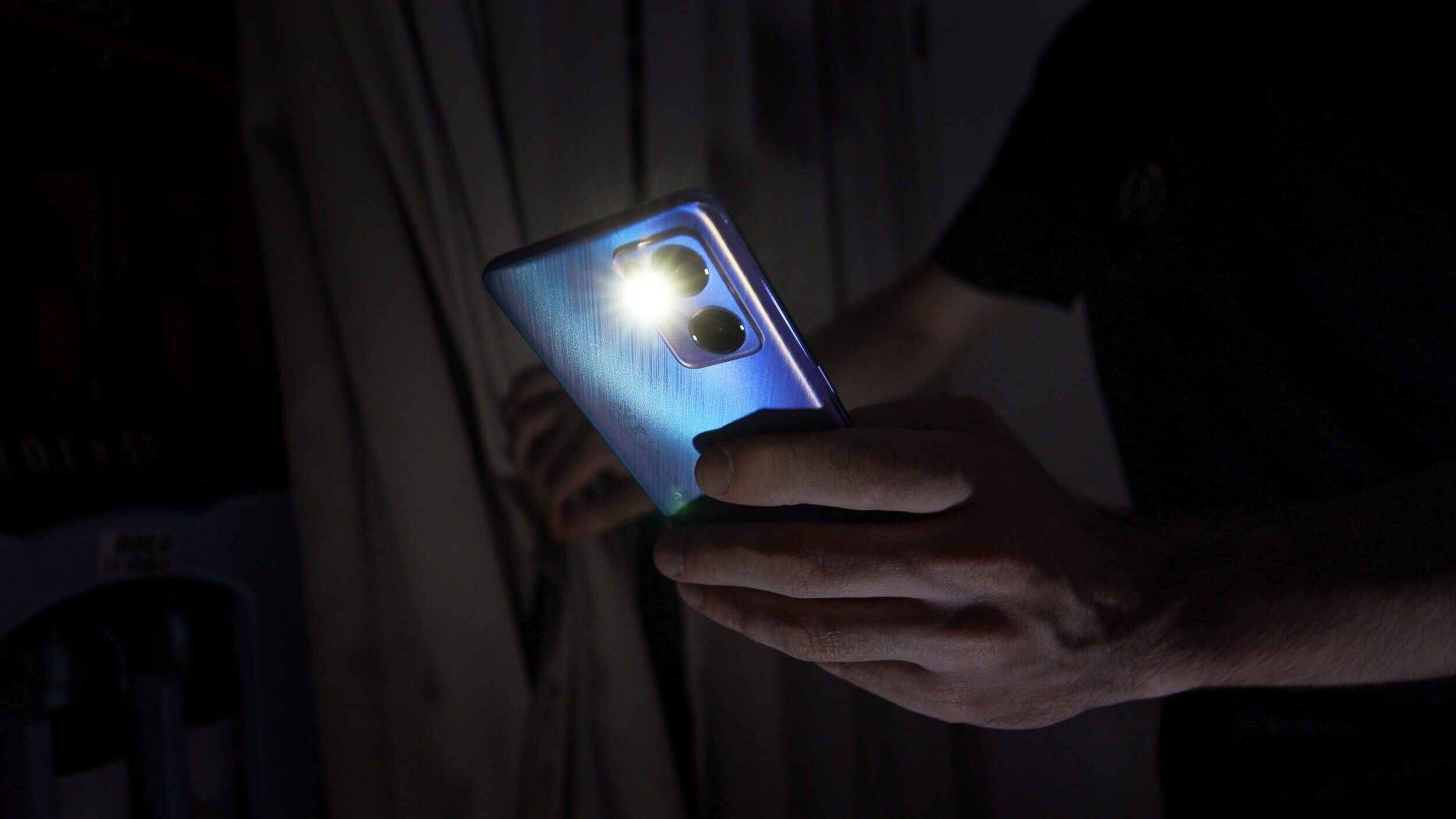
Introduction
In today's digital age, smartphones have become essential tools for daily life. One of the most useful features of a smartphone is the flashlight, which can be activated using various methods. This article will focus on how to implement a flashlight feature in an Android application programmatically. We will cover the necessary permissions, steps to check if a device has a flashlight, and code snippets to turn the flashlight on and off.
