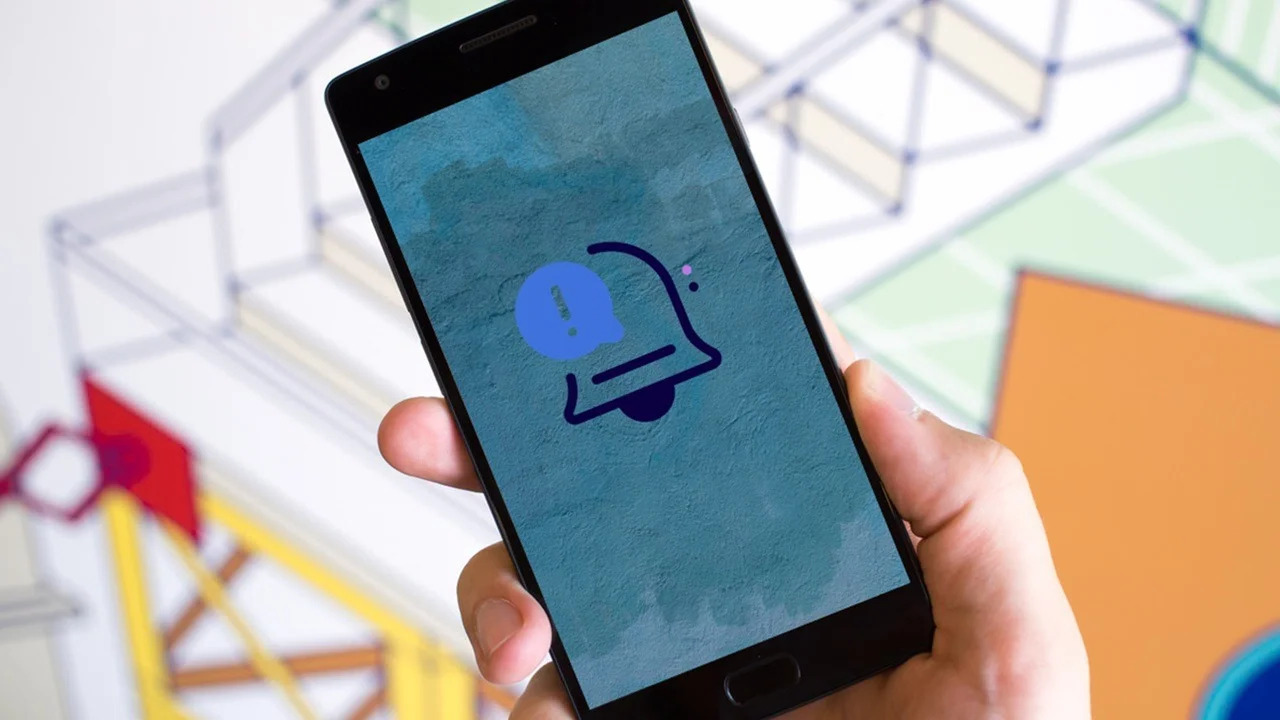
Introduction
In today's digital age, mobile applications play a significant role in our daily lives. With billions of smartphone users worldwide, competition for user attention is fierce. One effective way to engage users and maintain their interest in your app is through push notifications. These notifications allow you to send timely, relevant, and personalized messages to your users, even when the app is not actively in use. This comprehensive guide covers everything from the basics to advanced strategies for implementing and managing Android push notifications.
What Are Android Push Notifications?
Android push notifications are messages sent by applications to users' devices through services like Google's Firebase Cloud Messaging (FCM). These notifications can display text, alerts, sounds, and icon badges, making them a powerful tool for maintaining user engagement and providing real-time updates. Unlike traditional in-app notifications, push notifications can reach users even when the app is not running, making them an essential feature for many modern mobile applications.
Why Are Android Push Notifications Important?
Push notifications are crucial for several reasons:
- User Retention and Engagement: Timely and relevant messages remind users to return to your app and interact with new content or features, maintaining high levels of user engagement and retention.
- Real-Time Updates: Developers can provide real-time updates, which is vital for apps that rely on timely information delivery, such as news or weather apps.
- Personalization and User Experience: Targeted messages based on user preferences and behaviors offer a personalized user experience, making it more tailored to individual needs.
- Cost-Effective Marketing: Push notifications offer a cost-effective way to promote new features, products, or services directly within the app, reducing reliance on external marketing channels and increasing conversion rates.
