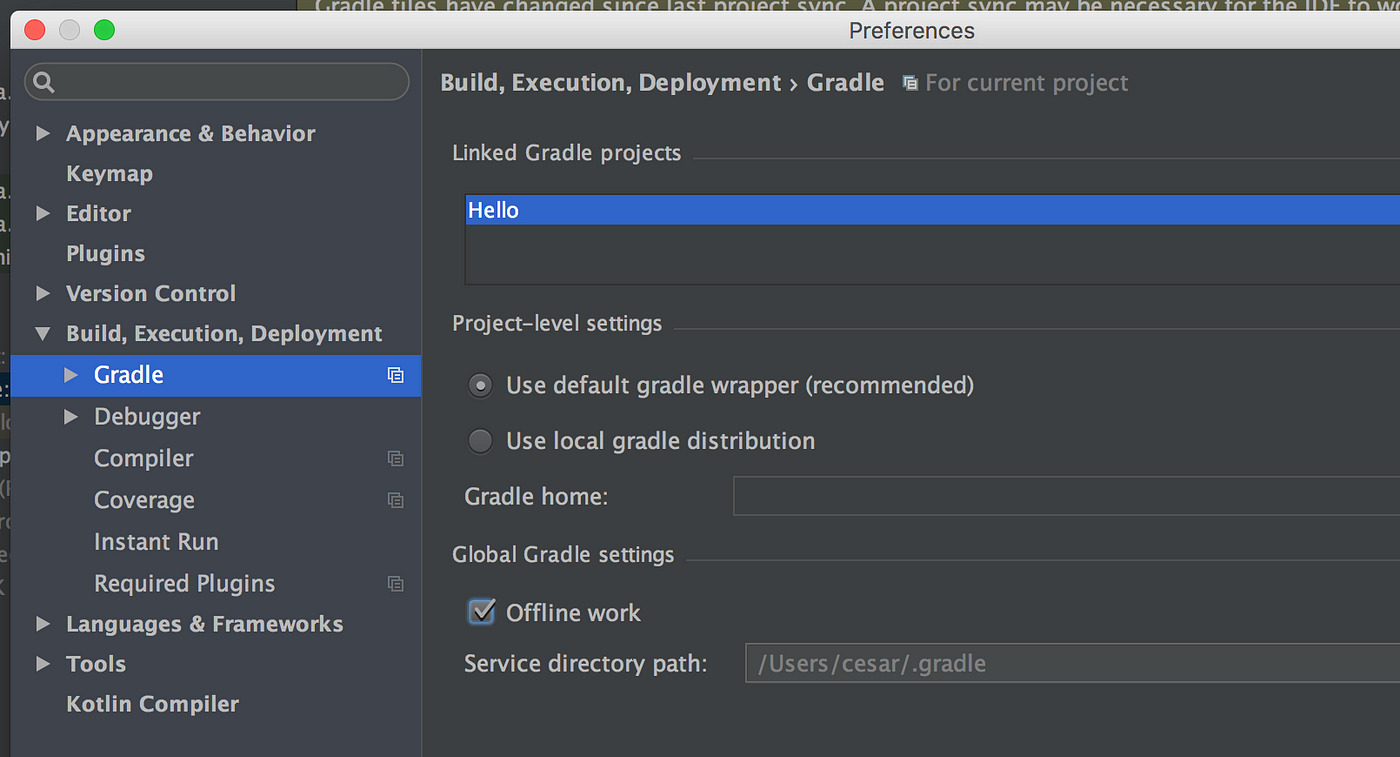
Introduction
Gradle is a powerful build tool used in Android development to manage dependencies, automate tasks, and streamline the development process. Mastering Gradle sync ensures that projects build correctly, dependencies resolve efficiently, and code compiles without errors. This article explores Gradle sync's importance, setup steps, and tips to optimize the build process.
What is Gradle?
Gradle, an open-source build automation tool, was first released in 2008. Created by Hans Dockter, it is now maintained by Gradle Inc. Designed to be flexible and extensible, Gradle allows developers to customize it according to their needs. Unlike other build tools like Maven, Gradle uses a Groovy-based DSL (Domain-Specific Language) for defining build scripts, making them more readable and maintainable.