Setting Up the Project
Before diving into the code, let's set up a new Android project in Android Studio. This will help understand the basic structure of an Android application and where the necessary code will go.
Step 1: Create a New Project
- Open Android Studio: Launch Android Studio and click on "Start a new Android Studio project."
- Choose the Project Template: Select "Empty Activity" under the "Android" section.
- Configure the Project: Enter your application name, company domain, and package name. For example, name it "FlashlightApp" and set the package name to "com.example.flashlightapp."
- Set Minimum SDK: Choose the minimum SDK version you want to support. For this example, keep it at the default setting.
- Finish the Setup: Click "Finish" to create the project.
Step 2: Add Permissions in the Manifest File
To access the camera and flashlight features, add the necessary permissions to the AndroidManifest.xml
file.
xml
These permissions are required to access the camera and flashlight features of the device.
Checking for Flashlight Availability
Before turning on the flashlight, check if the device has a flashlight available. Use the PackageManager
to check for this feature.
java
Boolean isFlashAvailable = getApplicationContext().getPackageManager()
.hasSystemFeature(PackageManager.FEATURE_CAMERA_FLASH);
This code snippet checks if the device has a flashlight feature available. If it does, it returns true
; otherwise, it returns false
.
Implementing the Flashlight Feature
Now that the project is set up and the availability of the flashlight is checked, let's implement the code to turn the flashlight on and off.
Step 3: Initialize Camera Manager
To control the flashlight programmatically, use the CameraManager
API. This API is available from Android 5.0 (API level 21) onwards.
java
CameraManager camManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
try {
String[] cameraIds = camManager.getCameraIdList();
if (cameraIds.length > 0) {
String cameraId = cameraIds[0];
camManager.setTorchMode(cameraId, true);
}
} catch (CameraAccessException e) {
e.printStackTrace();
}
This code initializes the CameraManager
, retrieves the list of camera IDs, and sets the torch mode to true
to turn on the flashlight.
Step 4: Toggle Flashlight On and Off
To create a toggle button that turns the flashlight on and off, handle button clicks and call the setTorchMode
method accordingly.
java
ivOnOFF.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
if (isTorchOn) {
turnOffLight();
isTorchOn = false;
} else {
turnOnLight();
isTorchOn = true;
}
} catch (Exception e) {
e.printStackTrace();
}
}
});
This code snippet sets up an OnClickListener
for the toggle button. When the button is clicked, it checks the current state of the flashlight and calls either turnOffLight
or turnOnLight
method.
Step 5: Implementing Turn On and Turn Off Methods
The turnOnLight
and turnOffLight
methods are responsible for setting the torch mode to true
or false
, respectively.
java
private void turnOnLight() throws CameraAccessException {
camManager.setTorchMode(cameraId, true);
Toast.makeText(MainActivity.this, "Flashlight is turned ON", Toast.LENGTH_SHORT).show();
}
private void turnOffLight() throws CameraAccessException {
camManager.setTorchMode(cameraId, false);
Toast.makeText(MainActivity.this, "Flashlight is turned OFF", Toast.LENGTH_SHORT).show();
}
These methods use the CameraManager
to set the torch mode and display a toast message indicating whether the flashlight is turned on or off.
Handling Runtime Permissions
For Android versions above Marshmallow (API level 23), handle runtime permissions. This involves requesting permissions at runtime and checking if the user grants or denies them.
java
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (checkSelfPermission(Manifest.permission.CAMERA) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(new String[]{Manifest.permission.CAMERA}, 1);
}
if (checkSelfPermission(Manifest.permission.FLASHLIGHT) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(new String[]{Manifest.permission.FLASHLIGHT}, 2);
}
}
This code snippet checks if the required permissions are granted and requests them if not.