Prerequisites for Implementing Android Push Notifications
Before diving into the implementation details, it's essential to understand the prerequisites:
- FCM Setup: Set up Firebase Cloud Messaging (FCM) in your project. This involves obtaining the server key for FCM and registering it with your server.
- Device Token Generation: When users opt-in for push notifications, a device token is generated. This token identifies users in the application and sends them notifications.
- Server Integration: Integrate your server with FCM to send and manage push notifications.
- User Opt-In: Users must opt-in to receive push notifications from your application, typically done through a settings menu or during the initial app setup.
Step-by-Step Guide to Implementing Android Push Notifications
Step 1: Set Up Firebase Cloud Messaging (FCM)
To start implementing push notifications, first set up FCM in your project:
-
Add FCM to Your Project:
- Open your Android project in Android Studio.
- Add the Firebase configuration file (
google-services.json
) to your project. - Add the Firebase SDK to your app-level
build.gradle
file:
groovy
dependencies {
implementation 'com.google.firebase:firebase-messaging:23.0.0'
}
-
Get the Server Key for FCM:
- Go to the Firebase console and navigate to your project.
- Click on "Cloud Messaging" under the "Navigation menu".
- Click on "Web API key" and copy the server key.
-
Register FCM Server Key to Sendbird Dashboard (Optional):
- If using a service like Sendbird for chat and notifications, register the FCM server key in their dashboard.
Step 2: Register FCM Server Key to Your Server
Once you have the server key, register it with your server. This involves integrating the FCM server key into your backend code so that it can send notifications to users.
Step 3: Set Up an FCM Client App in Your Android Project
After setting up FCM on your server, set up the client app in your Android project:
-
Get the Device Token:
- In your Android app, get the device token when the user opts-in for push notifications by overriding the
onNewToken
method in theFirebaseMessagingService
class:
java
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onNewToken(@NonNull String token) {
FirebaseInstanceId.getInstance().getInstanceId().addOnCompleteListener(new OnCompleteListener() {
@Override
public void onComplete(@NonNull Tasktask) {
if (!task.isSuccessful()) {
Log.w(TAG, "getInstanceId failed", task.getException());
return;
}String newToken = task.getResult().getToken();
sendTokenToServer(newToken);
}
});
}private void sendTokenToServer(String token) {
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://your-server.com")
.addConverterFactory(GsonConverterFactory.create())
.build();APIService apiService = retrofit.create(APIService.class);
Call
call = apiService.sendToken(token); call.enqueue(new Callback
() {
@Override
public void onResponse(Callcall, Response response) {
if (response.isSuccessful()) {
Log.d(TAG, "Token sent successfully");
} else {
Log.d(TAG, "Failed to send token");
}
}@Override
public void onFailure(Callcall, Throwable t) {
Log.e(TAG, "Failed to send token", t);
}
});
}
}
- In your Android app, get the device token when the user opts-in for push notifications by overriding the
-
Handle Incoming Messages:
- Override the
onMessageReceived
method in theFirebaseMessagingService
class to handle incoming messages:
java
public class MyFirebaseMessagingService extends FirebaseMessagingService {
@Override
public void onMessageReceived(@NonNull RemoteMessage remoteMessage) {
if (remoteMessage.getData().size() > 0) {
String message = remoteMessage.getData().get("message");
Log.d(TAG, "Received data payload: " + message);
displayNotification(message);
}displayNotification(remoteMessage.getNotification().getTitle(), remoteMessage.getNotification().getBody());
}private void displayNotification(String title, String body) {
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.ic_notification)
.setContentTitle(title)
.setContentText(body)
.setPriority(NotificationCompat.PRIORITY_DEFAULT);Intent intent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_IMMUTABLE);builder.setContentIntent(pendingIntent);
NotificationManagerCompat notificationManagerCompat = NotificationManagerCompat.from(this);
notificationManagerCompat.notify(1, builder.build());
}
}
- Override the
Step 4: Register a Push Token to the Sendbird Server
If using a service like Sendbird for chat and notifications, register the push token with their server.
Step 5: Add the Push Event Handlers
Add event handlers to handle incoming push notifications and update your app accordingly.
Step 6: Handle an FCM Message Payload
Handle the payload of incoming messages to display notifications or update the UI.
Designing Effective Push Notifications
Designing effective push notifications is crucial for maintaining user engagement. Here are some tips:
- Keep It Short and Sweet: Push notifications should be concise and to the point. Avoid lengthy messages that might confuse or overwhelm users.
