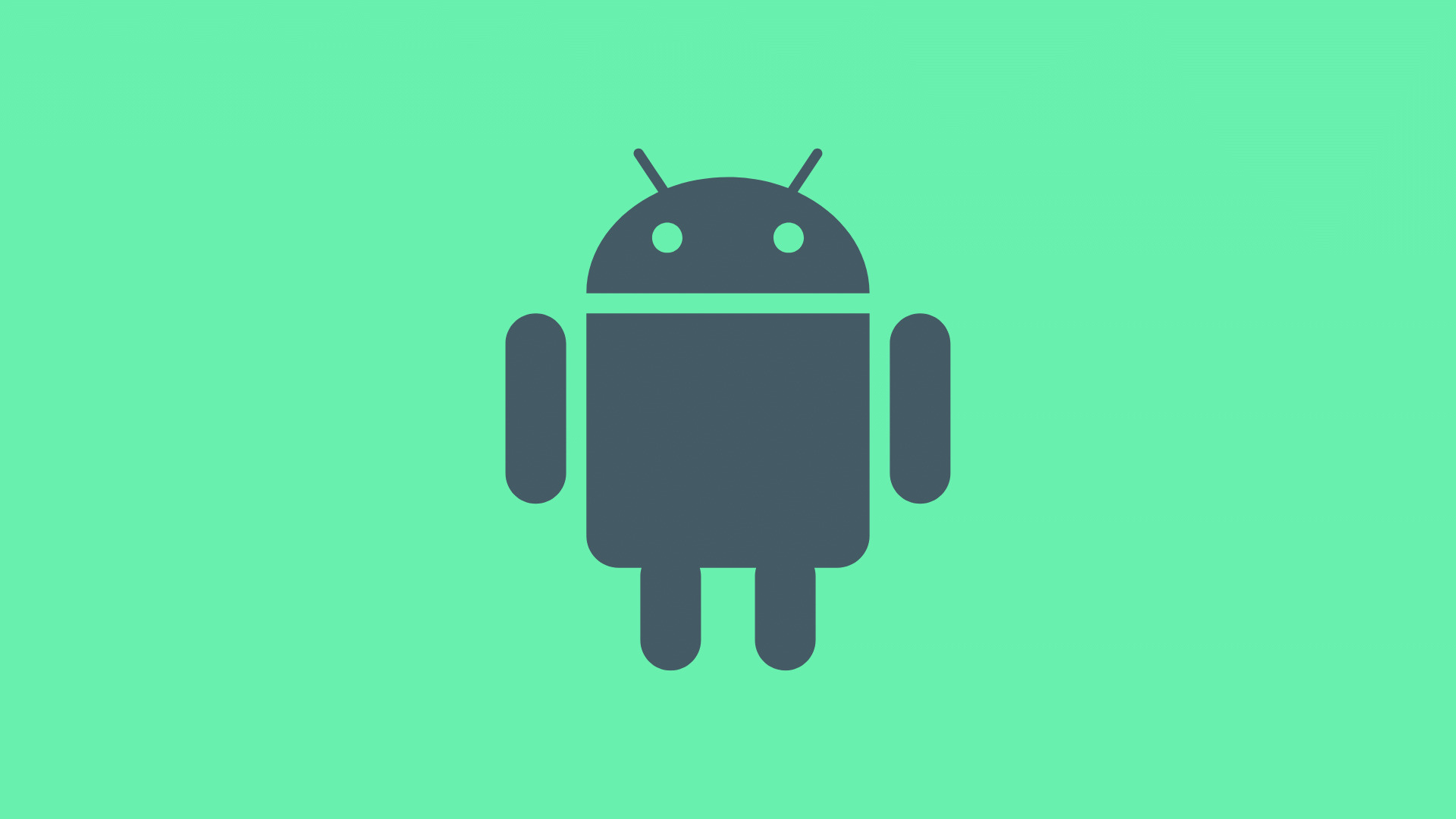
Introduction
Android development involves a multitude of tools and processes, one of the most critical being the Gradle build system. Gradle is a powerful tool for managing dependencies and building Android applications, but it can sometimes become a bottleneck due to its caching mechanisms. This article will explore Gradle caching, how to clear the cache, and various optimization techniques to improve build speed.
Understanding Gradle Cache
The Gradle cache is designed to speed up builds by storing outputs of previous builds and reusing them in subsequent builds. This approach significantly reduces the time required for building applications by avoiding the need to regenerate task outputs every time a build is executed.
Types of Gradle Cache
There are two primary types of Gradle caches: local and remote.
