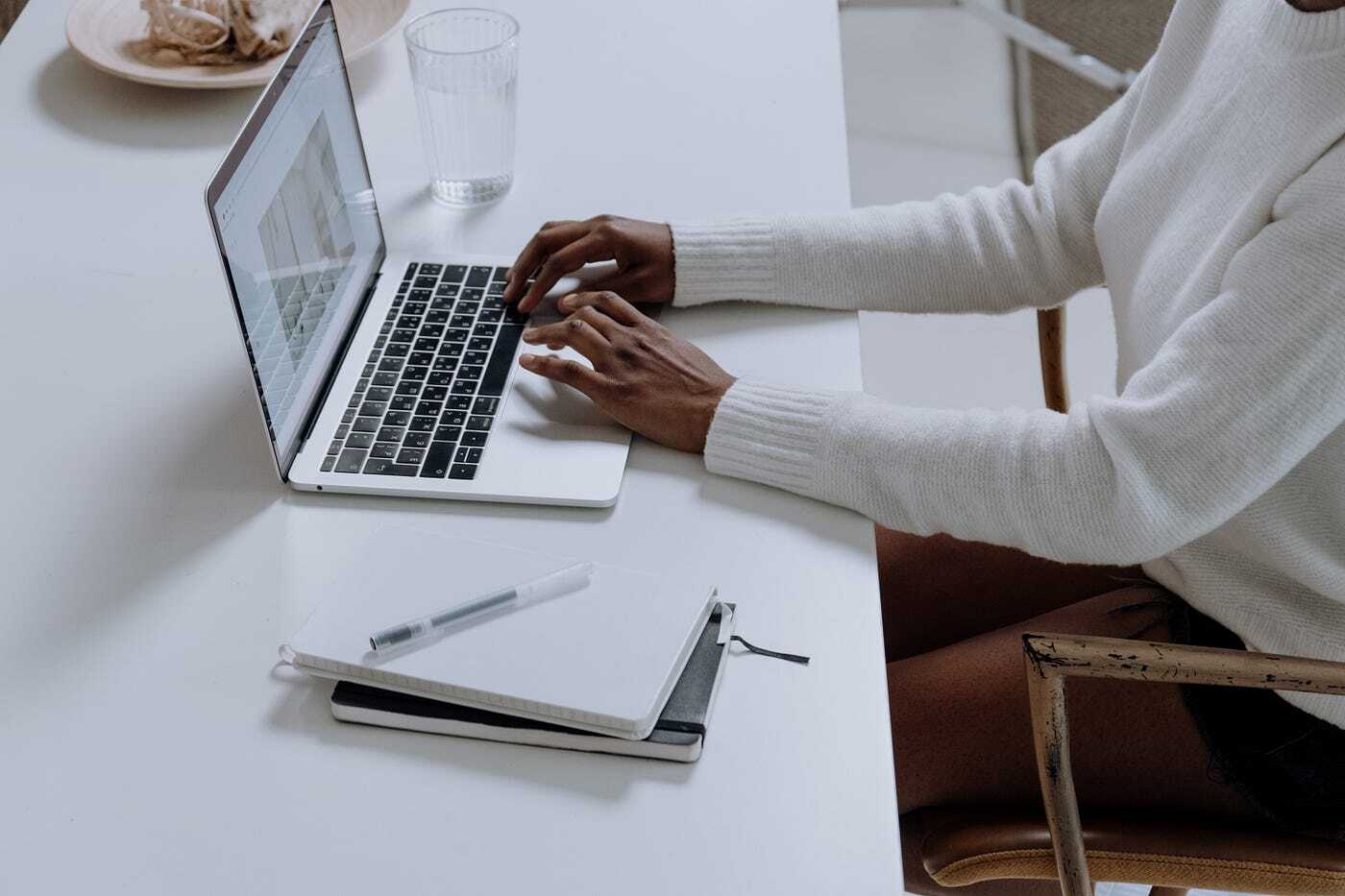
Introduction to Building a Search Engine in Android Studio
Search engines play a crucial role in mobile apps, enhancing user experience by allowing users to quickly find what they need. Whether it's searching for products in a shopping app or finding a specific article in a news app, a well-implemented search function can significantly improve usability. This article will guide you through the process of building a search engine in Android Studio, covering prerequisites, key takeaways, and step-by-step instructions.
