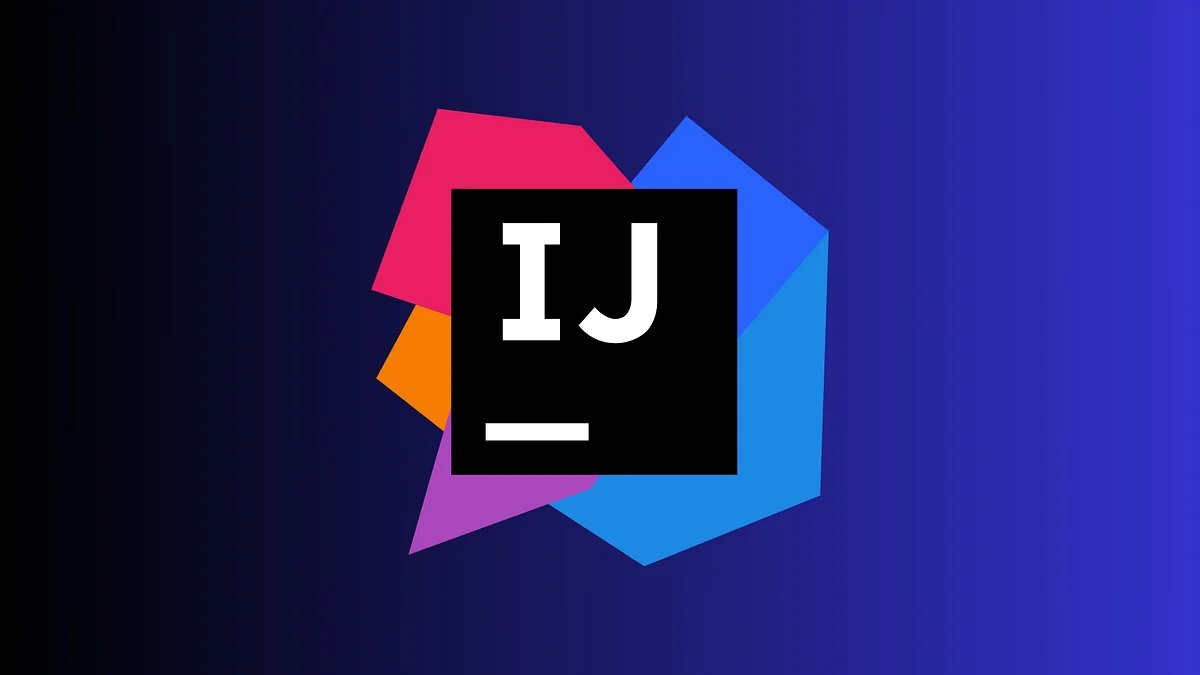
Introduction to IntelliJ IDEA for Android Development
Overview of IntelliJ IDEA:
IntelliJ IDEA is a powerful integrated development environment (IDE) created by JetBrains. It's designed to help developers write code more efficiently. With features like intelligent code completion, on-the-fly code analysis, and refactoring tools, it makes coding smoother. IntelliJ IDEA supports many programming languages, but it's especially popular for Java and Kotlin, which are essential for Android development.
Why Use IntelliJ IDEA for Android Development:
IntelliJ IDEA offers several benefits for Android developers. It provides a robust set of tools for coding, debugging, and testing Android apps. The IDE integrates seamlessly with the Android SDK and offers a user-friendly interface. Features like smart code completion, real-time error detection, and powerful debugging tools help streamline the development process. Additionally, IntelliJ IDEA's support for version control systems like Git makes collaboration easier.
Key Takeaways:
- IntelliJ IDEA is a powerful tool that makes coding Android apps easier with features like smart code completion and real-time error detection.
- Setting up IntelliJ IDEA for Android involves installing the JDK, Android SDK, and configuring the IDE, making it ready for creating and testing awesome apps.
Setting Up the Development Environment
Download and Install Java Development Kit (JDK):
To start, you'll need the Java Development Kit (JDK). Head over to the Oracle website and find the latest JDK version. Click the download link, accept the license agreement, and choose the appropriate installer for your operating system. Once downloaded, run the installer and follow the prompts. After installation, set the JAVA_HOME environment variable to point to the JDK installation directory.