- Use Clear and Concise Language: Use simple, clear language that is easy to understand. Avoid jargon or technical terms unless they are specific to your app.
- Include Visual Elements: Use icons, images, or other visual elements to make your notifications more engaging. Ensure that these elements do not overwhelm the text.
- Personalize Notifications: Personalize notifications based on user preferences and behaviors. This makes the experience more tailored to individual needs.
- Timing is Everything: Timing is crucial when sending push notifications. Send notifications at times when users are most likely to be engaged, such as during peak usage hours or when they are likely to be idle.
- Avoid Spamming Users: Avoid spamming users with too many notifications in a short period of time. This can lead to user fatigue and decreased engagement.
- Provide Clear Call-to-Actions (CTAs): Provide clear CTAs in your notifications that tell users what action to take next. This could be opening the app, visiting a webpage, or making a purchase.
- Test Your Notifications: Test your notifications thoroughly before sending them out to a large audience. Ensure that they are working as intended and that there are no issues with delivery or display.
Advanced Features of Android Push Notifications
Android push notifications offer several advanced features that can enhance the user experience:
- Action Buttons: Starting in Android 7.0 (API level 24), you can add action buttons to your notifications that allow users to take specific actions directly from the notification.
- Replying to Messages: Starting in Android 7.0 (API level 24), users can reply to messages directly from the notification using inline reply.
- Notification Groups: Starting in Android 7.0 (API level 24), you can group notifications together using the
setGroup
method, which is supported on handheld devices like phones and tablets. - Security Features: On Android 12 (API level 31) and higher, you can configure a notification action such that the device must be unlocked for your app to invoke that action, adding an extra layer of security to notifications on locked devices.
- Notification Compatibility: To use the latest notification API features while supporting older devices, use the Support Library notification API (
NotificationCompat
) and its subclasses.
Troubleshooting Push Notifications
Troubleshooting push notifications can be complex, but here are some tips to help you debug and resolve issues:
- Check Device Tokens: Ensure that device tokens are being generated correctly and sent to your server.
- Verify Server Configuration: Verify that your server is correctly configured to send push notifications using FCM.
- Test Notifications: Test your notifications thoroughly before sending them out to a large audience.
- Use FCM Error Codes: If you encounter issues, refer to FCM error codes for troubleshooting.
- Use Debugging Tools: Use debugging tools provided by services like Sendbird to troubleshoot push notifications.
Implementing and managing Android push notifications is a critical aspect of maintaining user engagement and providing real-time updates. By following the steps outlined in this guide, you can create effective push notifications that enhance the user experience and drive engagement for your app. Keep your notifications concise, personalized, and timely, and always test them thoroughly before deployment. With these strategies in place, you can build a loyal user base and drive success for your mobile application.
Understanding Push Notifications
Push notifications alert users to new messages, updates, or reminders directly on their devices. They appear as banners, pop-ups, or badges on app icons. This feature ensures users stay informed without needing to open the app. Notifications can be customized for different types of alerts, such as promotions, news, or personal messages. They can also be scheduled to appear at specific times or triggered by certain actions.
What You Need to Get Started
To ensure your device supports push notifications, check these requirements:
- Operating System: Your device must run Android 4.1 (Jelly Bean) or later. Older versions won't support this feature.
- Google Play Services: Ensure Google Play Services is installed and updated. This is crucial for receiving notifications.
- Internet Connection: A stable Wi-Fi or mobile data connection is necessary. Without internet, notifications won't arrive.
- Battery Optimization: Disable battery optimization for the app sending notifications. This prevents the system from blocking them.
- App Permissions: Grant the app permission to send notifications. Go to Settings > Apps & notifications > [App Name] > Notifications and enable them.
- Background Data: Allow the app to use data in the background. Navigate to Settings > Apps & notifications > [App Name] > Data usage and toggle on background data.
- Do Not Disturb Mode: Ensure Do Not Disturb mode is off or configured to allow notifications from the app. Check Settings > Sound > Do Not Disturb.
- Device Storage: Maintain enough free storage. Low storage can hinder app performance and notification delivery.
Meeting these requirements ensures your device can receive push notifications without issues.
How to Set Up Notifications
- Open Settings on your Android device.
- Scroll down and tap on "Apps & notifications."
- Select "See all apps" to view all installed applications.
- Choose the app for which you want to enable push notifications.