Why Use Gradle?
- Dependency Management: Gradle excels in managing dependencies. Specify dependencies in the build script, and Gradle automatically downloads them, simplifying the management of third-party libraries and frameworks.
- Efficient Builds: Gradle performs incremental builds, rebuilding only the parts of the project that have changed. This significantly reduces build time.
- Customization: Gradle's Groovy-based DSL makes it highly customizable. Write custom tasks, plugins, and scripts to automate various tasks in the build process.
- Multi-Project Builds: Gradle supports multi-project builds, essential for large-scale applications. Manage multiple projects within a single build script.
- Integration with IDEs: Most Integrated Development Environments (IDEs) like Android Studio, IntelliJ IDEA, and Eclipse support Gradle out of the box. This integration makes it easier to manage the build process directly from the IDE.
Setting Up Gradle for Android
Step 1: Create a New Android Project
- Open Android Studio: Launch Android Studio and click on "Start a new Android Studio project."
- Choose Project Template: Select "Empty Activity" or any other template that suits your needs.
- Configure Project Details: Fill in the project details such as project name, package name, and location.
- Create Project: Click on "Finish" to create the project.
Step 2: Configure Gradle Build File
By default, Android Studio sets up a basic Gradle build file. However, customization might be necessary based on project requirements.
- Open Build File: Open the
build.gradle
file located in the project's root directory. - Review Build Script: Ensure the build script includes all necessary dependencies and configurations.
Step 3: Understand Build Script Components
A typical build.gradle
file for an Android project consists of several components:
Project-Level Build Script (build.gradle
)
Defines project-wide configurations such as repositories, plugins, and properties.
groovy
// Top-level build file where you can add configuration options common to all sub-projects/modules.
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.3.1'
classpath 'com.google.gms:google-services:4.3.10'
}
}
allprojects {
repositories {
google()
mavenCentral()
}
}
task clean(type: Delete) {
delete rootProject.buildDir
}
Module-Level Build Script (app/build.gradle
)
Defines configurations specific to the module (in this case, the app).
groovy
// Apply the java plugin to add support for Java and compile the Java code.
apply plugin: 'com.android.application'
android {
compileSdk 33
defaultConfig {
applicationId "com.example.myapp"
minSdk 21
targetSdk 33
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_11
targetCompatibility JavaVersion.VERSION_11
}
}
dependencies {
implementation 'androidx.core:core-ktx:1.9.0'
implementation 'androidx.appcompat:appcompat:1.4.1'
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
Optimizing Gradle Sync
Use Gradle Wrapper
The Gradle wrapper allows running Gradle without having it installed on the system. This is particularly useful for team members who might not have Gradle installed on their machines.
-
Generate Gradle Wrapper: Run the following command in the terminal:
sh
./gradlew wrapper –gradle-version 7.3.1 -
Update Build Script: Update the
build.gradle
file to include the Gradle wrapper:
groovy
buildscript {
repositories {
google()
mavenCentral()
}
dependencies {
classpath 'com.android.tools.build:gradle:7.3.1'
classpath 'com.google.gms:google-services:4.3.10'
}
}allprojects {
repositories {
google()
mavenCentral()
}
}task clean(type: Delete) {
delete rootProject.buildDir
}// Add this line to include the Gradle wrapper
task wrapper(type: Wrapper) {
gradleVersion = '7.3.1'
}
Use Multi-Project Builds
For large-scale applications, managing multiple projects within a single build script is essential. Multi-project builds come into play here.
- Create Sub-Projects: Create sub-projects within the main project directory.
- Configure Build Script: Configure the build script to include these sub-projects.
