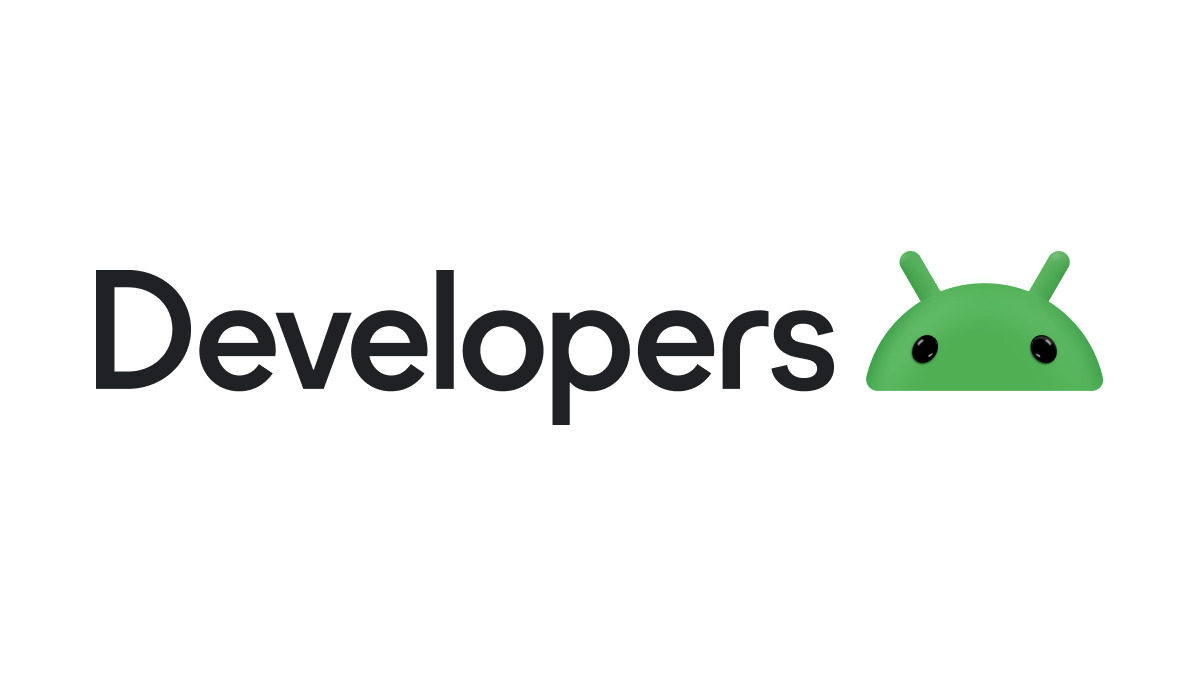
Introduction
Android app development has transformed how we interact with mobile devices. With over 2.43 million apps on the Google Play Store, the demand for skilled Android developers continues to grow. This article covers everything from setting up your development environment to advanced topics like app architecture and publishing your app on the Google Play Store.
Setting Up Your Development Environment
Before developing Android apps, setting up the right tools and software is essential.
Native Android Development
Native Android development targets only Android devices, providing the best performance and user experience. Choose between Android Studio or Visual Studio for this approach.
Using Android Studio
Android Studio is the official Integrated Development Environment (IDE) for Android app development. Follow these steps to get started:
- Download and Install Android Studio: Obtain Android Studio from the official website. The installation process includes setting up necessary SDKs and tools.
- Create a New Project: Launch Android Studio and select "Start a new Android Studio project." Choose the project type, name it, and select the save location.
- Set Up Your Project Structure: The project structure appears in the left-hand pane, including folders for source code, resources, and other files.
- Write Your Code: Begin coding in the
MainActivity.java
file or use Kotlin. The layout editor helps design the user interface. - Run Your App: Click the "Run" button or press Shift+F10 to run your app on an emulator or a connected physical device.
Using Visual Studio
Visual Studio is popular for developing Android apps, especially for cross-platform development using .NET MAUI or React Native. Here’s how to start:
- Download and Install Visual Studio: Obtain Visual Studio from the official website and install it.
- Install the Android SDK: Within Visual Studio, go to Tools > SDK Manager and install necessary Android SDKs.
- Create a New Project: Select "Create a new project" under the Start page. Choose the .NET MAUI or React Native template.
- Set Up Your Project Structure: Visual Studio creates a project structure tailored for cross-platform development.
- Write Your Code: Code in C# or JavaScript, depending on the chosen framework.
- Run Your App: Click the "Run" button or press F5 to run your app on an emulator or a connected physical device.
