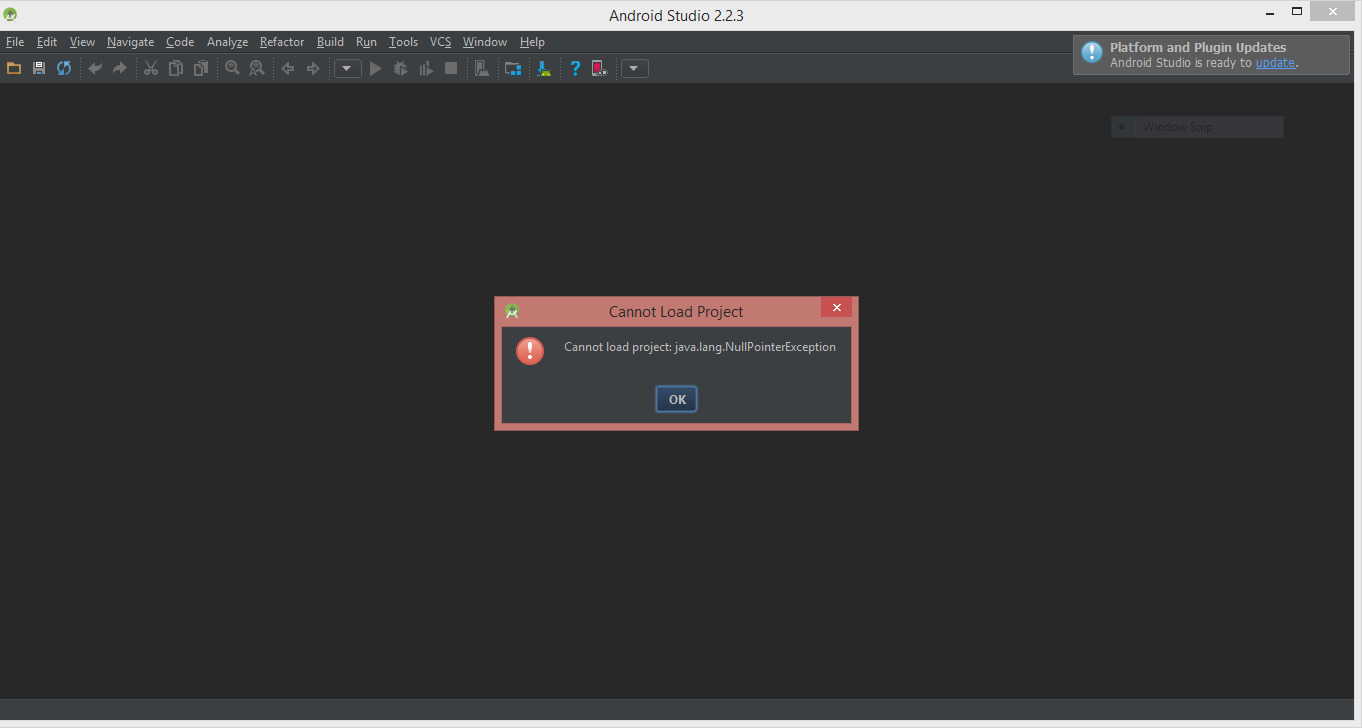
Introduction
NullPointerExceptions are common and frustrating errors in Android app development. These exceptions occur when an application attempts to use an object reference that hasn't been initialized, resulting in a runtime error. This article explores the causes, identification methods, and techniques to fix NullPointerExceptions using Android Studio.
Understanding NullPointerExceptions
What is a NullPointerException?
A NullPointerException (NPE) is a runtime exception occurring when an application tries to use an object reference with a null value. This happens because the object hasn't been properly initialized or was set to null at some point. In Java, null represents the absence of a value. Attempting to use a null value results in a NullPointerException because the operation cannot be completed.
Causes of NullPointerExceptions
NullPointerExceptions can occur in various situations, including:
- Uninitialized Variables: When a variable is declared but not initialized before use.
- Null References: When an object reference is set to null and then used.
- Missing Initialization: When an object isn't initialized before its methods are called.
- Incorrect Data Retrieval: When data retrieved from a database or network call results in null.
