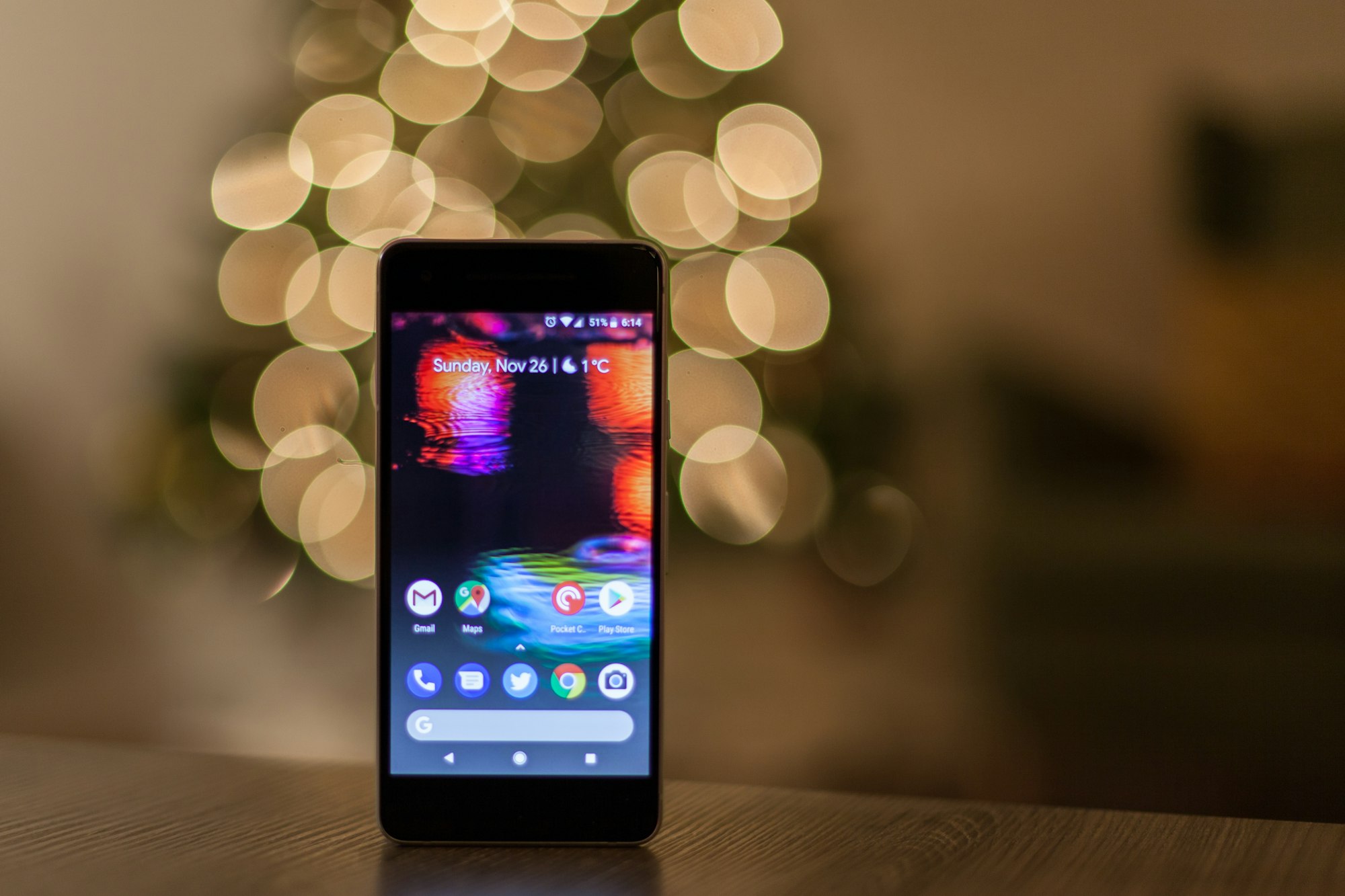
Introduction
Xamarin is a powerful framework enabling developers to create cross-platform applications using C# and the .NET framework. One key component is Xamarin.Android, which allows building fully native Android applications with C#. This guide will walk you through setting up Xamarin.Android in Visual Studio, creating your first Android project, and understanding the various components and tools involved.
Setting Up Xamarin.Android in Visual Studio
Install Visual Studio
- Download and install Visual Studio from the official Microsoft website. Choose between Community, Professional, or Enterprise versions. The Community version suffices for Xamarin development.
Install Xamarin Workload
- Open Visual Studio and go to the "Modify" option in the installer.
- In the "Workloads" section, select "Mobile development with .NET" to install necessary components.
Install Additional Components
- Under "Individual components," check "Android SDK" and "Google Android Emulator" to install essential tools.
- Optionally, install "Intel Hardware Accelerated Execution Manager" for better performance.
