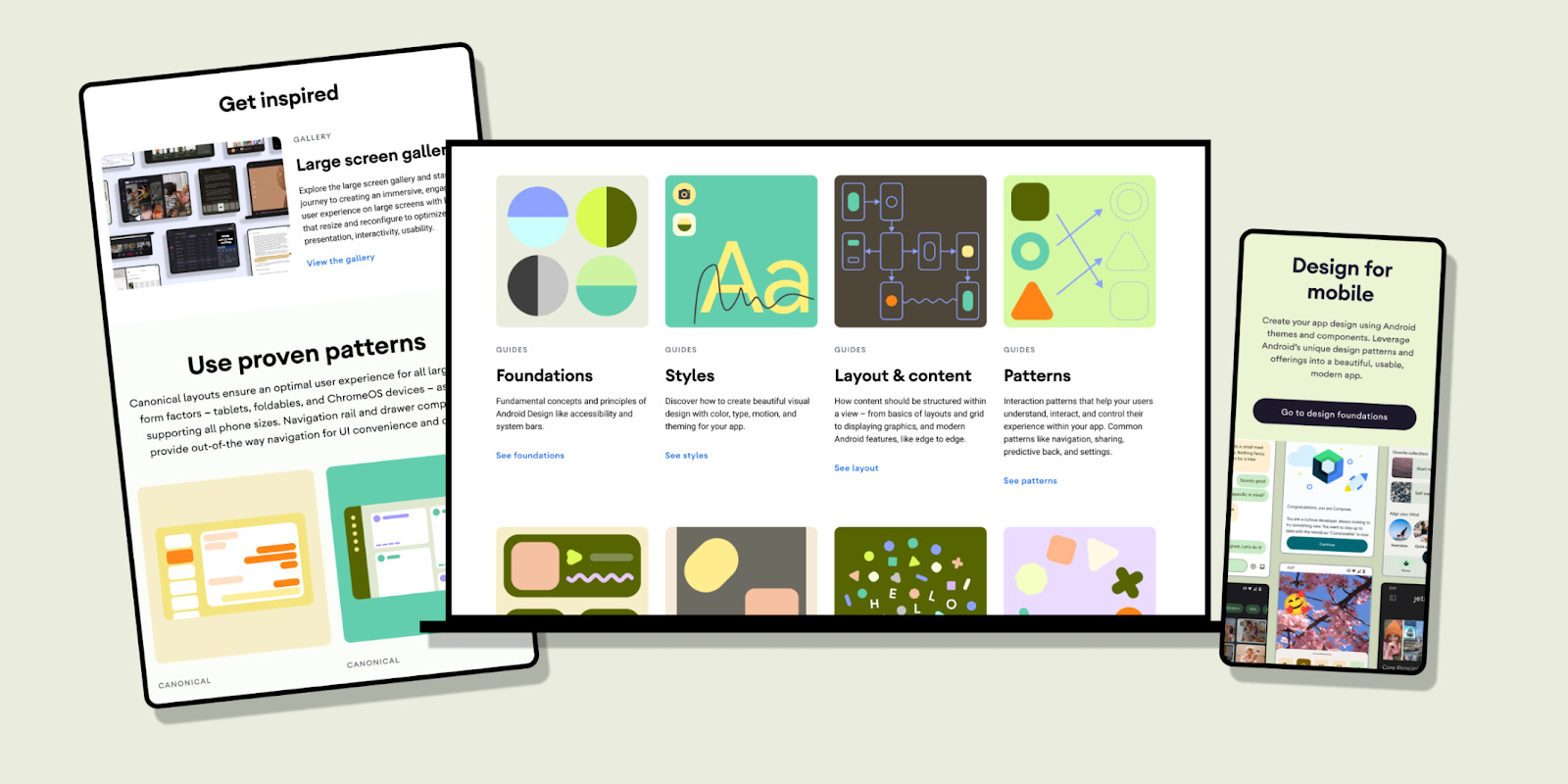
Introduction
Android app development offers numerous opportunities for developers to create innovative and user-friendly applications. With the rise in mobile device usage, the demand for high-quality Android apps has surged. This comprehensive guide covers everything from the basics to advanced topics, including the latest trends and tools.
What is Android App Development?
Android app development involves creating software applications for devices running on the Android operating system. Developed by Google, Android is an open-source platform providing a robust set of tools and APIs for building various applications, from simple games to complex productivity tools.
Key Components of Android App Development
- Programming Languages: Java and Kotlin are the primary programming languages used. Java is the official language, while Kotlin is popular due to its concise syntax and interoperability with Java.
- Integrated Development Environment (IDE): Android Studio is the most widely used IDE, offering a comprehensive set of tools for coding, testing, and debugging applications.
- Android SDK: The Android Software Development Kit (SDK) includes libraries, tools, and APIs essential for building apps that run on a wide range of devices.
- APIs and Libraries: Various APIs and libraries help integrate different functionalities into apps. For example, the Google Maps API adds location-based services, while the Firebase SDK provides tools for real-time data synchronization and analytics.
Steps to Develop an Android App
Developing an Android app involves several stages, each requiring careful planning and execution.
1. Research and Planning
Conduct thorough market research to validate your app idea. Understand the target audience, identify the problem your app solves, and analyze the competition.
Product Discovery:
Product discovery ensures your app meets the needs of your target audience. It involves understanding the market and validating if there is a right fit for your app idea. The DECODE team uses a four-step process taking around 4-10 weeks to complete this phase.
Competitive Analysis:
Analyzing competitors' app performance provides valuable insights. Evaluate their strengths and weaknesses, launch dates, downloads, updates, ratings, and reviews. Identifying their shortcomings helps find creative solutions for your app.
