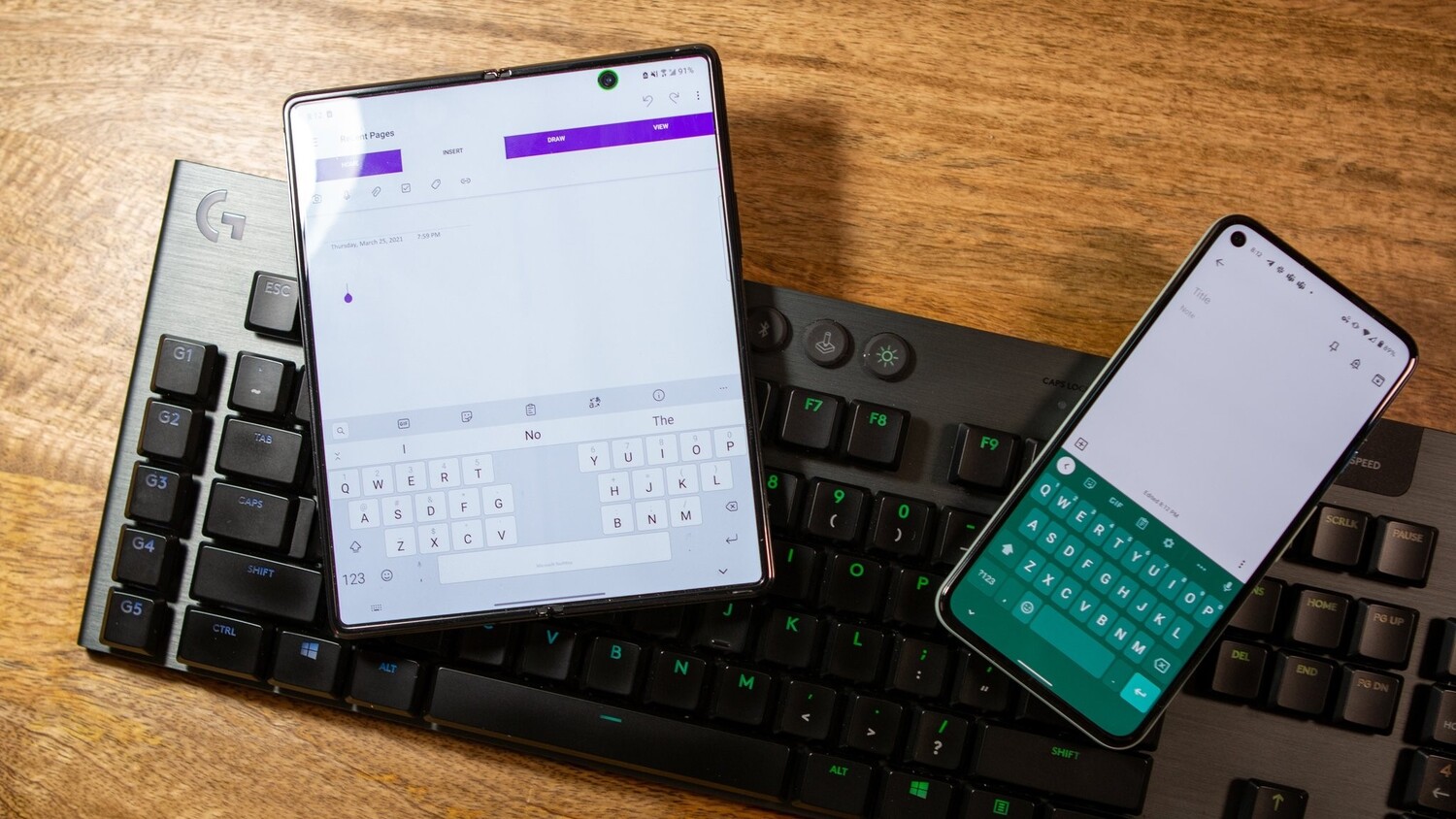
Prerequisites
Before starting, ensure you have a basic understanding of Android app development, including activities, layouts, and resources. You will also need Android Studio installed on your computer and an Android device or emulator for testing.
