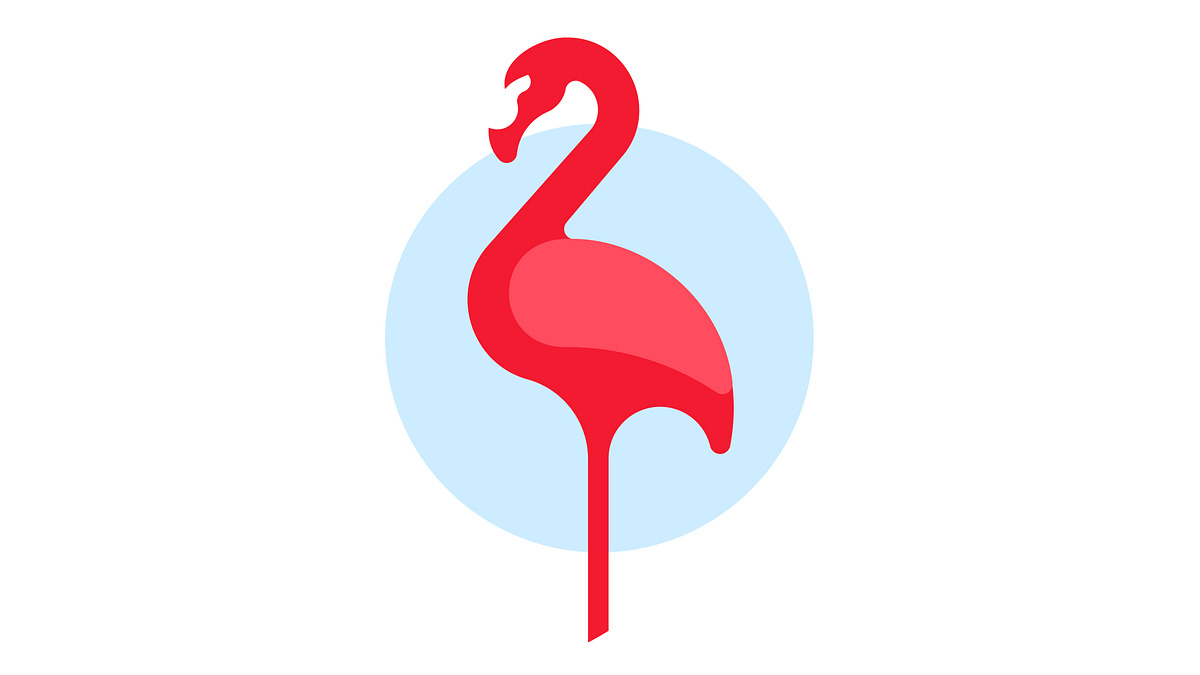
Introduction
Android Studio serves as the primary Integrated Development Environment (IDE) for Android app development. It offers a comprehensive set of tools and features to help developers create, test, and debug applications efficiently. However, the vast array of tools and features can be overwhelming for new developers. Flamingo is designed to help developers get the most out of Android Studio.
What is Flamingo?
Flamingo is an extensive guide and tutorial series created to help developers master Android Studio. Covering everything from setting up the environment to advanced topics like optimizing performance and debugging complex issues, Flamingo is user-friendly and accessible to both beginners and experienced developers.
Setting Up Android Studio
Downloading and Installing Android Studio
- Visit the official Android Studio website and download the latest version.
- Follow the installation instructions provided by Google. The installer will guide you through the process, ensuring all necessary components are installed correctly.
Setting Up Your Development Environment
- Launch Android Studio and follow the initial setup wizard.
- Choose your preferred language (Java or Kotlin) and select the type of project you want to create (e.g., Empty Activity, Basic Activity).
- Define the package name, project location, and other project settings to set up your project structure.
Configuring Your IDE
- Customize your IDE settings according to your preferences, including configuring keyboard shortcuts, font sizes, and other visual settings.
- Install any additional plugins or extensions needed for specific tasks.
Understanding the Interface
Project Window
- Displays the project structure in a tree-like format, showing all files and folders within your project.
- Navigate through different directories and files easily using this window.
Editor Window
- Write your code here. The editor supports syntax highlighting, code completion, and other features that simplify coding.
- Use different modes like Code Inspection or Code Analysis to identify potential issues in your code.
Tool Window
- Provides various tools and panels that help with different aspects of development such as debugging, testing, and version control.
- Common tools include the Layout Editor, Resource Manager, and Profiler.
Terminal Window
- Execute terminal commands directly within Android Studio.
- Useful for running Gradle tasks or executing shell commands related to your project.
Output Window
- Displays output from various tools like the compiler or debugger.
- Monitor the progress of your build process and identify any errors or warnings.
Basic Development Tasks
Creating a New Project
- Go to
File
>New
>New Project
. - Choose your project type (e.g., Empty Activity) and follow the wizard to set up your project structure.
Writing Code
- Start by writing your Java or Kotlin code in the editor window.
- Use syntax highlighting and code completion features to make coding easier.
- For example, when creating an activity, extend
AppCompatActivity
and override methods likeonCreate()
.
Designing Layouts
- Use the Layout Editor to design user interfaces for your activities.
- Drag and drop UI components like buttons, text views, and image views onto the layout canvas.
- Customize their properties using the Attributes panel.
Testing Your App
- Use the built-in emulator or connect a physical device to your computer.
- Run your app by clicking on the green play button in the toolbar or by using the keyboard shortcut
Shift+F10
.
Debugging Your App
- Use the debugger to step through your code line by line.
- Set breakpoints in your code by clicking on the line number margin.
- Use variables view to inspect variable values during execution.
Advanced Development Topics
Optimizing Performance
- Use profiling tools like the Profiler to identify performance bottlenecks in your app.
- Implement techniques such as lazy loading images and using caching mechanisms to improve performance.
Handling Complex Scenarios
- Manage asynchronous operations using coroutines or threads.
- Utilize data binding libraries like LiveData or Room persistence library for efficient data management.
Testing Strategies
- Implement different testing strategies such as unit testing, integration testing, and UI testing.
- Use testing frameworks like JUnit or Espresso for writing robust tests.
Security Best Practices
- Protect against common security threats like data breaches or unauthorized access.
- Encrypt sensitive data using Android KeyStore or implement secure authentication mechanisms.
Version Control Systems
- Use version control systems like Git for managing code changes and collaborating with team members.
- Set up Git repositories within Android Studio using the built-in VCS tools.
Additional Resources
For further learning, here are some additional resources that might be useful:
- Official Android Documentation: Detailed guides on various aspects of Android development, including setting up your environment, writing code, and testing your app.
- Android Developers Blog: Stay updated with the latest trends and best practices in Android development.
- Stack Overflow: A community-driven Q&A platform where you can ask questions related to Android development and get answers from experienced developers.
- Udacity Courses: Offers a range of courses on Android development that cover both basic and advanced topics.
By utilizing these resources along with Flamingo, you'll be well-equipped to tackle any challenge in the world of Android app development.