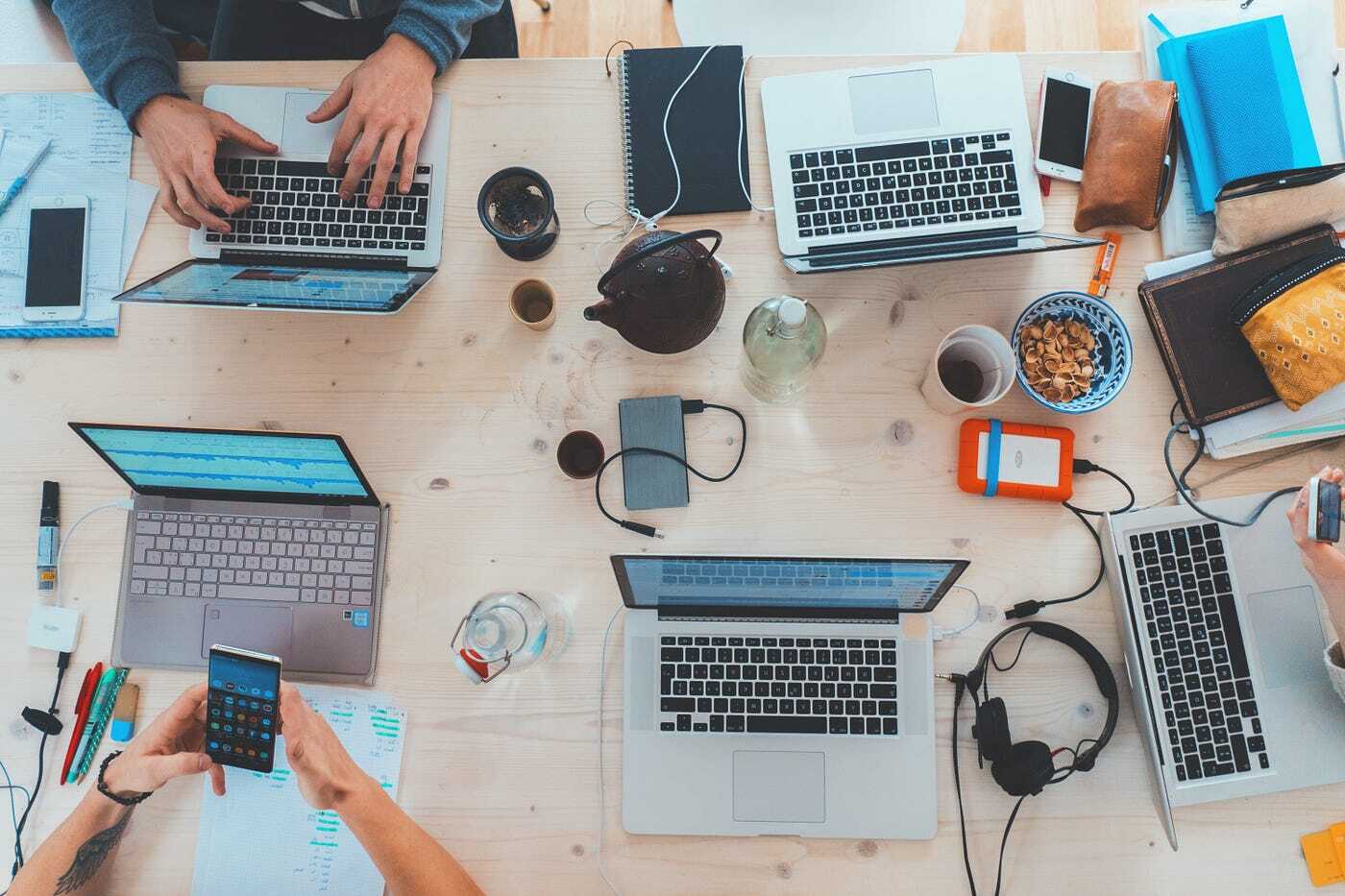
Introduction to Android Command Line Tools
Overview
Android Command Line Tools are a set of utilities that help developers interact with Android devices and manage their development environment. These tools are essential for tasks like debugging, installing apps, and managing device settings. They provide a powerful way to control Android devices directly from a computer's command line interface, making development more efficient and streamlined.
Components of Android SDK
The Android Software Development Kit (SDK) includes several components that are crucial for developing Android apps. Key components include:
- SDK Tools: These are the core tools needed to build and debug Android apps. They include the Android Debug Bridge (ADB), Fastboot, and others.
