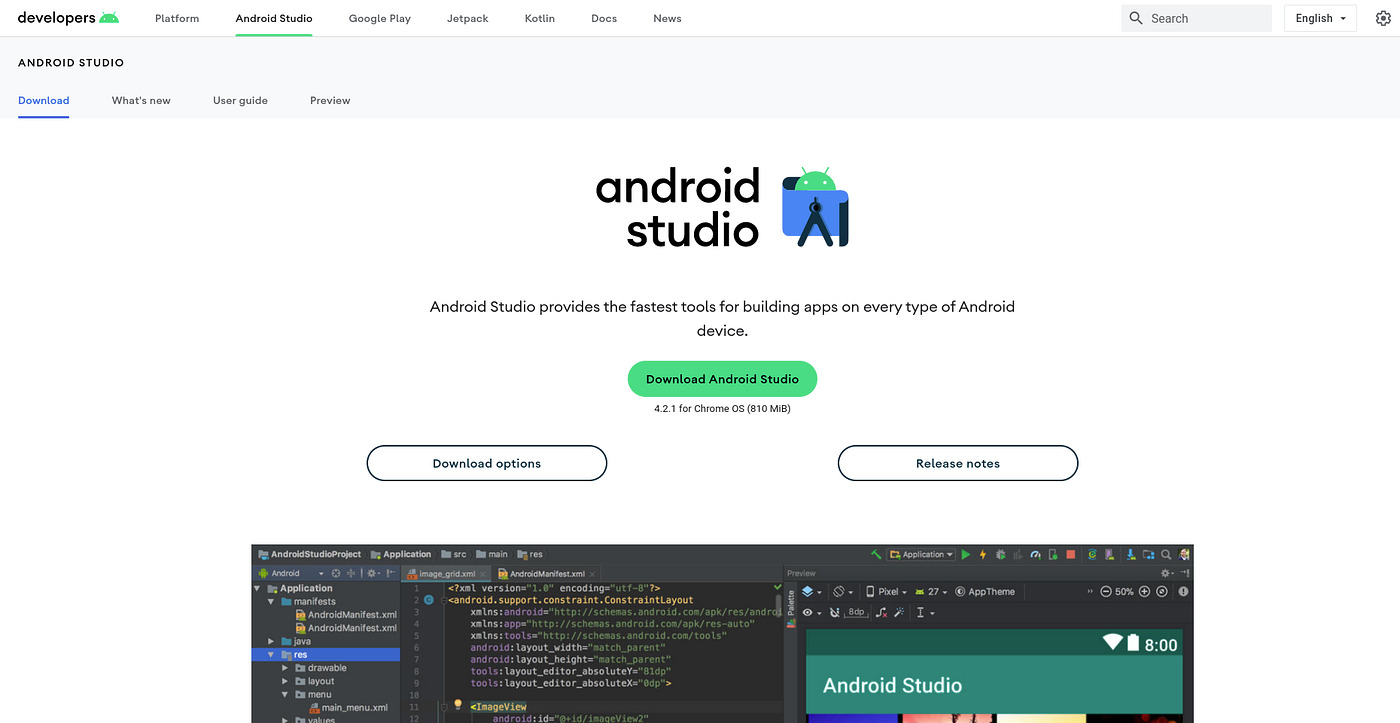
Introduction
In recent years, mobile app development has evolved significantly, with Android Studio on Chromebooks becoming a notable trend. This guide will walk you through installing and setting up Android Studio on your Chromebook, providing tools to deploy and test Android apps directly on your device. Whether you are a seasoned developer or just starting out, this comprehensive guide will help you navigate the steps required to get started with Android development on your Chromebook.
System Requirements
Before diving into the installation process, ensure your Chromebook meets the system requirements for running Android Studio. Here are the minimum specifications recommended by Google:
- RAM: 8 GB or more
- Disk Space: 20 GB of available disk space
- Screen Resolution: 1280 x 800 minimum
- Processor: Intel i5 or higher (U series or higher)
Recommended Chromebooks
For optimal performance and user experience, Google recommends specific Chromebooks:
- Acer: Chromebook 13/Spin 13, Chromebox CXI3, Chromebook 712 [C871]
- ASUS: Chromebox 3, Chromebook Flip C436FA
- CTL: Chromebox CBx1
- Dell: Inspiron Chromebook 14, Latitude 5300 2-in-1 Chromebook Enterprise, Latitude 5400 Chromebook Enterprise
- HP: Chromebook x360 14, Chromebox G2, Chromebook x360 14c
- Lenovo: Yoga C630 Chromebook, Flex 5 Chromebook
- ViewSonic: NMP660 Chromebox
Enabling Developer Mode (Optional)
Enabling Developer Mode on your Chromebook is crucial if you plan to debug apps directly on your device. Here’s how you can do it:
- Backup Important Files: Ensure all important files are backed up. Enabling Developer Mode will wipe your device every time it starts.
