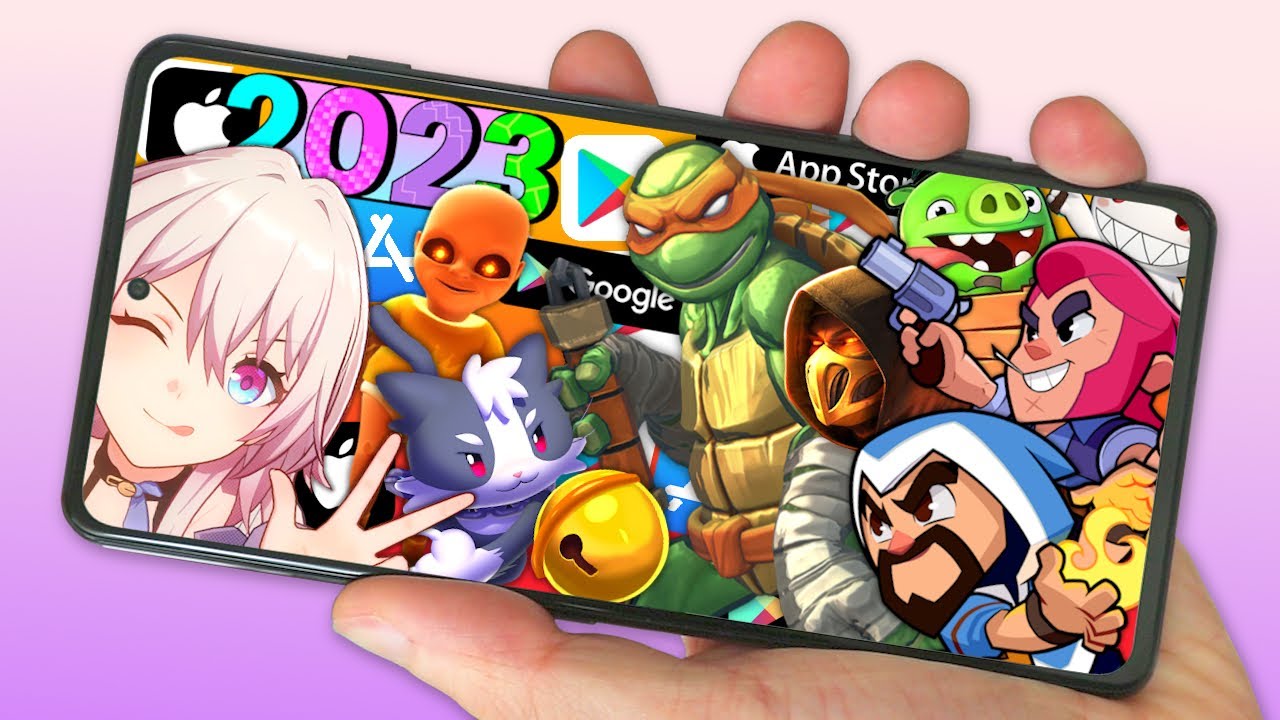
Introduction to Android Game Development
Android game development is a rapidly growing field, with millions of users worldwide eagerly awaiting new and exciting games. With the rise of mobile gaming, aspiring game developers are seeking ways to create their own Android games. This article will serve as a comprehensive guide, providing detailed tutorials and resources to help you get started on your journey towards creating engaging Android games.
Why Build Games for Android
Android offers several advantages for game developers:
- Large Audience: Android has a huge market share, giving developers access to a massive user base eager for new games.
- Easier Deployment: The Google Play Store has a simple and affordable process for uploading and launching games, making it more accessible to indie developers.
- Compatibility: Android games can be played on various devices, such as smartphones and tablets, expanding the potential audience for your game.
- Flexibility: Developers have more freedom to create unique experiences on Android, thanks to the variety of hardware available on the platform.
Key Differences Between Mobile and PC/Console Games
While mobile games share some similarities with PC and console games, there are essential differences to consider when developing for mobile platforms like Android:
- Touchscreen Controls: Mobile games rely on touchscreen input, which often requires simpler controls and UI elements designed for touch interactions.
- Form Factor: Mobile devices have different screen sizes, resolutions, and aspect ratios, requiring developers to ensure compatibility across multiple devices.
- Performance Limitations: Mobile devices generally have less processing power and memory than PCs or consoles, making it crucial to optimize game performance for smooth gameplay on phones and tablets.