- Platform Tools: These tools support the latest features of the Android platform. They are updated with each new version of Android.
- Build Tools: These are used to compile and package apps. They include tools like
aapt
(Android Asset Packaging Tool) anddx
(Dalvik Executable). - SDK Manager: This tool helps manage the different SDK packages, allowing developers to download, update, and manage the various components of the SDK.
Setting Up the Environment
Download and Install Command Line Tools
To get started with Android Command Line Tools, you first need to download them from the official Android SDK download page. Here’s how:
- Visit the Android Studio download page.
- Scroll down to the "Command line tools only" section.
- Download the appropriate package for your operating system (Windows, macOS, or Linux).
- Extract the downloaded archive to a preferred location on your computer.
- Open a terminal or command prompt and navigate to the extracted folder to verify the installation.
Set Environment Variables
Setting up environment variables ensures that you can use the command line tools from any directory on your computer. Here’s a quick guide:
-
Windows:
- Right-click on "This PC" or "My Computer" and select "Properties."
- Click on "Advanced system settings" and then "Environment Variables."
- Under "System variables," find the
Path
variable and click "Edit." - Add the path to the
tools
andplatform-tools
directories of your SDK installation.
-
macOS/Linux:
-
Open your terminal.
-
Edit your shell profile file (e.g.,
.bash_profile
,.zshrc
, or.bashrc
). -
Add the following lines:
sh
export ANDROID_HOME=/path/to/your/sdk
export PATH=$PATH:$ANDROID_HOME/tools
export PATH=$PATH:$ANDROID_HOME/platform-tools -
Save the file and run
source ~/.bash_profile
(or the equivalent for your shell) to apply the changes.
-
Core Command Line Tools
ADB (Android Debug Bridge)
ADB is a versatile tool that allows developers to communicate with an Android device. It can be used for a variety of tasks, such as:
- Installing and uninstalling apps: Quickly install or remove apps on a connected device.
- Debugging apps: Access logs and debug information to troubleshoot issues.
- File transfer: Move files between your computer and the device.
- Shell access: Open a command shell on the device to execute commands directly.
Fastboot
Fastboot is a protocol used for flashing filesystems on Android devices. It is particularly useful for:
- Unlocking bootloaders: Enable the ability to install custom firmware.
- Flashing custom recoveries: Install custom recovery images like TWRP.
- Updating firmware: Flash new versions of the Android operating system.
SDK Manager
The SDK Manager is a tool that helps developers manage the various SDK packages. It allows you to:
- Download and update SDK components: Ensure you have the latest tools and libraries.
- Manage system images: Download and manage different versions of Android for testing.
- Install additional tools: Add-ons like Google Play services and other libraries can be easily installed.
Using Command Line Tools for Development
Building and Debugging Apps
Building and debugging Android apps using command line tools can be a game-changer. First, ensure your project is set up correctly. Navigate to your project directory in the terminal. Use Gradle to build your app by running:
sh
./gradlew assembleDebug
This command compiles the code and generates an APK file. To install the APK on a connected device or emulator, use ADB:
sh
adb install -r app/build/outputs/apk/debug/app-debug.apk
For debugging, start by launching the app on your device. Then, use ADB to connect the debugger:
sh
adb shell am start -D -n com.example.yourapp/.MainActivity
Attach the debugger from your IDE or use jdb (Java Debugger) for command line debugging:
sh
jdb -attach localhost:8700
Managing Virtual Devices
Creating and managing Android Virtual Devices (AVDs) is straightforward with command line tools. First, list available device definitions:
sh
avdmanager list device
Create a new AVD using a specific device definition and system image:
sh
avdmanager create avd -n my_avd -k "system-images;android-30;google_apis;x86" -d "pixel"
To start the AVD, use the emulator command:
sh
emulator -avd my_avd
You can also manage AVDs by listing, deleting, or updating them with similar commands.
Emulator
Running the Android Emulator from the command line offers flexibility. To start an emulator, specify the AVD name:
sh
emulator -avd my_avd
For advanced usage, you can pass additional options. For instance, to start the emulator with a specific resolution and RAM size:
sh
emulator -avd my_avd -skin 1080×1920 -memory 2048
To simulate different network conditions, use:
sh
emulator -avd my_avd -netdelay none -netspeed full
These commands help you test your app under various conditions without leaving the command line.
