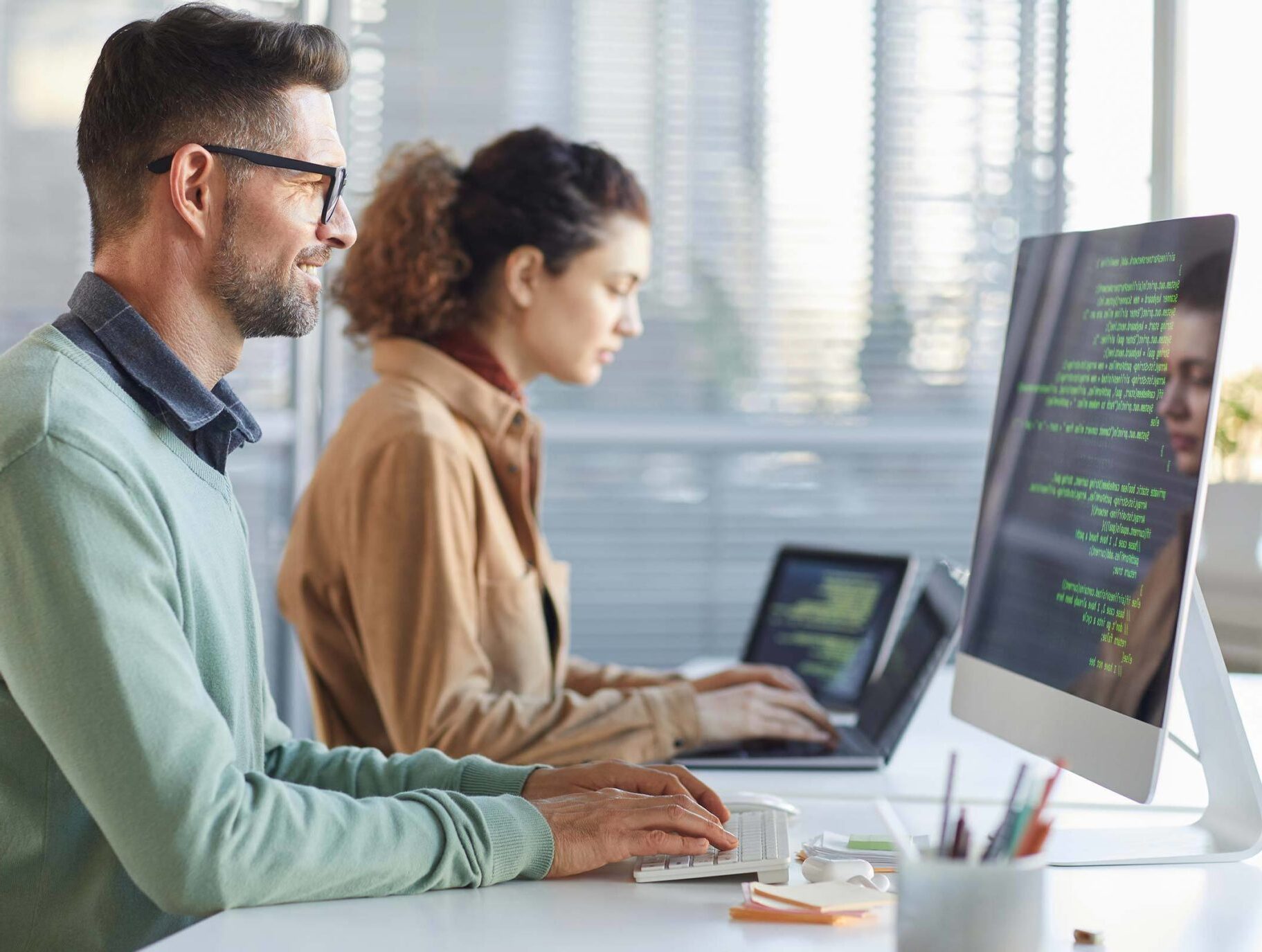
Introduction to Android Studio 3.0
Released in 2017, Android Studio 3.0 marked a significant milestone in Android app development tools. This version introduced several innovative features enhancing coding efficiency, debugging capabilities, and overall user experience. One of the most notable additions was the full integration of Kotlin, a modern and expressive programming language.
Key Features of Android Studio 3.0
-
Kotlin Support
- Kotlin, a statically typed language running on the Java Virtual Machine (JVM), offers concise syntax, high interoperability with Java, and robust null safety features. Android Studio 3.0 fully integrates Kotlin support, including code completion, debugging, and refactoring tools.
-
Java 8 Language Features
- Support for Java 8 language features includes lambda expressions, method references, and functional interfaces, improving code readability and conciseness.
-
Android Profiler
- The Android Profiler provides real-time performance monitoring, allowing checks on memory, CPU, and network usage to optimize app performance.
-
Layout Editor
- Enhanced Layout Editor supports drag-and-drop functionality, making UI design more intuitive and user-friendly.
-
APK Analyzer
- The APK Analyzer helps optimize app size by providing detailed insights into the contents of your APK file, identifying areas to reduce size without compromising functionality.
-
Instant Apps
- Instant Apps support lets users run apps without installation, useful for trying out an app without committing to a full installation.
