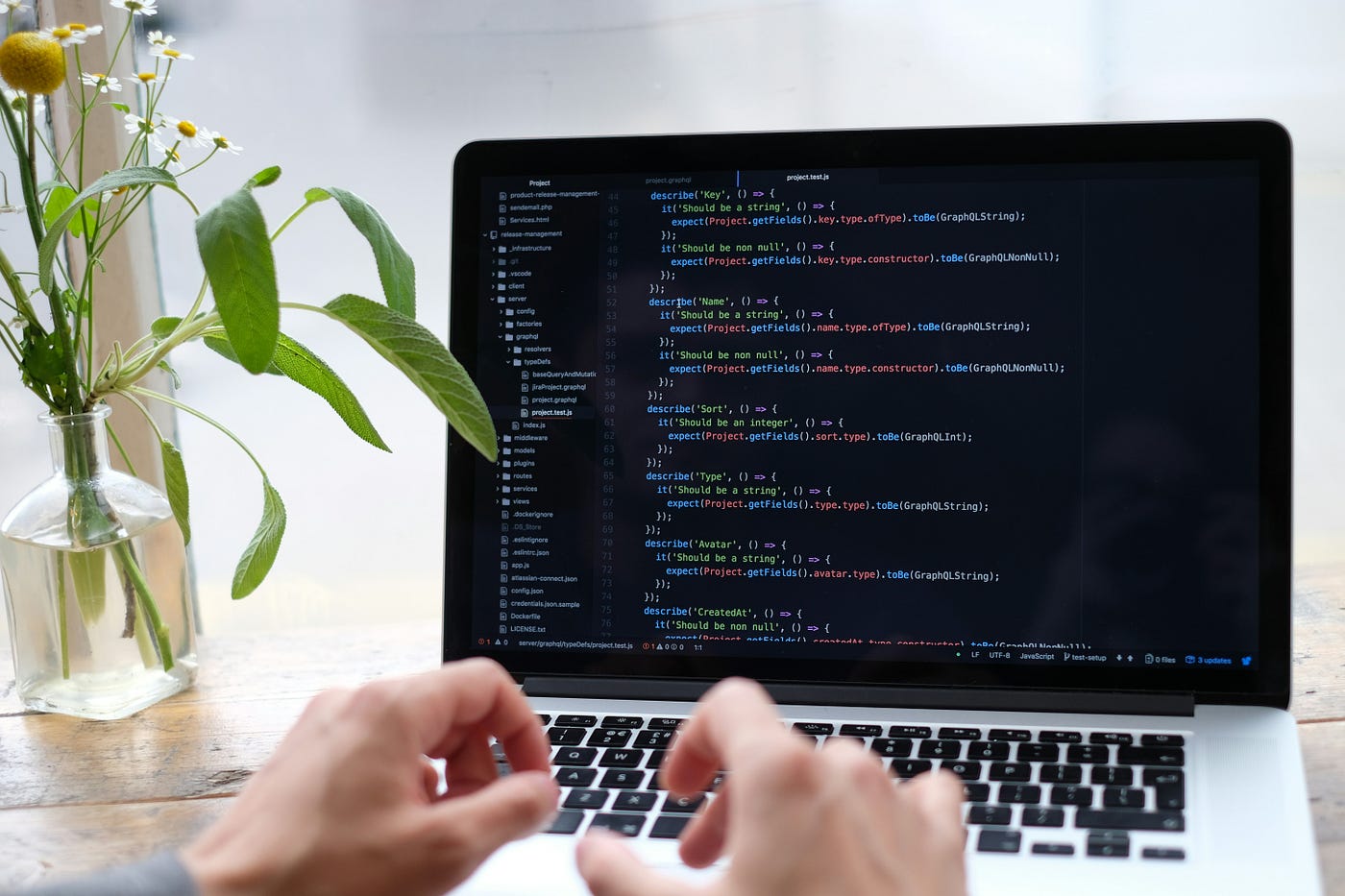
Introduction
Android devices have become an integral part of our daily lives, whether for communication, entertainment, or productivity. However, like any complex system, Android devices can sometimes encounter issues that require advanced troubleshooting and debugging techniques. This guide is designed to help you navigate the world of Android debugging using various tools and apps available for your device.
Understanding Android Debugging
Before diving into the specifics of using debug apps, it's essential to understand what Android debugging entails. Debugging involves identifying and resolving errors or bugs in software. In the context of Android, this means identifying issues with the operating system, apps, or hardware components.
Android provides several built-in tools for debugging, such as the Android Debug Bridge (ADB) and the Android Studio debugger. However, these tools are primarily designed for developers and may not be accessible to all users. Fortunately, numerous third-party apps offer user-friendly interfaces for debugging various aspects of your Android device.
Choosing the Right Debug App
When selecting a debug app, consider the specific issues you're experiencing with your device. Different apps specialize in different areas of debugging, so it's crucial to choose one that aligns with your needs.
System Performance Issues
-
CPU-Z and GPU-Z
- Monitor the performance of your device's CPU and GPU.
- Provide detailed information about hardware specifications and current usage levels.
