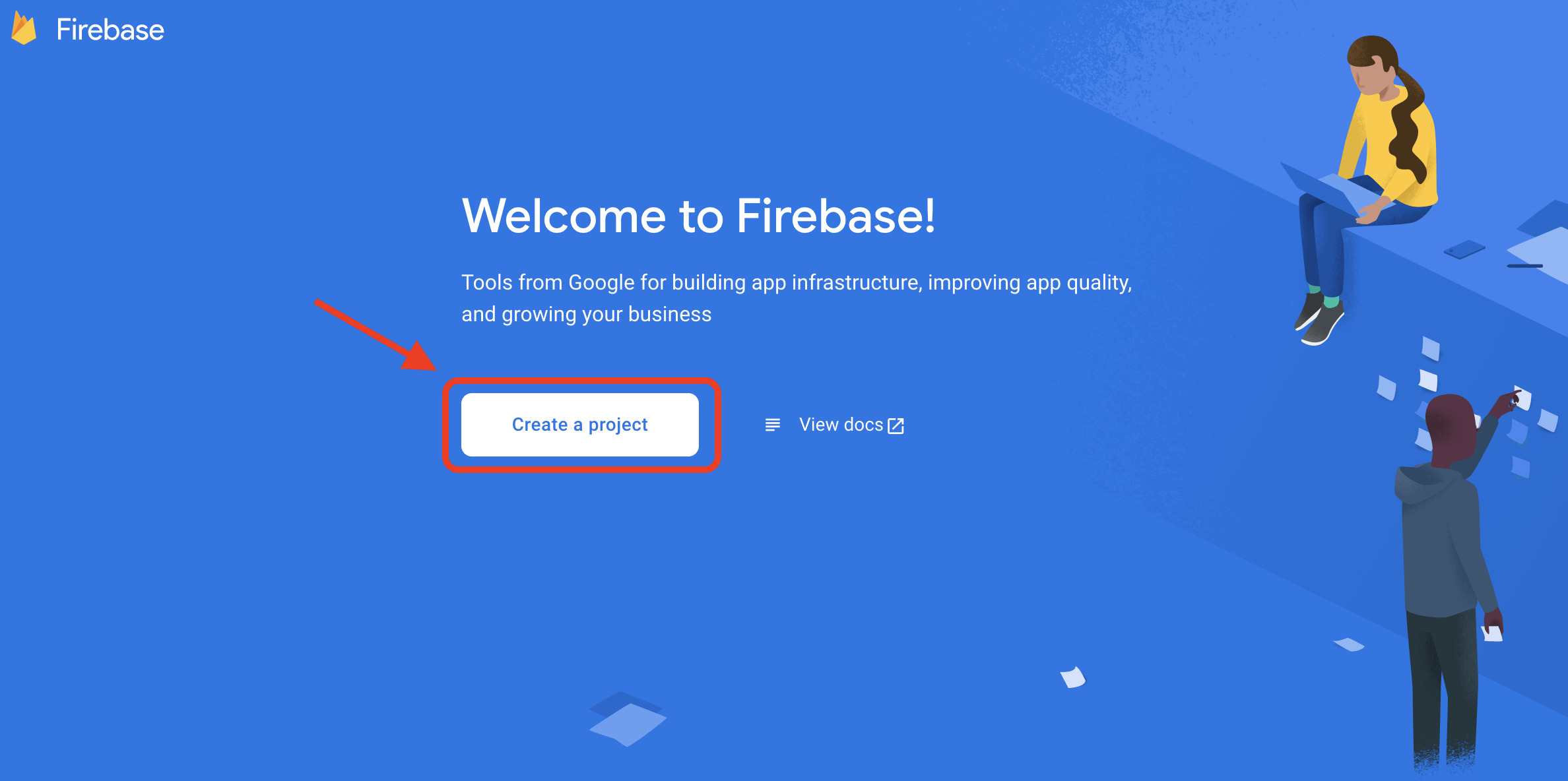
Introduction
Firebase is a powerful backend platform developed by Google that provides a suite of services to help developers build and manage their mobile applications. For Android developers, Firebase offers a wide range of tools and services that can significantly simplify the development process, enhance the user experience, and improve the overall performance of the app. This comprehensive guide will delve into the world of Firebase and explore how to integrate it into your Android app.
