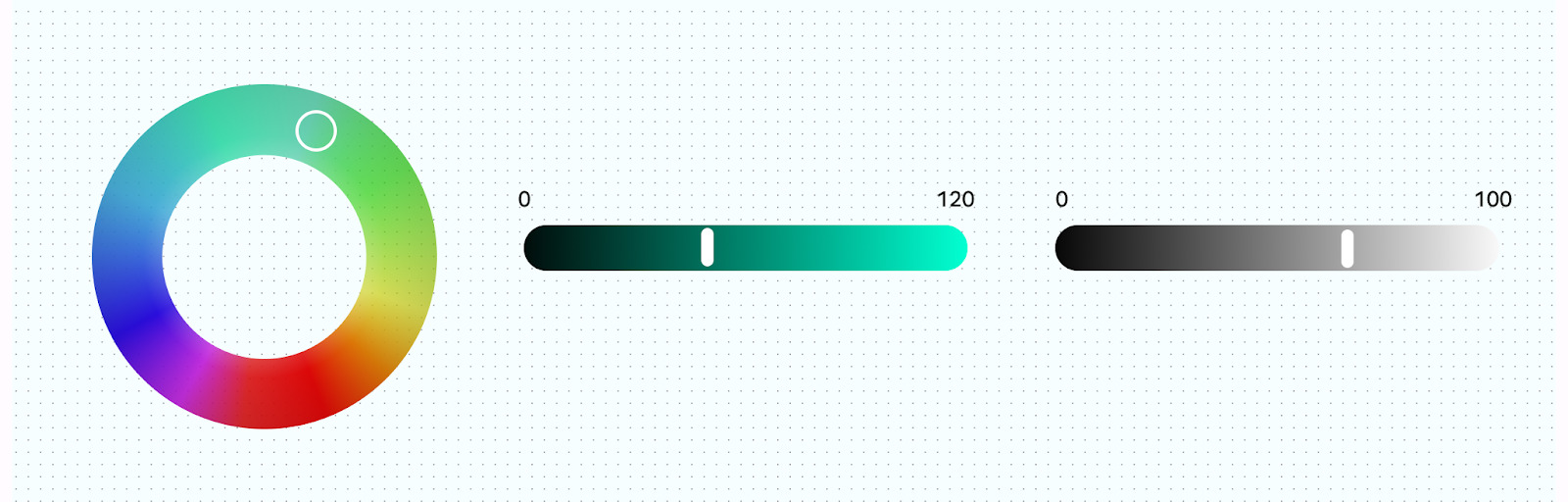
Introduction
Android Studio stands as one of the most popular Integrated Development Environments (IDEs) for Android app development. It offers a robust set of tools and features that simplify the creation, testing, and deployment of Android applications. Customizing visual elements, such as button colors, can enhance the user experience and make the development process more enjoyable.
This article will guide you through customizing button colors in Android Studio. We will cover the steps involved in modifying the color scheme, the benefits of customization, and additional tips for further personalization.
Understanding the Importance of Customization
Customization allows developers to tailor their workspace to specific needs and preferences, significantly improving productivity and overall satisfaction. In Android Studio, customizing button colors can enhance the interface's visual appeal and make it easier to distinguish between different components.
Prerequisites
Before customizing button colors in Android Studio, ensure you have the following:
- Android Studio Installed: Download it from the official Android Developers website if not already installed.
- Basic Knowledge of Android Studio: Familiarity with navigating and using Android Studio is necessary.
Steps to Customize Button Colors
Customizing button colors involves modifying the theme of your project. Follow these detailed steps:
Open Your Project
Open your Android project in Android Studio. If no existing project is available, create a new one by selecting "Start a new Android Studio project" from the start-up screen.
Locate the Styles.xml File
To customize button colors, modify the styles.xml file located in the res/values
directory of your project. Navigate to res > values > styles.xml
to find this file.
Edit the Styles.xml File
Open the styles.xml file in the text editor. This file contains all the styles and themes used in your project. You will see a list of predefined styles and themes.
Create a New Style
Add a new <style>
element inside the <resources>
tag to create a custom style for buttons. For example:
xml