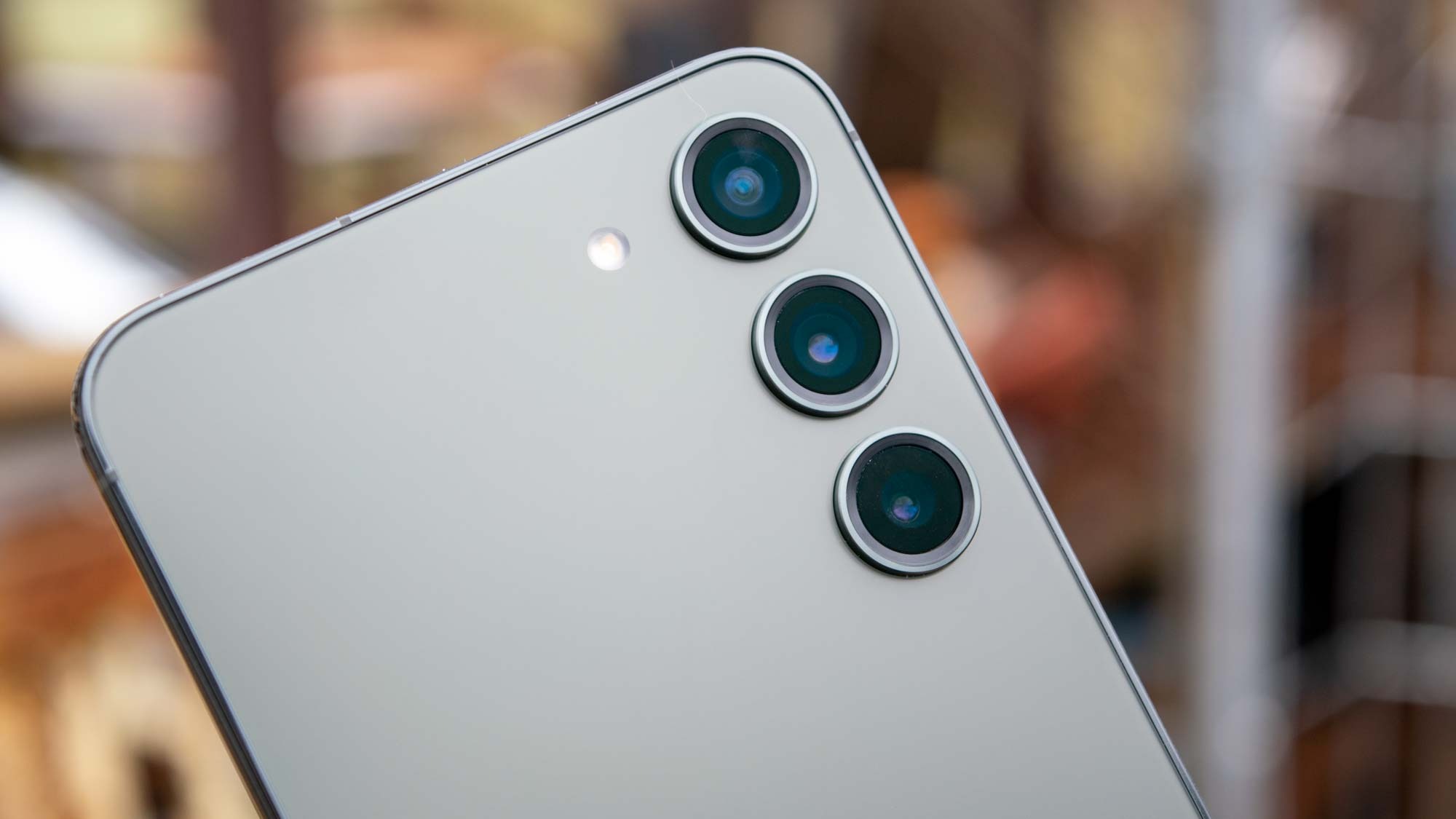
Introduction
In today's digital age, security is a top priority for all devices, especially those with sensitive features like cameras. Android devices often come equipped with high-resolution cameras that can capture a wide range of data. However, this increased functionality also raises significant security concerns. Implementing an Android security policy for camera use is crucial to protect your device and its users from potential threats. This article will focus on how to implement these policies specifically for camera use.
What Are Android Security Policies?
Android security policies are rules set to protect your device and its users from various threats. These policies can be applied at different levels, including the operating system, applications, and hardware components like the camera. The primary goal of these policies is to ensure that the device operates within a defined set of secure parameters, minimizing the risk of unauthorized access or data breaches.
Types of Android Security Policies
-
Device-Level Policies: Applied at the device level, these settings include screen lock timeouts, encryption, and app permissions. For camera use, device-level policies might involve settings like requiring a PIN or fingerprint scan to access the camera.
-
Application-Level Policies: Applied to individual applications, these include permissions, data encryption, and secure coding practices. For camera use, application-level policies might involve requesting specific permissions (e.g.,
CAMERA
permission) and ensuring that the app handles camera data securely. -
Network-Level Policies: Applied at the network level, these settings include VPN usage, firewall configurations, and network encryption. While not directly related to camera use, network-level policies can indirectly impact camera security by ensuring that data transmitted over the network is encrypted.
Why Implement Android Security Policies for Camera Use?
Implementing Android security policies for camera use is essential for several reasons:
- Data Protection: Cameras can capture sensitive information, including personal photos, videos, and even biometric data. Ensuring that this data is protected from unauthorized access is critical.
- Privacy: Users have a right to privacy, and camera usage can often infringe on this right if not properly managed. Implementing security policies helps maintain user privacy by controlling who can access the camera and under what conditions.
