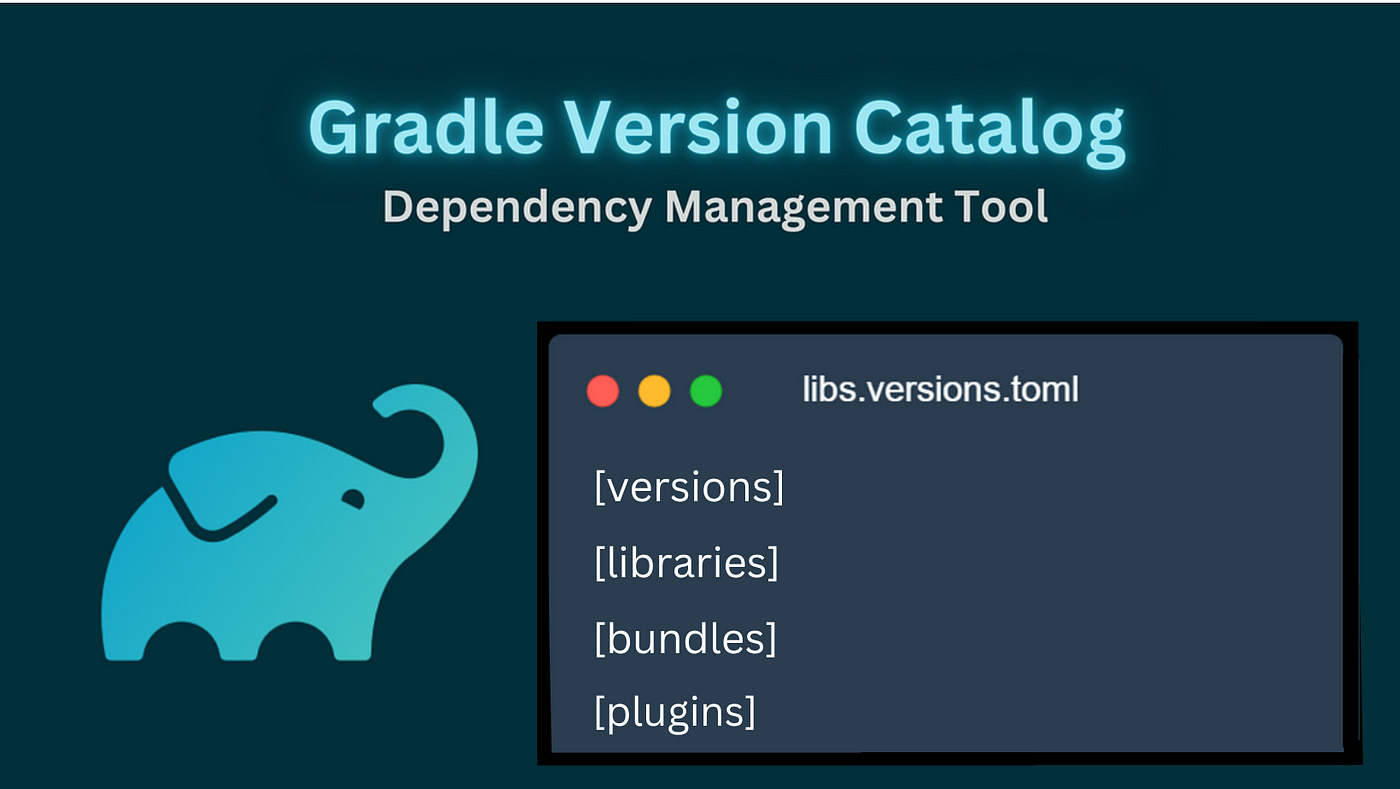
Introduction
Android Gradle is a crucial tool for Android app development, enabling developers to manage dependencies, build configurations, and automate various tasks. The Gradle build system has evolved significantly over the years, with new features and improvements being added regularly. This article explores the world of Android Gradle versions, covering their history, key features, and best practices for effective use.
History of Android Gradle
Early Days
Introduced in 2013 as part of Android Studio 0.4, the first version of Android Gradle aimed to simplify the build process by leveraging Gradle, a popular build tool for Java projects. Early versions focused on basic functionality such as managing dependencies and creating APKs.
Gradle 1.x Series
The Gradle 1.x series saw significant improvements in stability and performance. One notable feature introduced was the ability to use Groovy scripts for custom build logic, allowing developers to extend the build process with custom tasks and plugins.
Gradle 2.x Series
The Gradle 2.x series marked a major milestone with the introduction of multi-module projects. This feature enabled developers to manage multiple modules within a single project, simplifying the handling of complex applications with multiple components.