What is Firebase?
Firebase is a cloud-hosted backend service that allows developers to build web and mobile applications without managing the infrastructure. It provides a real-time database, authentication services, cloud storage, and various other features essential for modern mobile applications. Firebase is designed to be user-friendly and scalable, making it an ideal choice for both small and large-scale projects.
Key Features of Firebase
Realtime Database
Store and synchronize data in real-time across all connected devices. This feature is particularly useful for applications requiring live updates, such as chat apps or live scores.
Authentication
Firebase provides multiple authentication methods, including:
- Email/password
- Google Sign-In
- Facebook Sign-In
- Twitter Sign-In
- GitHub Sign-In
These methods ensure that your app can securely handle user logins and manage user data.
Cloud Storage
Store files such as images, videos, and documents securely in the cloud. It provides easy-to-use APIs for uploading and downloading files.
Cloud Functions
Serverless functions that run on Google Cloud infrastructure. Use them to perform backend tasks such as sending notifications or processing data.
Cloud Messaging
Send notifications to users even when the app is not running. This service supports both Android and iOS platforms.
Analytics
Firebase provides analytics tools that help you understand how users interact with your app. Use this data to improve the user experience and make data-driven decisions.
Crashlytics
Identify and fix crashes in your app. It provides detailed reports on crashes, including the device type, operating system version, and other relevant information.
Dynamic Links
Special URLs that can open specific screens in your app when the user clicks on them. They are particularly useful for sharing content between different platforms.
Setting Up Firebase for Android
Step 1: Create a Firebase Project
- Go to the Firebase Console: Open the Firebase console by navigating to firebase.google.com.
- Create a New Project: Click on the "Add project" button and follow the prompts to create a new project.
- Set Up Project Details: Enter your project name and select the location where you want to host your project.
Step 2: Register Your App with Firebase
- Go to the Project Overview Page: Once your project is created, go to the project overview page.
- Click on the Android Icon: In the center of the page, click on the Android icon (or Add app) to launch the setup workflow.
- Enter Your App's Package Name: Enter your app's package name in the Android package name field. The package name uniquely identifies your app on the device and in the Google Play Store.
- Optional Fields: Optionally enter an app nickname and the SHA-1 hash of your debug signing certificate. The app nickname is an internal identifier that helps you identify your app in the Firebase console, while the SHA-1 hash is required by Firebase Authentication when using Google Sign-In or phone number sign-in.
- Register App: Click on the Register app button to complete this step.
Step 3: Add a Firebase Configuration File
- Download google-services.json: After registering your app, Firebase will give you a file called google-services.json. This file contains configuration values necessary for connecting your Android app to your Firebase project.
- Move the File to Your App Module Root Directory: Move this file into the module (app-level) root directory of your Android project.
- Add the Google Services Gradle Plugin: To make the google-services.json config values accessible to Firebase SDKs, add the Google Services Gradle plugin to your build.gradle file.
groovy
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.google.gms:google-services:4.3.10'
}
}
allprojects {
repositories {
google()
mavenCentral()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
Step 4: Add Firebase SDKs to Your App
- Add Dependencies in build.gradle: Add the necessary dependencies for Firebase services in your app-level build.gradle file.
For example, if you want to use Firebase Authentication and Realtime Database:
groovy
dependencies {
implementation 'com.google.firebase:firebase-auth:22.0.1'
implementation 'com.google.firebase:firebase-database:19.3.3'
}
- Apply the Google Services Plugin: Apply the Google Services plugin at the bottom of your app-level build.gradle file.
groovy
apply plugin: 'com.google.gms.google-services'
Using Authentication in Firebase
Firebase provides multiple authentication methods to securely handle user logins. Here’s how you can implement authentication in your Android app:
Step 1: Add Firebase Authentication
- Add Dependencies: Add the Firebase Authentication dependency in your build.gradle file.
groovy
dependencies {
implementation 'com.google.firebase:firebase-auth:22.0.1'
}
- Initialize Firebase: Initialize Firebase in your application class by calling
FirebaseApp.initializeApp(this)
.