-
Instant Run
- Instant Run allows quick viewing of changes without restarting the app, saving time during debugging sessions.
-
Project View
- Project View helps organize code by keeping files tidy, grouping related files in folders for easier navigation.
-
Keyboard Shortcuts
- Learning keyboard shortcuts, like
Ctrl + Shift + N
for finding files quickly, enhances coding efficiency.
- Learning keyboard shortcuts, like
-
Debugging Tools
- Comprehensive debugging tools allow setting breakpoints to pause code execution and inspect variables, identifying issues faster.
-
Android Emulator
- The Android Emulator tests apps on different devices with various screen sizes and resolutions, ensuring seamless functionality across devices.
-
Version Control
- Integrating Git for version control tracks changes and facilitates collaboration, crucial for maintaining a clean codebase.
-
ConstraintLayout
- ConstraintLayout, a flexible and responsive UI design tool, ensures apps look good on all devices by defining constraints between views.
-
Plugins
- Plugins like Kotlin, Firebase, or ButterKnife enhance functionality and simplify development tasks, integrating additional features into Android Studio.
-
Code Analysis
- Running Lint checks identifies potential bugs and performance issues, ensuring high code quality and reducing errors.
-
Refactor Code
- Refactor tools allow renaming, moving, or changing code structure without breaking functionality, keeping code clean and maintainable.
-
Unit Tests
- Writing and running unit tests ensures code works as expected, reducing bugs and improving reliability by catching errors early.
-
Documentation
- Commenting code using Javadoc makes it easier for others (and future you) to understand, essential for maintaining a well-documented codebase.
-
Sample Projects and Tutorials
- Sample projects and tutorials provide practical insights and shortcuts for common tasks, invaluable for learning from experienced developers.
Best Practices for Android App Development
Maintaining high code quality is key to a successful project. Here are some best practices to achieve this:
Code Quality
- Lint Checks: Regularly run lint checks to analyze code for potential issues like performance problems and resource leaks.
- Code Analysis: Use tools like Lint to identify potential bugs and performance issues.
- Refactor Code: Utilize refactor tools to rename, move, or change code structure without breaking functionality.
- Comment Code: Use Javadoc for commenting code to make it easier for others to understand.
Version Control
- Git Integration: Integrate Git for version control to track changes and collaborate with others.
- Branching: Employ branching strategies like feature or release branching to manage different versions of the codebase.
- Pull Requests: Use pull requests to review changes before merging them into the main branch.
Continuous Integration
- CI Tools: Use continuous integration tools like Jenkins or Travis CI to automate the build process.
- Automated Testing: Write automated tests that run on every build to ensure code works as expected.
- Deployment Automation: Automate deployment processes using tools like Fastlane or Fabric.
Debugging
- Breakpoints: Set breakpoints to pause code execution and inspect variables.
- Logcat: Use Logcat to view logs and debug issues.
- Espresso: Use Espresso for UI testing to ensure the app's UI works correctly.
Testing
- Unit Tests: Write unit tests to ensure individual components work correctly.
- Integration Tests: Write integration tests to ensure different components work together seamlessly.
- UI Tests: Use tools like Espresso or UI Automator to write UI tests.
Performance Optimization
- Android Profiler: Use the Android Profiler to monitor memory, CPU, and network usage.
- APK Analyzer: Use the APK Analyzer to optimize app size by identifying unnecessary resources.
- Instant Run: Use Instant Run to quickly see changes without restarting the app.
Tips for Efficient Development
Here are some tips to help develop efficiently:
Organize Your Code
- Project View: Use Project View to keep files tidy by grouping related files in folders.
- Folder Structure: Maintain a clean folder structure by organizing files logically.
Use Shortcuts
- Keyboard Shortcuts: Learn keyboard shortcuts for common tasks like finding files (
Ctrl + Shift + N
) or navigating between files (Ctrl + Tab
). - Code Completion: Use code completion features like auto-completion and code snippets to speed up coding.
Debug Efficiently
- Breakpoints: Set breakpoints strategically to pause code execution at critical points.
- Inspect Variables: Use the debugger to inspect variables and understand code flow.
- Log Statements: Print debug information using log statements to understand what's happening in the app.
Test Thoroughly
- Unit Tests: Write unit tests to ensure individual components work correctly.
- Integration Tests: Write integration tests to ensure different components work together seamlessly.
- UI Tests: Use tools like Espresso or UI Automator to write UI tests.
Stay Updated
- Regular Updates: Regularly update Android Studio and SDK tools to access the latest features and improvements.
- Plugin Updates: Keep plugins like Kotlin, Firebase, or ButterKnife updated to ensure they work seamlessly with the latest versions of Android Studio.
Android Studio 3.0 is a powerhouse in Android app development, offering features like Kotlin support, Java 8 language features, and a robust Android Profiler. By following best practices like version control and continuous integration, developers can streamline their workflow and maintain high-quality code. Whether you're a beginner or an experienced developer, this guide provides a comprehensive overview of how to get the most out of Android Studio 3.0. By following these tips and best practices, you can create efficient, reliable, and high-performance Android apps that meet the demands of modern mobile users.