- Monetization Strategies: Mobile games often use different monetization methods, such as in-app purchases, ads, and freemium models.
Key Takeaways:
- Creating Android games is exciting and rewarding, with tools like Android Studio and game engines like Unity making it easier for anyone to develop and publish their own games.
- Testing and optimizing your game for different devices is crucial to ensure it runs smoothly, and using strategies like in-app purchases or ads can help you make money from your game.
Steps to Create an Android Game
Creating an Android game involves several steps, including idea generation, game design, coding, testing, and deployment. Here’s a detailed breakdown of each step:
Step 1: Idea Generation
The first step in creating an Android game is to come up with an idea. This can be inspired by various sources such as personal experiences, popular trends, or even just a simple concept. For example, you might want to create a puzzle game, an action-adventure game, or even a strategy game.
Step 2: Game Design
Once you have your idea, it’s time to design your game. This involves creating detailed specifications about the gameplay mechanics, storyline (if applicable), and visuals. You should also consider the user interface (UI) and user experience (UX) to ensure that your game is both fun and easy to play.
Step 3: Choosing the Right Tools
To create an Android game, you need the right tools. While Android Studio is the official tool for Android development, many developers prefer using game engines like Unity or Unreal Engine. These engines provide a more beginner-friendly interface and pre-built physics and behavior systems.
Unity
Unity is one of the most popular game engines used for developing Android games. It supports both 2D and 3D game development and offers a wide range of features such as:
- Cross-platform support: Unity allows you to deploy your game on multiple platforms including Android, iOS, Windows, and more.
- Pre-built physics: Unity comes with built-in physics engines that make it easier to create realistic game mechanics.
- Visual scripting: Unity’s visual scripting system allows you to create game logic without writing code.
Unreal Engine
Unreal Engine is another powerful game engine that supports high-performance graphics and realistic simulations. It is particularly useful for creating visually stunning games but requires more technical expertise compared to Unity.
Godot
Godot is an open-source game engine that offers a lot of flexibility and customization options. It supports both 2D and 3D game development and has a growing community of developers who contribute to its development.
Step 4: Coding
After setting up your game engine, it’s time to start coding. This involves writing scripts in languages like C#, Java, or even Python depending on the engine you choose. Here’s a brief overview of how you might approach coding in Unity:
Creating a New Project in Unity
To create a new project in Unity:
- Download and Install Unity: You can download Unity from the official website.
- Create a New Project: Once installed, open Unity Hub and click on “New” to create a new project.
- Choose Project Settings: Select the project type (2D or 3D), location, and name for your project.
- Set Up Your Scene: Create your game scene by adding necessary components such as cameras, lights, and game objects.
Basic Coding Concepts
Here’s a simple example of how you might create a basic game using Unity:
- Create a Ball Game Object: Add a sphere game object to your scene.
- Add Script: Attach a script to the ball object that will handle its movement.
- Write Script Code: Use C# to write code that makes the ball move when touched by the player.
csharp
using UnityEngine;
public class BallController : MonoBehaviour
{
public float speed = 5f;
private Rigidbody rb;
void Start()
{
rb = GetComponent<Rigidbody>();
}
void FixedUpdate()
{
// Move the ball based on user input
float moveX = Input.GetAxis("Horizontal");
float moveZ = Input.GetAxis("Vertical");
Vector3 movement = new Vector3(moveX, 0f, moveZ);
rb.AddForce(movement * speed);
}
}
Step 5: Testing
Testing is crucial to ensure that your game works as intended and is free from bugs. You should test different scenarios such as different screen sizes, orientations, and hardware configurations.
Using Device Simulators
Unity provides device simulators that allow you to test your game on various devices without needing physical hardware.
Debugging Tools
Unity also comes with built-in debugging tools like the Unity Debugger and Profiler which help identify performance issues and debug code.
Step 6: Deployment
Once you're satisfied with your game's performance and functionality, it's time to deploy it on the Google Play Store.
Preparing Your Game for Release
Before uploading your game to the Google Play Store:
- Optimize Graphics: Use tools like the Android GPU Inspector (AGI) to analyze graphics performance and optimize assets.
- Test on Real Devices: Test your game on real devices to ensure compatibility and performance.
- Create Screenshots & Videos: Prepare high-quality screenshots and promotional videos for your game listing.
