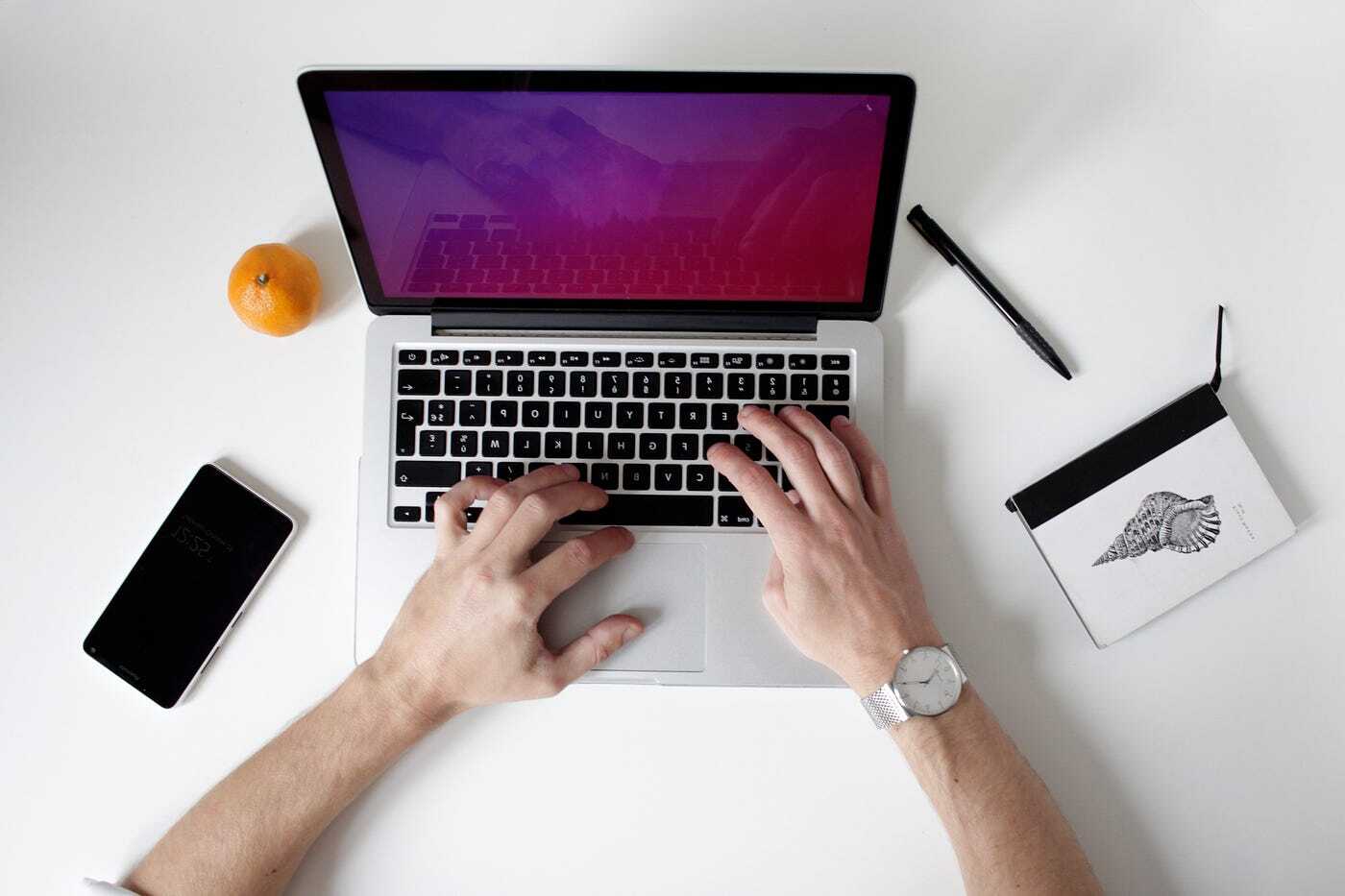
Introduction to Android Studio
Android Studio serves as the official Integrated Development Environment (IDE) for Android app development. It offers a comprehensive set of tools and features, simplifying the creation, testing, and debugging of apps. The IDE includes the Android SDK, which contains essential tools, libraries, and APIs for Android development. Additionally, Android Studio utilizes the Gradle Build System, automating the build process to ensure proper app compilation.
