprecating Old Ways of Defining Compiler Options
Encourages using the new DSL for better configuration management, reducing errors and improving the overall build process.
Bumped Minimum AGP-Supported Version
The minimum supported version of the Android Gradle plugin (AGP) has been bumped to ensure compatibility with the latest features and improvements in AGP.
New Gradle Property to Try the Latest Language Version
A new Gradle property allows developers to easily test the latest features and improvements without affecting the main build process.
New JSON Output Format for Build Reports
Introduces a new JSON output format for build reports, providing more detailed and structured information about the build process, making it easier to analyze and debug complex issues.
kapt Configurations Inherit Annotation Processors from Super Configurations
kapt configurations now inherit annotation processors from super configurations, reducing the need for manual configuration and improving the consistency of annotation processing.
Kotlin Gradle Plugin No Longer Uses Deprecated Gradle Conventions
Ensures the plugin remains compatible with the latest Gradle features and best practices by no longer using deprecated Gradle conventions.
Standard Library Enhancements
The standard library in Kotlin 2.0 has been enhanced to provide more stability and universality across platforms.
Key Enhancements
- Stable Replacement of Enum Class Value's Generic Function: Ensures enum values can be used more consistently and safely across different platforms.
- Stable AutoCloseable Interface: Provides a robust way to manage resources and ensure they are properly closed after use.
- Common Protected Property AbstractMutableList.modCount: Helps track the number of modifications made to a list, improving the reliability and performance of list-based operations.
- Common Protected Function AbstractMutableList.removeRange: Allows developers to remove a range of elements from a list in a safe and efficient manner.
- Common String.toCharArray(destination): Converts a string into a character array, providing a more flexible way to manipulate strings.
Practical Applications of Kotlin 2.0
Migrating to Kotlin 2.0
Migrating to Kotlin 2.0 involves several steps, including updating the Gradle configuration, updating dependencies, and configuring the Compose compiler.
-
Update Gradle Configuration: Update the
gradle/libs.versions.toml
file to reference Kotlin 2.0.
toml
[versions]
agp = "8.4.1"
kotlin = "2.0.0"
ksp = "2.0.0-1.0.21" -
Update Dependencies: Update the dependencies in the
build.gradle.kts
file to reference Kotlin 2.0.
kotlin
plugins {
alias(libs.plugins.compose.compiler)
} -
Configure Compose Compiler: Remove the
composeOptions
configuration and add the reference to thekotlin-compose-compiler
plugin.
kotlin
plugins {
alias(libs.plugins.compose.compiler)
} -
Run the Build: Run the
gradle clean build
command or build the project from Android Studio.
Example of Using New Features
Here’s an example of using some of the new features in Kotlin 2.0:
kotlin
// Using the new K2 compiler mode
@file:OptIn(K2CompilerMode::class)
fun main() {
// Using the underscore operator for type args
val list = listOf(1, 2, 3)
val newList = list.map { it _ 2 } // Automatically infers the type of argument
println(newList) // Output: [1, 4, 9]
}
// Using the new enum class values function
enum class Color {
RED, GREEN, BLUE
}
fun main() {
val colors = Color.values()
for (color in colors) {
println(color.name)
}
}
// Using the new operator for open-ended ranges
fun main() {
val range = 1..10 // Open-ended range from 1 to 10
for (i in range) {
println(i)
}
}
Final Thoughts
Kotlin 2.0 represents a significant leap forward in the evolution of the language. Its performance improvements, multiplatform support, and tooling enhancements make it an indispensable tool for modern software development. The K2 compiler, with its stable and Beta modes, provides developers with the flexibility to choose between speed and stability based on their specific needs. The enhanced multiplatform integration and standard library features ensure that developers can create cross-platform applications with ease. The practical applications of Kotlin 2.0, including migrating to the new version and using its new features, demonstrate its potential to transform the way developers create applications.
Kotlin 2.0 is a powerful tool that offers numerous benefits for developers. Its robust features and improvements make it an excellent choice for building complex enterprise solutions, innovative mobile apps, and everything in between. Whether transitioning from Java or starting a new project, embracing Kotlin 2.0 will empower developers to deliver high-quality, efficient, and scalable applications.
By exploring the latest features and enhancements of Kotlin 2.0, developers can unlock new possibilities in software development. The language's continued evolution ensures that it remains a leading choice for modern programming needs, providing a fusion of safety, expressiveness, and conciseness that is unmatched by many other languages. As the software development landscape continues to evolve, Kotlin 2.0 stands ready to meet the challenges head-on, offering developers an infinitely rewarding journey propelled by ingenuity, productivity, and limitless possibilities.