Download and Install Android Software Development Kit (SDK):
Next, grab the Android Software Development Kit (SDK). Visit the Android developer website and download the SDK tools package. Extract the downloaded file to a convenient location. Open the extracted folder and run the SDK Manager. In the SDK Manager, select the necessary packages, including the latest Android platform and build tools, then click "Install."
Install IntelliJ IDEA:
Now, it's time to install IntelliJ IDEA. Go to the JetBrains website and download the Community Edition, which is free. Run the installer and follow the instructions. Once installed, launch IntelliJ IDEA. During the initial setup, you can customize the IDE to your liking, including choosing a theme and installing plugins.
Configure IntelliJ IDEA for Android Development:
With IntelliJ IDEA open, configure it for Android development. Go to "File" > "Project Structure" and set the JDK location. Then, navigate to "File" > "Settings" and find the "Plugins" section. Search for and install the "Android" plugin. After installation, restart IntelliJ IDEA. Finally, set up the Android SDK path by going to "File" > "Project Structure" > "SDKs" and adding the path to your Android SDK directory.
Creating Your First Android Project
Starting a New Project
To kick off your first Android project in IntelliJ IDEA, follow these steps:
- Open IntelliJ IDEA: Launch the application.
- Create New Project: Click on "Create New Project" from the welcome screen.
- Select Project Type: Choose "Android" from the list of project types.
- Configure Project: Enter your project name, save location, and select the appropriate JDK and Android SDK.
- Choose Template: Pick a project template, like "Empty Activity," to get started quickly.
- Finish Setup: Click "Finish" to create your project.
Configuring Project Settings
After creating your project, you'll need to configure some settings:
- Project Structure: Go to "File" > "Project Structure."
- SDK Configuration: Ensure the correct JDK and Android SDK versions are selected.
- Dependencies: Add any necessary libraries or dependencies under the "Modules" section.
- Build Tools: Configure build tools like Gradle to match your project requirements.
Understanding Project Structure
An Android project typically has the following structure:
- app/src/main/java: Contains Java or Kotlin source files.
- app/src/main/res: Holds resource files like layouts, images, and strings.
- app/src/main/AndroidManifest.xml: The manifest file that defines app components.
- build.gradle: Script files for managing project dependencies and build configurations.
Designing the User Interface
Using the UI Designer
IntelliJ IDEA offers a robust UI designer for creating Android interfaces:
- Open Layout File: Navigate to
res/layout
and open an XML layout file. - Design View: Switch to the "Design" tab to use the visual editor.
- Drag and Drop: Add UI components by dragging them from the palette to the design surface.
- Properties Panel: Adjust component properties like size, color, and text.
Adding UI Components
To add and configure UI components:
- Buttons: Drag a Button from the palette to the layout. Set its
id
andtext
properties. - Text Fields: Add a TextView or EditText for displaying or inputting text.
- Images: Use an ImageView to display images. Set the
src
attribute to the desired image resource.
Styling the UI
Enhance your app's look and feel with these styling tips:
- Themes: Define themes in
res/values/styles.xml
to apply consistent styles across the app. - Colors: Use
res/values/colors.xml
to define and manage color resources. - Dimensions: Store size values in
res/values/dimens.xml
for easy adjustments.
Making the Application Interactive
Handling User Input
Capturing user input is a fundamental part of making your app interactive. You can gather input through various UI components like text fields, buttons, and sliders. For instance, to get text from an EditText, you can use the getText()
method. Always validate user input to avoid errors and ensure data integrity. For example, checking if a text field is empty before processing its content can prevent crashes.
Implementing Event Listeners
Event listeners are crucial for responding to user actions. They allow your app to react when users click buttons, swipe screens, or perform other interactions. To add an event listener to a button, use the setOnClickListener()
method. Inside the listener, define what should happen when the button gets clicked. This could be anything from displaying a message to navigating to another screen.
Using Intents and Intent Filters
Intents are powerful tools for navigation and communication between different parts of your app. They can start new activities, send data, or even interact with other apps. To navigate to another activity, create an Intent object and call startActivity()
. Intent filters, on the other hand, define how your app responds to different types of intents. They are specified in the AndroidManifest.xml file and can make your app handle specific actions or data types.
