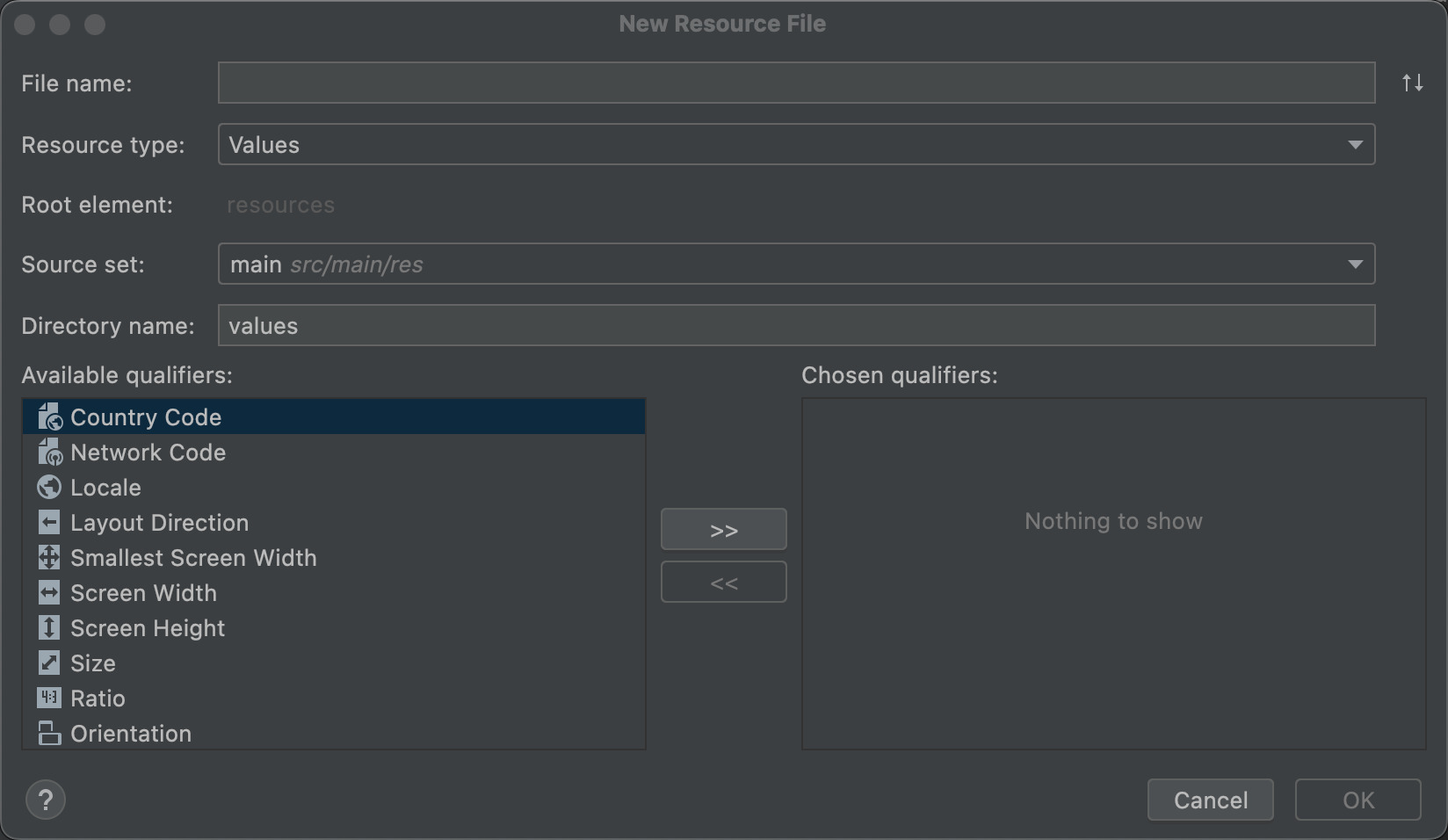
Source: Developer.android.com
Introduction to Android Studio
Android Studio, developed by Google, is the go-to Integrated Development Environment (IDE) for building Android applications. Since its release in 2013, it has become indispensable for Android developers, offering a robust set of tools that simplify the app development process.