Prerequisites
Before diving into building a search engine in Android Studio, ensure you have the necessary tools and knowledge:
- Android Studio Installation: Install Android Studio on your computer. Download it from the official Android website.
- Programming Skills: Basic understanding of Java or Kotlin is required, as these languages are commonly used for developing Android apps.
- XML Knowledge: Familiarity with XML (Extensible Markup Language) is essential for defining the structure of your app's user interface.
- Understanding of Android Apps: Basic knowledge of how Android apps work, including the lifecycle of activities and fragments, is crucial.
Key Takeaways
Building a search engine in Android Studio offers several benefits:
- User-Friendly Interface: Helps users find what they need quickly and easily.
- Enhanced Usability: Integrating features like voice search and caching can make your app faster and more enjoyable to use.
- Testing and Debugging: Thorough testing ensures everything works perfectly, delivering a smooth user experience.
Setting Up Your Project
Creating a New Project
- Open Android Studio: Launch Android Studio on your computer.
- Start a New Project: Click on "Start a new Android Studio project."
- Choose Template: Select a template that fits your needs. For this example, choose "Empty Activity."
- Project Details:
- Name your project.
- Choose a save location.
- Set the language to Java or Kotlin.
- Finish Setup: Click "Finish," and Android Studio will set up your project.
Adding Necessary Dependencies
- Open build.gradle File: Open the
build.gradle
file in theapp
module. - Add Dependencies: Add the following dependencies to your
build.gradle
file:
gradle
dependencies {
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.recyclerview:recyclerview:1.1.0'
implementation 'androidx.cardview:cardview:1.0.0'
}
- Sync Project: Sync your project to download these libraries.
Implementing the Search Engine
Designing the User Interface
- Create Layout XML File: Create a new layout XML file (e.g.,
activity_main.xml
) in theres/layout
directory. Add aSearchView
widget to this layout file:
xml
<androidx.appcompat.widget.SearchView
android:id="@+id/searchView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
app:queryHint="Search Here" />
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
- Inflate Layout in Activity: In your main activity (e.g.,
MainActivity.java
), inflate this layout and initialize theSearchView
.
java
public class MainActivity extends AppCompatActivity {
private SearchView searchView;
private RecyclerView recyclerView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
searchView = findViewById(R.id.searchView);
recyclerView = findViewById(R.id.recyclerView);
// Initialize SearchView
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
// Handle search query submission
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
// Handle search query change
return false;
}
});
}
}
Handling Search Queries
- Create Data Model: Create a data model class (e.g.,
SearchResult.java
) to represent each search result.
java
public class SearchResult {
private String title;
private String description;
public SearchResult(String title, String description) {
this.title = title;
this.description = description;
}
public String getTitle() {
return title;
}
public String getDescription() {
return description;
}
}
- Fetch Data: Implement logic to fetch data based on the search query. For simplicity, assume a list of predefined search results.
java
private List
new SearchResult("Android Studio", "A comprehensive IDE for Android app development"),
new SearchResult("Kotlin", "A modern programming language for Android app development"),
new SearchResult("XML", "A markup language used to define user interfaces in Android apps")
);
// Method to fetch data based on search query
private List
List
for (SearchResult result : searchResults) {
if (result.getTitle().toLowerCase().contains(query.toLowerCase())) {
results.add(result);
}
}
return results;
}
- Update RecyclerView: Update the RecyclerView with the fetched data.
java
// Method to update RecyclerView with fetched data
private void updateRecyclerView(String query) {
List
// Create an adapter for RecyclerView
SearchAdapter adapter = new SearchAdapter(results);
recyclerView.setAdapter(adapter);
}
- Handle Search Query Submission: Handle the submission of search queries by calling the updateRecyclerView method.