- Write Descriptions & Tags: Write detailed descriptions and tags for your game listing.
Uploading Your Game
To upload your game:
- Create a Developer Account: If you haven't already, create a developer account on the Google Play Console.
- Prepare APK File: Export your game as an APK file from Unity.
- Upload APK File: Upload the APK file to the Google Play Console.
- Fill Out Details: Fill out all required details including title, description, screenshots, and promotional videos.
Detailed Tutorials
Here are some detailed tutorials that can help you get started with Android game development:
Tutorial 1: Building a Simple Ball Shooting Game with Unity
This tutorial will guide you through creating a simple ball shooting game using Unity.
Step-by-Step Guide
- Install Unity: Download and install Unity from the official website.
- Set Up Android SDK & NDK: Install Android SDK and NDK from the Unity Hub.
- Create New Project: Create a new project in Unity and set up your scene.
- Add Ball Game Object: Add a sphere game object to your scene.
- Add Script: Attach a script to the ball object that will handle its movement.
- Write Script Code: Use C# to write code that makes the ball move when touched by the player.
Here’s a video tutorial that walks through this process in detail:
- Video Title: "How To Build An Android Game in 40 Minutes – Unity (2024 Working)"
- Video Link: Watch Here
Tutorial 2: Building a Complete Android Game Today with Unity
This tutorial will guide you through creating a complete Android game step-by-step using Unity.
Step-by-Step Guide
- Install Unity: Download and install Unity from the official website.
- Set Up Android SDK & NDK: Install Android SDK and NDK from the Unity Hub.
- Create New Project: Create a new project in Unity and set up your scene.
- Build 2D Block Dodging Game: Build a simple 2D block dodging game where you can move left or right to avoid blocks.
- Add Score System: Add a score system that updates as you dodge blocks successfully.
- Add Winning Message: Add a winning message that appears when you successfully dodge all blocks.
Here’s a video tutorial that walks through this process in detail:
- Video Title: "Build A Complete Android Game Today – Unity Android Tutorial 2023"
- Video Link: Watch Here
Tutorial 3: Building Multiple Android Games with Unity
This tutorial will guide you through building multiple Android games using Unity.
Step-by-Step Guide
- Install Unity: Download and install Unity from the official website.
- Set Up Android SDK & NDK: Install Android SDK and NDK from the Unity Hub.
- Create Multiple Projects: Create multiple projects in Unity each with different game mechanics.
- Build Carrot Collector Game: Build a carrot collector game where you move a rabbit character to collect carrots within boundaries.
- Build Balloon Popper Game: Build a balloon popper game where you pop balloons by tapping on them.
- Build Block Dodge Game: Build a block dodge game where you move left or right to avoid blocks.
Here’s a video tutorial that walks through this process in detail:
- Video Title: "Unity Android Game Development – Full Course"
- Video Link: Watch Here
Additional Resources
Here are some additional resources that can help you learn more about Android game development:
Game Engines
- Unity: Unity is one of the most popular game engines used for developing Android games. It supports both 2D and 3D game development and offers a wide range of features such as cross-platform support, pre-built physics, and visual scripting.
- Unreal Engine: Unreal Engine is another powerful game engine that supports high-performance graphics and realistic simulations. It is particularly useful for creating visually stunning games but requires more technical expertise compared to Unity.
- Godot: Godot is an open-source game engine that offers a lot of flexibility and customization options. It supports both 2D and 3D game development and has a growing community of developers who contribute to its development.
Programming Languages
- C#: C# is widely used in Unity for scripting game logic. It provides a lot of built-in functionality that makes it easier to create complex game mechanics.
- Java: Java is used in Android Studio for developing Android apps including games. It provides a lot of built-in functionality that makes it easier to create complex game mechanics.
- Python: Python can be used with various game engines like Pygame or Panda3D for creating 2D games.
Online Courses
- Unity By Example: This course provides 20+ mini projects that help you learn Unity by example. It covers various aspects of Unity including 2D and 3D game development.
- Build 5 Games in Unity & C#: This course helps you build five different games using Unity and C#. It covers various aspects of game development including game design, coding, testing, and deployment.
- Complete C# Scripting for Unity: This course provides comprehensive C# scripting tutorials for Unity. It covers various aspects of C# programming including object-oriented programming concepts, data structures, algorithms, and more.
