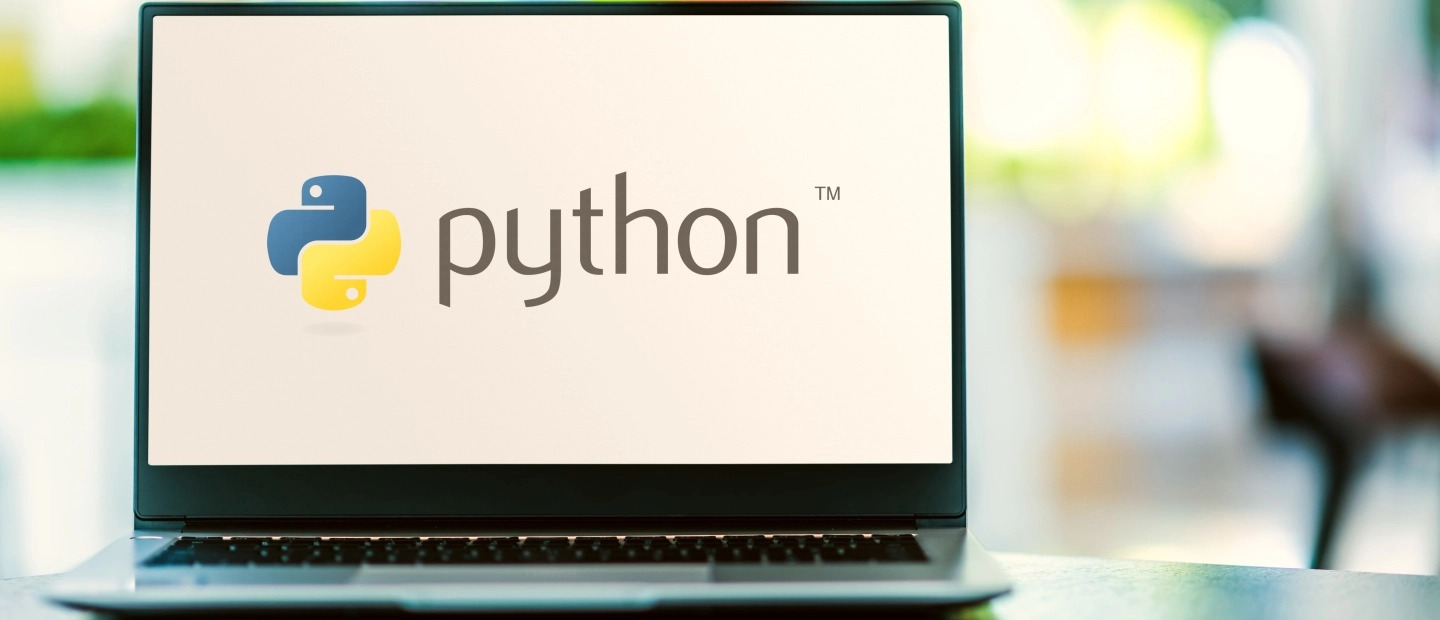
Why Choose Python for Android App Development?
Python's simplicity and readability make it an excellent choice for both inexperienced and seasoned developers. Here are some key reasons why Python stands out in Android app development:
Ease of Learning and Readability
Python's syntax is designed to be easy to understand, even for those with little programming experience. This simplicity allows beginners to quickly grasp the basics and start building applications, accelerating the development process.
Versatility
Python is widely used in various domains such as artificial intelligence, data research, and web development. Its versatility allows developers to effortlessly tap into these domains when building Android applications. For instance, Python's extensive libraries for machine learning can be leveraged to create intelligent apps that offer personalized experiences to users.
Rapid Prototyping
Python's dynamic typing and interpreted nature enable rapid prototyping. Developers can quickly iterate and test ideas, speeding up the development process. This rapid prototyping capability is particularly beneficial in the fast-paced world of mobile app development where time-to-market is crucial.
Large Community and Libraries
Python has a sizable, vibrant community that has produced a substantial collection of libraries and frameworks. These tools make various development tasks easier, including database integration and user interface design. For example, libraries like Kivy and BeeWare provide essential functionalities for cross-platform app development.
Cross-Platform App Development
Python makes it easier to create cross-platform apps. Developers can write code that runs on both Android and iOS by using frameworks like Kivy and BeeWare. This cross-platform capability saves time and effort, allowing developers to deploy their applications on multiple platforms with minimal modifications.
