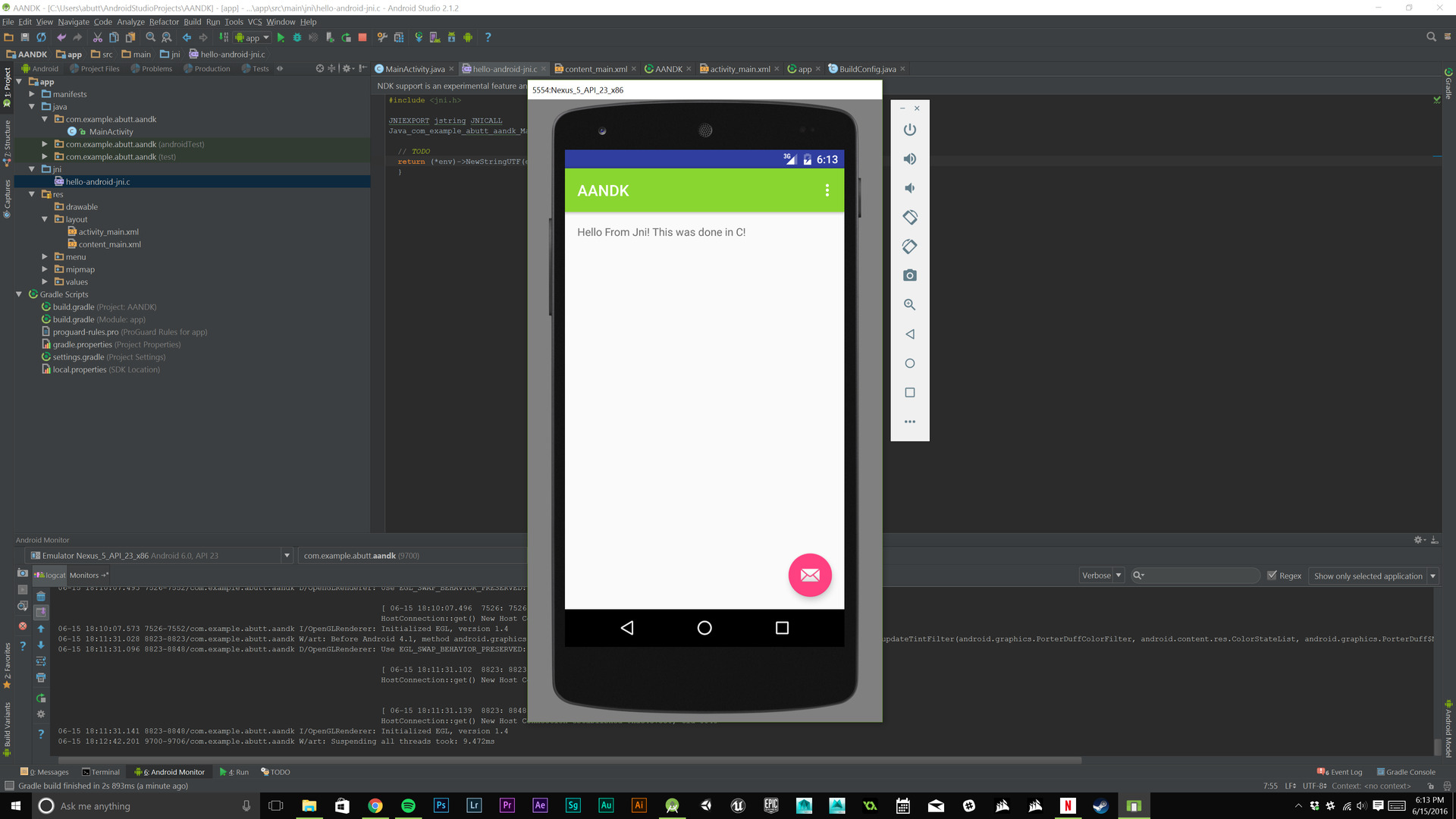
Introduction
Android Studio 1.4 is a powerful integrated development environment (IDE) for developing Android applications. One of its key features is the Native Development Kit (NDK), which allows developers to use C and C++ code alongside Java to enhance the performance and functionality of their apps. This guide will walk you through the process of setting up and using the NDK in Android Studio 1.4, covering everything from downloading and installing the necessary tools to integrating native code into your project.
Setting Up Android Studio
Before diving into the NDK, ensure that the latest version of Android Studio is installed. Here’s how to set up a new project in Android Studio 1.4:
- Launch Android Studio: Open Android Studio on your computer.
- Create a New Project: Select “Start a new Android Studio project” from the Quick Start menu.
- Application Name and Company Name: Enter your application name and company name. This will be used as the package name, so make sure it follows standard Java package naming conventions.
