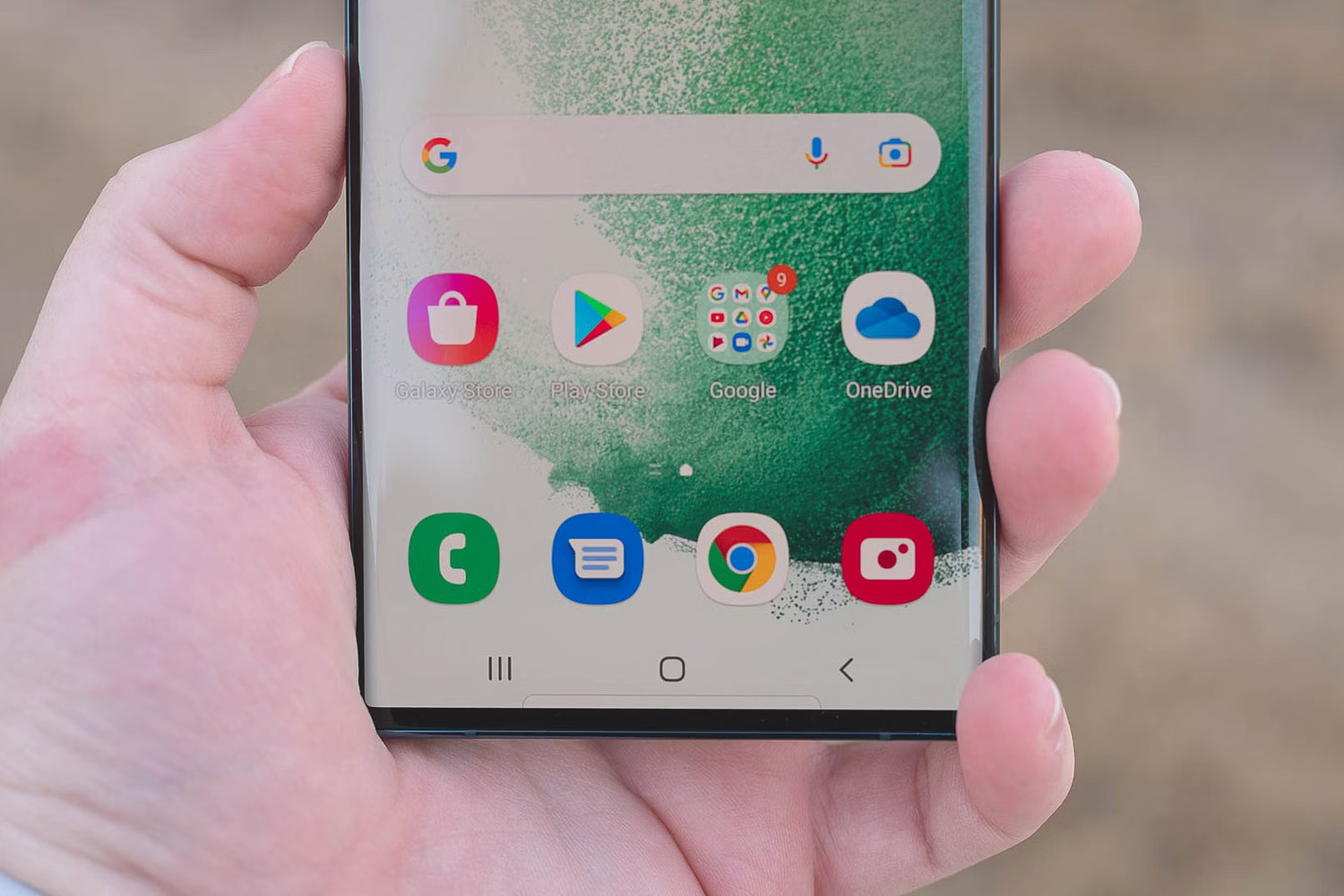
Setting Up Your Development Environment
Before adding buttons, set up your development environment with these steps:
-
Install Android Studio:
- Visit the official Android Studio website to download the latest version.
- Follow the installation instructions provided by the installer.
-
Create a New Project:
- Launch Android Studio.
- Click "Start a new Android Studio project."
- Choose "Empty Activity" as the project template.
- Fill in the required details such as project name, package name, and location.
- Click "Next" and then "Finish."
-
Understand the Project Structure:
- Your project will have several directories and files.
- The
app
directory contains all the source code for your app. - The
res
directory holds all the resources like layouts, strings, and drawables. - The
java
directory contains the Java classes for your app.
Understanding Layouts in Android
Layouts define the user interface of your application. The most common type is activity_main.xml
, the default layout file created when starting a new project.
Creating a New Layout File
To create a custom layout file, follow these steps:
-
Navigate to the
res/layout
directory:- In the Project window, navigate to
app/src/main/res/layout
.
- In the Project window, navigate to
-
Create a New Layout File:
- Right-click on the
layout
directory and select "New" > "Layout resource file." - Name your layout file (e.g.,
custom_layout.xml
) and click "OK."
- Right-click on the
-
Design Your Layout:
- Open the newly created
custom_layout.xml
file. - Use the Layout Editor tool provided by Android Studio to design your layout.