-
Local Cache: Stored on your machine, this is the default cache type used when running Gradle commands locally. The local cache directory is typically located in the
.gradle/caches
folder within your user home directory. -
Remote Cache: Stored on a remote server, this cache type is useful in multi-machine environments where developers and continuous integration agents need to share build outputs. The remote cache can be configured using a shared build cache.
How to Clear the Gradle Cache
Clearing the Gradle cache involves deleting cache files. Here are the steps to clear both local and remote caches:
Clearing Local Cache
- Locate the Cache Directory: The local cache directory is usually located in
.gradle/caches
within your user home directory. - Delete Cache Files: Navigate to the
.gradle/caches
directory and delete all files and subdirectories.
plaintext
rm -rf ~/.gradle/caches/
This command will delete the entire local cache directory, ensuring that all cached build outputs are removed.
Clearing Remote Cache
Clearing a remote cache involves more complex steps, as it typically requires administrative access to the server hosting the cache. Here are the general steps:
- Identify Remote Cache Location: Determine where the remote build cache is stored.
- Delete Cache Entries: Use the appropriate command or interface to delete cache entries from the remote server.
For example, if using a shared build cache with a CI tool like Jenkins, you might need to run a script or task that clears out the cache entries.
Enabling and Configuring the Build Cache
By default, the build cache is not enabled. Here are ways to enable it:
Enabling Build Cache via Command Line
Enable the build cache for a specific build by running the command with the --build-cache
flag.
plaintext
gradle –build-cache assemble
This command will use the build cache for the current build only.
Enabling Build Cache via gradle.properties
To enable the build cache for all builds, add the following line to your gradle.properties
file.
plaintext
org.gradle.caching=true
This setting will attempt to reuse outputs from previous builds for all subsequent builds unless explicitly disabled with the --no-build-cache
flag.
Task Output Caching
Task output caching leverages the same intelligence used in up-to-date checks to avoid redundant work. Instead of being limited to previous builds in the same workspace, task output caching allows Gradle to reuse task outputs from any earlier build on the local machine or even across different machines via a shared build cache.
Example of Task Output Caching
-
Initial Build: Run a build command without the build cache.
plaintext
gradle assemble -
Subsequent Build: Run the same build command with the build cache enabled.
plaintext
gradle –build-cache assemble
In the second run, Gradle will load task outputs from the cache instead of re-executing them, significantly speeding up the build process.
Configuration Cache
The configuration cache improves build speed by recording information about the build tasks graph and reusing it in subsequent builds. This feature is particularly useful when dealing with complex projects that involve multiple plugins and configurations.
Enabling the Configuration Cache
-
Check Plugin Compatibility: Ensure that all project plugins are compatible with the configuration cache. Use the Build Analyzer to check compatibility.
-
Add Configuration Cache Flag: Add the following line to your
gradle.properties
file.
plaintext
org.gradle.configuration-cache=true -
Handle Potential Issues: Be aware that some plugins might not be fully compatible with the configuration cache. If issues arise, set
org.gradle.configuration-cache.problems=warn
to get warnings instead of errors.
Optimization Techniques
Several optimization techniques can be employed to improve build speed in Android projects.
Disable PNG Crunching
PNG crunching is an optimization technique used by Android Gradle Plugin to compress PNG images. While it can save space, it can also slow down builds. If automatic image compression is not needed, disable PNG crunching for specific build types.
For example, in your build.gradle
file, add the following configuration for the release build type:
plaintext
android {
buildTypes {
release {
crunchPngs false
}
}
}
Experiment with JVM Parallel Garbage Collector
The JVM garbage collector used by Gradle can significantly impact build performance. While JDK 8 uses the parallel garbage collector by default, JDK 9 and higher use the G1 garbage collector. Test your Gradle builds with the parallel garbage collector by setting the following JVM arguments in your gradle.properties
file:
plaintext
org.gradle.jvmargs=-XX:+UseParallelGC
If there are other JVM arguments already set, add this new option:
plaintext
org.gradle.jvmargs=-Xmx1536m -XX:+UseParallelGC
Profile Your Build
To measure build speed with different configurations, use the Build Analyzer to profile your build. This tool helps identify bottlenecks and provides insights into how different configurations affect build performance.
Additional Tips
- Regularly Clean Up Cache: Periodically clean up the local cache to prevent it from growing too large and slowing down builds.
- Monitor Build Performance: Use tools like the Build Analyzer to monitor build performance and identify areas for further optimization.
- Test Different Configurations: Experiment with different configurations, such as JVM garbage collectors, to find the optimal settings for your project.
By following these guidelines and staying up-to-date with the latest Gradle features and best practices, you can ensure that your Android development workflow is efficient and productive.