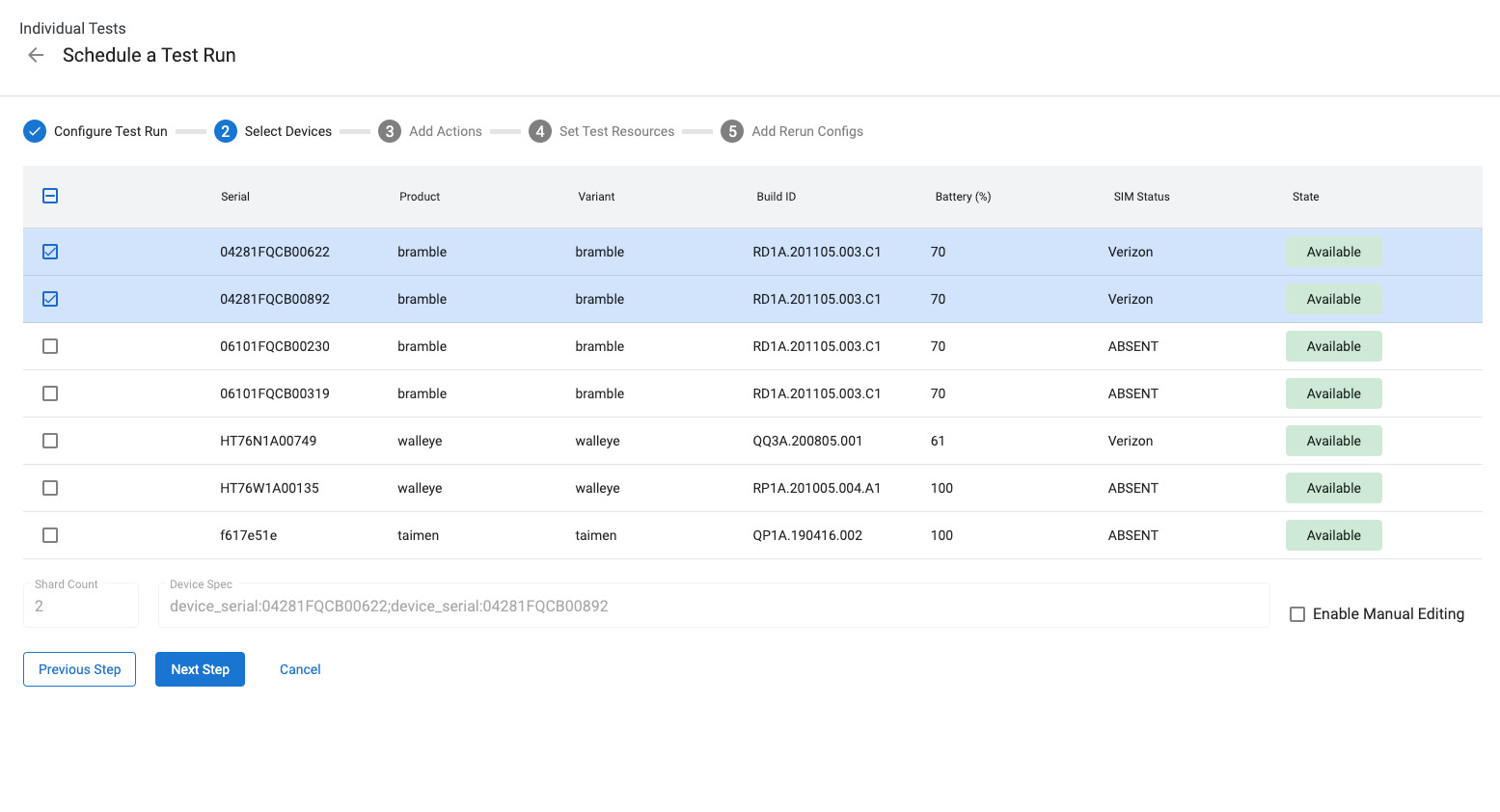
Introduction
In Android development, ensuring application quality and reliability is paramount. Testing plays a critical role in this process. Android Testing Tool Hub offers a suite of tools designed to streamline and enhance testing for Android applications. This article explores what the Android Testing Tool Hub offers, its components, and how to effectively utilize these tools to improve your development workflow.
What is Android Testing Tool Hub?
Android Testing Tool Hub is a collection of testing tools and frameworks provided by Google to help developers create robust and reliable Android applications. It includes various tools catering to different stages of the testing process, from unit testing to UI testing and performance testing. The hub integrates seamlessly with Android Studio, making it an essential part of any Android developer's toolkit.
Components of Android Testing Tool Hub
JUnit
Overview: JUnit is a popular unit testing framework for Java, widely used in Android development to write unit tests for individual components.
