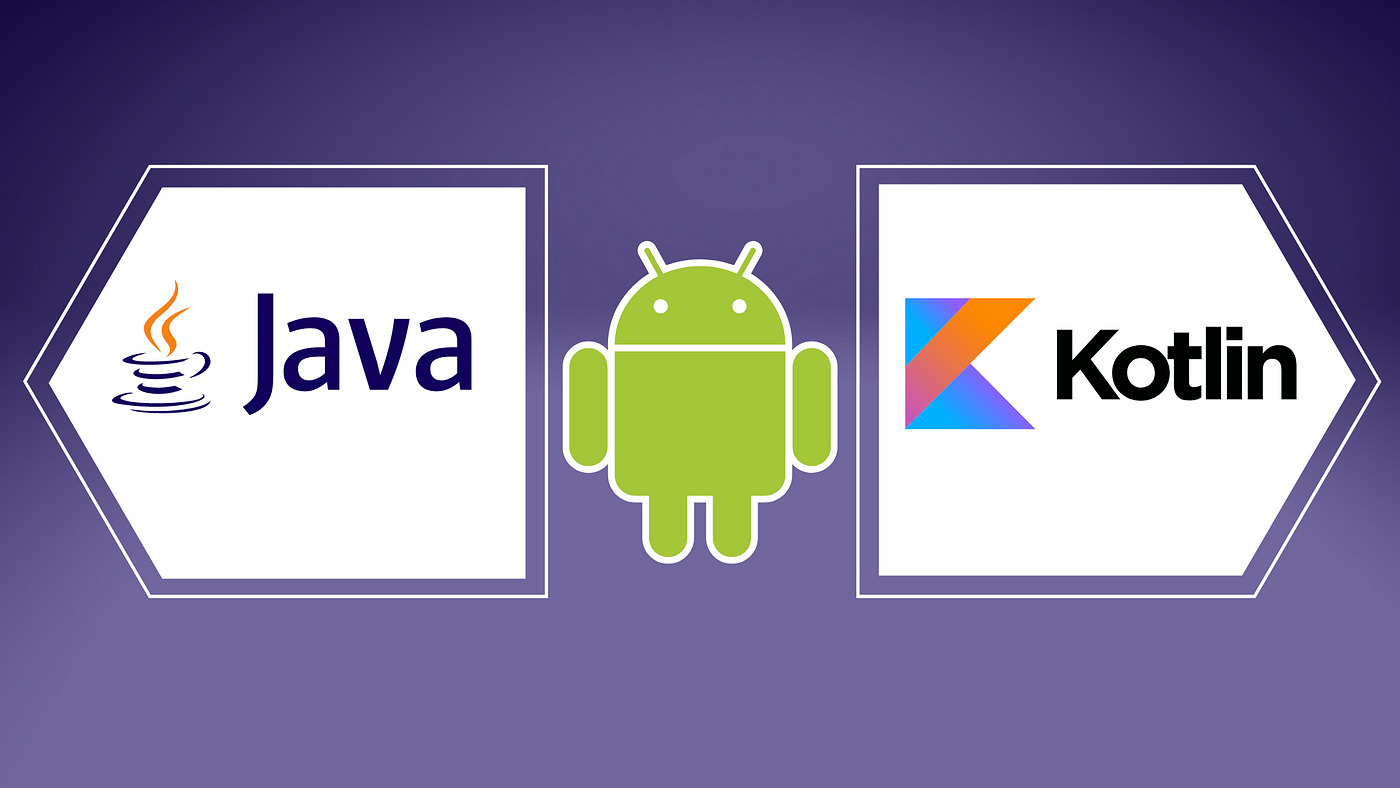
Introduction
Kotlin is a modern, statically typed programming language running on the Java Virtual Machine (JVM). Designed to be more concise and safe than Java, it offers features like null safety and extension functions. For Android developers, Kotlin provides a compelling alternative to Java for building applications.
Why Convert to Kotlin?
- Null Safety: Kotlin's null safety feature helps prevent NullPointerExceptions, common in Java.
- Conciseness: Kotlin code is often shorter and more expressive than its Java counterpart.
- Coroutines: Built-in support for coroutines makes writing asynchronous code easier.
- Interoperability: Seamlessly integrates with Java, allowing the use of existing Java libraries and frameworks.
Setting Up Your Environment
Before converting Java code to Kotlin, ensure that your Android Studio environment is set up correctly.
Installing Kotlin Plugin
- Open Android Studio: Launch Android Studio and go to
File
>Settings
(orPreferences
on macOS). - Plugins: Navigate to the
Plugins
section. - Marketplace: Click on the
Marketplace
button. - Search for Kotlin: Type "Kotlin" in the search bar and press Enter.
- Install Plugin: Select the "Kotlin" plugin and click
Install
.
Configuring Kotlin in Your Project
- Create a New Project: If you haven't already, create a new Android project.
- Choose Kotlin: During project creation, select "Kotlin" as the language for your project.
Converting Java to Kotlin
The process of converting Java to Kotlin involves understanding syntax differences and leveraging Kotlin-specific features.