Testing and Debugging
Configuring an Android Virtual Device (AVD)
An Android Virtual Device (AVD) emulates a physical Android device on your computer, allowing you to test your app without needing actual hardware. To set up an AVD, open the AVD Manager in IntelliJ IDEA, click on "Create Virtual Device," and follow the prompts to choose a device model and system image. Once configured, you can launch the AVD to test your app in a simulated environment.
Running the Application
Building and running your application is straightforward in IntelliJ IDEA. Click the "Run" button, and choose whether to run the app on an emulator or a connected physical device. Ensure your development environment is properly set up with the necessary SDKs and emulators. Running the app helps you see how it performs in real-time and identify any issues that need fixing.
Debugging Tips and Tricks
Debugging is an essential skill for any developer. IntelliJ IDEA offers various tools to help you find and fix bugs. Use breakpoints to pause execution and inspect variables. The Logcat tool provides real-time logging of system messages, which can help you track down issues. Additionally, familiarize yourself with the debugger's features, like step-over, step-into, and step-out, to navigate through your code efficiently.
Advanced Topics
Using Fragments and Adapters
Fragments allow you to create modular and reusable UI components. They are particularly useful for designing flexible and dynamic interfaces. Adapters, on the other hand, act as a bridge between your data source and UI components like RecyclerView. They help display data in a structured manner. Understanding how to use fragments and adapters can significantly enhance your app's design and functionality.
Working with Databases
Integrating databases into your app enables you to store and manage data efficiently. SQLite is a popular choice for Android apps. Use the SQLiteOpenHelper class to manage database creation and version management. You can perform CRUD (Create, Read, Update, Delete) operations using SQL queries. For more advanced database needs, consider using Room, a persistence library that provides an abstraction layer over SQLite.
Implementing Material Design
Material Design principles help create visually appealing and user-friendly interfaces. Use Material Design components like floating action buttons, snackbars, and cards to enhance your app's look and feel. Follow the guidelines for color schemes, typography, and spacing to ensure a consistent and intuitive user experience. Implementing these principles can make your app stand out and provide a better user experience.
Final Thoughts on Mastering IntelliJ IDEA for Android Development
Mastering IntelliJ IDEA for Android development is a rewarding journey that equips you with powerful tools for creating amazing apps. From setting up the development environment to designing sleek user interfaces and handling interactivity, IntelliJ IDEA simplifies each step. Smart features like code completion and real-time error detection make coding smoother, while robust debugging tools help squash bugs efficiently. As you dive deeper, you'll appreciate the versatility of fragments, adapters, and databases. Embrace Material Design principles to enhance your app’s aesthetic and usability. With dedication and practice, you'll unlock new possibilities and boost your coding skills, making your Android projects truly shine!
Feature Overview
IntelliJ Android simplifies app development by offering a robust IDE tailored for Android. It provides code completion, syntax highlighting, and refactoring tools to streamline coding. The feature includes a visual layout editor for designing interfaces, emulators for testing apps on virtual devices, and integrated debugging to catch and fix issues quickly. Additionally, it supports version control systems like Git, making collaboration easier.
Compatibility and Requirements
To ensure your device supports the feature, check these requirements:
- Operating System: Your device must run Android 8.0 (Oreo) or higher. Older versions won't support the latest features.
- RAM: At least 2GB of RAM is necessary for smooth performance. Devices with less may experience lag or crashes.
- Storage: Ensure you have at least 500MB of free storage. This space is needed for installation and updates.
- Processor: A Quad-core processor or better is recommended. Slower processors might struggle with intensive tasks.
- Screen Resolution: A minimum resolution of 720p (1280x720 pixels) ensures clear visuals. Lower resolutions might not display all features correctly.
- Bluetooth: If the feature involves connectivity, your device should support Bluetooth 4.0 or newer.
- Internet Connection: A stable Wi-Fi or 4G LTE connection is crucial for features requiring online access.
- Permissions: Grant necessary permissions like location, camera, and microphone access. Without these, some functionalities might be restricted.
