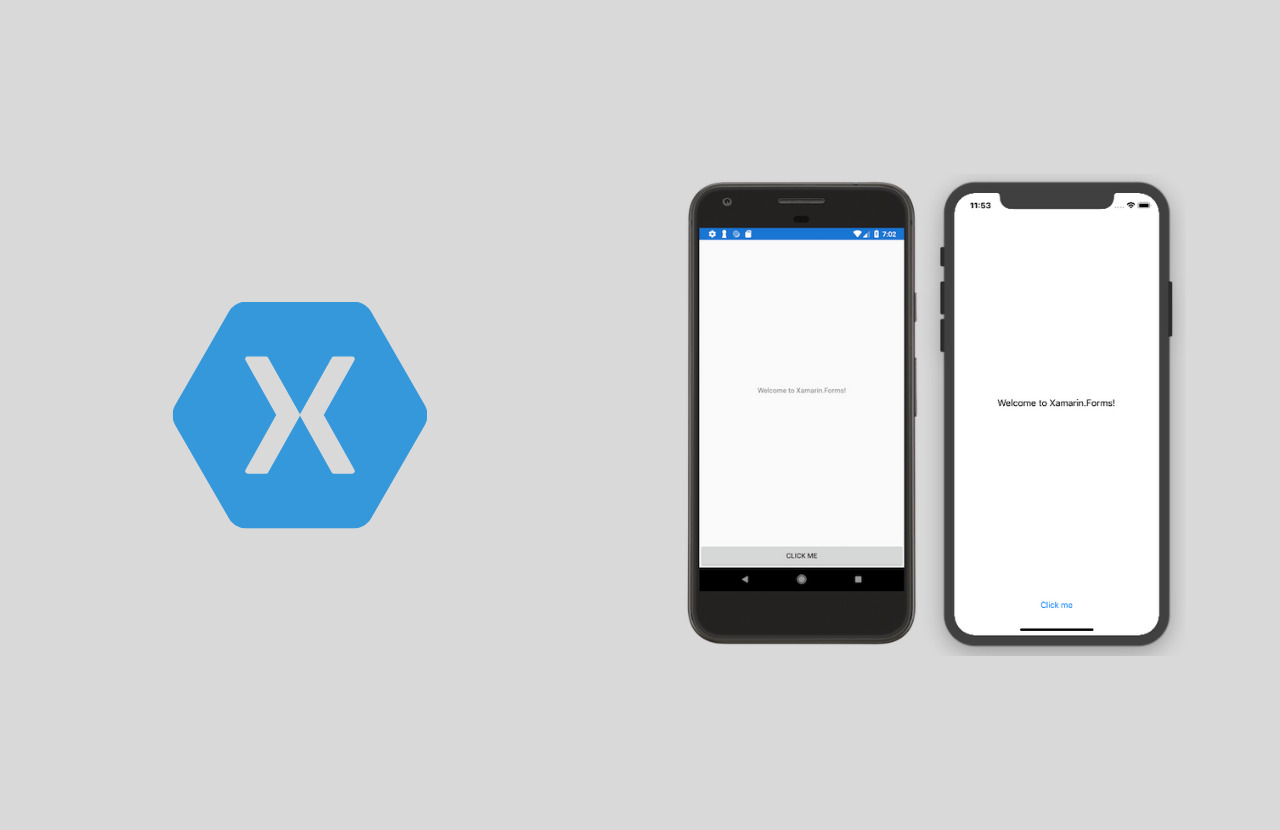
Introduction
Xamarin is a powerful framework that allows developers to create cross-platform applications using C# and the .NET framework. Understanding the concept of Android resources is crucial for developing Android applications with Xamarin. This article explores Android resources, their usage, and best practices for managing them in a Xamarin Android project.
What Are Android Resources?
Android resources are non-source code files compiled with the source code during the build process and packaged as an APK for distribution and installation on devices. These resources include images, layouts, strings, styles, and other data essential for building a user interface and providing a rich user experience.
Default Project Files and Folders
When a new Android project is created in Xamarin, several default project files and folders are added:
- AndroidManifest.xml: Contains information about your Android application, such as the application name, permissions, and other metadata.
- Resources Folder: Contains various types of resources like images, layouts, strings, and styles.
- Resources/Drawable Folder: Stores all the images used in your application.
- Resources/Layout Folder: Contains all the Android XML files (.axml) used to build user interfaces.
