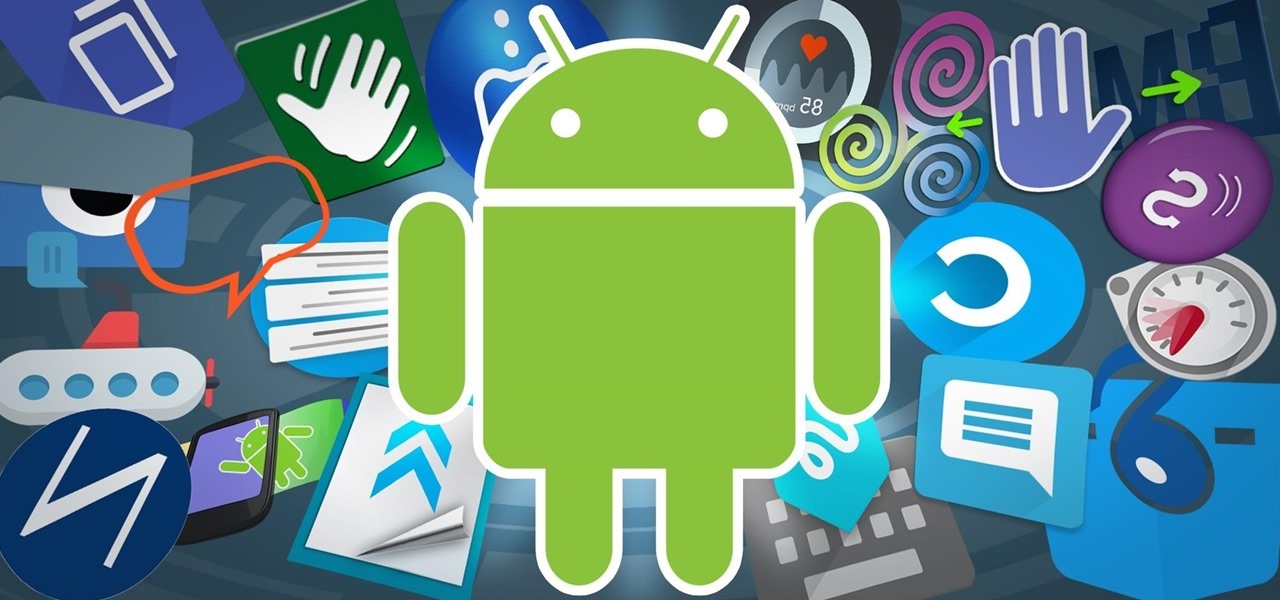
Understanding Android Security
Securing Android apps is vital in today's mobile-centric world. Before diving into security measures, understanding the Android security model is crucial. Android, being an open-source operating system, is more vulnerable to attacks compared to closed-source systems like iOS. However, Google has implemented several security features to protect Android devices and apps:
- Google Play Protect: Scans apps for malware and other threats before installation.
- Google Play Store: The official app store for Android, vetting apps for security before they are available for download.
- SELinux (Security-Enhanced Linux): Ensures applications run in a sandboxed environment, limiting access to sensitive data.
Key Concepts in Android Security
- Permissions: Apps require permissions to access device resources such as the camera, microphone, and location services. Properly managing these permissions is crucial for security.
- Data Encryption: Encrypting data both in transit and at rest helps protect sensitive information from unauthorized access.
- Secure Coding Practices: Following secure coding practices such as input validation, secure storage of credentials, and regular updates can significantly reduce the risk of vulnerabilities.
Setting Up a Secure Development Environment
Ensuring app security starts with a secure development environment. This involves:
- Using Secure Tools: Utilize tools like Android Studio, which includes built-in security features such as lint checks that help identify potential security issues.
- Code Analysis: Regularly run code analysis tools to detect vulnerabilities in your code.
- Version Control: Use version control systems like Git to track changes and collaborate securely.
Essential Tools for Android Security
- Android Studio: The official integrated development environment (IDE) for Android development, which includes features like lint checks and code analysis.
- Burp Suite: A tool for web application security testing that can be used to analyze network traffic and identify potential vulnerabilities.
