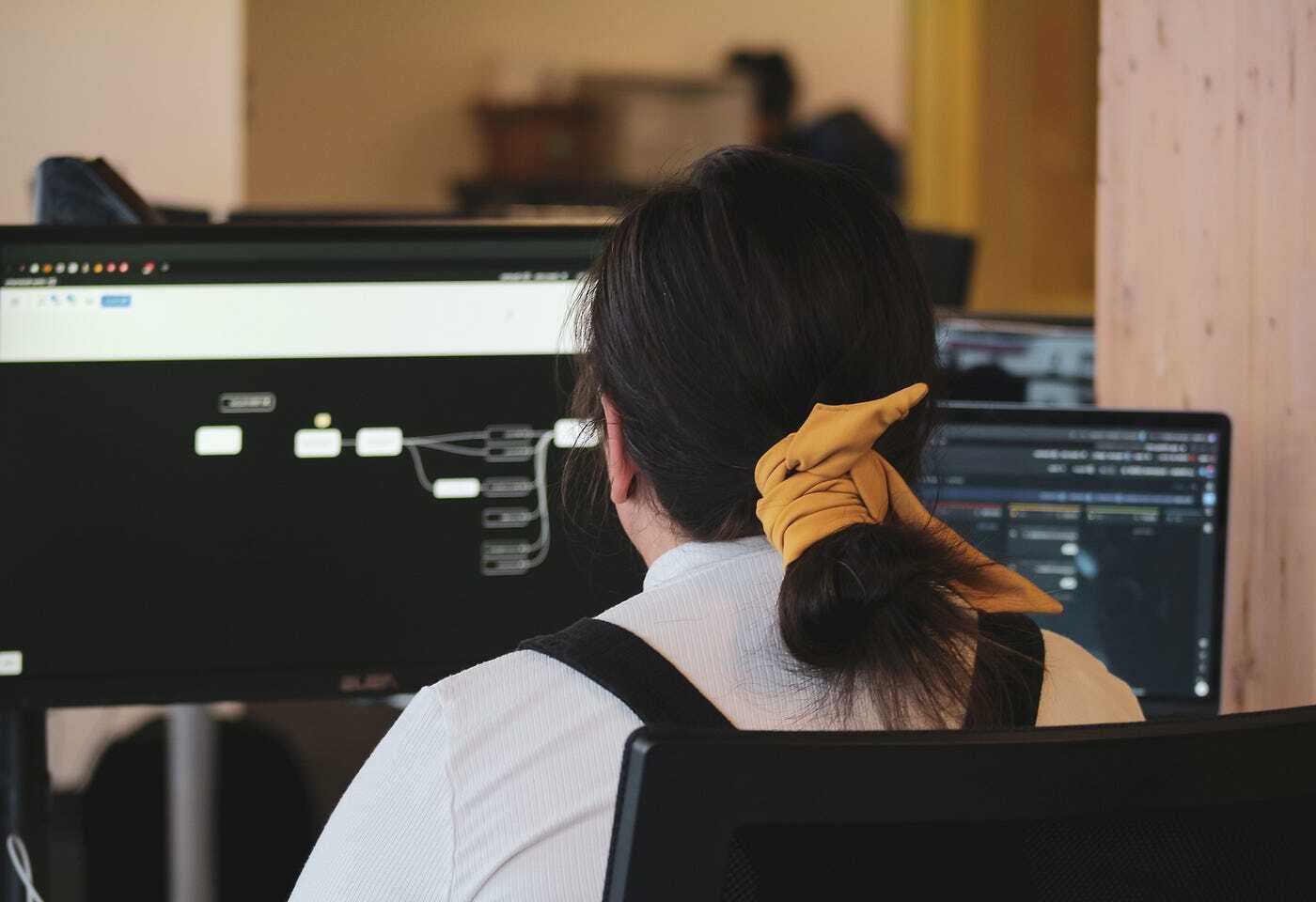
Introduction
Integrating RESTful web services is a crucial aspect of creating robust and feature-rich Android applications. One of the most popular libraries for handling network requests in Android is Retrofit. Developed by Square, Retrofit simplifies the process of interacting with web services by providing a type-safe REST client for Android, Java, and Kotlin. This guide covers setup, API integration, network requests, and best practices for using Retrofit in your Android projects.