Cross-Platform Development
Cross-platform frameworks allow apps to run on multiple platforms, including Android, iOS, and Windows. This approach benefits developers who want a single codebase across different platforms.
Using .NET MAUI
.NET MAUI (Multi-platform App UI) is a cross-platform framework by Microsoft. It allows code sharing across platforms using C# and XAML.
- Install .NET MAUI: Obtain .NET MAUI from the official website or through Visual Studio.
- Create a New Project: In Visual Studio, select ".NET MAUI App" under the Start page.
- Set Up Your Project Structure: .NET MAUI creates a project structure with shared and platform-specific code.
- Write Your Code: Code in C# and XAML.
- Run Your App: Click the "Run" button or press F5 to run your app on an emulator or a connected physical device.
Using React Native
React Native, developed by Facebook, uses JavaScript and React to build native mobile apps.
- Install React Native: Use npm or yarn by running
npx react-native init YourProjectName
. - Set Up Your Project Structure: React Native creates a project structure with JavaScript files and native modules.
- Write Your Code: Code in JavaScript and JSX.
- Run Your App: Use the command
npx react-native run-android
to run it on an emulator or physical device.
App Architecture
App architecture is crucial for building robust and maintainable Android apps. Here are some best practices and common architectural principles:
Separation of Concerns
Each component in your app should have a single responsibility.
- Avoid Mixing UI Logic: Activities and Fragments should only contain logic related to UI and operating system interactions.
- Keep Components Lean: Minimize dependencies on Android classes and reduce coupling within your app.
Drive UI from Data Models
Data models represent the app's data and should be independent of UI elements.
- Use Persistent Models: Ensure users don't lose data if the Android OS destroys the app's process from memory.
General Best Practices
- Don't Store Data in App Components: Avoid storing application data and state in app components like Activities or Services.
- Reduce Dependencies on Android Classes: Abstract other classes away from Android framework SDK APIs like Context or Toast.
- Create Well-Defined Boundaries of Responsibility: Define clear responsibilities between various modules.
- Expose as Little as Possible from Each Module: Prevent exposing internal implementation details from modules.
- Focus on Unique Core Features: Concentrate on what makes your app unique.
- Make Each Part Testable in Isolation: Improve maintainability and reduce bugs.
Programming Languages
Android app development primarily uses Java and Kotlin. Here’s a brief overview:
Java
Java was the official programming language of Android until 2019. Many developers still use Java due to its extensive support community and compatibility with the Google Play Store.
Kotlin
Kotlin, declared the preferred language by Google in 2019, offers concise syntax and interoperability with Java.
Dart
Dart, created by Google, focuses on creating apps quickly with built-in features and easy-to-design graphics. It emphasizes user experience during development.
Game Development
Game development for Android differs from standard app development. Games typically use custom rendering logic written in OpenGL or Vulkan. Here’s how to start:
- Use C/C++ with NDK: Developers commonly use C/C++ with the Android Native Development Kit (NDK) for game creation.
- Game Engines: Utilize game engines like Unity, Unreal Engine, Defold, or Godot.
- IDEs: Use IDEs like Android Studio or Visual Studio for game development.
Publishing Your App on Google Play Store
After developing your app, publish it on the Google Play Store. Here’s how:
Registering for a Google Play Developer Account
- Sign Up: Use your Google Account to sign up for a Play Console developer account.
- Review Agreement: Accept the Google Play Developer Distribution Agreement during signup.
- Pay Registration Fee: Pay a one-time registration fee of $25 using accepted credit or debit cards.
- Choose Account Type: Select between Personal and Organization account types.
- Verify Developer Identity Information: Complete verification requirements.
- Add More Information: Add additional account information after creating your account.
Publishing Your App
- Create an App Listing: Include details like title, description, screenshots, and promotional graphics.
- Set Up Pricing: Configure pricing based on different regions and currencies.
- Set Up Testing: Arrange internal or open testing before public release.
- Submit for Review: Submit your app for review by Google Play Store moderators.
- Monitor Performance: Track performance metrics like downloads, revenue, and user reviews through Play Console.
Additional Resources
For further learning and troubleshooting, consider these resources:
- Android Developer Guides: Detailed documentation on building Android apps using APIs in the Android framework and other libraries.
- Codelabs: Short self-paced tutorials covering discrete topics like building small apps or adding new features.
- Courses: Guided training paths teaching how to build Android apps.
- Play Console Support: Resources for troubleshooting common issues related to publishing apps on Google Play.
By following these guidelines, you can become proficient in Android app development and contribute to the ever-growing world of mobile applications.