Books
Here are some books that can help you learn more about Android game development:
- "Android Game Programming by Example": This book provides practical examples of how to create Android games using various tools and techniques.
- "Unity Game Development Essentials": This book provides comprehensive tutorials on how to create games using Unity.
- "Android Game Development Cookbook": This book provides recipes for solving common problems encountered during Android game development.
Creating an Android game involves several steps including idea generation, game design, coding, testing, and deployment. By following these steps and using the right tools like Unity or Unreal Engine, you can create engaging Android games that attract a large audience. Test your game thoroughly on different devices and optimize its performance using tools like the Android GPU Inspector (AGI). With practice and dedication, you can become proficient in Android game development and create games that stand out in the market.
Feature Overview
This feature offers step-by-step tutorials for creating Android games. It covers coding basics, game design principles, and optimization techniques. Users can access sample projects, interactive lessons, and community forums. The tutorials cater to beginners and advanced developers alike. Key functionalities include real-time code editing, debugging tools, and performance analytics.
What You Need and Compatibility
To ensure your device supports the feature, check these requirements and compatibility details:
- Operating System: Your device must run Android 8.0 (Oreo) or higher. Older versions won't support the latest features.
- Processor: A 64-bit processor is necessary. Devices with 32-bit processors might face performance issues.
- RAM: At least 3GB of RAM is required. More RAM ensures smoother performance.
- Storage: Ensure you have at least 2GB of free storage. This space is needed for installation and updates.
- Graphics: A GPU supporting OpenGL ES 3.0 or higher is essential for optimal graphics rendering.
- Screen Resolution: A minimum resolution of 1280x720 pixels is recommended. Higher resolutions provide better visual experiences.
- Internet Connection: A stable Wi-Fi or 4G connection is crucial for downloading and online features.
- Battery: Devices should have a battery capacity of at least 3000mAh. This ensures longer playtime without frequent charging.
- Sensors: Some features may require accelerometer and gyroscope sensors. Check your device specifications.
Ensure your device meets these criteria for the best experience. If unsure, consult your device's manual or settings to verify compatibility.
Getting Started
- Download Android Studio from the official website.
- Install Android Studio by following the on-screen instructions.
- Open Android Studio and click on "Start a new Android Studio project."
- Select "Empty Activity" and click "Next."
- Name your project and choose a save location.
- Set the language to Java or Kotlin.
- Click "Finish" to create your project.
- Wait for the project to load and sync.
- Open the "activity_main.xml" file in the "res/layout" folder.
- Design your game layout using the drag-and-drop interface.
- Switch to the "MainActivity.java" or "MainActivity.kt" file.
- Write your game logic in this file.
- Connect your Android device via USB or use an emulator.
- Click the green play button to run your game.
- Test your game on the device or emulator.
- Debug any issues that arise.
- Repeat steps 10-16 until satisfied with the game.
Tips for Effective Use
- Start Simple: Begin with basic tutorials. Master the fundamentals before diving into complex features.
- Use Official Resources: Google offers extensive documentation. Leverage these to understand best practices.
- Join Communities: Forums and groups like Reddit or Stack Overflow can provide valuable insights and solutions.
- Experiment: Create small projects to test new features. Hands-on practice solidifies learning.
- Stay Updated: Follow Android developer blogs. Updates often bring new tools and improvements.
- Optimize Performance: Focus on efficient coding. Laggy games frustrate users.
- Test on Multiple Devices: Ensure compatibility across various screen sizes and hardware.
- Seek Feedback: Share your game with friends or beta testers. Constructive criticism helps improve.
- Monetize Wisely: Integrate ads or in-app purchases without disrupting gameplay.
- Keep Learning: Technology evolves. Stay curious and keep enhancing your skills.
Troubleshooting Guide
Game Crashes Frequently:
- Solution: Update the game and your device's software. Clear the game's cache in settings. If the problem persists, reinstall the game.
Slow Performance:
- Solution: Close background apps to free up memory. Lower the game's graphics settings. Ensure your device has enough storage space.
Battery Drains Quickly:
- Solution: Reduce screen brightness. Turn off unnecessary features like Bluetooth and GPS. Use a battery saver mode if available.
Game Won't Download:
- Solution: Check your internet connection. Ensure you have enough storage space. Restart your device and try downloading again.