- Enter Recovery Mode: Turn off your Chromebook, then hold the Escape and Refresh keys while powering it back on. This will put your device into Recovery Mode.
- Confirm Developer Mode: In Recovery Mode, press Ctrl-D to enter Developer Mode. You will be prompted to confirm, and your device will be wiped every time it starts.
- Bypass Wipe Reminder: Press Ctrl-D to bypass the reminder about your device being in Developer Mode.
- Set Up Account Again: Because your device has been wiped, you will need to set up your account again.
Enabling ADB Debugging (Optional)
If you plan to debug Android apps directly on your Chromebook, enable ADB debugging. Here’s how:
- Open Settings App: Open the Settings app on your Chromebook.
- Manage Android Preferences: Click on “Google Play Store” and then “Manage Android Preferences” to open the Android Settings app.
- Enable Developer Options: In the Developer Options menu, enable ADB debugging.
Enabling Linux Apps
To run Android Studio on your Chromebook, enable Linux apps. Here’s how:
- Open Settings App: Open the Settings app on your Chromebook.
- Find Linux Apps: Navigate to the “Linux Apps” section and click “Turn On.”
- Download Required Files: Your Chromebook will download the required files to use Linux apps. When complete, a new Terminal app will open.
Downloading Android Studio
Once Linux apps are enabled, download Android Studio from the official Android Studio downloads page. Here’s how:
- Visit Downloads Page: Visit the Android Studio downloads page and download the Android Studio package for Linux.
- Copy Zip File: Once the download is complete, open the Files app and copy the zip file from your Chrome OS Downloads to Linux Files.
Installing Android Studio
After downloading the zip file, unzip it and install Android Studio. Here’s how:
-
Open Terminal App: Open the Terminal app that was downloaded with Linux.
-
Unzip Android Studio: Type in the following command to unzip Android Studio’s files:
bash
unzip android-studio-ide-173.4907809-linux.zip -
Start Android Studio: Run one more command to start Android Studio:
bash
./android-studio/bin/studio.sh -
Setup Wizard: You will now be greeted with the standard Android Studio setup wizard. Proceed with the setup as normal to download the required Android files.
Setting Up ADB Debugging
To deploy and debug apps on your Chromebook, set up ADB debugging. Here’s how:
- Enable ADB Debugging: Go to Settings and turn on Linux if you haven’t done so already.
- Open Linux Settings: Once Linux is available, open the Linux settings.
- Enable ADB Debugging: Open the “Develop Android apps” option and toggle “Enable ADB debugging.” The Chromebook will restart.
- Authorize ADB: After the Chromebook restarts, a message might appear letting you know that there might be applications that were not downloaded from the app store on the device.
- ADB Authorization: ADB is now available to deploy apps to your Chromebook, run debugging commands, and interact directly with the device.
Deploying Apps with Android Studio
After setting up ADB debugging, load an Android app directly onto your ChromeOS device using Android Studio. Here’s how:
- Open Android Studio: Launch Android Studio from your Linux environment.
- Connect to Device: In the device menu, select your Chromebook as an option to push your app.
- Authorize ADB: An ADB authorization dialog will appear. Grant the authorization to deploy your app.
- Launch App: Your application will launch in a new window, allowing you to test and debug it directly on your Chromebook.
Deploying Apps with Terminal
Alternatively, deploy your app using the terminal. Here’s how:
-
Install ADB: If necessary, install ADB using the following command:
bash
sudo apt install adb -
Connect to Device: Connect to the device using the following command:
bash
adb connect arc -
Authorize ADB: An authorization dialog for USB debugging will appear. Grant the authorization.
-
Install App: Install your app from the terminal using the following command:
bash
adb install [path to your APK]
Testing and Debugging
Testing and debugging your app on a Chromebook provides several benefits, including:
- User Experience: Better understand the user experience by testing on different form factors.
- Large Screens: Optimize your app’s experience for large screens and input support.
- Cross-Platform Testing: Deploy and test your app from your ChromeOS device to a phone, tablet, or another ChromeOS device.
Recommended Devices for Testing
To ensure your Android app works well on various Chromebook devices and available form factors, Google recommends testing on the following devices:
- ARM-based Chromebook
- x86-based Chromebook
- Device with Touchscreen and without Touchscreen
- Convertible Device
- Device with Stylus
Additional Tools for Android Development
In addition to Android Studio, several other tools can be useful for Android development on ChromeOS:
- Android Emulator: Supported Chromebooks can now run a full version of the Android Emulator, allowing developers to test apps on any Android version and device without needing the actual hardware.
- Lint Rules: An in-depth guide on how to improve the experience of large screens on ChromeOS with lint rules in Android Studio.
- ADB over Wi-Fi and Ethernet: Test Android apps on real devices with ADB over Wi-Fi and Ethernet.
By following these steps, you can deploy and test your Android apps directly on your Chromebook, providing a seamless development experience. Whether you are a beginner or an experienced developer, this guide has provided the necessary tools and information to get started with Android development on your Chromebook.