Basic Syntax Differences
-
Variables and Data Types:
- Java: Declare variables using the
type variableName;
syntax. - Kotlin: Declare variables using
val
orvar
followed by the type and variable name.
kotlin
val name: String = "John Doe"
var age: Int = 30
- Java: Declare variables using the
-
Functions:
- Java: Declare functions using the
return
keyword. - Kotlin: Declare functions using the
fun
keyword. No explicit return type needed if the function returns nothing (Unit
).
kotlin
fun greet(name: String) {
println("Hello, $name!")
}
- Java: Declare functions using the
-
Classes and Objects:
- Java: Declare classes using the
class
keyword. - Kotlin: Declare classes using the
class
keyword with features like primary and secondary constructors.
kotlin
class Person(val name: String, val age: Int) {
fun greet() {
println("Hello, my name is $name and I am $age years old.")
}
}
- Java: Declare classes using the
-
Inheritance and Interfaces:
-
Java: Declare inheritance using the
extends
keyword. -
Kotlin: Declare inheritance using the
:
operator followed by the superclass name.
kotlin
open class Animal {
open fun sound() {}
}class Dog : Animal() {
override fun sound() {
println("Woof!")
}
}
-
-
Extension Functions:
- Java: Cannot extend functions.
- Kotlin: Extend functions using extension functions, adding functionality to an existing class without inheriting from it.
kotlin
fun String.upperCase() = this.toUpperCase()
println("Hello".upperCase()) // Output: HELLO
Common Issues During Conversion
-
Null Safety:
- Java: Common to encounter NullPointerExceptions due to unchecked references.
- Kotlin: Use the
?
operator to indicate a reference might be null or the?.
safe call operator to avoid exceptions.
kotlin
val person = Person("John Doe", 30)
println(person?.name) // Output: John Doe
println(person?.age) // Output: 30
-
Lambda Expressions:
- Java: Declare lambda expressions using the
->
operator. - Kotlin: Declare lambda expressions using curly braces
{}
. Use directly as function arguments or return values.
kotlin
val numbers = listOf(1, 2, 3, 4)
val sum = numbers.sumBy { it * it } // Output: 30
- Java: Declare lambda expressions using the
-
Data Classes:
- Kotlin: Provide a concise way to create classes that mainly hold data. Automatically generate implementations for
equals()
,hashCode()
, andtoString()
.
kotlin
data class Person(val name: String, val age: Int)
val person = Person("John Doe", 30)
println(person) // Output: Person(name=John Doe, age=30)
- Kotlin: Provide a concise way to create classes that mainly hold data. Automatically generate implementations for
-
Coroutines:
-
Kotlin: Allow writing asynchronous code that's easier to read and maintain than traditional callbacks or threads.
kotlin
import kotlinx.coroutines.*fun main() = runBlocking {
launch {
delay(1000)
println("World!")
}
println("Hello!")
}
-
Handling Specific Scenarios
Using RecyclerView with Kotlin
When working with RecyclerView in Kotlin, issues related to the apply
function or other Kotlin-specific features might arise.
Using apply
Function
The apply
function in Kotlin calls multiple functions on an object in a chain-like manner. Incorrect usage can cause confusion.
kotlin
// Incorrect usage:
RecyclerView.apply { this: RecyclerView!
var layoutManager = LinearLayoutManager(activity)
}
// Correct usage:
val recyclerView = RecyclerView(context)
recyclerView.apply {
layoutManager = LinearLayoutManager(context)
}
Understanding Auto-Import Suggestions
Auto-import suggestions related to gravity or gradle often result from misconfiguration or incomplete project setup.
- Check Dependencies: Ensure all necessary dependencies are included in your build.gradle file.
- Review Project Structure: Verify the project structure is correctly set up with necessary modules and configurations.
Handling Errors with apply
Function
Errors with the apply
function might stem from incorrect usage or missing imports.
- Check Syntax: Ensure syntax correctness and proper usage of the
apply
function. - Import Necessary Classes: Make sure necessary classes and functions are imported.
For example, if using RecyclerView and encountering issues with the apply
function, suggestions might look like:
kotlin
// Incorrect usage:
RecyclerView.apply { this: RecyclerView!
var layoutManager = LinearLayoutManager(activity)
}
// Correct usage:
val recyclerView = RecyclerView(context)
recyclerView.apply {
layoutManager = LinearLayoutManager(context)
}
Additional Tips
- Use Kotlin Extensions: Add functionality to existing classes without inheriting from them.
- Utilize Coroutines: Make asynchronous programming easier and more readable.
- Employ Data Classes: Provide a concise way to create classes that mainly hold data.
- Explore Jetpack Libraries: Jetpack libraries like Room for databases, LiveData for data binding, and Paging for efficient data loading are powerful tools that can enhance application performance and maintainability.
By understanding syntax differences and leveraging Kotlin-specific features, converting Java to Kotlin in Android Studio becomes a smooth process. This guide helps overcome common issues, enabling effective use of Kotlin for building robust and efficient Android applications.