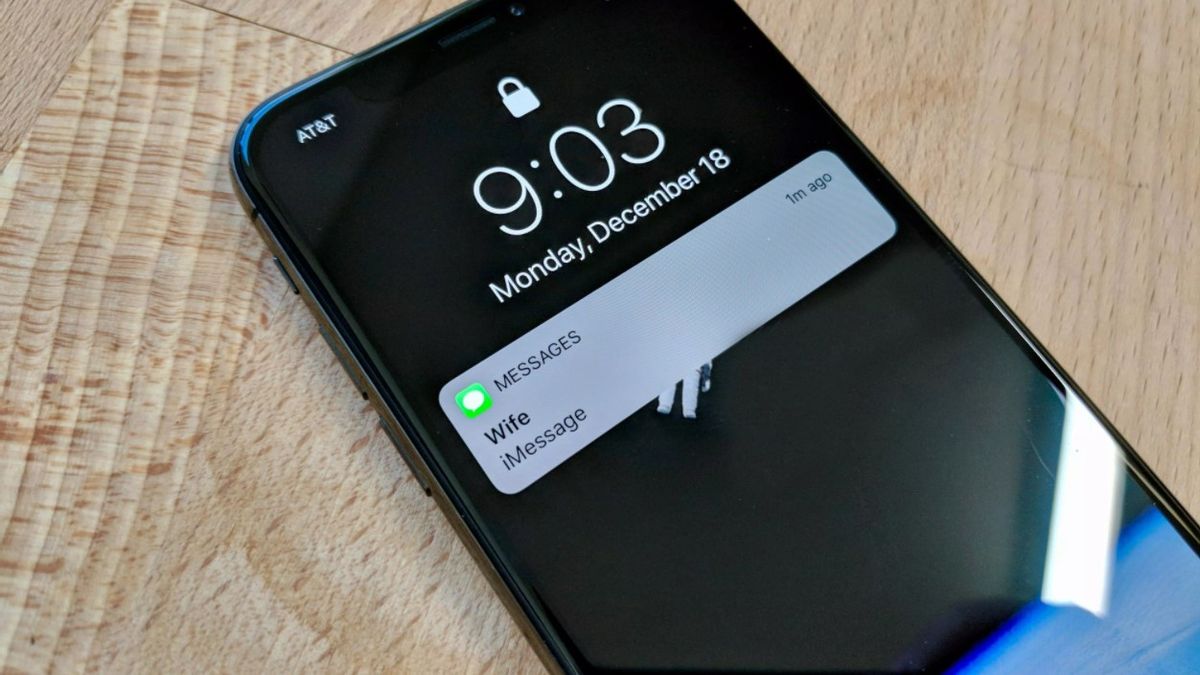
Introduction
Notifications have become a crucial aspect of user engagement in mobile technology. Android, one of the most popular mobile operating systems, offers a wide range of features to customize and enhance app notifications. This guide will walk you through creating custom app notifications on Android, providing tools and techniques to improve user interaction and app functionality.
Understanding Android Notifications
Before diving into the customization process, understanding the basics of Android notifications is essential. Android notifications are visual indicators that alert users to specific events or updates within an app. They can be displayed on the lock screen, in the notification shade, or as a heads-up notification.
Types of Android Notifications
- Basic Notifications: Standard notifications that most apps use, typically including a title, message, and an optional icon.
- Rich Notifications: Allow for more complex content, such as images, videos, and interactive elements like buttons and carousels.
- Channel-Based Notifications: Introduced in Android Oreo (8.0), these notifications allow developers to group similar notifications into channels, making it easier for users to manage their notifications.
Setting Up Your Development Environment
To create custom app notifications, setting up your development environment is necessary. Follow these steps:
-
Install Android Studio:
- Download and install Android Studio from the official website.
- Follow the installation instructions to set up the IDE.
-
Create a New Project:
- Launch Android Studio and create a new project.
- Choose the type of project you want to create (e.g., Empty Activity).
- Name your project and set up the project structure.
-
Add Dependencies:
- For creating custom notifications, additional dependencies like
androidx.core:core-ktx
andcom.google.android.material:material
might be needed. - Add these dependencies in your
build.gradle
file.
- For creating custom notifications, additional dependencies like
-
Set Up Your Project Structure:
- Create necessary folders and files for your project (e.g., layout files, Java/Kotlin classes).
Creating Basic Notifications
Basic notifications form the foundation of any notification system. Here’s how to create them:
-
Declare Notification Permissions:
- In your
AndroidManifest.xml
, declare the necessary permissions for sending notifications:
xml
- In your
-
Create a Notification Channel:
- For Android Oreo and later, create a notification channel:
java
private void createNotificationChannel() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
NotificationChannel channel = new NotificationChannel(
"your_channel_id",
"Your Channel Name",
NotificationManager.IMPORTANCE_DEFAULT
);
channel.setDescription("Your Channel Description");
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.createNotificationChannel(channel);
}
}
}
- For Android Oreo and later, create a notification channel:
-
Create a Notification Builder:
- Use the
NotificationCompat.Builder
class to build your notification:
java
private void showNotification() {
Intent intent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.your_icon)
.setContentTitle("Your Title")
.setContentText("Your Message")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setContentIntent(pendingIntent);NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.notify(1, builder.build());
}
}
- Use the
-
Display the Notification:
- Call the
showNotification()
method whenever you want to display the notification.