Install Xcode (for iOS Development)
- If planning to develop iOS applications, install Xcode on your macOS machine.
Creating Your First Android Project
Launch Visual Studio
- Open Visual Studio and go to the "Start" window.
Create a New Project
- In the "Start" window, click "Create a new project."
- In the "New Project" dialog box, select "Mobile App (Xamarin.Forms)" under the "Mobile" section.
Select Project Template
- Choose from templates like "Flyout," "Tabbed," and "Blank." Use the "Flyout" template for this guide.
Configure Project Details
- Configure project details as prompted. Leave default settings and click "Create."
Project Structure
- After creation, you will see three main projects in your solution:
- AwesomeApp: Main project for most application code.
- AwesomeApp.Android: Android-specific project for platform-specific code.
- AwesomeApp.iOS: iOS-specific project for platform-specific code.
Understanding Xamarin.Forms
Xamarin.Forms allows sharing code across multiple platforms, including Android and iOS. It uses XAML (Extensible Application Markup Language) to define user interfaces, which can be shared across different platforms.
XAML Hot Reload
XAML Hot Reload lets you see changes made to XAML code in real-time without rebuilding the application.
MVVM Architecture
Xamarin.Forms uses the Model-View-ViewModel (MVVM) architecture to separate the user interface from business logic, making maintenance and testing easier.
Shell in Xamarin.Forms
Xamarin.Forms Shell, introduced in version 5.0, provides a shell for applications, including features like URL-based navigation and a flyout menu.
Building the User Interface
Using XAML
-
Define the Layout:
- Open
MainPage.xaml
and define the layout using XAML. - Use a
StackLayout
to stack elements vertically or horizontally.
- Open
-
Add Controls:
- Add controls like
Label
,Button
, andEntry
to the layout. - Use more complex controls like
ListView
andCollectionView
.
- Add controls like
-
Style Your Controls:
- Customize control appearance using styles.
- Define global styles in
App.xaml
and apply them to controls.
Using C#
-
Define the Layout:
- Use the
ContentPage
class to define the layout programmatically.
- Use the
-
Add Controls:
- Add controls like
Label
,Button
, andEntry
to the page. - Use complex controls like
ListView
andCollectionView
.
- Add controls like
-
Style Your Controls:
- Customize control appearance using styles.
- Define global styles in
App.xaml.cs
and apply them to controls.
Handling Platform-Specific Code
Using DependencyService
-
Register Services:
- Use
DependencyService
to register services for different platforms. - Register a service providing a platform-specific implementation.
- Use
-
Resolve Services:
- Resolve services at runtime using
DependencyService
. - Resolve a service providing a platform-specific implementation.
- Resolve services at runtime using
Using Platform-Specific Code
-
Write Platform-Specific Code:
- Write platform-specific code in the
Android
oriOS
project. - Use Android-specific or iOS-specific APIs.
- Write platform-specific code in the
-
Call Platform-Specific Code:
- Call platform-specific code from shared code.
- Call methods using Android-specific or iOS-specific APIs.
Debugging Your Application
Using Visual Studio Debugger
-
Set Breakpoints:
- Set breakpoints where you want to pause execution.
- Set a breakpoint in
MainPage.xaml.cs
.
-
Run Your Application:
- Run the application in debug mode.
- Visual Studio will attach the debugger and pause execution at breakpoints.
-
Inspect Variables:
- Inspect variables and objects to understand the application's state.
- Inspect the value of a variable or object properties.
-
Use Debugging Tools:
- Use tools like the Locals window, Autos window, and Call Stack window to understand execution flow.
Using Android Debug Bridge (ADB)
-
Install ADB:
- Install ADB on your machine if not already installed.
- ADB is a command-line tool for interacting with Android devices or emulators.
-
Run Commands:
- Run ADB commands to interact with the device or emulator.
- Use
adb logcat
to view log messages.
-
Use ADB for Debugging:
- Use ADB for debugging purposes like capturing screenshots or viewing log messages.
- Use
adb shell
to capture a screenshot.
Deploying Your Application
Creating an APK File
-
Build Your Application:
- Build the application in release mode.
- This creates an APK file for distribution.
-
Sign Your APK File:
- Sign the APK file with a keystore.
- Required for publishing on the Google Play Store.
-
Upload to Google Play Store:
- Upload the signed APK file to the Google Play Store.
- Fill out necessary details like title, description, and screenshots.
-
Publish Your Application:
- After uploading all details, click “Publish” to make the application available to the public.
Xamarin.Android provides a robust platform for building high-quality mobile applications. By following these steps, you can set up Xamarin.Android in Visual Studio, create your first Android project, and understand the various components and tools involved. Whether a beginner or an experienced developer, Xamarin.Android offers a comprehensive solution for building fully native Android applications using C#.