What is Retrofit?
Retrofit is a powerful networking library designed to make consuming RESTful web services in Android applications easier. It allows developers to define API endpoints as simple Java interfaces with annotations, making interaction with web services straightforward. The library supports both synchronous and asynchronous network requests, ensuring your app remains responsive and efficient.
Key Benefits of Retrofit
- Easy to Use: Provides a simple way to interact with web services through annotated Java interfaces.
- Type Safety: Ensures type safety, preventing errors due to incorrect data types.
- Synchronous and Asynchronous Calls: Supports both synchronous and asynchronous network requests.
- Dynamic URLs: Allows for dynamic URLs in API endpoints.
- Converters: Supports various converters like Gson, Jackson, and OkHttp for parsing responses.
- Request Cancellation: Enables request cancellation, useful for handling network failures.
- Mock Responses: Supports mock responses using tools like MockWebServer for testing purposes.
Setting Up Retrofit
To use Retrofit in your Android project, add the necessary dependencies to your build.gradle
file:
groovy
dependencies {
implementation 'com.squareup.retrofit2:retrofit:2.9.0'
implementation 'com.squareup.retrofit2:converter-gson:2.9.0'
}
Next, create a Retrofit instance with your base URL and converter factory:
java
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.addConverterFactory(GsonConverterFactory.create())
.build();
Defining API Endpoints
Create an interface with methods annotated with Retrofit’s annotations to define API endpoints. Here’s an example for retrieving users:
java
public interface API {
@GET("users")
Call<List
}
In this example, the @GET
annotation specifies that the getUsers
method should make a GET request to the /users
endpoint. The Call
object returned by Retrofit allows handling the response asynchronously or synchronously.
Making API Calls
Once API endpoints are defined, create an instance of the Retrofit client and use it to make API calls:
java
API api = retrofit.create(API.class);
Call<List
call.enqueue(new Callback<List
@Override
public void onResponse(Call<List
if (response.isSuccessful()) {
List
// Handle the response data
} else {
// Handle the error
}
}
@Override
public void onFailure(Call<List<User>> call, Throwable t) {
// Handle the failure
}
});
Best Practices for Retrofit
Use Model Classes
Define model classes to parse JSON responses. For example, if retrieving a list of users, you might have a User
class with fields like id
, name
, and email
.
java
public class User {
@SerializedName("id")
private int id;
@SerializedName("name")
private String name;
@SerializedName("email")
private String email;
// Constructor and getters
}
Handle Errors
Always handle errors in API calls. Use the onFailure
method of the Callback
interface to manage failures.
Use Interceptors
Interceptors can add headers, modify requests, or handle responses. For example, use an interceptor to add an authentication token to every request.
Cache Responses
Caching responses can improve performance by reducing the number of requests made to the server. Retrofit supports caching through OkHttp.
Mock Responses
Use tools like MockWebServer to test API calls without hitting the actual server. This is especially useful during development and testing phases.
Support Multiple HTTP Methods
Retrofit supports various HTTP methods like GET, POST, PUT, and DELETE. Use the appropriate annotation for each method.
Use Converters
Converters like Gson and Jackson can parse JSON responses into Java objects.
Request Cancellation
Use request cancellation to handle network failures and improve the overall user experience.
Advanced Features of Retrofit
Using OkHttp
Retrofit uses OkHttp under the hood for making HTTP requests. Customize OkHttp by adding interceptors or modifying its configuration.