java
// Handle search query submission
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
updateRecyclerView(query);
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
updateRecyclerView(newText);
return false;
}
});
Implementing Voice Search
- Add Voice Search Library: Add the necessary dependencies for voice search libraries like Google's Speech Recognition API.
gradle
dependencies {
implementation 'com.google.android.gms:play-services-speech:20.0.0'
}
- Initialize Speech Recognition: Initialize Speech Recognition in your activity.
java
private SpeechRecognizer speechRecognizer;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// Initialize Speech Recognition
speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this);
speechRecognizer.setSpeechRecognizerListener(new SpeechRecognizerListener() {
@Override
public void onResults(Bundle results) {
// Handle recognition results
String query = results.getStringArrayList(SpeechRecognizer.RESULTS_RECOGNITION).get(0);
updateRecyclerView(query);
}
@Override
public void onError(int errorCode) {
// Handle recognition errors
Log.e("SpeechRecognition", "Error: " + errorCode);
}
});
// Initialize SearchView
searchView.setOnQueryTextListener(new SearchView.OnQueryTextListener() {
@Override
public boolean onQueryTextSubmit(String query) {
return false;
}
@Override
public boolean onQueryTextChange(String newText) {
return false;
}
});
// Set up voice search button click listener
Button voiceSearchButton = findViewById(R.id.voiceSearchButton);
voiceSearchButton.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
Intent intent = new Intent(RecognizerIntent.ACTION_RECOGNIZE_SPEECH);
intent.putExtra(RecognizerIntent.EXTRA_LANGUAGE_MODEL, RecognizerIntent.LANGUAGE_MODEL_FREE_FORM);
intent.putExtra(RecognizerIntent.EXTRA_PROMPT, "Please speak your query");
try {
speechRecognizer.startListening(intent);
} catch (Exception e) {
Log.e("SpeechRecognition", "Error starting speech recognition: " + e.getMessage());
}
}
});
}
Implementing Caching
- Use LRU Cache: Use an LRU (Least Recently Used) cache to store frequently searched items.
java
import android.util.LruCache;
private LruCache<String, List
public void initCache() {
int maxCacheSize = (int) Runtime.getRuntime().maxMemory() / 4;
cache = new LruCache<String, List
@Override
protected int sizeOf(String key, List
return value.size();
}
};
}
public List
return cache.get(query);
}
public void putInCache(String query, List
cache.put(query, results);
}
- Update RecyclerView from Cache: Update the RecyclerView with data from the cache if available.
java
// Method to update RecyclerView with fetched data from cache or network
private void updateRecyclerView(String query) {
List
if (resultsFromCache != null && !resultsFromCache.isEmpty()) {
// Update RecyclerView with cached results
SearchAdapter adapter = new SearchAdapter(resultsFromCache);
recyclerView.setAdapter(adapter);
} else {
// Fetch data from network and update RecyclerView
List
putInCache(query, resultsFromNetwork);
SearchAdapter adapter = new SearchAdapter(resultsFromNetwork);
recyclerView.setAdapter(adapter);
}
}
Final Thoughts on Building a Search Engine with Android Studio
Building a search engine with Android Studio involves several key steps:
- Setting Up Your Project: Start by setting up Android Studio and creating a new project.
- Designing the User Interface: Design the user interface where users will input their search queries.
- Handling Search Queries: Handle the submission of search queries by fetching relevant data and updating the RecyclerView.
- Implementing Voice Search: Integrate voice search functionality using Google's Speech Recognition API.
- Implementing Caching: Use an LRU cache to store frequently searched items and improve performance.
Each step plays a pivotal role in delivering swift and accurate search results. With thorough testing and debugging, you can ensure that everything runs smoothly, making your app a powerful tool that users will love.
By following these steps and integrating features like voice search and caching, you can enhance your app's usability significantly, making it more user-friendly and efficient. Building a robust search engine is not just about coding; it's also about understanding user needs and providing them with an intuitive experience.