In-App Purchases Not Working:
- Solution: Verify payment methods in your account settings. Check if there are any restrictions on your account. Contact the game's support team if needed.
Game Freezes:
- Solution: Force stop the game in settings. Clear the game's cache. Restart your device and try again.
Audio Issues:
- Solution: Check the game's audio settings. Ensure your device's volume is up. Restart the game or device if necessary.
Connectivity Problems:
- Solution: Ensure a stable internet connection. Restart your router. Switch between Wi-Fi and mobile data to see if one works better.
Account Login Issues:
- Solution: Verify your login credentials. Reset your password if needed. Check if the game's servers are down.
Game Not Compatible:
- Solution: Check the game's requirements. Update your device's software. Consider using a different device that meets the game's specifications.
Privacy and Security Tips
When using this feature, user data is often collected to enhance the experience. To keep your information safe, always check the app's privacy policy. Make sure the app uses encryption to protect your data. Avoid sharing personal details unless absolutely necessary. Use strong passwords and enable two-factor authentication for added security. Regularly update your device and apps to patch any vulnerabilities. Be cautious of permissions requested by the app; only grant those that are essential. If possible, use a VPN to keep your online activities private.
Other Options and Comparisons
Pros:
Customization: Android games can be highly customized. Unlike iOS, Android allows more freedom in tweaking settings and features.
Variety: Android offers a wide range of devices from budget to high-end. This variety isn't as broad in systems like iOS or Windows.
Open Source: Android's open-source nature means more developers can contribute, leading to diverse game options. iOS is more closed off.
Google Play Store: Easy access to a vast library of games. Windows Store and Apple App Store have fewer options.
Cons:
Fragmentation: Android's wide range of devices can lead to compatibility issues. iOS, with fewer devices, faces this less often.
Security: Android is more prone to malware. iOS has stricter security measures.
Performance: High-end games may not run smoothly on all Android devices. iOS devices generally offer more consistent performance.
Alternatives:
iOS: Offers a more controlled environment with better security and performance consistency.
Windows: Good for PC gaming with robust hardware but fewer mobile game options.
Nintendo Switch: Great for exclusive games and portability but lacks the customization and variety of Android.
PlayStation/Xbox: Excellent for high-quality gaming but not portable like Android devices.
Android game development tutorials are a treasure trove for budding developers. They offer step-by-step guidance, making complex concepts easier to grasp. From basic coding to advanced graphics, these tutorials cover it all. They help you understand game engines like Unity and Unreal Engine, which are crucial for creating high-quality games. Plus, they often include practical examples, so you can see how theory translates into practice.
These tutorials also keep you updated with the latest trends and technologies in the gaming industry. They teach you how to optimize your games for better performance and user experience. By following these tutorials, you can build a strong foundation in game development and even create your own games from scratch.
In short, Android game development tutorials are an invaluable resource. They equip you with the skills and knowledge needed to succeed in the competitive world of game development.
Is Android game development worth it?
Rest assured, mobile game development will stay profitable in 2023 and beyond. According to Statista, the global app market grew to $420 billion in 2022 and is expected to hit $674 billion by 2027—an annual growth rate of 9.59%. The mobile game segment alone has a $155 billion market value.
Can you make money developing Android games?
Absolutely! A mobile game business model can drive revenue through one-time payments, in-app purchases (microtransactions), or ad monetization. Pick the strategy that fits your game best.
What programming language should I learn to make Android games?
For mobile-specific games, Java and Swift are great choices. Java is widely used for Android games, as it runs smoothly on Android devices. For iOS, Swift is the go-to language, designed by Apple for iOS with access to the latest frameworks.
How long does it take to develop an Android game?
Development time varies based on game complexity. Simple games might take a few months, while more complex ones could take a year or more. Planning, coding, testing, and polishing all add to the timeline.
Do I need a team to develop an Android game?
Not necessarily. Solo developers can create successful games, but having a team can speed up the process and bring diverse skills to the table. Artists, designers, and marketers can help make your game shine.
What tools do I need for Android game development?
Essential tools include Android Studio for coding, Unity or Unreal Engine for game design, and graphic design software like Photoshop or GIMP. These tools help streamline development and bring your game to life.
How do I market my Android game?
Marketing involves social media promotion, creating a website, and using app store optimization (ASO). Engaging with gaming communities and running ads can also boost visibility and downloads.