java
OkHttpClient okHttpClient = new OkHttpClient.Builder()
.addInterceptor(new Interceptor() {
@Override
public Response intercept(Chain chain) throws IOException {
Request request = chain.request();
Request newRequest = request.newBuilder()
.addHeader("Authorization", "Bearer YOUR_TOKEN")
.build();
return chain.proceed(newRequest);
}
})
.build();
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.client(okHttpClient)
.addConverterFactory(GsonConverterFactory.create())
.build();
Using Interceptors
Interceptors can add headers, modify requests, or handle responses. Here’s an example of adding an interceptor to include an authentication token:
java
OkHttpClient okHttpClient = new OkHttpClient.Builder()
.addInterceptor(new Interceptor() {
@Override
public Response intercept(Chain chain) throws IOException {
Request request = chain.request();
Request newRequest = request.newBuilder()
.addHeader("Authorization", "Bearer YOUR_TOKEN")
.build();
return chain.proceed(newRequest);
}
})
.build();
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("https://api.example.com/")
.client(okHttpClient)
.addConverterFactory(GsonConverterFactory.create())
.build();
Using Mock Responses
Mock responses can test API calls without hitting the actual server. Tools like MockWebServer can create mock responses.
java
MockWebServer server = new MockWebServer();
server.enqueue(new MockResponse().setResponseCode(200).setBody("{"name":"John Doe"}"));
server.start(8080);
Retrofit retrofit = new Retrofit.Builder()
.baseUrl("http://localhost:8080/")
.addConverterFactory(GsonConverterFactory.create())
.build();
API api = retrofit.create(API.class);
Call
call.enqueue(new Callback
@Override
public void onResponse(Call
if (response.isSuccessful()) {
User user = response.body();
// Handle the response data
} else {
// Handle the error
}
}
@Override
public void onFailure(Call<User> call, Throwable t) {
// Handle the failure
}
});
Example Use Cases
GET Request
A GET request retrieves data from a server. Here’s an example of performing a GET request using Retrofit:
java
interface UserApiService {
@GET("users/{id}")
suspend fun getUser(@Path("id") userId: String): Response
}
val retrofit = Retrofit.Builder()
.baseUrl(BASE_URL)
.addConverterFactory(GsonConverterFactory.create())
.build()
val apiService = retrofit.create(UserApiService::class.java)
// Making the GET request
val response = apiService.getUser("999")
if (response.isSuccessful) {
val user = response.body()
// Handle the user data
} else {
// Handle the error
}
POST Request
A POST request sends data to a server. Here’s an example of performing a POST request using Retrofit:
java
interface UserApiService {
@POST("users")
suspend fun createUser(@Body user: User): Response
}
val user = User("Romman Sabbir", "rommansabbir@gmail.com")
// Making the POST request
val response = apiService.createUser(user)
if (response.isSuccessful) {
val createdUser = response.body()
// Handle the created user data
} else {
// Handle the error
}
PUT Request
A PUT request updates existing resources on the server. Here’s an example of performing a PUT request using Retrofit:
java
interface UserApiService {
@PUT("users/{id}")
suspend fun updateUser(@Path("id") userId: String, @Body user: User): Response
}
// Making the PUT request
val response = apiService.updateUser("999", user)
if (response.isSuccessful) {
val updatedUser = response.body()
// Handle the updated user data
} else {
// Handle the error
}
Next Steps
Now that you have a solid understanding of Retrofit, it’s time to take your skills to the next level. Here are some recommendations for further learning and exploration:
- Explore Retrofit’s Official Documentation: Learn more about Retrofit’s features and examples.
- Take Online Courses and Tutorials: Improve your skills in Android networking and Retrofit.
- Join Online Communities and Forums: Connect with other developers and get help with Retrofit-related issues.
- Experiment with Different APIs and Projects: Practice your Retrofit skills and learn from real-world examples.
Practice makes perfect. Keep learning, experimenting, and improving your skills to become proficient in Retrofit and Android development. Happy coding!
Feature Overview
Retrofit simplifies network requests in Android apps. It converts HTTP API into a Java interface, making it easy to call web services. It handles JSON parsing, error handling, and asynchronous operations. With Retrofit, developers can easily send requests, receive responses, and manage data. It supports various converters like Gson, Moshi, and Protobuf. This tool also integrates seamlessly with RxJava for reactive programming.
Compatibility and Requirements
To ensure your device supports this feature, check these requirements:
- Operating System: Your device must run Android 8.0 (Oreo) or later. Older versions won't support the feature.