Key Features of Android Studio
Integrated Development Environment (IDE)
- Provides a user-friendly interface with code completion, syntax highlighting, and project navigation.
Code Editor
- Highly customizable, supporting multiple programming languages like Java, Kotlin, and C++. Features include code completion, refactoring, and debugging.
Project Explorer
- Efficiently manage project structure by organizing files into logical folders like
lib
,assets
, andtest
.
Debugging Tools
- Advanced tools for identifying and fixing issues quickly, supporting both local and remote debugging.
Testing Frameworks
- Includes JUnit for unit testing and Espresso for UI testing, simplifying the process of writing and running tests.
Performance Profiler
- Analyzes app performance by identifying bottlenecks and optimizing resource usage.
Layout Editor
- Design user interfaces visually using drag-and-drop functionality, supporting various layouts like linear, relative, and constraint layouts.
Emulator
- Run and test apps on different Android versions without needing physical devices.
Gradle Build System
- Automates the build process by managing dependencies and configurations.
Version Control Integration
- Supports version control systems like Git, facilitating collaboration and change tracking.
Setting Up Android Studio
Download and Install
- Visit the official Android Studio website and download the latest version.
- Run the installer and follow prompts to complete installation.
Launch Android Studio
- Open Android Studio from the start menu or applications folder.
Create a New Project
- Upon first launch, create a new project by choosing an activity type (e.g., Empty Activity) and providing basic details like project name, package name, and location.
Configure SDK Tools
- After project creation, configure SDK tools by selecting the target Android version and required SDK components.
Set Up Virtual Devices
- Use the AVD manager to create virtual devices for testing apps on different Android versions and screen sizes.
Effective Usage Tips
Organizing Your Project Structure
- Logical Folders: Maintain a clean project structure by keeping files in logical folders like
lib
,assets
, andtest
. - Meaningful Names: Improve readability by using meaningful names for classes and methods.
- Utilizing Widgets Efficiently: Use stateless widgets for static content and stateful widgets for dynamic content.
Debugging and Testing
- Local Debugging: Identify issues quickly by setting breakpoints and inspecting variables.
- Remote Debugging: Debug apps running on physical devices or emulators connected over Wi-Fi.
- JUnit Testing: Ensure individual components function correctly by writing unit tests using JUnit.
- Espresso Testing: Write UI tests using Espresso to ensure the user interface behaves as expected.
Performance Optimization
- Performance Profiler: Analyze app performance by identifying bottlenecks and optimizing resource usage.
- Memory Profiler: Analyze memory usage and identify memory leaks.
- CPU Profiler: Analyze CPU usage and identify performance bottlenecks.
Designing User Interfaces
- Layout Editor: Design user interfaces visually using drag-and-drop functionality.
- Constraint Layout: Create complex layouts with ease by defining constraints between views.
- Material Design Guidelines: Follow material design guidelines to create visually appealing and consistent user interfaces.
Integrating with Other Tools
Integrating with Flutter
- Android Studio supports Flutter development through a plugin that integrates Flutter tools into the IDE, allowing developers to write, debug, and test Flutter apps.
Integrating with Version Control Systems
- Supports version control systems like Git, facilitating collaboration and change tracking.
Integrating with Third-Party Libraries
- Supports third-party libraries like Retrofit for networking and OkHttp for HTTP requests, simplifying common tasks and improving code quality.
Advanced Features
Code Completion and Refactoring
- Includes advanced code completion and refactoring features that help write clean and efficient code.
Code Inspection
- Features code inspection tools that help identify potential issues like null pointer exceptions or resource leaks.
Code Formatting
- Includes code formatting tools that help maintain consistent coding standards across projects.
Additional Resources
Official Documentation
- Google's official documentation is an excellent resource for learning more about Android Studio's features and functionalities.
Tutorials and Guides
- Numerous online tutorials and guides provide step-by-step instructions on using various features of Android Studio.
Community Forums
- Joining community forums like Stack Overflow or Reddit's r/AndroidDev community can provide valuable insights and solutions to common issues faced by developers.
Books and Courses
- Several books and courses cover advanced topics in Android development using Android Studio.