-
Greenify and RAM Manager
- Assist in managing memory usage.
- Close background applications consuming resources unnecessarily.
Battery Life
-
Battery Doctor
- Monitor battery health.
- Identify power-hungry apps.
-
DU Battery Saver
- Offer automatic power-saving modes.
- Provide detailed battery usage reports.
Network Issues
-
Network Signal Info
- Provide detailed information about network signal strength and type (2G, 3G, 4G).
- Offer other network-related metrics.
-
Wi-Fi Analyzer
- Identify nearby Wi-Fi networks and their signal strengths.
- Optimize Wi-Fi connectivity.
Storage Management
-
Disk Cleanup and Storage Analyzer
- Identify and delete unnecessary files.
- Free up storage space on your device.
-
ES File Explorer
- Offer advanced file management features.
- Move files between internal and external storage.
Security Concerns
-
Malwarebytes and Avast Mobile Security
- Scan your device for malware and other security threats.
-
Find My Device
- Locate your device if it's lost or stolen.
Customization and Tweaks
-
Xposed Framework and GravityBox
- Customize various aspects of your device's interface and behavior.
-
Tasker
- Automate tasks based on specific conditions or triggers.
Using Duet Display for Second Monitor Setup
While this guide primarily focuses on debugging tools, it's worth mentioning an app that can significantly enhance your productivity: Duet Display. This app transforms your Android tablet into a second monitor for your computer, extending your desktop space and making multitasking easier.
Steps to Set Up Duet Display
-
Download and Install Duet Display
- Download the Duet Display app from the Google Play Store on your Android tablet.
- Install the app and open it.
-
Connect Your Devices
- On your computer, download and install the Duet Display software from their official website.
- Connect both devices to the same Wi-Fi network.
-
Pair Your Devices
- Open the Duet Display app on your Android tablet and follow the in-app instructions to pair it with your computer.
- Grant permission for the app to access your device's display settings if needed.
-
Configure Display Settings
- Adjust the display settings in both the app and the computer software to optimize the layout and resolution of your second monitor.
-
Start Using Your Tablet as a Second Monitor
- With some tweaks in display settings and battery management, create a seamless multi-monitor experience.
- Drag windows between your main screen and the tablet, making multitasking easier.
Tips for Effective Use of Your Tablet as a Monitor
Extend Your Workspace
Use your Android tablet to spread out multiple documents or apps. This helps when working on reports or research.
Boost Productivity
Place your email or chat app on the tablet while keeping your main screen for primary tasks. This way, you won't miss important messages.
Presentations
Use the tablet to show notes or slides while presenting on your main screen. This keeps you organized and professional.
Creative Projects
Artists can use the tablet for drawing or editing while keeping reference images on the main screen. This makes creative work smoother.
Gaming
Gamers can use the tablet for game maps or chat, freeing up the main screen for gameplay. This enhances the gaming experience.
Video Calls
Keep video calls on the tablet and use the main screen for taking notes or accessing documents. This keeps everything in view.
Coding
Developers can code on the main screen and use the tablet for documentation or testing. This setup improves efficiency.
Entertainment
Watch videos or stream music on the tablet while browsing or working on the main screen. This keeps entertainment separate from tasks.
Travel
On the go, use the tablet as a second screen for your laptop. This makes working from anywhere more effective.
Study Sessions
Students can use the tablet for textbooks or research while writing essays on the main screen. This helps manage study materials better.
Virtual Meetings
Keep meeting agendas or notes on the tablet while participating in virtual meetings on the main screen. This keeps you prepared and engaged.
Social Media
Use the tablet for social media updates while keeping the main screen for work. This balances productivity and leisure.
Photo Editing
Photographers can use the tablet for editing tools while viewing the full image on the main screen. This enhances precision.
Stock Trading
Traders can monitor stocks on the tablet while making trades on the main screen. This setup allows quick decisions.
Reading
Use the tablet for reading articles or eBooks while taking notes on the main screen. This makes learning more interactive.
Troubleshooting Common Problems
Screen Flickering
Screen flickering can be annoying. Check the cable connection first. If the cable is loose or damaged, it may cause flickering issues. Ensure that all cables are securely connected.
Display Settings
Sometimes, display settings might not be optimized for your setup. Adjusting the resolution and refresh rate of your second monitor can resolve display issues.
Battery Drain
Using a second monitor can drain your tablet's battery faster. Adjusting the brightness and turning off unnecessary features can help manage battery life.
App Compatibility
Some apps might not be compatible with your second monitor setup. Check the app's documentation or contact the developer for support.
Additional Resources
- Android Debug Bridge (ADB): A command-line tool for debugging Android devices.
- Android Studio Debugger: An integrated development environment (IDE) for developers to debug their apps.
- Xposed Framework: A framework that allows users to customize their Android devices by modifying system applications without needing to root their device.
- Tasker: An automation tool that allows users to create custom tasks based on specific conditions or triggers.
By leveraging these tools and apps, you'll be well-equipped to handle any debugging task that comes your way, ensuring your Android device runs smoothly and efficiently.