- RAM: At least 2GB of RAM is necessary. Devices with less memory might struggle or fail to run the feature smoothly.
- Storage: Ensure you have at least 500MB of free storage. This space is needed for installation and operation.
- Processor: A quad-core processor or better is recommended. Slower processors might lead to lag or crashes.
- Screen Resolution: The feature works best on devices with a minimum resolution of 720p. Lower resolutions might affect visual quality.
- Battery: A battery capacity of 3000mAh or more is ideal. High power consumption could drain smaller batteries quickly.
- Connectivity: Wi-Fi or 4G LTE is required for optimal performance. Slower connections may cause delays or interruptions.
- Permissions: Ensure you grant necessary permissions like location, storage, and camera access. Without these, the feature might not function correctly.
- Updates: Keep your device updated with the latest security patches and software updates. Outdated software can cause compatibility issues.
Check these details to confirm your device can handle the feature without issues.
How to Set Up
Download the Retrofit library from the official website or GitHub.
Open your Android Studio project.
Add the Retrofit dependency in your
build.gradle
file: groovy implementation 'com.squareup.retrofit2:retrofit:2.9.0' implementation 'com.squareup.retrofit2:converter-gson:2.9.0'Sync your project to download the dependencies.
Create an interface for your API endpoints: java public interface ApiService { @GET("users/{user}/repos") Call<List
> listRepos(@Path("user") String user); } Build a Retrofit instance in your main activity or a singleton class: java Retrofit retrofit = new Retrofit.Builder() .baseUrl("https://api.github.com/") .addConverterFactory(GsonConverterFactory.create()) .build();
Create an implementation of the API endpoints defined by the interface: java ApiService service = retrofit.create(ApiService.class);
Make a network request using the service: java Call<List
> repos = service.listRepos("octocat"); repos.enqueue(new Callback<List >() { @Override public void onResponse(Call<List > call, Response<List > response) { if (response.isSuccessful()) { List repoList = response.body(); // Handle the response } } @Override public void onFailure(Call<List > call, Throwable t) { // Handle the error } }); Run your app to see Retrofit in action.
Effective Usage Tips
Battery Life: Lower screen brightness and turn off unused features like Bluetooth or Wi-Fi. Use battery saver mode when needed.
Storage Management: Regularly clear cache and delete unused apps. Store photos and videos in cloud services like Google Photos.
Security: Enable two-factor authentication and use a strong password. Keep your device updated with the latest security patches.
Customization: Use widgets for quick access to important apps. Change your wallpaper and theme to suit your style.
Productivity: Use split-screen mode to multitask efficiently. Download productivity apps like Google Keep or Trello.
Connectivity: Use Wi-Fi calling if cellular signal is weak. Connect to trusted networks only to avoid security risks.
Camera Use: Experiment with different modes like panorama or night mode. Use grid lines to improve photo composition.
App Management: Organize apps into folders for easy access. Use app shortcuts for quick actions.
Voice Commands: Utilize Google Assistant for hands-free operation. Set up voice match for personalized responses.
Backup: Regularly backup your data to Google Drive. Enable automatic backups for peace of mind.
Performance: Restart your device periodically to clear memory. Disable or uninstall bloatware that slows down your phone.
Notifications: Customize notification settings to reduce distractions. Use Do Not Disturb mode during important tasks.
Accessibility: Enable features like magnification or text-to-speech for easier use. Adjust font size and display settings for better readability.
Updates: Keep your device updated with the latest software. Enable automatic updates for apps and system software.
Data Usage: Monitor and manage data usage with built-in tools. Use data saver mode to limit background data consumption.
Troubleshooting Common Problems
Battery draining too fast? Lower screen brightness, close unused apps, and turn off Wi-Fi or Bluetooth when not needed.
Phone running slow? Clear cache, delete unused apps, and restart the device.
Apps crashing? Update the app, clear its cache, or reinstall it.
Can't connect to Wi-Fi? Restart the router, forget the network on your phone, then reconnect.
Storage full? Delete old photos, videos, and apps. Move files to cloud storage or an SD card.
Overheating? Avoid using the phone while charging, close background apps, and keep it out of direct sunlight.