Identifying NullPointerExceptions
Identifying NullPointerExceptions can be challenging, especially in complex applications. However, several tools and techniques can help diagnose these issues:
- Logcat: A powerful tool in Android Studio providing detailed logs of your application's activities. When a NullPointerException occurs, logcat displays the stack trace, including the line number, class name, and other relevant information.
- Stack Trace: A crucial part of the logcat output showing the sequence of method calls leading to the exception, making it easier to pinpoint the exact location of the error.
- Debugging: Using breakpoints and the debugger in Android Studio helps step through your code and see exactly where the null reference is being accessed.
Fixing NullPointerExceptions
Fixing NullPointerExceptions involves ensuring all object references are properly initialized before use. Here are some steps to fix these errors:
1. Initialize Variables
Always initialize variables before using them. For example, if you have a TextView, ensure initialization before setting its text.
java
// Incorrect initialization
TextView textView = null;
textView.setText("Hello World");
// Correct initialization
TextView textView = findViewById(R.id.textView);
textView.setText("Hello World");
2. Use Try-Catch Blocks
Try-catch blocks are essential for handling potential null references and preventing abrupt app crashes. By catching the NullPointerException, you can handle it gracefully and provide a better user experience.
java
try {
// Code that might throw a NullPointerException
String str = null;
int length = str.length();
} catch (NullPointerException e) {
// Handle the exception
Toast.makeText(this, "String is null", Toast.LENGTH_SHORT).show();
}
3. Check for Null Before Use
Always check if an object reference is null before using it. This can be done using the != null
operator.
java
// Correct way to check for null
String str = null;
if (str != null) {
int length = str.length();
} else {
// Handle the case when str is null
}
4. Use Safe Navigation Operator (?.) in Kotlin
In Kotlin, use the safe navigation operator (?.
) to avoid NullPointerExceptions when dealing with nullable objects.
kotlin
// Safe navigation operator in Kotlin
val str: String? = null
val length = str?.length
5. Use Non-Null Assert Operator (!!) in Kotlin
If certain that an object reference should not be null, use the non-null assert operator (!!
) to assert that it is not null.
kotlin
// Non-null assert operator in Kotlin
val str: String? = null
val length = str!!.length // This will throw an exception if str is null
6. Logcat and Debugging
Using logcat and debugging tools in Android Studio can help identify where the null reference is being accessed. By setting breakpoints and stepping through your code, you can see exactly where the error occurs.
java
// Example of logging in logcat
try {
// Code that might throw a NullPointerException
} catch (NullPointerException e) {
Log.e("App", "NullPointerException occurred", e);
}
Advanced Techniques
Using Android Studio's Built-in Features
Android Studio provides several built-in features to help diagnose and fix NullPointerExceptions:
- Logcat Filtering: Filter logcat output to focus on specific exceptions or messages.
- Stack Trace Analysis: Android Studio's stack trace analysis tool helps understand the sequence of method calls leading up to the exception.
- Breakpoints: Setting breakpoints in your code allows stepping through and inspecting variables at runtime.
Example Code
Here is an example of handling a potential NullPointerException in a real-world scenario:
java
// Example of handling a potential NullPointerException
public class MainActivity extends AppCompatActivity {
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize TextView
TextView textView = findViewById(R.id.textView);
// Check if textView is not null before setting its text
if (textView != null) {
textView.setText("Hello World");
} else {
// Handle the case when textView is null
Toast.makeText(this, "TextView is null", Toast.LENGTH_SHORT).show();
}
}
}
Additional Tips
Cleaning and Rebuilding Your Project
Sometimes, cleaning and rebuilding your project can resolve issues related to NullPointerExceptions. This is especially true if you have recently added or modified dependencies in your project.
java
// Clean and rebuild your project
Build > Clean Project
Build > Rebuild Project
Checking for Gradle Issues
Gradle issues can sometimes cause NullPointerExceptions. Ensure that your Gradle files are up-to-date and that there are no conflicts between dependencies.
java
// Sync project with Gradle files
Build > Sync Project with Gradle Files
By following these steps and techniques, you can efficiently diagnose and fix NullPointerExceptions in your Android applications, ensuring that your app runs smoothly and provides a great user experience.