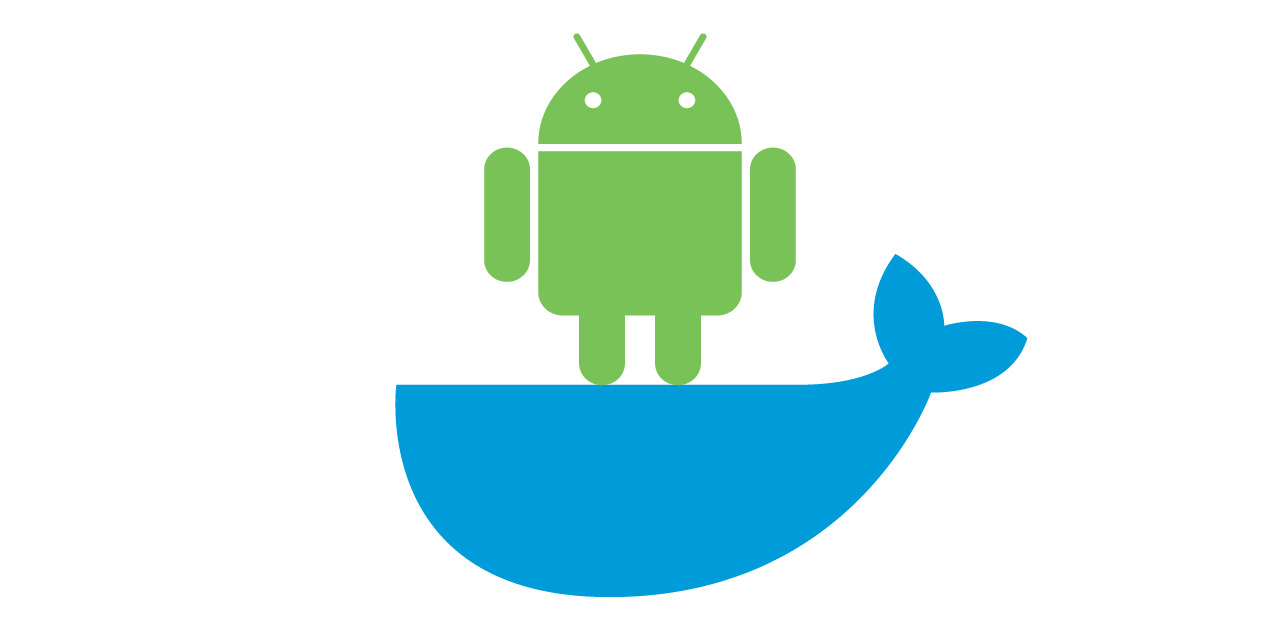
Introduction to Docker and Android Studio
What is Docker?
Docker is a containerization platform enabling developers to package applications and dependencies into a single container. This container can run on any machine with Docker installed, regardless of the operating system. Containers are lightweight and efficient, making them faster to start and stop compared to virtual machines. This efficiency benefits developers working on large projects or those needing to share development environments with team members.
What is Android Studio Docker?
Android Studio Docker leverages Docker's power to containerize the Android development environment. This integration creates isolated environments, ensuring consistent builds and reducing the "it works on my machine" issues. Containerizing the development setup allows easy sharing of configurations, streamlining workflows, and boosting productivity.
Benefits of Using Android Studio Docker
- Consistent Environments: Create consistent environments across different machines, ensuring a predictable and reliable development process.
- Simplified Dependency Management: Manage project dependencies easily by containerizing them, making it simpler to reproduce the same environment on any machine.
- Easy Collaboration: Facilitate collaboration among team members by sharing the containerized development environment, eliminating the need for individual setups.
- Enhanced Testing: Run tests in isolated environments, keeping the development environment clean and manageable for thorough testing and debugging.
- Efficiency and Speed: Docker containers are lightweight and fast to start and stop, saving time in large projects compared to virtual machines.
Setting Up Android Studio Docker
Step 1: Install Docker
Download and install Docker from the official website. Follow the installation prompts based on your operating system (Windows, macOS, or Linux).
Step 2: Pull the Android Studio Image
Open your terminal and pull the Android Studio image using:
docker pull androidstudio/android-studio
This command downloads the pre-configured Android Studio image from Docker Hub.
Step 3: Create a Container
Create a new container with:
docker run -d –name android-studio -p 6080:6080 androidstudio/android-studio
This command creates a container named android-studio
, maps port 6080 on your local machine to port 6080 in the container, and runs it in detached mode.
Step 4: Access Android Studio
Open your web browser and navigate to http://localhost:6080
to access Android Studio within the container.
Step 5: Configure Android Studio
Follow the on-screen instructions to set up Android Studio. This includes setting up the SDK manager by going to Configure > SDK Manager
, selecting necessary SDK versions, and installing them.
Step 6: Create a Project
Click on Start a new Android Studio project
. Choose a template (e.g., "Empty Activity"), name your project, choose a save location, and set the language to Java or Kotlin. Hit "Finish," and Android Studio will set up your project.
Step 7: Run Emulator
Open the AVD Manager from the toolbar, create a new virtual device, and start it.
Step 8: Connect to Container
Access the container's terminal using:
docker exec -it android-studio /bin/bash
This command allows interaction with the container's terminal.
Step 9: Transfer Files
Copy files between your local machine and the container with:
docker cp
Replace <local-path>
with the path on your local machine and <container-path>
with the path inside the container.
Step 10: Stop and Remove Container
Stop the container using:
docker stop android-studio
Remove it if no longer needed with:
docker rm android-studio
Practical Applications of Android Studio Docker
- Consistent Builds: Ensure consistent builds across different machines, crucial for large-scale projects with multiple developers.
- Collaboration: Share the containerized development environment for easier collaboration without individual setups.
- Efficient Testing: Run tests in isolated environments, keeping the development environment clean and manageable.
- Development Speed: Docker containers are lightweight and fast to start and stop, saving time in large projects compared to virtual machines.
- Portability: Docker containers can run on any machine with Docker installed, offering unparalleled portability for moving projects between different machines.
Building a Search Engine in Android Studio
Prerequisites
Ensure Android Studio is installed and have a basic understanding of Java or Kotlin, XML, and how Android apps work.
Setting Up Your Project
-
Create a New Project:
- Open Android Studio and click on "Start a new Android Studio project."
- Choose a template (e.g., "Empty Activity").
- Name your project, choose a save location, and set the language to Java or Kotlin.
- Hit "Finish," and Android Studio will set up your project.
-
Add Necessary Dependencies:
- Open the
build.gradle
file in theapp
module. - Add necessary dependencies for search functionality (e.g.,
appcompat
,recyclerview
,cardview
). - Sync your project to download these libraries.
- Open the
-
Design the Layout:
- Open
activity_main.xml
. - Add a
SearchView
and aRecyclerView
.
- Open
-
Create Data Model:
- Define a class for your data items (e.g.,
Item
class).
- Define a class for your data items (e.g.,
-
Set Up Adapter:
- Create an adapter for the
RecyclerView
.
- Create an adapter for the
-
Integrate Search Functionality:
- Implement search functionality in your app.
-
Test Thoroughly:
- Ensure everything works perfectly by testing thoroughly.
Final Thoughts
Android Studio Docker simplifies the development process by providing consistent environments, easy collaboration, efficient testing, and portability. Integrating Docker with Android Studio enhances productivity and streamlines workflows. Understanding how to use Android Studio Docker can transform your development experience, making it smoother and more reliable.