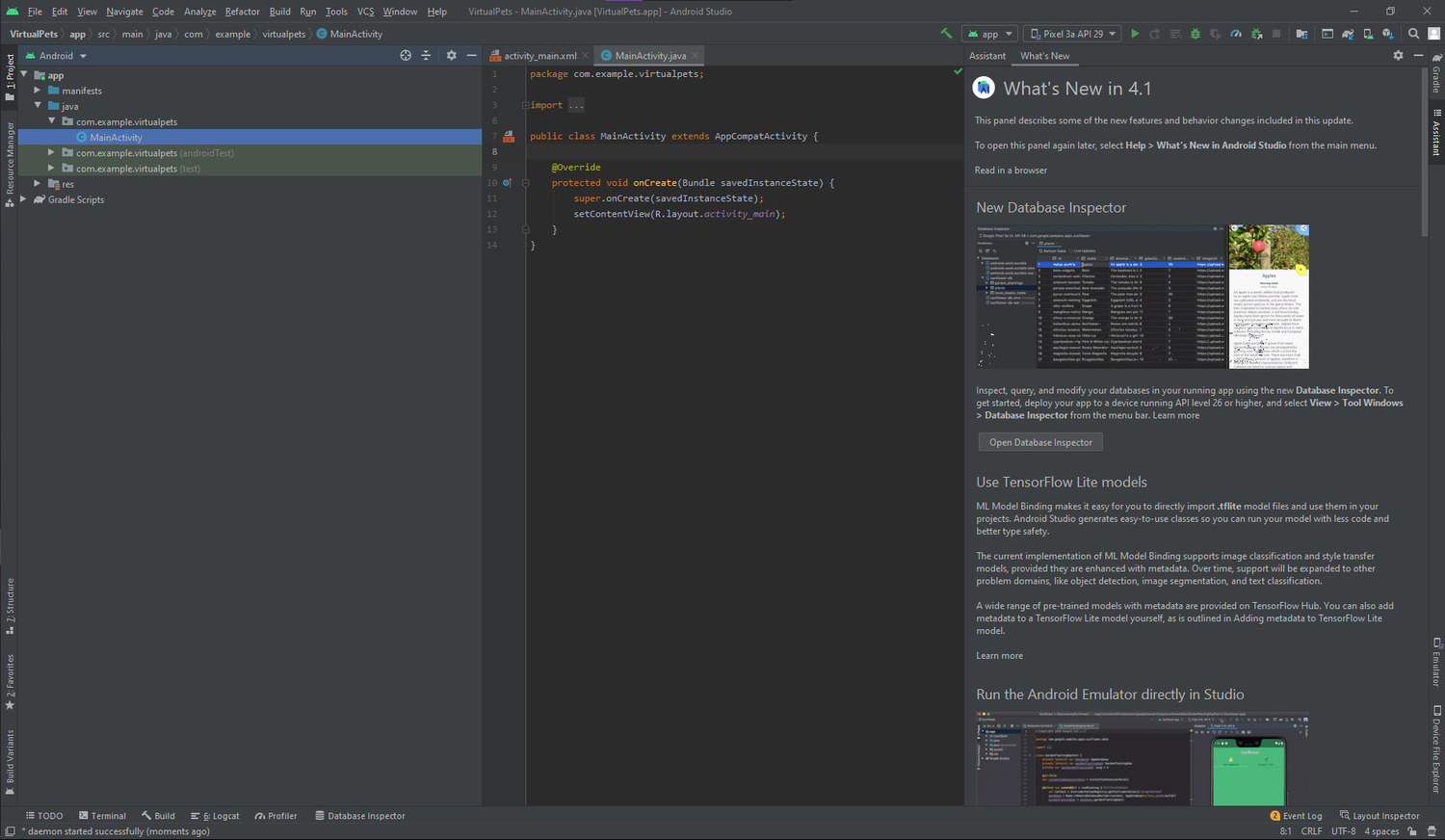
Introduction
Android Studio is an integrated development environment (IDE) specifically designed for Android app development. Based on the IntelliJ platform, it provides a comprehensive set of tools for creating, debugging, and testing Android applications. This tutorial will guide you through setting up Android Studio, understanding its interface, and creating your first Android application.
Prerequisites
Before diving into the tutorial, ensure you have the following prerequisites:
- Java Installed: Android Studio uses Java as its primary programming language. Ensure Java is installed on your computer. Download the Java Runtime Environment (JRE) from Oracle's official website.
- Android SDK: The Android Software Development Kit (SDK) is necessary for developing Android applications. Download the SDK from the Android Developer website.
- Android Studio Installed: Download and install Android Studio from the official website. Follow the installation instructions provided by the installer.
Setting Up Android Studio
Downloading the SDK
To start developing Android applications, download the latest version of the Android SDK. The SDK is publicly available on GitHub and can be downloaded as a ZIP file.
-
Accessing the SDK Repository:
- Open your web browser and navigate to the GitHub repository where the latest SDK is hosted.