Step 2: Create and Configure the App
- Create a FirebaseUI Configuration: Create a FirebaseUI configuration to handle sign-in flows.
java
FirebaseAuth auth = FirebaseAuth.getInstance();
FirebaseAuthSettings settings = auth.getSettings();
settings.setAppVerificationDisabledForTesting(true);
- Code the Main Activity: In your main activity, create a button to trigger the sign-in flow.
java
public class MainActivity extends AppCompatActivity {
private FirebaseAuth auth;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize Firebase
FirebaseApp.initializeApp(this);
// Initialize Firebase Authentication
auth = FirebaseAuth.getInstance();
// Button to trigger sign-in flow
Button signInButton = findViewById(R.id.sign_in_button);
signInButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Start the sign-in flow
Intent intent = AuthUI.getIntent(
getApplicationContext(), // Context
AuthUI.getInstance().createSignInIntentBuilder()
.setIsSmartLockEnabled(true)
.setProviders(
AuthUI.FACEBOOK_PROVIDER,
AuthUI.GOOGLE_PROVIDER)
.build());
startActivityForResult(intent, RC_SIGN_IN);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == RC_SIGN_IN) {
if (resultCode == RESULT_OK) {
// Successfully signed in with result: data
FirebaseUser user = auth.getCurrentUser();
Toast.makeText(this, "Signed in as: " + user.getDisplayName(), Toast.LENGTH_SHORT).show();
} else {
// Sign in failed. If result is RESULT_CANCELED, the user cancelled the sign-in flow.
// If result is RESULT_OK but data is null, the user pressed a back button after selecting a provider.
// If result is RESULT_OK and data is not null, the user successfully signed in.
// However, we are passing null here because we are not getting any data back from the intent.
Toast.makeText(this, "Sign in failed", Toast.LENGTH_SHORT).show();
}
}
}
}
Step 3: Create and Code the Signed-In Activity
Once the user is signed in, redirect them to a signed-in activity where they can manage their account settings.
java
public class SignedInActivity extends AppCompatActivity {
private FirebaseAuth auth;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_signed_in);
// Initialize Firebase
FirebaseApp.initializeApp(this);
// Initialize Firebase Authentication
auth = FirebaseAuth.getInstance();
// Get current user
FirebaseUser user = auth.getCurrentUser();
// Display user details
TextView displayNameTextView = findViewById(R.id.display_name_text_view);
displayNameTextView.setText(user.getDisplayName());
Button signOutButton = findViewById(R.id.sign_out_button);
signOutButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Sign out the user
auth.signOut();
Intent intent = new Intent(SignedInActivity.this, MainActivity.class);
startActivity(intent);
finish();
}
});
Button resetPasswordButton = findViewById(R.id.reset_password_button);
resetPasswordButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Reset password dialog
Intent intent = AuthUI.getIntent(
getApplicationContext(), // Context
AuthUI.getInstance().createResetPasswordIntent(user.getUid()));
startActivityForResult(intent, RC_RESET_PASSWORD);
}
});
}
@Override
protected void onActivityResult(int requestCode, int resultCode, Intent data) {
super.onActivityResult(requestCode, resultCode, data);
if (requestCode == RC_RESET_PASSWORD) {
if (resultCode == RESULT_OK) {
// Password reset successful
Toast.makeText(this, "Password reset successful", Toast.LENGTH_SHORT).show();
} else {
// Password reset failed
Toast.makeText(this, "Password reset failed", Toast.LENGTH_SHORT).show();
}
}
}
}
Using the Realtime Database
The Realtime Database is a NoSQL database that allows you to store and synchronize data in real-time across all connected devices. Here’s how you can use it in your Android app:
Step 1: Add Firebase Realtime Database Dependency
- Add Dependency: Add the Firebase Realtime Database dependency in your build.gradle file.
groovy
dependencies {
implementation 'com.google.firebase:firebase-database:19.3.3'
}
Step 2: Initialize Firebase Realtime Database
- Initialize Database: Initialize the Realtime Database in your application class by calling
FirebaseDatabase.getInstance()
.
java
FirebaseDatabase database = FirebaseDatabase.getInstance();
Step 3: Write Data to the Database
- Create a Reference: Create a reference to the specific location where you want to write data.
java
DatabaseReference reference = database.getReference("users");
- Write Data: Use the
setValue()
method to write data to the database.