Screen unresponsive? Restart the phone, remove any screen protector, and clean the screen.
Bluetooth not working? Turn Bluetooth off and on, unpair and re-pair the device, or restart the phone.
Can't receive texts? Check signal strength, ensure the phone isn't in airplane mode, and restart the device.
Apps not downloading? Check internet connection, clear Google Play Store cache, and ensure there's enough storage space.
Privacy and Security Tips
Using this feature, security and privacy become top priorities. Your data gets encrypted, ensuring only you can access it. To maintain privacy, always use strong passwords and enable two-factor authentication. Avoid public Wi-Fi when accessing sensitive information. Regularly update your device to patch any vulnerabilities. Be cautious of phishing attempts; never click on suspicious links. Adjust app permissions to limit data access. Use a VPN for an extra layer of security. Always back up your data to prevent loss.
Comparing Alternatives
Pros of Android:
- Customization: Android allows extensive customization of the user interface, unlike iOS, which has a more rigid structure.
- Variety: Numerous devices from different manufacturers offer a wide range of choices in terms of features, design, and price.
- Google Integration: Seamless integration with Google services like Gmail, Google Drive, and Google Photos.
- Expandable Storage: Many Android devices support microSD cards for additional storage, unlike iPhones.
- App Availability: Access to a vast number of apps on the Google Play Store, often with more free options compared to the Apple App Store.
Cons of Android:
- Fragmentation: Different devices run different versions of Android, leading to inconsistent user experiences and delayed updates.
- Security: More susceptible to malware and security threats compared to iOS.
- Bloatware: Many manufacturers pre-install unnecessary apps, which can’t always be removed.
- Battery Life: Varies significantly between devices, with some models having poor battery performance.
- Customer Support: Support quality can vary widely depending on the manufacturer.
Alternatives:
- iOS (Apple): Offers a more controlled and secure environment, regular updates, and superior customer support.
- Windows Phone: Provides a unique interface and integration with Microsoft services, though app availability is limited.
- HarmonyOS (Huawei): A newer system with growing app support, focusing on seamless integration across Huawei devices.
- KaiOS: Aimed at feature phones, offering essential apps and services for basic internet and smartphone functionality.
Making the Most of Retrofit
Retrofit simplifies API integration for Android apps. It handles network requests, parsing responses, and error handling efficiently. With annotations, you can define endpoints, request methods, and parameters without boilerplate code. Retrofit supports converters like Gson, making JSON parsing a breeze.
To get started, add Retrofit to your project, define your API interface, and create a Retrofit instance. Use it to make asynchronous calls and handle responses in a background thread. Retrofit's flexibility allows customization, like adding interceptors for logging or modifying requests.
By mastering Retrofit, you'll streamline your app's network communication, improve code readability, and reduce development time. It's a powerful tool for any Android developer aiming to build robust, maintainable apps. Dive in, experiment, and watch your productivity soar.
What is Retrofit in Android development?
Retrofit is a type-safe HTTP client for Android and Java. It simplifies network requests by converting HTTP API into a Java interface.
How does Retrofit work?
Retrofit uses annotations to describe HTTP requests. It then converts the API into a Java interface and handles the network operations for you.
Why should I use Retrofit over other libraries?
Retrofit is known for its simplicity and ease of use. It supports various data formats like JSON and XML, integrates well with OkHttp, and offers built-in error handling.
Can Retrofit handle different data formats?
Yes, Retrofit can handle JSON, XML, and other data formats. You can use converters like Gson, Moshi, or Simple XML to parse the data.
Is Retrofit suitable for large-scale applications?
Absolutely! Retrofit is scalable and can handle complex API requests. It's widely used in large-scale applications due to its flexibility and robustness.
How do I integrate Retrofit into my Android project?
To integrate Retrofit, add the Retrofit dependency to your build.gradle file, create a Java interface for your API, and use annotations to define your HTTP methods.
Does Retrofit support asynchronous requests?
Yes, Retrofit supports both synchronous and asynchronous requests. You can use Call objects for synchronous requests or enqueue for asynchronous ones.