- Minimum SDK: Choose the minimum SDK or Android version that your application will support. This determines the compatibility of your app with different devices.
- Form Factors: Select the form factors your app will support, such as Android Wear, TV, and Auto.
- Project Location: Choose the location where you want to save your project.
- Project Name: Enter a name for your project.
- Language: Select the programming language you want to use (Java or Kotlin).
- Activity Type: Choose the type of activity you want to create (e.g., Empty Activity).
- Finish: Click “Finish” to create the project.
Downloading and Installing the NDK
Once the project is set up, download and install the NDK:
- Accessing SDK Manager: Open the SDK Manager by going to
File > Settings > Appearance & Behavior > System Settings > Android SDK
or by using the tools menuTools > Android > SDK Manager
. - Selecting NDK: In the SDK Manager, navigate to the
SDK Tools
tab and select the checkbox next toAndroid NDK
. - Installing NDK: Click
Apply
and thenOK
to start the installation process. The NDK will be downloaded and installed in the specified directory. - Configuring Properties: After installing the NDK, configure some properties in your project files.
Configuring Gradle
To use the NDK with Gradle, configure it properly:
-
Editing
grade.properties
: Open thegrade.properties
file and add the following property:
plaintext
android.useDeprecatedNdk=true -
Configuring NDK Path: In the
local.properties
file, add the path to your NDK distribution:
plaintext
ndk.dir=[path to sdk]/ndk-bundle -
Building the Project: Once these properties are configured, build your project by selecting
Build > Make project
from the toolbar.
Adding Native Library to Your App
To add a native library to your app, configure Gradle further:
-
Editing
build.gradle
: Open thebuild.gradle
file for your module and add an NDK configuration inside the default config:
plaintext
ndk {
moduleName "_demoproject"
} -
Loading Native Library: Load the native library into your Java code by adding a static initialization block with
System.loadLibrary
and a reference to your native library:
plaintext
static {
System.loadLibrary("_demoproject");
}
Using CMake
Android Studio supports both ndk-build
and CMake for building native libraries. For new native libraries, it is recommended to use CMake:
-
Installing CMake: Ensure that CMake is installed on your system. Download it from the official CMake website.
-
Configuring CMake: In your project’s
build.gradle
file, add the following configuration:
plaintext
android {
…
externalNativeBuild {
cmake {
version "3.10.2"
}
}
} -
Creating CMakeLists.txt: Create a
CMakeLists.txt
file in your native source directory. This file contains the instructions for CMake to build your native library.
Example CMakeLists.txt
file:
plaintext
cmake_minimum_required(VERSION 3.10.2)
project(MyProject)
add_library(mylibrary SHARED mylibrary.cpp)
target_include_directories(mylibrary PRIVATE ${CMAKE_CURRENT_SOURCE_DIR})
target_link_libraries(mylibrary android)
- Building with CMake: After configuring CMake, build your project using Gradle. The native library will be compiled and linked into your APK.
Debugging Native Code
Debugging native code can be challenging, but Android Studio provides tools to help with this process:
- Using LLDB: Android Studio uses LLDB as its debugger for native code. By default, LLDB will be installed alongside Android Studio.
- Setting Breakpoints: Set breakpoints in your native code where you want to pause execution and inspect variables.
- Running Debug Session: Run a debug session by clicking the debug button or pressing
Shift+F9
. The debugger will attach to your process and allow you to step through your code. - Inspecting Variables: Use LLDB commands to inspect variables and understand the state of your program at different points.
Common Issues and Solutions
While setting up and using the NDK can be straightforward, some common issues might arise. Here are solutions to these problems:
Unable to Find Library for Device Architecture
If you are trying to build your app for an older device and getting an error like "Unable to find library for this device's architecture," it might be because the NDK does not support that architecture.
Solution: Ensure that the correct version of the NDK is installed and that it supports the device architecture you are targeting.
NDK Version Compatibility
Sometimes, issues arise due to compatibility between different versions of the NDK and your project's minimum SDK version.
Solution: Check the compatibility of your NDK version with your project's minimum SDK version. Download older unsupported versions of the NDK from the Unsupported NDK Downloads page if needed.
CMake Configuration Issues
If you are using CMake, issues with configuration files or build scripts might occur.
Solution: Ensure that your CMakeLists.txt
file is correctly configured and that all dependencies are properly linked. Check the CMake documentation for more detailed instructions.
Final Thoughts
Using the Native Development Kit (NDK) in Android Studio 1.4 can significantly enhance the performance and functionality of your Android applications. By following this guide, you should be able to set up and use the NDK effectively, whether using ndk-build
or CMake. Configure Gradle properly, use CMake for new native libraries, and debug your code using LLDB. With these tools and techniques, creating high-performance Android apps that take full advantage of native code becomes achievable.