java
User user = new User("John Doe", 30);
reference.setValue(user);
Step 4: Read Data from the Database
- Create a Reference: Create a reference to the specific location where you want to read data.
java
DatabaseReference reference = database.getReference("users");
- Read Data: Use the
addValueEventListener()
method to read data from the database and listen for changes.
java
reference.addValueEventListener(new ValueEventListener() {
@Override
public void onDataChange(@NonNull DataSnapshot snapshot) {
User user = snapshot.getValue(User.class);
if (user != null) {
Log.d("User", user.getName() + " " + user.getAge());
}
}
@Override
public void onCancelled(@NonNull DatabaseError error) {
Log.w("User", "Failed to read value.", error.toException());
}
});
Advanced Concepts
Managing App Installations
Firebase provides tools to manage app installations, such as importing and exporting data. Here’s how you can manage app installations:
-
Import Segments: Use the Firebase console to import segments of users based on specific criteria.
-
Export Data: Use the Firebase console to export data to BigQuery for further analysis.
Security
Security is a critical aspect of any application, especially when dealing with user data. Here are some best practices for securing your app using Firebase:
-
Data Access Rules: Use data access rules in the Realtime Database to restrict access to specific data based on user authentication.
-
Encryption: Use encryption to protect data both in transit and at rest.
-
Authentication: Use strong authentication methods to ensure that only authorized users can access your app.
Firebase is a powerful backend platform that provides a wide range of tools and services to help developers build and manage their mobile applications. By following the steps outlined in this guide, you can integrate Firebase into your Android app and leverage its features to enhance the user experience, improve performance, and simplify development. Whether you are building a simple chat app or a complex enterprise solution, Firebase has something to offer. With its real-time database, authentication services, cloud storage, and analytics tools, you can create robust and scalable applications that meet the needs of modern users.
Feature Overview
Firebase for Android is a powerful tool that helps developers build, improve, and grow apps. Real-time database allows data syncing across all clients instantly. Authentication simplifies user login with email, Google, Facebook, and more. Cloud Firestore offers scalable, flexible databases for mobile, web, and server development. Crashlytics provides real-time crash reports, helping fix bugs faster. Analytics gives insights into user behavior and app performance. Cloud Messaging enables sending notifications and messages to users. Remote Config allows changing app behavior and appearance without publishing an update. Performance Monitoring helps track app performance issues. App Indexing improves app visibility in Google Search. Dynamic Links create deep links that survive the app install process. AdMob integrates ads to monetize apps. Test Lab offers cloud-based infrastructure for testing apps on various devices.
Compatibility and Requirements
To ensure your device supports the feature, check these requirements:
Operating System: Your device must run Android 4.1 (Jelly Bean) or later. Older versions won't support the latest features.
Google Play Services: Ensure Google Play Services is updated to the latest version. This is crucial for Firebase functionalities.
Internet Connection: A stable Wi-Fi or mobile data connection is necessary for real-time updates and data sync.
Storage Space: Ensure at least 100 MB of free storage. Firebase services may require space for caching and data storage.
RAM: Devices should have at least 1 GB of RAM. More RAM ensures smoother performance, especially for data-heavy applications.
Processor: A dual-core processor or better is recommended. This ensures the device can handle background tasks efficiently.
Permissions: Grant necessary permissions like Internet, Storage, and Location if required by the app. Without these, some features may not work.
Google Account: A Google account is often needed for authentication and other Firebase services.
Screen Resolution: Ensure a minimum screen resolution of 480x800 pixels. Lower resolutions might not display the app correctly.
Battery Health: Good battery health ensures the device can handle prolonged usage without frequent charging.
How to Set Up
Install Android Studio: Download and install Android Studio from the official website.
Create a New Project: Open Android Studio, click on "Start a new Android Studio project," and follow the prompts to set up your project.
Add Firebase to Your Project:
- Go to the Firebase Console.
- Click on "Add project" and follow the steps to create a new Firebase project.
- Once created, click on "Add app" and select "Android."
Register Your App:
- Enter your app's package name.
- Click "Register app."
Download the
google-services.json
File:- After registering, download the
google-services.json
file. - Place this file in the
app
directory of your Android project.
