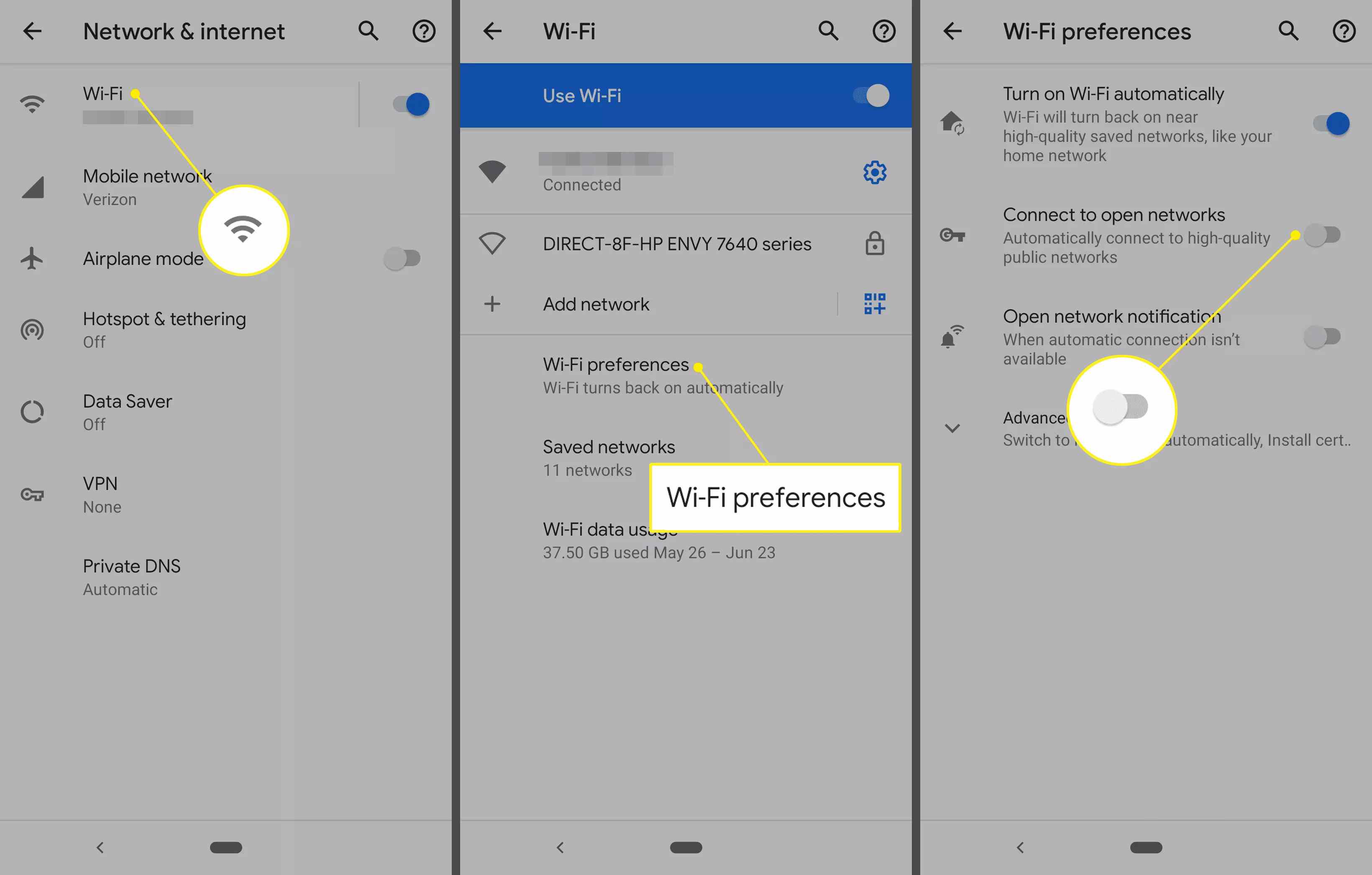
What is WiFi Direct?
WiFi Direct, also known as peer-to-peer (P2P) Wi-Fi, allows devices to connect directly without needing a central access point or router. This feature proves useful in scenarios where an internet connection is unavailable or when transferring large files quickly between devices.
How Does WiFi Direct Work on Android?
Steps to Use WiFi Direct
- Enable WiFi Direct: Ensure WiFi is enabled on your device. The WiFi Direct option is usually found in WiFi settings or under more connections settings.
- Discover Nearby Devices: Once WiFi Direct is enabled, your device will start broadcasting its presence. Other devices with WiFi Direct capabilities can detect and connect to it.