2. Designing the App’s User Interface
Once a wireframe is prepared and the layout finalized, use it to flesh out the rest of your app design. The user interface (UI) significantly impacts usability and user experience. A hard-to-use or unappealing UI can cause users to drop out and look elsewhere.
Design Principles:
Achieving a good UI involves following design principles such as simplicity, consistency, and responsiveness. Tools like Sketch or Figma help create high-fidelity designs that translate well into the development phase.
3. Building the App
With a solid design in place, start building your app using Android Studio. Here’s a high-level overview of the development process:
Setting Up the Project:
Create a new project in Android Studio by selecting the type of app you want to build (e.g., a basic app, a game, or a wearable app). Choose the minimum SDK version and target SDK version based on your requirements.
Writing Code:
Write the code for your app using Java or Kotlin. Start with basic components like activities, fragments, and layouts. Use APIs and libraries provided by the Android SDK to integrate additional functionalities.
Testing and Debugging:
Use testing tools like Espresso for UI testing and JUnit for unit testing to identify and address issues early in the development process. Debugging ensures your app runs smoothly without errors.
4. Testing and Quality Assurance
Testing ensures your app is stable, reliable, and meets expected standards. Here are some key testing strategies:
Unit Testing:
Unit testing involves testing individual components of your app in isolation. Tools like JUnit help write and run unit tests to ensure each module works as expected.
Integration Testing:
Integration testing involves testing how different components of your app interact with each other. This helps identify issues that may arise when different parts of the app are combined.
UI Testing:
UI testing focuses on the user interface of your app. Tools like Espresso help write tests that simulate user interactions and verify that the UI behaves as expected.
Beta Testing:
Beta testing involves releasing your app to a small group of users to gather feedback before the final release. This helps identify issues that may not have been caught during internal testing.
5. Deployment
Once your app is ready, deploy it to the Google Play Store. Here’s how to prepare for deployment:
Creating a Developer Account:
Create a developer account on the Google Play Console to publish your app.
Preparing the App Listing:
Prepare an app listing that includes details like screenshots, descriptions, and keywords. Optimize your listing to improve visibility and attract more downloads.
Publishing the App:
Publish your app by uploading the APK file to the Google Play Store. Follow the guidelines provided by Google to ensure your app meets all requirements.
Advanced Topics in Android App Development
1. Machine Learning and Artificial Intelligence
Machine learning and artificial intelligence are at the forefront of Android app development. Developers leverage these technologies to create apps providing more personalized and intelligent experiences.
Chatbots:
Chatbots can have natural conversations with users, providing instant support and assistance. Tools like Dialogflow help build chatbots that integrate seamlessly with your app.
Recommendation Engines:
Recommendation engines offer tailored content based on user behavior and preferences. This can be achieved using machine learning algorithms that analyze user data to provide relevant recommendations.
Image Recognition:
Image recognition capabilities are essential for augmented reality applications. Tools like Google’s ML Kit provide pre-trained models for image recognition tasks.
2. Security and Privacy
With increasing data breaches and privacy concerns, security and privacy have become paramount in Android app development.
Encryption Techniques:
Robust encryption techniques like SSL/TLS ensure user data is protected during transmission. Implementing end-to-end encryption safeguards sensitive information stored within the app.
Biometric Authentication:
Biometric authentication methods like fingerprint or facial recognition provide an additional layer of security. Tools like Android’s BiometricPrompt API make it easier to integrate biometric authentication into your app.
Compliance with Data Protection Regulations:
Compliance with data protection regulations like GDPR is crucial. Ensure your app adheres to all guidelines set by these regulations to build trust with users.
3. Foldable and Flexible Displays
Foldable and flexible displays have brought a new dimension to Android app development. These displays offer more screen real estate, allowing developers to create more immersive experiences.
Designing for Foldables:
Designing apps for foldables requires careful consideration of how the app will behave when the device is folded or unfolded. Tools like Android’s Foldable API provide guidelines and best practices for designing foldable apps.
Adapting to Different Form Factors:
Adapting your app to different form factors is essential. Use responsive design principles to ensure your app looks and functions well on various devices, including foldables and wearables.
Tools and Technologies for Android App Development
1. Android Studio
Android Studio is the official IDE for Android app development. It provides a comprehensive set of tools for coding, testing, and debugging applications.
Features of Android Studio:
Android Studio includes features like code completion, debugging tools, and project management. It also supports plugins like Gradle and NDK for advanced development tasks.
2. Git and GitHub
Effective version control is essential in collaborative Android app development. Git is a distributed version control system allowing you to track changes to your code, collaborate with team members, and manage code efficiently.
Using Git:
Use Git to manage your codebase by creating branches for different features or releases. Tools like GitHub provide a convenient space for hosting and sharing your code with the development community.
3. Testing Tools
Robust testing is vital for ensuring the quality and reliability of your Android apps. Here are some popular testing tools:
Espresso:
Espresso is a UI testing framework that helps write tests simulating user interactions and verifying that the UI behaves as expected.
JUnit:
JUnit is a unit testing framework that helps write and run unit tests to ensure each module works as expected.
CI/CD Tools:
CI/CD (Continuous Integration/Continuous Deployment) tools like Jenkins, Travis CI, and Bitrise automate the app deployment process. These tools help streamline the testing, building, and deployment of your Android app, reducing the risk of human error and speeding up the release process.
Android app development is a multifaceted journey that demands a well-equipped toolbox. By embracing the latest trends and harnessing the power of these tools, you can build innovative, secure, and user-friendly Android apps that stand out in the competitive app market. Whether you are a beginner or an experienced developer, this guide has provided you with a comprehensive overview of the steps involved in developing an Android app. Stay updated with the latest trends and use the right tools to ensure your app meets the changing needs and expectations of your users.