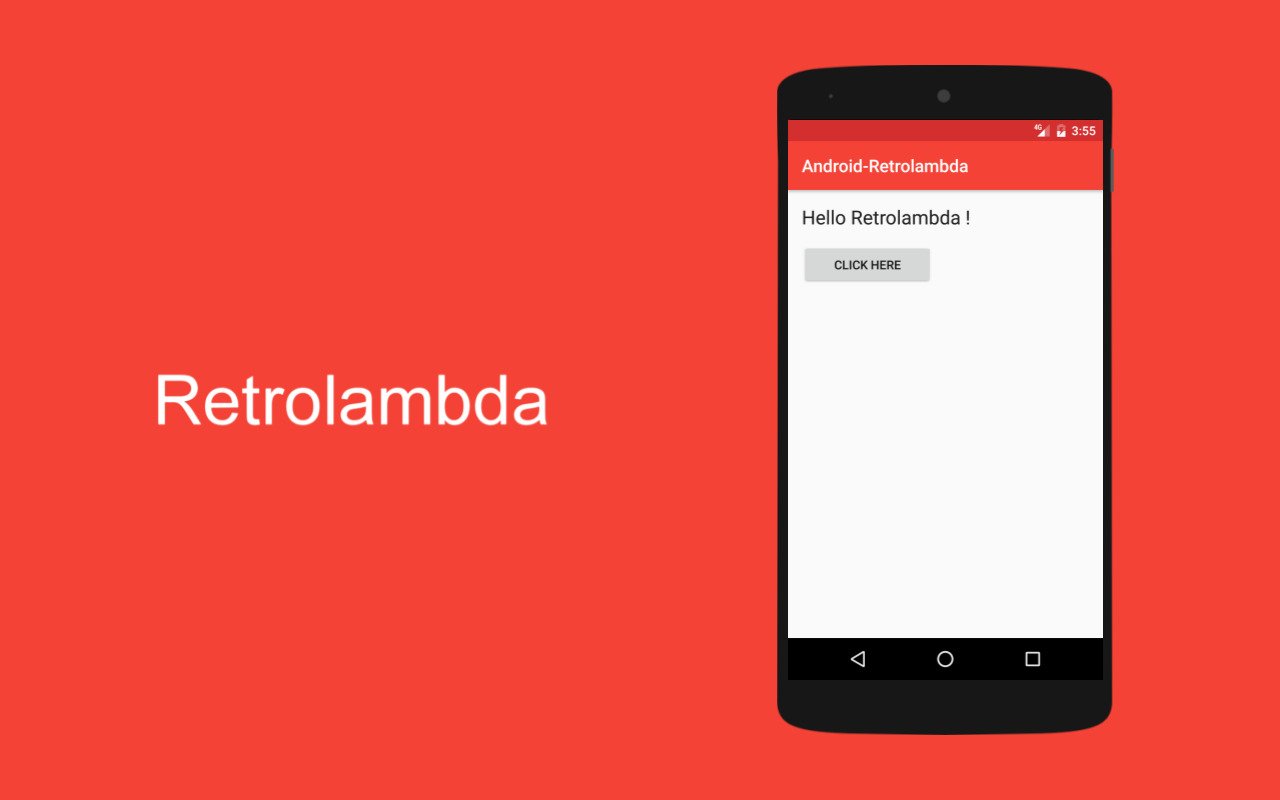
Introduction
In Android development, a significant challenge is the lack of support for Java 8 features in older Android SDK versions. This limitation hinders the use of modern programming constructs like lambda expressions, method references, and try-with-resources statements. To address this, the Retrolambda library was developed. This article provides a comprehensive guide for integrating Retrolambda with Android Studio, enabling developers to use Java 8 features in their Android projects.
What is Retrolambda?
Retrolambda allows developers to use Java 8 lambda expressions, method references, and try-with-resources statements in projects compiled with Java 6 or 7. It transforms the compiled bytecode to make it compatible with older Java runtime environments. This transformation is done using the Retrolambda plugin, integrated into a Gradle-based build process.
Setting Up Retrolambda
Step-by-Step Guide
Step 1: Download JDK 8
- Download JDK 8 from the official Oracle website.