- Tap on "Notifications."
- Toggle the switch to "On" to enable notifications.
- Customize notification settings by selecting "Advanced" options if needed.
- Repeat these steps for other apps as desired.
Done!
Tips for Effective Use
Personalize notifications with the user's name or preferences. Timing matters; send alerts when users are most active. Use rich media like images or videos to grab attention. Keep messages short and to the point. Segment your audience to send relevant content. A/B test different messages to see what works best. Include a call-to-action to guide users on what to do next. Avoid sending too many notifications to prevent annoyance. Use geolocation to send location-specific alerts. Monitor analytics to track performance and make improvements.
Troubleshooting Common Problems
Notifications not appearing? Check if "Do Not Disturb" mode is on. If it is, turn it off. Next, ensure app notifications are enabled. Go to Settings > Apps > [App Name] > Notifications. Toggle the switch to "On."
Battery saver mode can also block notifications. Disable it by going to Settings > Battery > Battery Saver. Turn it off.
Still no notifications? Clear the app cache. Go to Settings > Apps > [App Name] > Storage > Clear Cache.
If problems persist, update the app. Open Google Play Store, search for the app, and tap "Update" if available.
Restarting your device can also help. Hold the power button, then select "Restart."
Lastly, check for system updates. Go to Settings > System > System Update. Install any available updates.
Keeping Your Data Safe
Using push notifications on Android involves handling user data with care. Notifications often require permissions to access certain data, like location or contacts. Always review app permissions before granting access.
To maintain privacy, disable notifications for apps that don't need them. Regularly update your apps to ensure you have the latest security patches. Use strong passwords and enable two-factor authentication where possible.
Be cautious about public Wi-Fi; use a VPN to protect your data. Finally, clear app cache and data periodically to remove any stored information that might compromise your privacy.
Comparing Other Options
Pros of Android Push Notifications:
- Customizable: Users can tweak settings for individual apps.
- Rich Media: Supports images, videos, and interactive buttons.
- Wide Reach: Works on a vast range of devices.
Cons of Android Push Notifications:
- Battery Drain: Frequent notifications can reduce battery life.
- Overwhelming: Too many alerts can annoy users.
- Privacy Concerns: Potential for misuse by apps.
iOS Push Notifications:
- Pros: Seamless integration with Apple ecosystem, high user engagement, better privacy controls.
- Cons: Less customizable, limited to Apple devices.
Windows Push Notifications:
- Pros: Integrated with Windows OS, good for desktop users.
- Cons: Less popular on mobile, fewer customization options.
Alternative: Email Notifications
- Pros: Less intrusive, can be read at any time, easy to manage.
- Cons: Slower delivery, may end up in spam folders.
Alternative: SMS Notifications
- Pros: Immediate delivery, works on all phones, no internet needed.
- Cons: Limited character count, potential costs for users.
Final Thoughts on Android Push Notifications
Android push notifications are a powerful tool for engaging users. They help keep your audience informed and connected. To make the most of them, focus on timing, relevance, and personalization. Avoid spamming users with too many alerts, as this can lead to uninstalls.
Use clear, concise messages that provide value. Test different approaches to see what works best for your audience. Keep an eye on analytics to measure success and make adjustments as needed.
Remember, the goal is to enhance the user experience, not overwhelm it. With thoughtful implementation, push notifications can boost engagement and retention. So, take the time to craft meaningful messages that resonate with your users. This way, you’ll build a loyal and satisfied audience.
What are push notifications on Android?
Push notifications are messages sent by apps to your device. They pop up like text messages but come from apps you’ve installed.
How do I enable or disable push notifications?
Go to your device’s Settings. Tap on Apps & notifications, then select the app you want. Toggle the Allow notifications switch on or off.
Why am I not receiving push notifications?
Check if you’ve accidentally turned off notifications for the app. Also, make sure your device isn’t in Do Not Disturb mode and that you have a stable internet connection.
Can I customize the type of notifications I get?
Yes, you can. In the app’s notification settings, you can choose which types of notifications you want to receive, like alerts, sounds, or vibrations.
Do push notifications drain battery?
They can, especially if you receive a lot of them. To save battery, limit notifications to only the most important apps.
Are push notifications secure?
Generally, yes. However, be cautious about the information you share through notifications, especially if your device is not locked.
How can I manage notifications for multiple apps?
Use the Settings app on your device. Under Apps & notifications, you can see a list of all apps and manage their notification settings individually.