Tools and Frameworks for Android App Development with Python
Several tools and frameworks are available to help developers build Android apps using Python. Here are some of the most popular ones:
Kivy
Kivy is an open-source Python library for developing multi-touch applications. Initially released in 2011, it has become a staple in the Python Android app development ecosystem. Kivy supports cross-platform development, allowing applications to run on various operating systems including Android, iOS, Linux, OS X, and Windows. Its flexibility and extensive community support make it a go-to choice for many developers.
BeeWare
BeeWare is another powerful tool for developing Android apps using Python. It allows developers to write apps in Python and deploy them across multiple platforms without rewriting them in different programming languages. BeeWare's modular design enables easy updates and integration of modules as needed, making it an efficient choice for complex applications.
QPython
QPython is an ideal framework for running Python projects and scripts on Android devices. It includes a library, interpreter, editor, and SL4A (Scripting Layer for Android) libraries, making it a comprehensive solution for native app development. QPython's integration with the phone's hardware allows for more control over the device, although it can be buggy at times.
PySide
PySide is a Python binding of the cross-platform GUI toolkit Qt. It offers a high-level programming language combined with GUI capabilities, making it suitable for complex applications. The open-source nature of PySide allows developers to share ideas freely without needing permission, and its ability to deploy applications on different operating systems without rewriting the source code is a significant advantage.
Chaquopy
Chaquopy is a plugin for the Android Studio Gradle-based build system that allows developers to use both Python and Java interchangeably. It supports a wide range of features and is currently one of the best tools for developing Android applications using Python. Chaquopy's APIs enable developers to write parts or entire applications in Python, making it a versatile choice for various development needs.
Step-by-Step Guide to Building Android Apps with Python
Developing an Android app with Python involves several steps, each requiring careful planning and execution. Here’s a detailed guide on how to get started:
Plan the Pre-requisites
Before diving into the development process, define the prerequisites based on your requirements and ultimate app goal. Know the technologies within your tech stack that you need to implement and the infrastructure for app development. Ensure you have a compatible device for testing and a project folder to develop object-oriented Python programs.
Create the Simulation Environment
Set up a simulation environment for Python mobile development. This involves creating a project folder where you can write and test your code. Use tools like PyCharm or Visual Studio Code with Python extensions to create and manage your project.
Prototyping and Wireframing
Creating user movement and interaction with the app interface is crucial. Use tools like Kivy or PySide to devise a mobile app prototype with Python. This step helps realize how the software solution will flow, including different screens and navigation plans. Custom UI toolkits can help get started with the design for Python language.
Coding with Python
Start working on the backend code for the software development using Python’s web-based automation tool. Ensure a smooth flow of components between the graphical user interface and the server side of the application. If developing an image processing app, libraries like PyTesseract can be used for object detection.
Selecting the Framework
As discussed earlier, a GUI Python framework is needed to translate the code to native modules. For iOS development, Kivy might be used, while for Android development, BeeWare is often preferred. Install the necessary package for your chosen framework and run the code you have built on it.
Effective Libraries for Mobile App Development
Several Python libraries can help you get started with mobile app development:
- Kivy: For cross-platform development.
- BeeWare: For deploying apps across multiple platforms.
- PySide: For GUI development.
- Chaquopy: For integrating Python with Android Studio.
Challenges and Considerations
While Python offers numerous advantages, it also comes with some challenges:
Performance
Python is an interpreted language, which can impact how quickly apps load compared to natively built languages like Java. However, this can be mitigated with proper optimizations and framework selection.
Variability in the Ecosystem
Python's environment for developing Android apps is still evolving compared to Java and Kotlin, which have extensive libraries at their disposal. Some features may require developers to implement them independently or use native code.
Real-World Examples
Several well-known applications use Python for their development:
- Spotify: Uses Python to manage various features like Radio and Discover based on individual user music choices.
- Dropbox: Employs Python for its readability, strong support, and memorability, enabling rapid and continuous development cycles.
- Uber: Utilizes Python for its ability to execute complex code quickly, ensuring seamless communication between drivers and passengers.
Final Thoughts
Python is a strong tool for making Android apps and other mobile applications. Its quick development, adaptability, and smooth operation across different platforms make it an attractive choice for both developers and businesses. By leveraging tools like Kivy, BeeWare, QPython, PySide, and Chaquopy, developers can create robust and efficient applications that meet the demands of the modern mobile landscape.