Usage: Add the JUnit test runner to your build.gradle file:
groovy
testImplementation 'junit:junit:4.13.2'
Example: A simple JUnit test for an Android class:
java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
public class MyClassTest {
@Test
public void testMyMethod() {
MyClass myClass = new MyClass();
String result = myClass.myMethod();
assertEquals("Expected result", result);
}
}
Espresso
Overview: Espresso is a UI testing framework provided by Google, allowing you to write robust UI tests covering various scenarios.
Usage: Add the Espresso test runner to your build.gradle file:
groovy
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
Example: A simple Espresso test for an Android activity:
java
import androidx.test.espresso.Espresso.onView;
import androidx.test.espresso.assertion.ViewAssertions.matches;
import androidx.test.espresso.matcher.ViewMatchers.withId;
import androidx.test.espresso.matcher.ViewMatchers.withText;
public class MainActivityTest {
@Test
public void testTextView() {
onView(withId(R.id.textView)).check(matches(withText("Hello World")));
}
}
Robolectric
Overview: Robolectric allows you to run JUnit tests directly on the JVM without needing an emulator or device, making it faster and more efficient for unit testing.
Usage: Add the Robolectric dependency to your build.gradle file:
groovy
testImplementation 'org.robolectric:robolectric:4.4'
Example: A simple Robolectric test for an Android class:
java
import org.junit.Test;
import static org.junit.Assert.assertEquals;
@Config(manifest = "src/test/resources/AndroidManifest.xml")
public class MyClassTest {
@Test
public void testMyMethod() {
MyClass myClass = new MyClass();
String result = myClass.myMethod();
assertEquals("Expected result", result);
}
}
UI Automator
Overview: UI Automator is a tool for automating UI tests on Android devices, allowing interaction with UI elements.
Usage: Create a test class extending UiAutomatorTestCase:
java
import android.support.test.uiautomator.By;
import android.support.test.uiautomator.UiAutomator;
import android.support.test.uiautomator.UiObject;
import android.support.test.uiautomator.UiObject2;
import android.support.test.uiautomator.UiSelector;
public class MainActivityTest extends UiAutomatorTestCase {
@Override
public void test() throws UiObjectNotFoundException {
UiObject2 button = UiObject2.getInstance(new UiSelector().className("android.widget.Button"));
button.click();
}
}
Monkey
Overview: Monkey generates pseudo-random events to test application stability, simulating user interactions like touches, gestures, and key presses.
Usage: Run Monkey from the command line:
sh
adb shell monkey -p
Example: Running Monkey with specific parameters:
sh
adb shell monkey -p com.example.app –throttle 500 –pct-touch 50 –pct-motion 20 –pct-appswitch 10 –pct-anyevent 10 -v 10000
Android Debug Bridge (ADB)
Overview: ADB is a command-line tool allowing interaction with your Android device or emulator from your development machine, essential for debugging and testing.
Usage: Run various commands to manage your device or emulator:
sh
List connected devices
adb devices
Install an APK on a device
adb install
Uninstall an APK from a device
adb uninstall
Run a command on a device
adb shell
Integrating Android Testing Tool Hub with Android Studio
Setting Up Your Project
Set up your project in Android Studio by creating a new project or opening an existing one. Add the necessary dependencies for testing in your build.gradle file.
Creating Tests
Create tests using the various tools provided by the Android Testing Tool Hub. For example, to write a JUnit test, create a new test class and add the necessary annotations and assertions.
Running Tests
Use the built-in test runner in Android Studio to run your tests. Right-click on your test class and select "Run 'testMyClassTest'" or use the "Run" button in the toolbar.
Debugging Tests
Debugging is an essential part of the testing process. Android Studio provides robust debugging tools that allow you to step through your code and identify issues. Set breakpoints in your test code and use the debugger to inspect variables and understand why a test is failing.
Best Practices for Using Android Testing Tool Hub
-
Write Comprehensive Tests: Ensure your application works as expected by writing comprehensive tests, including unit tests, UI tests, and performance tests.
-
Use Mocking Libraries: Mocking libraries like Mockito can help isolate dependencies in your code, making it easier to write unit tests.
-
Test-Driven Development (TDD): TDD involves writing tests before writing the actual code, ensuring your code is testable and meets required specifications.
-
Run Tests Regularly: Regularly running tests helps catch bugs early in the development cycle, reducing the likelihood of downstream issues.
-
Use Continuous Integration/Continuous Deployment (CI/CD): Integrate your testing process with CI/CD pipelines to ensure every change in the codebase is thoroughly tested before deployment.
-
Document Your Tests: Documenting your tests helps other developers understand how the application works and how it should be tested.
By following these best practices and utilizing the tools provided by the Android Testing Tool Hub, you can significantly enhance the quality of your Android applications and ensure they meet the highest standards of reliability and performance.