- Apktool: A tool for reverse engineering Android APK files, which can help identify potential security issues.
- Dex2jar: Converts Android APK files to Java ARchive (JAR) files, making it easier to analyze the code.
- JD-GUI: A tool for viewing and editing Java class files, useful for analyzing the code of an Android app.
- Drozer: Analyzes the security of Android apps by simulating attacks and identifying vulnerabilities.
- GoatDroid App: An open-source penetration testing framework designed specifically for Android apps.
- QARK (Quick Android Review Kit): Automates the process of reviewing an Android app's security by checking for common vulnerabilities.
Securing Android Apps
Securing an Android app involves several steps:
Secure Coding Practices
- Input Validation: Always validate user input to prevent SQL injection and cross-site scripting (XSS) attacks.
- Secure Storage of Credentials: Store sensitive data like passwords securely using techniques like hashing and salting.
- Regular Updates: Regularly update your app to patch known vulnerabilities and fix bugs.
Permissions Management
- Request Only Necessary Permissions: Only request permissions that are necessary for the app's functionality.
- Handle Permissions at Runtime: Handle permissions at runtime rather than at install time to ensure that users are aware of what data the app is accessing.
Data Encryption
- Encrypt Data in Transit: Use HTTPS to encrypt data when transmitting it over the network.
- Encrypt Data at Rest: Use full-disk encryption or encrypt sensitive data stored on the device.
Authentication and Authorization
- Implement Strong Authentication: Implement strong authentication mechanisms like biometric authentication (fingerprint or face recognition) or two-factor authentication.
- Use Secure Protocols for Authentication: Use secure protocols like OAuth or OpenID Connect for authentication.
Secure Network Communication
- Use HTTPS: Always use HTTPS for secure communication between the app and the server.
- Enforce Minimum TLS Version: Enforce the use of minimum TLS version to prevent attacks on weak encryption algorithms.
Obfuscation
- Obfuscate App Logic: Use tools like Appdome to obfuscate app logic, making it harder for attackers to reverse-engineer the code.
Regular Security Audits
- Perform Regular Security Audits: Regularly perform security audits using tools like Burp Suite or QARK to identify potential vulnerabilities.
Advanced Security Techniques
Pin to Host
- What is Pin to Host?: Enforces the use of pre-defined cipher suites and TLS versions, minimizing risks associated with weak encryption algorithms and outdated security protocols.
- How to Implement Pin to Host?: Implement Pin to Host using tools like Appdome, which provides a simple three-step process to upload your app, select the Pin to Host feature, and build your app with enhanced security.
Mobile Bot Detection
- What is Mobile Bot Detection?: Essential for identifying and blocking automated attacks on your app.
- How to Implement Mobile Bot Detection?: Implement mobile bot detection using tools like Appdome's MobileBOTâ„¢ Defense, which provides features like Deep Proxy Detection to identify and block bots.
Best Practices for Secure App Development
Follow Secure Coding Guidelines
- Use Secure Libraries: Use secure libraries and frameworks designed with security in mind.
- Avoid Common Vulnerabilities: Avoid common vulnerabilities like SQL injection and XSS attacks by following secure coding guidelines.
Test Your App Thoroughly
- Perform Unit Testing: Ensure that individual components of your app are working correctly.
- Perform Integration Testing: Ensure that different components of your app are working together correctly.
- Perform Penetration Testing: Simulate real-world attacks and identify vulnerabilities.
Use Secure Storage Mechanisms
- Use KeyStore: Store sensitive data like keys and certificates securely.
- Use SharedPreferences: Store small amounts of data securely.
Additional Resources
For further learning, consider the following resources:
- LinkedIn Learning Course on Securing Android Apps: This course provides an in-depth look at the Android security model and techniques to harden an Android app.
- Appdome's Mobile App Security Build System: This system provides tools like Pin to Host and Obfuscate App Logic to enhance the security of your app.
- Ghera: This resource provides guidelines on how to secure Android apps, including code snippets and examples of good practices.
By following these guidelines and using the right tools, you can create a secure and reliable Android app that protects user data and ensures a safe user experience.