- Google Play Services: Ensure Google Play Services are up-to-date. This is vital for compatibility and security.
Meeting these requirements guarantees your device can handle the feature without issues.
Getting Started with IntelliJ Android
Download IntelliJ IDEA: Go to the JetBrains website. Grab the Community Edition if you want free stuff.
Install IntelliJ IDEA: Open the downloaded file. Follow the on-screen instructions. Click "Next" a few times.
Launch IntelliJ IDEA: Find the IntelliJ IDEA icon. Double-click it. Wait for it to load.
Install Android SDK: Open IntelliJ. Go to "Configure" > "Plugins". Search for "Android". Install the Android plugin.
Create a New Project: Click "New Project". Select "Android". Click "Next".
Configure Project: Name your project. Choose a location. Select the SDK version. Click "Finish".
Set Up Emulator: Go to "Tools" > "AVD Manager". Click "Create Virtual Device". Pick a device. Click "Next".
Download System Image: Choose a system image. Click "Download". Wait for it to finish. Click "Next".
Finish Emulator Setup: Click "Finish". Your emulator is ready.
Run Your App: Click the green play button. Select your emulator. Watch your app come to life.
Boom! You're all set.
Effective Use of IntelliJ Android
Organize your project structure by using modules. This keeps things tidy and manageable.
Utilize the Code Completion feature to speed up coding. It suggests relevant code snippets as you type.
Refactor your code often. Use the Refactor menu to rename variables, methods, or classes without breaking your code.
Debugging is crucial. Set breakpoints and use the Debugger to step through your code and find issues.
Version Control is your friend. Integrate Git to keep track of changes and collaborate with others.
Use Shortcuts to save time. Learn key keyboard shortcuts for common tasks like Ctrl+Shift+F10 to run your app.
Customize your IDE. Adjust the theme, font size, and layout to suit your preferences.
Test your code. Write unit tests and use the JUnit framework to ensure your code works as expected.
Monitor Performance. Use the Profiler to check your app’s performance and optimize where needed.
Stay Updated. Regularly update IntelliJ and your SDK to access new features and improvements.
Troubleshooting Common Problems
App crashes often? Clear the cache. Go to Settings, then Apps, select the app, and tap Clear Cache.
Battery drains quickly? Lower screen brightness. Disable background apps. Turn off Wi-Fi and Bluetooth when not needed.
Phone overheating? Remove the case. Close unused apps. Avoid using the phone while charging.
Slow performance? Restart the device. Delete unused apps. Free up storage space by removing old files and photos.
Wi-Fi not connecting? Restart the router. Forget the network on your phone, then reconnect. Ensure the password is correct.
Bluetooth issues? Turn Bluetooth off and on. Unpair and re-pair the device. Check for software updates.
Screen unresponsive? Restart the phone. Remove any screen protector. Ensure the screen is clean and dry.
Apps not downloading? Check internet connection. Clear Google Play Store cache. Ensure enough storage space.
Camera not working? Restart the phone. Clear the camera app cache. Check for software updates.
No sound? Check volume settings. Ensure Do Not Disturb is off. Restart the phone.
Privacy and Security Tips
When using IntelliJ Android, security and privacy are key. User data gets handled with care, ensuring encryption during transmission. To maintain privacy, avoid using public Wi-Fi for sensitive tasks. Always update your software to patch vulnerabilities. Use strong passwords and enable two-factor authentication. Regularly review app permissions and disable those unnecessary. Be cautious about third-party plugins; only install from trusted sources. Backup your data frequently to prevent loss.
Comparing Alternatives
Pros of IntelliJ Android:
- Integrated Development Environment (IDE): Offers a robust environment for Android development.
- Smart Code Completion: Helps speed up coding with intelligent suggestions.
- Built-in Tools: Includes tools for debugging, testing, and profiling.
- Version Control Integration: Supports Git, SVN, and other version control systems.
- Extensive Plugin Support: Allows customization and additional features through plugins.
Cons of IntelliJ Android:
- Resource Intensive: Requires significant system resources, which can slow down older machines.
- Steep Learning Curve: May be challenging for beginners to master.