- Compliance: Many industries have strict regulations regarding data privacy and security. Implementing robust security policies can help organizations comply with these regulations.
- Reputation: Companies that fail to protect user data may suffer reputational damage. Implementing strong security policies demonstrates a commitment to user security and privacy.
Steps to Implement Android Security Policy for Camera Use
Understand the Permissions System
Android's permission system is a fundamental aspect of its security model. The CAMERA
permission is required for any app that wants to access the device's camera. Understanding how this permission works is crucial for implementing effective security policies.
Requesting Permissions
When an app requests the CAMERA
permission, it must provide a clear explanation of why it needs access to the camera. Users are then prompted to grant or deny this permission. This two-step process ensures that users are aware of what data is being accessed and can make informed decisions about their privacy.
Handling Permission Requests
Developers should handle permission requests responsibly. For example, if an app requires the CAMERA
permission but does not use it immediately, it should request the permission only when necessary to avoid unnecessary prompts to the user.
Implement Data Encryption
Data encryption is a powerful tool in protecting sensitive information captured by the camera. Here’s how you can implement data encryption:
Using Android’s Built-in Encryption
Android provides built-in encryption mechanisms that can be used to protect camera data. For example, you can use the File
class to encrypt files containing camera data.
java
import java.io.File;
import java.io.FileInputStream;
import java.io.FileOutputStream;
import java.io.IOException;
import java.security.KeyStore;
import java.security.KeyStoreException;
import java.security.NoSuchAlgorithmException;
import java.security.cert.CertificateException;
import javax.crypto.Cipher;
import javax.crypto.KeyGenerator;
import javax.crypto.SecretKey;
import javax.crypto.spec.SecretKeySpec;
public class CameraDataEncryptor {
public static void main(String[] args) throws Exception {
// Generate a secret key
KeyGenerator keyGen = KeyGenerator.getInstance("AES");
keyGen.init(128);
SecretKey secretKey = keyGen.generateKey();
// Encrypt the file
File inputFile = new File("path/to/input/file");
File outputFile = new File("path/to/output/file");
Cipher cipher = Cipher.getInstance("AES");
cipher.init(Cipher.ENCRYPT_MODE, secretKey);
FileInputStream fis = new FileInputStream(inputFile);
FileOutputStream fos = new FileOutputStream(outputFile);
byte[] buffer = new byte[1024];
int bytesRead;
while ((bytesRead = fis.read(buffer)) != -1) {
byte[] encryptedBuffer = cipher.update(buffer, 0, bytesRead);
fos.write(encryptedBuffer);
}
byte[] encryptedFinalBlock = cipher.doFinal();
fos.write(encryptedFinalBlock);
fis.close();
fos.close();
}
}
Using Third-Party Libraries
Several third-party libraries can simplify the process of encrypting and decrypting data on Android. For example, the CryptoUtils
library provides a simple way to encrypt and decrypt files.
Limit Camera Access
Limiting camera access is another crucial step in implementing an effective security policy. Here’s how you can limit camera access:
Using Conditional Permissions
Conditional permissions allow you to request permissions only when certain conditions are met. For example, you can request the CAMERA
permission only when the user is actively using the camera app.
java
import android.content.Context;
import android.content.pm.PackageManager;
import android.os.Build;
import android.util.Log;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
public class CameraPermissionChecker {
public static boolean hasCameraPermission(Context context) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
return ContextCompat.checkSelfPermission(context, android.Manifest.permission.CAMERA) == PackageManager.PERMISSION_GRANTED;
} else {
return true; // Permission is implicitly granted on older versions
}
}
public static void requestCameraPermission(Context context, int requestCode) {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M && !hasCameraPermission(context)) {
ActivityCompat.requestPermissions((Activity) context, new String[]{android.Manifest.permission.CAMERA}, requestCode);
}
}
}
Using Secure Coding Practices
Secure coding practices are essential for ensuring that your app handles camera data securely. Here are some best practices:
- Validate User Input: Always validate user input to prevent malicious data from being processed.
- Use Secure Protocols: Use secure communication protocols like HTTPS to protect data transmitted over the network.
- Implement Error Handling: Implement robust error handling mechanisms to prevent crashes and data leaks.
Monitor and Audit Camera Usage
Monitoring and auditing camera usage is critical for detecting potential security breaches. Here’s how you can monitor and audit camera usage:
Using Logging Mechanisms
Logging mechanisms can help track camera usage and detect any suspicious activity. You can use Android’s built-in logging mechanisms like Logcat or third-party logging libraries like Timber.
java
import android.util.Log;
public class CameraLogger {
public static void logCameraUsage(String message) {
Log.d("CameraUsage", message);
}
}
Using Analytics Tools
Analytics tools can provide insights into how the camera is being used within your app. You can use Google Analytics or other third-party analytics tools to track camera usage.
java
import com.google.firebase.analytics.FirebaseAnalytics;
public class AnalyticsTracker {
private FirebaseAnalytics mFirebaseAnalytics;
public AnalyticsTracker(Context context) {
mFirebaseAnalytics = FirebaseAnalytics.getInstance(context);
}
public void trackCameraEvent(String event) {
Bundle bundle = new Bundle();
bundle.putString(FirebaseAnalytics.Param.ITEM_ID, event);
mFirebaseAnalytics.logEvent("CameraEvent", bundle);
}
}
Final Thoughts
Implementing an Android security policy for camera use involves understanding the permissions system, implementing data encryption, limiting camera access, and monitoring and auditing usage. By following these steps, developers can ensure that their apps handle camera data securely and protect user privacy. In today’s landscape where data breaches are common, prioritizing security when developing apps that use sensitive features like cameras is essential.