Step 1: Create a New Android Project
Open Android Studio
Visit the Android Studio website, download the latest version, and follow the installation instructions. Once installed, open Android Studio.
Create a New Project
- Click on "Start a new Android Studio project" in the welcome screen.
- Choose "Empty Activity" as the project template.
- Name your project (e.g., CustomKeyboard).
- Set the package name (e.g., com.example.customkeyboard).
- Set the minimum SDK to API 21 (Android 5.0 Lollipop) or higher.
- Click "Next" and then "Finish."
Step 2: Create the Keyboard Layout
Create a New XML Layout File
-
In the 'res/layout' folder, create a new XML layout file named 'keyboard.xml'.
-
Add the following code to define a basic linear layout for your keyboard:
xml
Add Custom Keyboard Keys
Inside the LinearLayout, add buttons or other views to represent the keys of your custom keyboard. For example, you might add a Button for each key:
xml
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:orientation="vertical"
android:background="@android:color/darker_gray">
<!-- Example of adding a button for each key -->
<Button
android:id="@+id/key_1"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Key 1" />
<Button
android:id="@+id/key_2"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="Key 2" />
<!-- Add more buttons as needed -->
</LinearLayout>
Step 3: Create the KeyboardView Class
Create a New Java Class
Create a new Java class named CustomKeyboardView
and extend the InputMethodService
class.
Override onCreateInputView() Method
In this method, inflate your custom keyboard layout using the getLayoutInflater()
method.
java
import android.inputmethodservice.InputMethodService;
import android.view.View;
public class CustomKeyboardView extends InputMethodService {
@Override
public View onCreateInputView() {
View keyboardView = getLayoutInflater().inflate(R.layout.keyboard, null);
return keyboardView;
}
}
Step 4: Register the Custom Keyboard in the Manifest
Open AndroidManifest.xml
Open the AndroidManifest.xml file and add the following code inside the <application>
tag:
xml
<service
android:name=".CustomKeyboardView"
android:permission="android.permission.BIND_INPUT_METHOD">
<meta-data
android:name="android.view.im"
android:resource="@xml/method" />
<intent-filter>
<action android:name="android.view.InputMethod" />
</intent-filter>
</service>
Create Input Method XML
-
Create a new folder named 'xml' inside the 'res' folder.
-
Inside this folder, create a new XML file named 'method.xml'.
-
Add the following code to specify the class name of your custom keyboard:
xml
<!-- Specify the class name of the custom keyboard --> <subclass android:name=".CustomKeyboardView" /> <!-- Optional settings activity --> <!-- <settings android:activity="com.example.customkeyboard.KeyboardSettingsActivity" /> -->
Step 5: Test the Custom Keyboard
Build and Run the App
Build and run your app on an Android device or emulator.
Enable Custom Keyboard
- Go to 'Settings' > 'Language & input' > 'Virtual keyboard' > 'Manage keyboards', and enable your custom keyboard.
- Now, you can select your custom keyboard as the input method in any app that requires text input.
Additional Considerations
Designing Layout and Appearance
Designing the layout and appearance of your custom keyboard is crucial for user experience. Use XML and Java code to customize the layout, including adding custom keys, changing colors, and adjusting sizes.
Implementing Functionality
Implementing the functionality of your custom keyboard involves handling key presses, text input, and other events. Use Java code to implement these functionalities, such as handling key presses by overriding methods like onKey()
in the KeyboardView
class.
Testing and Maintenance
Testing your custom keyboard thoroughly is essential to ensure it works correctly and is user-friendly. Test it on different devices and scenarios to identify any bugs or issues. Additionally, maintaining and updating your custom keyboard as needed is important for fixing bugs or adding new features.