- After registering, download the
Add Firebase SDK:
- Open your project's
build.gradle
file (Project level). - Add the following line to the
dependencies
section: gradle classpath 'com.google.gms:google-services:4.3.10'
- Open your project's
Modify App-Level
build.gradle
:Open the
build.gradle
file (App level).Add the following lines: gradle apply plugin: 'com.google.gms.google-services'
Add Firebase dependencies: gradle implementation 'com.google.firebase:firebase-analytics:19.0.0'
Sync Your Project: Click "Sync Now" in the bar that appears to sync your project with the new dependencies.
Initialize Firebase in Your App:
- Open your
MainActivity.java
orMainActivity.kt
. - Add the following code in the
onCreate
method: java FirebaseApp.initializeApp(this);
- Open your
Run Your App: Connect your device or start an emulator, then run your app to ensure everything is set up correctly.
Boom! Firebase is now integrated into your Android project.
Effective Usage Tips
1. Real-time Database: Use Firebase Realtime Database for apps needing live updates, like chat apps or collaborative tools. Structure your data in a way that minimizes read and write operations to save bandwidth and improve performance.
2. Authentication: Implement Firebase Authentication to manage user sign-ins. Use OAuth providers like Google or Facebook for a smoother user experience. Always validate user input to prevent security issues.
3. Cloud Firestore: Opt for Cloud Firestore when you need more complex queries and offline support. Use collections and documents to organize data efficiently. Set up indexes for faster query performance.
4. Cloud Functions: Use Firebase Cloud Functions to run backend code in response to events triggered by Firebase features. For example, send a welcome email when a user signs up. Keep functions small and focused to reduce latency.
5. Analytics: Integrate Firebase Analytics to track user behavior. Set up custom events to gather specific data relevant to your app. Use this data to make informed decisions about feature improvements.
6. Crashlytics: Implement Firebase Crashlytics to monitor app stability. Prioritize fixing crashes that affect the most users. Use breadcrumbs to log key events leading up to a crash for easier debugging.
7. Cloud Messaging: Use Firebase Cloud Messaging (FCM) for push notifications. Segment your audience to send targeted messages. Schedule notifications to be sent at optimal times for user engagement.
8. Performance Monitoring: Enable Firebase Performance Monitoring to track app performance. Focus on network requests and app startup time. Use this data to identify and fix performance bottlenecks.
9. Remote Config: Utilize Firebase Remote Config to update your app without requiring a new release. Use it to run A/B tests and determine the best configurations for your users.
10. Storage: Use Firebase Storage for storing user-generated content like photos or videos. Implement security rules to ensure only authorized users can upload or download files. Compress files before uploading to save storage space and reduce download times.
Troubleshooting Common Problems
App Crashes on Launch:
- Problem: Missing or incorrect Firebase configuration.
- Solution: Check the
google-services.json
file in your app'sapp
directory. Ensure it matches the Firebase project settings.
Authentication Issues:
- Problem: Users can't sign in.
- Solution: Verify the authentication method is enabled in the Firebase console. Check API keys and ensure they are correct.
Database Read/Write Errors:
- Problem: Permission denied.
- Solution: Review Firebase database rules. Ensure the rules allow read/write access for authenticated users.
Push Notifications Not Working:
- Problem: Users not receiving notifications.
- Solution: Confirm the Firebase Cloud Messaging (FCM) setup. Check the server key and ensure the device token is correctly registered.
Analytics Data Missing:
- Problem: No data appearing in Firebase Analytics.
- Solution: Confirm the Firebase SDK is correctly integrated. Check for any filters in the Firebase console that might hide data.
Storage Upload Failures:
- Problem: Files not uploading to Firebase Storage.
- Solution: Verify storage rules. Ensure the app has the necessary permissions to access storage.
Slow Performance:
- Problem: App running slowly.
- Solution: Optimize database queries. Use indexing in Firebase Firestore to speed up data retrieval.
Incorrect Data Display:
- Problem: Data not showing as expected.
- Solution: Check the data structure in Firebase. Ensure the app's code correctly parses and displays the data.
Failed Builds:
- Problem: Build errors related to Firebase.
- Solution: Update Firebase dependencies in the
build.gradle
file. Ensure all versions are compatible.
Security Concerns:
- Problem: Potential security vulnerabilities.
- Solution: Regularly review and update Firebase security rules. Use Firebase Authentication to secure user data.