Example:
groovy
// Project-level build file
// Define sub-projects
subprojects {
project.ext {
// Shared properties for all sub-projects
buildDir = "${project.buildDir}/${name}"
}
// Apply common configurations to all sub-projects
apply plugin: 'com.android.application'
android {
// Common configurations for all sub-projects
compileSdk 33
defaultConfig {
minSdk 21
targetSdk 33
}
}
// Add dependencies common to all sub-projects
dependencies {
implementation 'androidx.core:core-ktx:1.9.0'
implementation 'androidx.appcompat:appcompat:1.4.1'
}
}
// Define specific configurations for each sub-project
// Module-level build file for main app module
apply plugin: 'com.android.application'
android {
// Specific configurations for main app module
compileSdk 33
defaultConfig {
applicationId "com.example.myapp"
minSdk 21
targetSdk 33
versionCode 1
versionName "1.0"
testInstrumentationRunner "androidx.test.runner.AndroidJUnitRunner"
}
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
compileOptions {
sourceCompatibility JavaVersion.VERSION_11
targetCompatibility JavaVersion.VERSION_11
}
}
dependencies {
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
// Module-level build file for library module
apply plugin: 'com.android.library'
android {
// Specific configurations for library module
compileSdk 33
defaultConfig {
minSdk 21
targetSdk 33
}
}
dependencies {
implementation 'androidx.core:core-ktx:1.9.0'
implementation 'androidx.appcompat:appcompat:1.4.1'
}
Use Dependency Management
Dependency management is one of Gradle's strongest features. Specify dependencies in the build script, and Gradle automatically downloads them.
- Add Dependencies: Add dependencies in the module-level
build.gradle
file.
Example:
groovy
dependencies {
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
testImplementation 'junit:junit:4.13.2'
androidTestImplementation 'androidx.test.ext:junit:1.1.3'
androidTestImplementation 'androidx.test.espresso:espresso-core:3.4.0'
}
- Use Dependency Versions: Specify exact versions of dependencies to avoid conflicts.
Example:
groovy
dependencies {
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
}
- Use Dependency Exclusions: Exclude specific modules from dependencies if they cause conflicts.
Example:
groovy
dependencies {
implementation('com.google.android.material:material:1.5.0') {
exclude group: 'com.android.support'
}
}
Use Build Variants
Build variants allow creating different versions of the app based on different configurations.
- Define Build Variants: Define build variants in the module-level
build.gradle
file.
Example:
groovy
android {
// Define build types (e.g., debug, release)
buildTypes {
debug {
debuggable true
jniDebuggable true
testCoverageEnabled true
}
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
// Define product flavors (e.g., free, paid)
flavorDimensions "versionCode", "versionName"
productFlavors {
free {
dimension "versionCode"
dimension "versionName"
}
paid {
dimension "versionCode"
dimension "versionName"
}
}
}
- Configure Build Variants: Configure build variants to create different versions of the app.
Example:
groovy
android {
// Configure build types and product flavors
buildTypes {
debug {
debuggable true
jniDebuggable true
testCoverageEnabled true
}
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
productFlavors {
free {
dimension "versionCode"
dimension "versionName"
}
paid {
dimension "versionCode"
dimension "versionName"
}
}
}
// Define different versions of the app based on build variants
// Example for free version
free {
applicationIdSuffix ".free"
versionNameSuffix "-free"
}
// Example for paid version
paid {
applicationIdSuffix ".paid"
versionNameSuffix "-paid"
}
Troubleshooting Common Issues
Gradle Build Fails Due to Missing Dependencies
If the Gradle build fails due to missing dependencies, ensure all necessary dependencies are specified in the build.gradle
file.
Example:
groovy
dependencies {
implementation 'com.google.android.material:material:1.5.0'
implementation 'androidx.constraintlayout:constraintlayout:2.1.3'
}
Gradle Build Takes Too Long
If the Gradle build takes too long, consider using incremental builds or caching dependencies.
Example:
groovy
// Enable incremental builds
tasks.withType(JavaCompile) {
options.incremental = true
}
// Cache dependencies
repositories {
mavenCentral()
}
// Enable caching for repositories
allprojects {
repositories {
mavenCentral()
}
}
Gradle Build Fails Due to Permission Issues
If the Gradle build fails due to permission issues, ensure necessary permissions are set up correctly in the AndroidManifest.xml
file.
Example:
xml
Final Thoughts
Mastering Gradle sync is vital for any Android developer looking to streamline their build process and ensure efficient dependency management. By understanding how to set up and optimize Gradle scripts, developers can significantly reduce build times and improve overall productivity. This article provides a comprehensive guide on setting up Gradle for Android projects, optimizing build processes, and troubleshooting common issues. With these tips and techniques, developers can create robust and efficient Android applications using Gradle as their build tool of choice.