- Initiate a Connection: Create a group and invite other devices to join. This can be done programmatically using the WiFi P2P APIs provided by Android.
- Configure the Connection: After creating the group, configure the connection settings such as WPS (Wi-Fi Protected Setup) mode and encryption type. The device that initiates the connection becomes the group owner and manages the network.
- Transfer Data: Once connected, transfer files or data between devices using standard Java sockets. This process does not require an internet connection, making it ideal for offline scenarios.
Permissions Required
To use WiFi Direct in an Android application, declare specific permissions in your app's manifest file. These include:
ACCESS_FINE_LOCATION
CHANGE_WIFI_STATE
ACCESS_WIFI_STATE
INTERNET
For apps targeting Android 13 (API level 33) or higher, declare the NEARBY_WIFI_DEVICES
permission.
Practical Implementation
Step 1: Add Permissions
In your app's AndroidManifest.xml
, add the necessary permissions:
xml
Step 2: Initialize WiFi P2P Manager
In your activity, initialize the WiFi P2P manager:
java
import android.net.wifi.p2p.WifiP2pManager;
import android.net.wifi.p2p.WifiP2pManager.Channel;
import android.net.wifi.p2p.WifiP2pManager.ConnectionInfoListener;
public class WiFiDirectActivity extends AppCompatActivity {
private WifiP2pManager manager;
private Channel channel;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
manager = (WifiP2pManager) getSystemService(Context.WIFI_P2P_SERVICE);
channel = manager.initialize(this, getMainLooper(), null);
}
}
Step 3: Discover Nearby Devices
Discover nearby devices using the discoverPeers
method:
java
manager.discoverPeers(channel, new WifiP2pManager.ActionListener() {
@Override
public void onSuccess() {
Log.d("WiFiDirect", "Discovery initiated");
}
@Override
public void onFailure(int reason) {
Log.d("WiFiDirect", "Discovery failed");
}
});
Step 4: Connect to a Device
Once you have discovered nearby devices, connect to one of them using the connect
method:
java
@Override
public void connect() {
WifiP2pDevice device = peers.get(0); // Assuming peers is a list of discovered devices
WifiP2pConfig config = new WifiP2pConfig();
config.deviceAddress = device.deviceAddress;
config.wps.setup = WpsInfo.PBC; // Push Button Configuration (PBC)
manager.connect(channel, config, new ActionListener() {
@Override
public void onSuccess() {
Log.d("WiFiDirect", "Connected to device");
}
@Override
public void onFailure(int reason) {
Log.d("WiFiDirect", "Connection failed");
}
});
}
Limitations and Workarounds
While WiFi Direct provides a robust solution for peer-to-peer connections on Android, some limitations and workarounds should be considered:
- iOS Compatibility: iOS devices do not support WiFi Direct. Use Bluetooth Low Energy (BLE) for communication between Android and iOS devices, although this might not be as efficient for high-bandwidth applications.
- Security: WiFi Direct supports WPA2 encryption, which is more secure than WEP encryption used in traditional ad-hoc networks. Ensure the connection is properly secured to prevent unauthorized access.
- Discovery: Discovering nearby devices is straightforward using the WiFi P2P APIs, but it may take some time for devices to appear in the list. Mitigate this by using other discovery methods like Network Service Discovery (NSD).
- Performance: The performance of WiFi Direct can vary depending on the environment and device capabilities. In some cases, issues with connection stability or speed might occur. Testing your application under different conditions can help identify these issues.
Alternative Solutions
If WiFi Direct is not suitable for your application due to its limitations or the need for cross-platform compatibility, consider alternative solutions:
- Google Nearby Connections API: This API provides a more reliable and easier-to-use solution for establishing peer-to-peer connections between devices. It supports both Android and iOS platforms and can handle various use cases including file transfer and video streaming.
- BLE (Bluetooth Low Energy): While BLE has lower bandwidth compared to WiFi Direct, it is widely supported across both Android and iOS devices. It can be used for applications requiring low-bandwidth communication such as proximity-based services or simple data exchange.
- Local WiFi Access Point: Another approach is to start a local WiFi access point on the Android device and run a server. The iOS device can then connect to this access point using its WiFi settings. This method requires more setup but provides a flexible solution for various use cases.
Android WiFi Direct offers a powerful tool for establishing peer-to-peer connections between devices without needing an internet connection. While it has its limitations, particularly in terms of iOS compatibility, it remains a robust solution for many applications. By understanding the capabilities and limitations of WiFi Direct and exploring alternative solutions when necessary, developers can create efficient and reliable connectivity solutions for their Android applications.