Complete Code Example
Here is a complete code example that includes all the steps discussed:
MainActivity.java
java
import android.Manifest;
import android.app.AlertDialog;
import android.content.Context;
import android.content.DialogInterface;
import android.content.pm.PackageManager;
import android.hardware.camera2.CameraAccessException;
import android.hardware.camera2.CameraManager;
import android.os.Build;
import android.os.Bundle;
import android.view.View;
import android.widget.ImageView;
import android.widget.Toast;
import androidx.appcompat.app.AppCompatActivity;
public class MainActivity extends AppCompatActivity {
private static final int REQUEST_CAMERA_PERMISSION = 1;
private static final int REQUEST_FLASHLIGHT_PERMISSION = 2;
private CameraManager camManager;
private String cameraId;
private boolean isTorchOn;
private ImageView ivOnOFF;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ivOnOFF = findViewById(R.id.ivOnOFF);
isTorchOn = false;
// Check if device has flashlight
Boolean isFlashAvailable = getApplicationContext().getPackageManager()
.hasSystemFeature(PackageManager.FEATURE_CAMERA_FLASH);
if (!isFlashAvailable) {
AlertDialog alert = new AlertDialog.Builder(MainActivity.this).create();
alert.setTitle(getString(R.string.app_name));
alert.setMessage(getString(R.string.msg_error));
alert.setButton(DialogInterface.BUTTON_POSITIVE, getString(R.string.lbl_ok), new DialogInterface.OnClickListener() {
public void onClick(DialogInterface dialog, int which) {
finish();
}
});
alert.show();
return;
}
// Initialize Camera Manager
camManager = (CameraManager) getSystemService(Context.CAMERA_SERVICE);
try {
cameraId = camManager.getCameraIdList()[0];
} catch (Exception e) {
e.printStackTrace();
}
// Request permissions if necessary
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.M) {
if (checkSelfPermission(Manifest.permission.CAMERA) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(new String[]{Manifest.permission.CAMERA}, REQUEST_CAMERA_PERMISSION);
}
if (checkSelfPermission(Manifest.permission.FLASHLIGHT) != PackageManager.PERMISSION_GRANTED) {
requestPermissions(new String[]{Manifest.permission.FLASHLIGHT}, REQUEST_FLASHLIGHT_PERMISSION);
}
}
// Set up toggle button click listener
ivOnOFF.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
try {
if (isTorchOn) {
turnOffLight();
isTorchOn = false;
} else {
turnOnLight();
isTorchOn = true;
}
} catch (Exception e) {
e.printStackTrace();
}
}
});
}
private void turnOnLight() throws CameraAccessException {
camManager.setTorchMode(cameraId, true);
Toast.makeText(MainActivity.this, "Flashlight is turned ON", Toast.LENGTH_SHORT).show();
}
private void turnOffLight() throws CameraAccessException {
camManager.setTorchMode(cameraId, false);
Toast.makeText(MainActivity.this, "Flashlight is turned OFF", Toast.LENGTH_SHORT).show();
}
@Override
public void onRequestPermissionsResult(int requestCode, String[] permissions, int[] grantResults) {
super.onRequestPermissionsResult(requestCode, permissions, grantResults);
if (requestCode == REQUEST_CAMERA_PERMISSION) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted, proceed with setting up the flashlight
} else {
Toast.makeText(this, "Camera permission not granted", Toast.LENGTH_SHORT).show();
}
} else if (requestCode == REQUEST_FLASHLIGHT_PERMISSION) {
if (grantResults.length > 0 && grantResults[0] == PackageManager.PERMISSION_GRANTED) {
// Permission granted, proceed with setting up the flashlight
} else {
Toast.makeText(this, "Flashlight permission not granted", Toast.LENGTH_SHORT).show();
}
}
}
}
activity_main.xml
xml
<TextView
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:layout_gravity="center"
android:layout_marginTop="100dp"
android:text="Flashlight"
android:textColor="@color/colorPrimary"
android:textSize="50sp"
android:textStyle="bold|italic" />
<!-- This is the simple divider between above TextView and ToggleButton -->
<View
android:layout_width="match_parent"
android:layout_height="1dp"
android:background="@color/colorAccent" />
<!-- Toggle Button to turn on/off the flashlight -->
<Button
android:id="@+id/ivOnOFF"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Toggle Flashlight" />
Additional Tips
- Customizing Lock Screen: Customize your lock screen to include a flashlight shortcut, providing quick access to the flashlight feature.
- Google Assistant: Use Google Assistant to activate the torch with voice commands.
- Shake to Switch: Third-party apps like Shake Flashlight allow you to turn on the flashlight by shaking your phone, which can be useful in emergency situations.
By integrating these features into your app, you can provide users with multiple ways to access and control the flashlight, enhancing the overall user experience.