Advanced Usage
Customizing Build Process
Customizing the build process can optimize your workflow. Modify the build.gradle file to include custom tasks. For example, to create a task that cleans and builds the project:
groovy
task cleanBuild(type: Exec) {
commandLine './gradlew', 'clean', 'assembleDebug'
}
Run this task from the command line:
sh
./gradlew cleanBuild
Automating Tasks
Automating repetitive tasks saves time. Use shell scripts or batch files to streamline processes. For instance, create a script to build, install, and launch your app:
sh
#!/bin/bash
./gradlew assembleDebug
adb install -r app/build/outputs/apk/debug/app-debug.apk
adb shell am start -n com.example.yourapp/.MainActivity
Run the script with:
sh
./build_and_run.sh
Jetifier
Jetifier helps migrate libraries to AndroidX. To enable Jetifier, add the following to your gradle.properties file:
properties
android.useAndroidX=true
android.enableJetifier=true
Run the Jetifier tool to update your dependencies:
sh
./gradlew clean build
This ensures compatibility with AndroidX, making your development process smoother.
Troubleshooting and Maintenance
Common Issues and Fixes
Sometimes, command line tools can act up. One common issue is the ADB device not found error. This usually happens when your device isn't properly connected or the drivers aren't installed. To fix this, check your USB connection and ensure the correct drivers are installed.
Another frequent problem is the SDK Manager not opening. This can be due to missing Java Development Kit (JDK) or incorrect environment variables. Verify that the JDK is installed and the JAVA_HOME variable is set correctly.
If you encounter emulator performance issues, it might be due to insufficient system resources. Allocating more RAM and CPU to the emulator often helps. Also, enabling hardware acceleration can significantly boost performance.
Updating Command Line Tools
Keeping your tools up-to-date is crucial for smooth development. To update, open a terminal and navigate to the SDK directory. Run the command sdkmanager --update
. This will fetch and install the latest versions of the command line tools.
For specific tools like ADB or Fastboot, you can use sdkmanager "platform-tools"
. This ensures you have the latest features and bug fixes.
Clearing Cache and Data
Over time, cache and data can pile up, causing tools to slow down or behave unpredictably. To clear the cache for ADB, use the command adb kill-server
followed by adb start-server
. This restarts the ADB server, clearing any temporary issues.
For the emulator, you can clear data by navigating to the AVD Manager and selecting Wipe Data. This resets the virtual device to its original state, removing any accumulated junk.
Best Practices
Version Control Integration
Integrating command line tools with version control systems like Git streamlines your workflow. Start by adding the SDK directory to your .gitignore
file to avoid committing large files. Use Git hooks to automate tasks like running tests before pushing code.
You can also use scripts to manage dependencies. For example, a setup.sh
script can install required SDK packages, ensuring consistency across different environments.
Security Considerations
Security is paramount when using command line tools. Always download tools from official sources to avoid malware. Regularly update your tools to patch vulnerabilities.
When using ADB, be cautious with commands that modify system files. Unauthorized access can compromise your device. Use secure connections and avoid running commands with elevated privileges unless necessary.
Performance Optimization
Optimizing performance can save time and resources. For ADB, use the adb install -r
command to replace existing apps without reinstalling. This speeds up the development cycle.
For the emulator, allocate more RAM and enable hardware acceleration. Use snapshots to quickly restore the emulator to a known state, reducing startup time.
Scripts can automate repetitive tasks, freeing up time for more critical work. For instance, a build script can compile your app, run tests, and deploy it to a device with a single command.
Wrapping It All Up
Technology keeps pushing boundaries, making our lives easier and more exciting. Android Command Line Tools are a prime example of how tech can streamline development, offering powerful utilities like ADB and Fastboot for everything from debugging to flashing firmware. Setting up the SDK and managing AVDs might seem a bit tricky at first, but with practice, it becomes second nature. Remember, keeping tools updated and optimizing performance can save a ton of headaches down the road. So, dive in, experiment, and let these tools take your Android development to the next level. Happy coding!
Introduction to Android Command Line Tools
The Android Command Line Tools empower developers to manage Android devices and projects using text commands. These tools allow for installation and uninstallation of apps, debugging, testing, and building applications. They also enable file transfers, log retrieval, and system updates. Developers can emulate devices, monitor performance, and automate tasks. This feature streamlines development, making it easier to control and optimize Android environments.