- Cost: The full version requires a paid license, which might not be ideal for hobbyists or small teams.
Alternatives:
Android Studio:
- Pros: Official IDE for Android development, free to use, strong community support.
- Cons: Similar resource demands, can be slow on older hardware.
Eclipse with ADT Plugin:
- Pros: Lightweight compared to IntelliJ, free, flexible.
- Cons: Less modern interface, fewer built-in tools, requires manual setup.
Visual Studio with Xamarin:
- Pros: Cross-platform development, integrates well with other Microsoft tools, strong debugging features.
- Cons: Can be complex to set up, requires a paid license for some features.
Xcode (for iOS development):
- Pros: Best for iOS development, free, strong integration with Apple’s ecosystem.
- Cons: Only available on macOS, not suitable for Android development.
NetBeans:
- Pros: Free, supports multiple languages, good for Java development.
- Cons: Less popular for Android development, fewer plugins compared to IntelliJ.
Conclusion: Choosing the right tool depends on your specific needs, system capabilities, and budget. Each option has its strengths and weaknesses, so consider what features are most important for your projects.
App crashes often? Clear the cache. Go to Settings, then Apps, select the app, and tap Clear Cache.
Battery drains quickly? Lower screen brightness. Disable background apps. Turn off Wi-Fi and Bluetooth when not needed.
Phone overheating? Remove the case. Close unused apps. Avoid using the phone while charging.
Slow performance? Restart the device. Delete unused apps. Free up storage space by removing old files and photos.
Wi-Fi not connecting? Restart the router. Forget the network on your phone, then reconnect. Ensure the password is correct.
Bluetooth issues? Turn Bluetooth off and on. Unpair and re-pair the device. Check for software updates.
Screen unresponsive? Restart the phone. Remove any screen protector. Ensure the screen is clean and dry.
Apps not downloading? Check internet connection. Clear Google Play Store cache. Ensure enough storage space.
Camera not working? Restart the phone. Clear the camera app cache. Check for software updates.
No sound? Check volume settings. Ensure Do Not Disturb is off. Restart the phone.
Mastering IntelliJ for Android Development
IntelliJ offers a powerful environment for Android development. Its features like code completion, refactoring tools, and integrated version control streamline the coding process. Plugins enhance functionality, making it adaptable to various needs. Debugging and testing tools ensure robust app performance.
Learning IntelliJ might seem challenging initially, but the efficiency it brings is worth the effort. Shortcuts and customizable settings save time, letting developers focus on creating quality apps.
Staying updated with IntelliJ's latest versions ensures access to new features and improvements. Community support and documentation provide valuable resources for troubleshooting and learning.
Incorporating IntelliJ into your workflow can significantly boost productivity and code quality. Embrace its capabilities to take your Android development skills to the next level.
Is IntelliJ good for Android development?
Yes, IntelliJ IDEA is great for Android development. It offers powerful code analysis, refactoring tools, and integrates well with Android SDKs.
Can you develop an Android app using IntelliJ?
Absolutely! You can create a new Android project by launching IntelliJ IDEA, selecting "New Project" from the Welcome screen, and choosing "Android" on the left in the New Project wizard.
How do you install the Android plugin in IntelliJ?
To install the Android plugin, press Ctrl+Alt+S to open settings, select Plugins, go to the Marketplace tab, find the Android plugin, and click Install. Restart the IDE if prompted.
What are the benefits of using IntelliJ over Android Studio?
IntelliJ IDEA offers advanced code analysis, better refactoring tools, and a more customizable interface. However, Android Studio provides specialized features tailored for Android development.
Can IntelliJ handle large Android projects efficiently?
Yes, IntelliJ is designed to handle large projects with ease. Its powerful indexing and search capabilities make navigating and managing big codebases straightforward.
Is there a difference between IntelliJ IDEA Community and Ultimate for Android development?
The Community edition is free and supports basic Android development. The Ultimate edition offers additional features like advanced debugging, profiling tools, and better support for enterprise frameworks.
How do you set up an Android emulator in IntelliJ?
You can set up an Android emulator by going to the AVD Manager in the Android SDK section of IntelliJ's settings. Create a new virtual device, configure its settings, and launch it to test your app.