Gradle 3.x Series
The Gradle 3.x series brought substantial enhancements to the build system. One of the most significant additions was support for Kotlin as a build language, allowing developers to write build scripts in Kotlin, a modern, expressive language that integrates well with the Android ecosystem.
Gradle 4.x Series
The Gradle 4.x series continued to refine the build process with improved performance and new features. One notable addition was the introduction of the androidx
library, which replaced the traditional android-support
library. This change aimed to improve the modularization of Android components and reduce APK sizes.
Gradle 5.x Series
The Gradle 5.x series saw further improvements in performance and stability. One key feature introduced was the ability to use Java 8 language features in build scripts, allowing developers to leverage modern Java features like lambda expressions and method references in their build logic.
Gradle 6.x Series
The Gradle 6.x series brought significant changes with the introduction of Gradle 6.0. This version included major updates such as improved performance, enhanced security features, and better support for multi-module projects. Additionally, Gradle 6.0 introduced a new plugin system that simplifies managing dependencies and plugins.
Gradle 7.x Series
The latest series, Gradle 7.x, continues to build upon the foundation laid by its predecessors. One of the notable features introduced in Gradle 7 is improved support for Kotlin DSL (Domain Specific Language), allowing developers to write build scripts entirely in Kotlin, making integration with other Kotlin-based projects easier.
Key Features of Android Gradle
Dependency Management
Managing dependencies is one of the primary functions of Android Gradle. Dependencies are libraries or modules that your app relies on to function properly. Android Gradle allows you to declare these dependencies in your build.gradle
file and manage them effectively.
Declaring Dependencies
To declare a dependency, add it to the dependencies
block in your build.gradle
file. For example:
groovy
dependencies {
implementation 'com.example:library:1.0'
}
This will include the specified library in your app's build process.
Managing Dependencies
Android Gradle provides several ways to manage dependencies, including:
- Implementation: For libraries required by your app.
- Api: For libraries you want to expose to other modules in your project.
- Compile Only: For libraries needed only during the compilation phase.
- Runtime Only: For libraries needed only at runtime.
Build Variants and Flavors
Android Gradle allows you to create different build variants and flavors based on your app's requirements. Build variants are different configurations of your app that can be built separately, such as debug and release builds. Flavors are different versions of your app that can be built separately, such as free and paid versions.
Creating Build Variants
To create a build variant, add a productFlavors
block in your build.gradle
file. For example:
groovy
productFlavors {
free {
applicationId "com.example.free"
versionCode 1
versionName "1.0"
}
paid {
applicationId "com.example.paid"
versionCode 2
versionName "2.0"
}
}
This will create two build variants: free
and paid
.
Creating Flavors
To create a flavor, add a productFlavors
block in your build.gradle
file. For example:
groovy
productFlavors {
flavor1 {
applicationId "com.example.flavor1"
versionCode 1
versionName "1.0"
}
flavor2 {
applicationId "com.example.flavor2"
versionCode 2
versionName "2.0"
}
}
This will create two flavors: flavor1
and flavor2
.
Advanced Gradle Features
Android Gradle offers several advanced features that can enhance your build process and make it more efficient.
Kotlin and Groovy Support
Android Gradle supports both Kotlin and Groovy as build languages, allowing developers to write their build scripts in either language based on their preference.
Custom Build Logic
Developers can extend the build process with custom tasks and plugins by creating custom Gradle tasks or plugins using either Groovy or Kotlin.
Build Cache
Introduced in Gradle 4.x, the build cache significantly improves the performance of the build process by caching intermediate build results. This reduces the time it takes to build your app by reusing previously computed values.
Best Practices for Using Android Gradle
Version Control
Using version control systems like Git is essential for managing changes to your codebase effectively. This allows multiple developers to collaborate on the same project without conflicts.
Continuous Integration
Continuous integration (CI) is a practice where code changes are automatically built, tested, and validated every time a change is pushed to the repository. Tools like Jenkins, Travis CI, or CircleCI can be used for CI.
Code Organization
Organizing your code is crucial for maintaining readability and efficiency. Use folders to group related files together and avoid cluttering your project directory.
Debugging and Testing
Debugging and testing are critical steps in ensuring that your app functions correctly. Tools like Logcat and Espresso provide comprehensive debugging and testing capabilities.
Performance Monitoring
Performance monitoring is essential for ensuring that your app runs smoothly on different devices. Tools like the Android Profiler provide real-time performance monitoring, helping you identify bottlenecks in your app.
Final Thoughts
Android Gradle has come a long way since its introduction in 2013. From basic dependency management to advanced features like custom build logic and build caching, it has evolved to meet the needs of modern Android app development. By understanding the history, key features, and best practices of Android Gradle, developers can streamline their workflow and maintain high-quality code. Whether working on a simple app or a complex multi-module project, Android Gradle is an indispensable tool that can help achieve development goals efficiently.