Necessary Requirements and Compatibility
To ensure your device supports Android Command Line Tools, check these requirements:
Operating System: Your device must run Android 4.0 (Ice Cream Sandwich) or later. Older versions won't support these tools.
Storage: Ensure at least 100 MB of free space. This space is necessary for downloading and installing the tools.
RAM: A minimum of 1 GB RAM is required. Devices with less memory might struggle with performance.
Processor: A dual-core processor or better is recommended. Single-core processors may experience lag.
USB Debugging: Enable USB Debugging in Developer Options. This setting allows your device to communicate with the command line tools.
Drivers: Install the appropriate USB drivers for your device on your computer. These drivers ensure proper communication between your device and the computer.
ADB and Fastboot: Ensure ADB (Android Debug Bridge) and Fastboot are installed on your computer. These tools are essential for executing commands.
Internet Connection: A stable internet connection is needed for downloading necessary files and updates.
Permissions: Grant necessary permissions on your device when prompted. These permissions allow the tools to function correctly.
Battery: Ensure your device has at least 50% battery or is connected to a power source. Running out of power during operations can cause issues.
Configuring Android Command Line Tools
- Download the Android Command Line Tools from the official Android developer website.
- Extract the downloaded ZIP file to a preferred location on your computer.
- Open your terminal or command prompt.
- Navigate to the extracted folder using the
cd
command. - Run
./sdkmanager --list
to view available packages. - Install necessary packages by running
./sdkmanager "platform-tools" "platforms;android-30"
. - Add the tools to your system's PATH. On Windows, search for "Environment Variables," then edit the PATH variable to include the tools directory. On Mac or Linux, add
export PATH=$PATH:/path/to/tools
to your.bash_profile
or.zshrc
. - Restart your terminal or command prompt.
- Verify installation by running
adb --version
andfastboot --version
.
Done!
Maximizing Use of Command Line Tools
Automate Tasks: Use scripts to automate repetitive tasks. This saves time and reduces errors.
Debugging: Use logcat to view logs. It helps identify issues quickly.
File Management: Use adb push and adb pull to transfer files between your computer and device. This is faster than manual methods.
App Installation: Use adb install to install apps directly from your computer. This is useful for testing.
Network Management: Use adb shell to access the device's shell. You can manage network settings or run commands as if you were on the device.
Backup and Restore: Use adb backup and adb restore to create backups of your device. This is crucial before making major changes.
Screen Recording: Use adb shell screenrecord to record your screen. This is helpful for creating tutorials or debugging UI issues.
Battery Management: Use adb shell dumpsys battery to get detailed battery stats. This helps in optimizing battery usage.
Permissions: Use adb shell pm grant to grant permissions to apps. This is useful for testing apps with specific permissions.
Factory Reset: Use adb shell recovery --wipe_data to perform a factory reset. This is useful for starting fresh or troubleshooting major issues.
Network Speed: Use adb shell netcfg to check network speed and connectivity. This helps in diagnosing network issues.
Device Info: Use adb shell getprop to get detailed device information. This is useful for debugging and support.
Reboot: Use adb reboot to restart your device. This is quicker than using the device's power button.
Custom Commands: Create aliases for frequently used commands. This saves time and makes your workflow more efficient.
Security: Always disconnect adb when not in use. This prevents unauthorized access to your device.
Troubleshooting Common Problems
Battery draining quickly? Close unused apps, lower screen brightness, and turn off Wi-Fi or Bluetooth when not needed.
Phone running slow? Clear cache, uninstall unused apps, and restart the device.
Apps crashing? Update the app, clear its cache, or reinstall it.
Storage full? Delete old files, move photos to cloud storage, and uninstall apps you don't use.
Wi-Fi not connecting? Restart the router, forget the network on your phone, and reconnect.
Bluetooth issues? Turn Bluetooth off and on, unpair and re-pair devices, and restart your phone.
Screen freezing? Force restart the device by holding the power button and volume down button together.
Phone overheating? Close background apps, avoid using the phone while charging, and keep it out of direct sunlight.
Can't receive calls? Check if Do Not Disturb mode is on, ensure Airplane mode is off, and restart your phone.
Poor signal? Move to an area with better reception, restart your phone, and check for carrier outages.
Privacy and Security Tips
Using Android command line tools involves handling user data with care. Encryption is key. Always ensure data is encrypted both in transit and at rest. Avoid storing sensitive information in plain text. Use strong passwords and enable two-factor authentication for added security.