- Call the
Creating Rich Notifications
Rich notifications offer more flexibility and can include multimedia content like images and videos. Here’s how to create them:
-
Add Multimedia Content:
- Use the
NotificationCompat.BigPictureStyle
orNotificationCompat.BigTextStyle
classes to add images or text styles:
java
private void showRichNotification() {
Intent intent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.your_icon)
.setContentTitle("Your Title")
.setContentText("Your Message")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setContentIntent(pendingIntent);if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
Bitmap bitmap = BitmapFactory.decodeResource(getResources(), R.drawable.your_image);
NotificationCompat.BigPictureStyle bigPictureStyle = new NotificationCompat.BigPictureStyle()
.bigPicture(bitmap)
.setSummaryText("Summary Text");
builder.setStyle(bigPictureStyle);
}NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.notify(2, builder.build());
}
}
- Use the
-
Add Interactive Elements:
- Use the
addAction()
method to add buttons or other interactive elements:
java
private void showInteractiveNotification() {
Intent intent = new Intent(this, MainActivity.class);
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.your_icon)
.setContentTitle("Your Title")
.setContentText("Your Message")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setContentIntent(pendingIntent);if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.JELLY_BEAN) {
NotificationCompat.Action action = new NotificationCompat.Action(
R.drawable.your_icon,
"Action Title",
PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT));
builder.addAction(action);
}NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.notify(3, builder.build());
}
}
- Use the
Managing Notification Channels
Managing notification channels is crucial for providing a better user experience. Here’s how to manage them:
-
Create a Notification Channel Group:
- If you have multiple channels related to a specific category or theme, group them together:
java
private void createNotificationChannelGroup() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
String channelGroup = "your_channel_group";
NotificationChannelGroup channelGroup = new NotificationChannelGroup(channelGroup, "Your Channel Group Name");
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.createNotificationChannelGroup(channelGroup);
}
}
}
- If you have multiple channels related to a specific category or theme, group them together:
-
Assign Channels to Groups:
- Assign each channel to a specific group:
java
private void assignChannelsToGroups() {
if (Build.VERSION.SDK_INT >= Build.VERSION_CODES.O) {
String channelGroup = "your_channel_group";
NotificationChannel channel = new NotificationChannel(
"your_channel_id",
"Your Channel Name",
NotificationManager.IMPORTANCE_DEFAULT
);
channel.setDescription("Your Channel Description");
channel.setGroup(channelGroup);
NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.createNotificationChannel(channel);
}
}
}
- Assign each channel to a specific group:
-
Provide Channel Management Options:
- Allow users to manage their channels by providing options in the app settings:
java
private void provideChannelManagementOptions() {
Intent intent = new Intent(Settings.ACTION_CHANNEL_NOTIFICATION_SETTINGS);
intent.putExtra(Settings.EXTRA_CHANNEL_ID, "your_channel_id");
intent.putExtra(Settings.EXTRA_APP_PACKAGE, getPackageName());
PendingIntent pendingIntent = PendingIntent.getActivity(this, 0, intent, PendingIntent.FLAG_UPDATE_CURRENT);
NotificationCompat.Builder builder = new NotificationCompat.Builder(this, "your_channel_id")
.setSmallIcon(R.drawable.your_icon)
.setContentTitle("Your Title")
.setContentText("Your Message")
.setPriority(NotificationCompat.PRIORITY_DEFAULT)
.setContentIntent(pendingIntent);NotificationManager notificationManager =
(NotificationManager) getSystemService(Context.NOTIFICATION_SERVICE);
if (notificationManager != null) {
notificationManager.notify(4, builder.build());
}
}
- Allow users to manage their channels by providing options in the app settings:
Best Practices for Custom App Notifications
-
Be Respectful of User Time:
- Avoid sending too many notifications as this can be intrusive and annoying.
-
Provide Clear and Concise Information:
- Ensure that your notifications are clear and concise, providing essential information without overwhelming the user.
-
Use Visual Hierarchy:
- Use visual hierarchy elements like icons, colors, and text styles to make your notifications more engaging and easier to understand.
-
Test Thoroughly:
- Test your notifications on different devices and Android versions to ensure they work as expected.
-
Follow Platform Guidelines:
- Always follow the guidelines set by Google for creating custom app notifications on Android.
Additional Resources
For more detailed information on creating custom app notifications on Android, refer to the official Android documentation or explore additional resources like tutorials and blogs from reputable sources. Implementing these strategies and techniques can create an effective notification system that enhances the overall user experience of your app.