Transitioning from Android to iOS
Switching from Android to iOS development can be smoother than anticipated. Here are key points to consider:
Programming Languages
- iOS: Primarily uses Swift and Objective-C. Swift, a modern language, integrates seamlessly with Apple's ecosystem.
- Android: Primarily uses Java and Kotlin. Kotlin, designed to be more concise and safer than Java, is gaining popularity.
Integrated Development Environment (IDE)
- iOS: Xcode is the preferred IDE, offering a comprehensive set of tools for creating, testing, and distributing apps.
- Android: Android Studio provides similar tools and features but with a different interface and functionalities.
UI Design
- iOS: Historically relied on storyboards, visual design methods showing app progressions. SwiftUI is now gaining traction for faster, more efficient code building.
- Android: Uses XML layouts, wireframes depicting different layouts within the app and screen elements they contain.
Interface Elements
- iOS: Utilizes UIKit for design and has introduced SwiftUI for user interfaces.
- Android: Employs Views and ViewGroups, providing an interactive skeleton of app operations on Android devices.
Key Differences in Design
Understanding the design philosophies of both platforms is crucial:
Minimalism vs Flexibility
- iOS: Emphasizes minimalism and simplicity, featuring elegant, intuitive designs with limited color palettes and simple shapes.
- Android: Offers more flexibility and customization, often featuring complex layouts with gradients, animations, and rich colors.
Navigation Design
- iOS: Navigation design is straightforward and intuitive, with clear buttons and minimal clutter.
- Android: Navigation design can be more complex, offering multiple customization options and animations.
Design Guidelines
- iOS: Adheres to Apple’s Human Interface Guidelines (HIG), ensuring UI compliance with Apple’s design standards.
- Android: Follows Material Design guidelines, providing a framework for creating visually appealing, consistent user interfaces.
Steps to Create an iOS App Using Android Studio
While Android Studio is not ideal for creating iOS apps directly, the following steps can help transition your skills:
Set Up Your Environment
- Continue using Android Studio for learning app development basics. For actual iOS app creation, set up Xcode on a Mac.
- If new to both platforms, set up both Xcode and Android Studio to familiarize yourself with their environments.
Learn Swift and Objective-C
- Swift, the primary language for iOS development, is designed for ease of learning and use. Objective-C, though less common, remains supported.
- Learn the basics of Swift and Objective-C through Apple’s official documentation or online courses.
Understand iOS Architecture
- iOS architecture relies on view controllers, managing entire screens or parts of them. Types include page view, tab, and split view controllers.
- Write view controllers in code or organize them in a storyboard, stored as an XML file, reducing errors and increasing development pace.
Familiarize Yourself with Xcode
- Xcode, the preferred IDE for iOS development, includes tools for creating, testing, and distributing apps.
- Explore Xcode’s interface and learn to use tools like the Interface Builder, which allows visual UI creation by dragging and dropping components.
Start with a Simple Project
- Begin with a simple project to get familiar with Xcode’s workflow and tools.
- Create a new project in Xcode and follow Apple’s tutorials to start your first iOS app.
Transitioning from Android Concepts
- Many concepts are similar but differ in terminology and implementation.
- For instance, Android uses activities and fragments to manage screens, while iOS uses view controllers.
Debugging and Testing
- Debugging and testing are crucial. Xcode includes a built-in simulator for testing apps across various iOS devices and screen sizes.
- Apple’s TestFlight platform enables easy distribution of beta versions to testers, facilitating feedback and iterative improvements.
Publishing Your App
- After developing and testing your app, publish it on the App Store.
- Ensure no bugs exist and follow Apple’s guidelines for app submission to ensure a smooth approval process.
Creating an iOS app using Android Studio is possible with the right tools and knowledge. While Android Studio offers comprehensive tools for Android development, Xcode provides a more streamlined experience for iOS development. Understanding the key differences between iOS and Android design philosophies and leveraging existing app development knowledge can make the transition smoother.