- Open the newly created
Adding Buttons to Your Layout
After creating your layout file, add buttons with these steps:
-
Open Your Layout File:
- Navigate to the
res/layout
directory and open your desired layout file (e.g.,activity_main.xml
).
- Navigate to the
-
Drag and Drop Button:
- In the Layout Editor, click on the "Palette" tab on the left side of the screen.
- Find the "Button" component in the Palette and drag it onto your layout.
-
Configure Button Properties:
- After dropping the button, configure its properties in the Attributes panel on the right side of the screen.
- Change the text, background color, and other properties as needed.
-
Add Multiple Buttons:
- To add multiple buttons, drag and drop another button from the Palette onto your layout.
- Arrange them as needed using the layout tools provided by Android Studio.
Writing Code for Button Click Events
Once buttons are added to your layout, write code to handle their click events.
Using XML Attributes
Handle button click events directly in your XML layout file using the android:onClick
attribute:
xml
In your MainActivity.java
file, define the onButtonClick
method:
java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = findViewById(R.id.button);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button click event here
Toast.makeText(MainActivity.this, "Button clicked!", Toast.LENGTH_SHORT).show();
}
});
}
}
Using Kotlin
Handle button click events similarly in Kotlin:
kotlin
class MainActivity : AppCompatActivity() {
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
val button = findViewById<Button>(R.id.button)
button.setOnClickListener {
// Handle button click event here
Toast.makeText(this, "Button clicked!", Toast.LENGTH_SHORT).show()
}
}
}
Using OnClickListener Interface
Another way to handle button click events is by implementing the OnClickListener
interface:
java
public class MainActivity extends AppCompatActivity implements View.OnClickListener {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button = findViewById(R.id.button);
button.setOnClickListener(this);
}
@Override
public void onClick(View v) {
// Handle button click event here
Toast.makeText(MainActivity.this, "Button clicked!", Toast.LENGTH_SHORT).show();
}
}
Advanced Techniques
Customizing Button Appearance
Customize the appearance of your buttons by changing their properties in the XML file or programmatically in your code.
Customizing Button Text
Change the text of a button programmatically using the setText
method:
java
Button button = findViewById(R.id.button);
button.setText("New Text");
Customizing Button Background
Change the background color of a button programmatically using the setBackgroundColor
method:
java
Button button = findViewById(R.id.button);
button.setBackgroundColor(Color.RED);
Customizing Button Size
Change the size of a button programmatically using the setLayoutParams
method:
java
Button button = findViewById(R.id.button);
LinearLayout.LayoutParams layoutParams = new LinearLayout.LayoutParams(
LinearLayout.LayoutParams.WRAP_CONTENT,
LinearLayout.LayoutParams.WRAP_CONTENT
);
button.setLayoutParams(layoutParams);
Handling Multiple Button Clicks
Handle multiple button clicks in different ways.
Using Different Methods for Different Buttons
Define different methods for different buttons using the android:onClick
attribute with different method names:
xml
Then, define these methods in your activity:
java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
}
public void onButton1Click(View view) {
// Handle button 1 click event here
Toast.makeText(MainActivity.this, "Button 1 clicked!", Toast.LENGTH_SHORT).show();
}
public void onButton2Click(View view) {
// Handle button 2 click event here
Toast.makeText(MainActivity.this, "Button 2 clicked!", Toast.LENGTH_SHORT).show();
}
}
Using OnClickListener Interface
Alternatively, use the OnClickListener
interface to handle multiple button clicks:
java
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button button1 = findViewById(R.id.button1);
Button button2 = findViewById(R.id.button2);
button1.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button 1 click event here
Toast.makeText(MainActivity.this, "Button 1 clicked!", Toast.LENGTH_SHORT).show();
}
});
button2.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
// Handle button 2 click event here
Toast.makeText(MainActivity.this, "Button 2 clicked!", Toast.LENGTH_SHORT).show();
}
});
}
}
Tips and Tricks
Using Layouts Efficiently
- Use ConstraintLayout: ConstraintLayout allows you to define constraints between views, making it easier to manage complex layouts.
- Avoid Nested Layouts: Nested layouts can increase the complexity of your UI and slow down performance. Try to avoid them unless necessary.
- Use Layout Inheritance: If multiple layouts share common elements, consider using layout inheritance to avoid duplicating code.
Optimizing Performance
- Minimize findViewById Calls: Avoid calling
findViewById
multiple times in the same method. Store the result in a variable to improve performance. - Use Data Binding: Data Binding allows you to bind data to your views directly in your layout files, reducing the need for findViewById calls.
Debugging Button Click Events
- Use Log Statements: Add log statements in your onClick methods to debug issues related to button clicks.
- Use Debugging Tools: Android Studio provides various debugging tools like the Debugger and the Layout Inspector that can help you identify issues with your UI and button click events.
Adding buttons to your Android application involves designing your layout and writing code to handle button click events. By following the tips and tricks outlined in this article, you can create efficient and user-friendly UIs that enhance the overall experience of your app. Whether you're a beginner or an experienced developer, mastering the art of adding buttons is essential for building robust and engaging Android applications.