- Set the path to the JDK in system environment variables to recognize JDK 8 as the default Java Development Kit.
Step 2: Add Dependencies to build.gradle
- Open the project's
build.gradle
file. - Add the necessary dependencies for the Retrolambda plugin by specifying the classpath and configuring the repositories.
groovy
buildscript {
repositories {
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:1.5.0'
classpath 'me.tatarka:gradle-retrolambda:3.7.1'
}
}
apply plugin: 'com.android.application'
apply plugin: 'me.tatarka.retrolambda'
android {
compileSdkVersion 22
buildToolsVersion "23.0.0 rc2"
defaultConfig {
applicationId "com.vogella.android.retrolambda"
minSdkVersion 22
targetSdkVersion 22
versionCode 1
versionName "1.0"
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
retrolambda {
javaVersion JavaVersion.VERSION_1_6
jvmArgs '-arg1', '-arg2'
defaultMethods false
incremental true
}
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android.txt'), 'proguard-rules.pro'
}
}
}
Step 3: Apply the Plugin
- Apply the Retrolambda plugin in the
build.gradle
file to enable bytecode transformation.
groovy
plugins {
id "me.tatarka.retrolambda" version "3.7.1"
}
Configuration Options
Retrolambda offers several configuration options to customize its behavior:
- Java Version: Set the Java version for compilation. For targeting Java 6 or 7, set it to
JavaVersion.VERSION_1_6
.
groovy
retrolambda {
javaVersion JavaVersion.VERSION_1_6
}
- JVM Arguments: Add JVM arguments for specific configurations or debugging purposes.
groovy
retrolambda {
jvmArgs '-arg1', '-arg2'
}
- Default Methods: Disable default methods if using Java 6 or 7 to avoid compatibility issues.
groovy
retrolambda {
defaultMethods false
}
- Incremental Compilation: Enable incremental compilation for better build performance.
groovy
retrolambda {
incremental true
}
Example Usage
After setting up Retrolambda, start using Java 8 features like lambda expressions and method references. Here’s an example of using lambda expressions in an Android activity:
java
package com.vogella.android.retrolambda;
import android.app.Activity;
import android.os.Bundle;
import android.widget.Button;
import android.widget.Toast;
public class MainActivity extends Activity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
Button view = (Button) findViewById(R.id.button);
view.setOnClickListener(e -> Toast.makeText(this, "Hello", Toast.LENGTH_LONG).show());
}
}
In this example, the setOnClickListener
method uses a lambda expression to show a toast message when the button is clicked. The Retrolambda plugin compiles this code and transforms it into bytecode compatible with older Java runtime versions.
Troubleshooting Common Issues
Lint Fails on Java Files with Lambdas
If lint fails on Java files containing lambdas, it might be due to compatibility issues with newer lint versions. Updating to the latest Android Gradle plugin version can resolve this. If updating is not possible, use an experimental fork to fix the issue.
Using Google Play Services Causes Retrolambda to Fail
Version 5.0.77 of Google Play Services contains bytecode incompatible with Retrolambda. This issue should be fixed in newer Play Services versions. If updating is not possible, use an earlier version like 4.4.52 or add -noverify
to the JVM arguments.
groovy
retrolambda {
jvmArgs '-noverify'
}
Proguard Configuration
Ensure Proguard does not warn about classes generated by Retrolambda by adding the following configuration to the Proguard file:
proguard
-dontwarn java.lang.invoke.*
-dontwarn **$Lambda$*
Android Studio Setup
Inform Android Studio about the language level by adding the following configuration to the build.gradle
file:
groovy
android {
compileOptions {
sourceCompatibility JavaVersion.VERSION_1_8
targetCompatibility JavaVersion.VERSION_1_8
}
}
Final Thoughts
Retrolambda is a powerful tool that allows developers to use modern Java features like lambda expressions and method references in older Android SDK versions. By following the steps outlined in this article, you can integrate Retrolambda into your Android project and start using these advanced programming constructs. Troubleshoot common issues and configure Proguard correctly to ensure smooth integration and optimal performance.
Using Retrolambda, write more efficient and readable code while maintaining compatibility with older Android SDK versions. This makes it an essential tool for any Android developer looking to stay current with the latest programming trends without compromising compatibility.