- Resources/Values Folder: Contains XML files to declare key-value pairs for strings and other types throughout an application.
- Resources.Designer.cs: Created automatically when the Android project is created, containing unique IDs for each resource. These IDs are used to access resources programmatically.
Loading Resources Programmatically
To load resources programmatically in a Xamarin Android project, use the unique IDs generated by the Resources.Designer.cs
file. Here’s an example of loading an image programmatically:
csharp
using Android.Content;
using Android.Graphics.Drawables;
namespace HelloGridView
{
public class MainActivity : Activity
{
protected override void OnCreate(Bundle savedInstanceState)
{
base.OnCreate(savedInstanceState);
SetContentView(Resource.Layout.Main);
// Load an image programmatically
ImageView imageView = FindViewById<ImageView>(Resource.Id.imageView);
Drawable drawable = ContextCompat.GetDrawable(this, Resource.Drawable.icon);
imageView.SetImageDrawable(drawable);
}
}
}
In this example, Resource.Id.imageView
is the unique ID for the ImageView
in your layout file, and Resource.Drawable.icon
is the unique ID for the image resource.
Difference Between Assets and Resources
In Xamarin Android, there are two types of non-source code files: assets and resources. Both store data not part of the source code but serve different purposes.
- Assets: Stored in the
Assets
folder of your project. They are not compiled into the APK and are accessed at runtime usingAssets.Open
orAssets.OpenStream
. Typically used for files like text data, audio files, or other data that do not need to be compiled into the APK. - Resources: Stored in the
Resources
folder of your project. Compiled into the APK and accessed using their unique IDs. Resources include images, layouts, strings, styles, and other data essential for building a user interface.
Managing Resources for Different Screen Densities
Android devices come in various screen densities, making it important to manage resources accordingly to ensure your application looks good on all devices. Here’s how to manage resources for different screen densities:
Create Different Versions of Images
- Create different versions of your images in different resolutions (e.g.,
drawable-hdpi
,drawable-xhdpi
, etc.). - Use the
Resources
folder to store these images.
Use Density-Specific Folders
- Use density-specific folders like
drawable-hdpi
,drawable-xhdpi
, etc., to store images optimized for specific screen densities.
Use Vector Drawables
- Use vector drawables (SVGs) which can be scaled up or down without losing quality.
- Store vector drawables in the
drawable-vectordrawable
folder.
Use Configuration Qualifiers
- Use configuration qualifiers like
layout-large
,layout-xlarge
, etc., to store layouts optimized for specific screen sizes.
Improvements in .NET 8
With the release of .NET 8, significant improvements have been made in how Android resources are generated and accessed. The old system that generated a Resource.designer.cs
file has been deprecated, and a new system generates a single _Microsoft.Android.Resource.Designer
assembly. This assembly contains all the final resource classes for every assembly.
New Resource Generation System
- The new system uses
aapt2
to compile resources into a binary form. - Generates a mapping from resource names to IDs in a file called
R.txt
. - This mapping is then exposed in the
_Microsoft.Android.Resource.Designer
assembly.
Accessing Resources Programmatically
- To access resources programmatically, use the IDs generated by this system.
- For example, instead of hardcoding IDs like
2131492864
, useResource.Layout.Main
.
Performance Optimization
Performance optimization is crucial when developing Android applications, especially those that use heavy Java objects like Android.Graphics.Bitmap
. Here are some tips for optimizing performance:
Use Reflection Wisely
- Reflection can be expensive and should be used sparingly.
- Use attributes like
[Preserve]
to preserve reflection metadata.
Enable ProGuard
- ProGuard can reduce the size of your dex file by removing unused code.
- However, it may cause some issues that need to be worked through.
Explicitly Clean Up Bitmaps
- The Mono GC may not track the full size of Bitmap objects, leading to out-of-memory exceptions.
- Explicitly clean up Bitmaps to avoid these issues.
Use Android APIs Instead of Reflection
- The
ResourceManager
class in Xamarin.Forms uses reflection extensively. - Consider using Android APIs instead to grab resource integer IDs from their names.
Understanding Android resources is essential for developing robust and efficient Xamarin Android applications. By managing resources effectively, using density-specific folders, and optimizing performance, you can create applications that run smoothly on a wide range of Android devices. With the improvements in .NET 8, accessing and managing resources has become even more streamlined and efficient. Whether you're a beginner or an experienced developer, mastering Android resources will help you build better applications with Xamarin.