- Click on the green "Code" button and select "Download ZIP."
-
Extracting the SDK:
- Save the downloaded ZIP file to a desired location on your computer.
- Extract the contents of the ZIP file to that location. You should see a folder containing all the necessary files for the SDK.
-
Using GitHub Desktop:
- If you prefer using GitHub Desktop, open it and navigate to the repository.
- Click on the green "Code" button and select "Open with GitHub Desktop."
- Follow the prompts to clone the project.
-
Using the Command Line:
- Open your terminal (usually bash) in the desired resource location.
- Use the command
git clone https://github.com/FIRST-Tech-Challenge/FtcRobotController.git
to clone the project.
Opening the SDK in Android Studio
-
Launching Android Studio:
- Open Android Studio. If another project is open, close it.
- Check for updates by clicking on the "Configure" dropdown and selecting "Check for Updates." If the latest version is not installed, download the updates.
-
Importing the Project:
- Select "Import Project" from the start-up screen of Android Studio.
- Navigate to where the SDK is saved on your computer. Choose the directory that has the Android logo.
-
Project View:
- Change to project view by selecting it from the top left corner of the window.
- Wait for the project to complete its build. This indicator should be located at the bottom of the window by default.
Understanding the Interface
Android Studio provides a comprehensive interface that includes several key components:
-
Project Window:
- This window displays all the files and directories in your project. It is essential for navigating through your code and resources.
-
Tool Window:
- The tool window provides various tools such as the terminal, logcat, and other debugging tools.
-
Editor Window:
- This is where you write your code. It supports syntax highlighting and code completion.
-
Run/Debug Configurations:
- These configurations allow you to set up how your application will be run or debugged.
Creating Classes
To create a new Java class in Android Studio:
-
Navigate to the TeamCode Folder:
- In the project view, navigate to the
org.firstinspires.ftc.teamcode
package.
- In the project view, navigate to the
-
Create a New Java Class:
- Right-click on the package and select "New" > "Java Class."
- Alternatively, select "Package" if you want to create a subfolder for organization purposes and then create classes in those packages.
Terminal and Logcat
-
Terminal:
- The terminal is located near the bottom left of the application window. It allows you to run commands directly from within Android Studio.
- Build your program through the command line via the local terminal by clicking on the terminal tab and inputting
gradlew :TeamCode:clean :TeamCode:build
. This will delete the previously compiled files and build your TeamCode module.
-
Logcat:
- Logcat is also located near the bottom left of the application window. It displays logs from your application, which can be useful for debugging purposes.
- Some useful information on using logcat can be found in the official documentation.
Installing Your Program
To install your program onto the Robot Controller:
-
Connect Your Robot Controller:
- Use a USB-C cable to connect your robot controller to your computer. Your computer should detect it and select it as the target device for builds.
-
Wireless Installation:
- If you prefer wireless installation, ensure ADB is installed. Connect to your bot's WiFi connection (it defaults to FTC-XXXXX with random X's but should be set to RC-
if used at a competition). - Run
adb connect 192.168.43.1:5555
in a terminal/command prompt. This should make the device appear in the dropdown list of Android Studio. - Use
adb disconnect
before turning off the robot or restarting it to avoid bugs.
- If you prefer wireless installation, ensure ADB is installed. Connect to your bot's WiFi connection (it defaults to FTC-XXXXX with random X's but should be set to RC-
-
Installing via USB:
- Once connected via USB, click the play button located near the top right of the application window.
- Select your device from the dropdown list, and your program will install onto the device.
Debugging Your Application
Debugging is an essential part of the development process. Here are some steps to debug your application:
-
Setting Breakpoints:
- Open your code file and click in the left margin where you want to set a breakpoint. This will pause execution at that point when you run your application.
-
Running Your Application:
- Click on the green play button to run your application. You can also use the debug button (a bug icon) to run your application in debug mode.
-
Viewing Logs:
- Use logcat to view logs from your application. This can help identify issues and errors.
-
Using the Terminal:
- The terminal can be used to run commands directly from within Android Studio. This is useful for tasks like cleaning and rebuilding your project.
Advanced Debugging Techniques
-
Remote Debugging:
- If you're having trouble with the robot controller, remotely start the Robot Controller app using
adb shell am start -n com.qualcomm.ftcrobotcontroller/org.firstinspires.ftc.robotcontroller.internal.PermissionValidatorWrapper
.
- If you're having trouble with the robot controller, remotely start the Robot Controller app using
-
Version Control:
- Version control systems like Git can be used to manage changes to your source code. This is particularly useful for collaborative projects.
Additional Resources
- Official Documentation: The official documentation provided by Google is an excellent resource for learning more about Android Studio and its features.
- Tutorials and Guides: Numerous tutorials and guides available online can help you learn specific aspects of Android Studio.
- Community Support: Joining communities like the FTC Discord server can provide valuable support and resources from experienced developers.
By following these steps and utilizing the resources available, you'll be well on your way to becoming proficient in using Android Studio for developing Android applications.