Privacy and Security Tips
When using Android Firebase, security and privacy are top priorities. Firebase employs encryption to protect data both in transit and at rest. User data is stored in Google's secure servers, which comply with industry standards like ISO 27001 and SOC 1, 2, and 3.
To maintain privacy, follow these tips:
- Use Firebase Authentication: This ensures only authorized users access your app.
- Enable Firestore Security Rules: Customize rules to control who can read or write data.
- Regularly Update: Keep Firebase SDKs and your app up-to-date to patch vulnerabilities.
- Limit Data Collection: Only collect necessary user data to minimize risk.
- Anonymize Data: Remove personally identifiable information (PII) whenever possible.
- Monitor Access: Use Firebase's built-in tools to track who accesses your data.
- Educate Users: Inform users about your data practices and obtain consent where required.
By following these steps, you can ensure a secure and private experience for your users.
Comparing Alternatives
Pros of Android Firebase:
- Real-time Database: Syncs data instantly across all clients. Similar to Apple's CloudKit but more flexible.
- Authentication: Easy user sign-in with email, Google, Facebook. Comparable to AWS Cognito.
- Cloud Messaging: Sends notifications to users. Matches Apple's Push Notification Service.
- Analytics: Tracks user behavior. Google Analytics for Firebase is robust like Mixpanel.
- Crashlytics: Monitors app crashes. Similar to Bugsnag.
Cons of Android Firebase:
- Complex Setup: Initial setup can be tricky. AWS Amplify might be simpler.
- Pricing: Costs can rise with heavy usage. Consider using MongoDB Realm for cost control.
- Limited Querying: Advanced querying is limited. Firestore or SQL databases offer more flexibility.
- Vendor Lock-in: Tied to Google's ecosystem. AWS or Azure provide more vendor options.
- Data Privacy: Concerns with data stored on Google servers. Self-hosted solutions like Parse Server offer more control.
Understanding Android Firebase
Android Firebase offers a powerful toolkit for developers. It simplifies backend tasks, allowing more focus on creating great apps. With features like real-time database, authentication, and cloud messaging, Firebase covers many needs. It’s easy to integrate and scales well with growing user bases.
Using Firebase, developers can quickly implement user authentication, manage data storage, and send notifications. This saves time and reduces complexity. The platform also provides robust analytics tools, helping track user behavior and app performance.
Firebase’s versatility makes it suitable for various app types, from simple to complex. Its real-time capabilities enhance user experience, keeping data synchronized across devices. This ensures users always have the latest information.
In short, Android Firebase is a valuable resource for app development. It streamlines many processes, making it easier to build, manage, and grow applications.
What is Firebase and why should I use it with Android?
Firebase is a platform by Google that helps developers build apps faster. It offers tools for authentication, database management, analytics, and more. Using Firebase with Android can make your app development smoother and more efficient.
How do I set up Firebase in my Android project?
First, go to the Firebase Console and create a new project. Then, add your Android app to the project by following the steps provided. Download the google-services.json file and place it in your app's root directory. Finally, add the necessary dependencies to your build.gradle files.
What are the main features of Firebase that can benefit my Android app?
Firebase offers a range of features like Realtime Database, Cloud Firestore, Authentication, Cloud Messaging, Crashlytics, and Analytics. These tools help with data storage, user authentication, push notifications, crash reporting, and tracking user behavior.
How does Firebase Authentication work with Android?
Firebase Authentication provides backend services to help authenticate users easily. It supports various methods like email/password, phone numbers, and third-party providers like Google and Facebook. You can integrate it into your Android app using the Firebase Authentication SDK.
Can I use Firebase for both iOS and Android apps?
Absolutely! Firebase is a cross-platform tool, so you can use it for both iOS and Android apps. This makes it easier to manage your app's backend services across different platforms.
How does Firebase Realtime Database differ from Cloud Firestore?
Firebase Realtime Database is a NoSQL database that stores data in JSON format and syncs it in real-time. Cloud Firestore is also a NoSQL database but offers more advanced features like queries, offline support, and better scalability. Choose based on your app's needs.
Is Firebase free to use?
Firebase offers a free tier with limited usage for most of its services. However, if your app grows and requires more resources, you might need to switch to a paid plan. Check the Firebase pricing page for detailed information.