Be cautious with permissions. Only grant necessary permissions to apps and tools. Regularly review and revoke permissions that seem excessive. Keep your device's software updated to protect against vulnerabilities.
For privacy, consider using a VPN to mask your IP address and encrypt your internet connection. Disable location services when not needed to prevent apps from tracking your movements. Clear your cache and cookies regularly to remove stored data that could be exploited.
Lastly, be mindful of public Wi-Fi. Avoid accessing sensitive information on unsecured networks. If you must use public Wi-Fi, ensure your connection is secure by using a VPN.
Comparing Other Options
Pros of Android Command Line Tools:
- Flexibility: Allows for custom scripts and automation.
- Open Source: Free to use and modify.
- Integration: Works well with other Android development tools.
- Community Support: Large user base for troubleshooting.
Cons of Android Command Line Tools:
- Complexity: Steep learning curve for beginners.
- Limited GUI: Mostly text-based, less visual.
- Dependency Issues: Requires proper setup of environment variables.
Alternatives:
iOS Command Line Tools:
- Pros: Seamless integration with Xcode, strong Apple support.
- Cons: Limited to macOS, less community support compared to Android.
Windows PowerShell:
- Pros: Powerful scripting capabilities, integrates with Windows OS.
- Cons: Not as versatile for mobile development, Windows-specific.
Linux Terminal:
- Pros: Highly flexible, strong community support.
- Cons: Requires familiarity with Linux commands, less mobile-specific.
Conclusion:
Android Command Line Tools offer great flexibility and integration but come with a learning curve. iOS tools are well-supported but limited to macOS. PowerShell is powerful for Windows, while Linux Terminal provides flexibility but needs Linux knowledge. Choose based on your specific needs and operating system.
Battery draining quickly? Close unused apps, lower screen brightness, and turn off Wi-Fi or Bluetooth when not needed.
Phone running slow? Clear cache, uninstall unused apps, and restart the device.
Apps crashing? Update the app, clear its cache, or reinstall it.
Storage full? Delete old files, move photos to cloud storage, and uninstall apps you don't use.
Wi-Fi not connecting? Restart the router, forget the network on your phone, and reconnect.
Bluetooth issues? Turn Bluetooth off and on, unpair and re-pair devices, and restart your phone.
Screen freezing? Force restart the device by holding the power button and volume down button together.
Phone overheating? Close background apps, avoid using the phone while charging, and keep it out of direct sunlight.
Can't receive calls? Check if Do Not Disturb mode is on, ensure Airplane mode is off, and restart your phone.
Poor signal? Move to an area with better reception, restart your phone, and check for carrier outages.
Mastering Android Command Line Tools
Mastering Android command line tools can really boost your development skills. These tools offer a lot of power and flexibility, letting you automate tasks, debug apps, and manage devices more efficiently. By getting comfortable with commands like adb, fastboot, and gradle, you can streamline your workflow and tackle complex tasks with ease.
Don't be afraid to experiment and explore different commands. The more you practice, the more intuitive these tools will become. Plus, there are tons of resources and communities out there to help you along the way.
In short, investing time in learning these tools pays off big time. You'll save time, reduce errors, and gain a deeper understanding of how Android works under the hood. So, grab your terminal and start exploring the world of Android command line tools today!
What are Android Command Line Tools?
Android Command Line Tools are a set of utilities that let you manage your Android devices and apps using text commands. They’re great for developers who need to automate tasks or work without a graphical interface.
How do I install Android Command Line Tools?
To install, download the SDK tools package from the Android developer website. Extract the files and add the tools
and platform-tools
directories to your system’s PATH.
What can I do with these tools?
You can do a bunch of stuff like installing apps, debugging, emulating devices, and accessing device logs. They’re super handy for testing and development.
Do I need to be a developer to use them?
Not really. While they’re designed for developers, anyone with basic command line knowledge can use them. They’re useful for tech enthusiasts too.
Are these tools available for all operating systems?
Yes, they work on Windows, macOS, and Linux. Just make sure you download the correct version for your OS.
How do I update the tools?
You can update by downloading the latest version from the Android developer site and replacing the old files. Keeping them updated ensures you have the latest features and bug fixes.
Can I use these tools to root my Android device?
While they can assist in the process, rooting involves more steps and risks. Be careful and make sure you know what you’re doing before attempting to root your device.