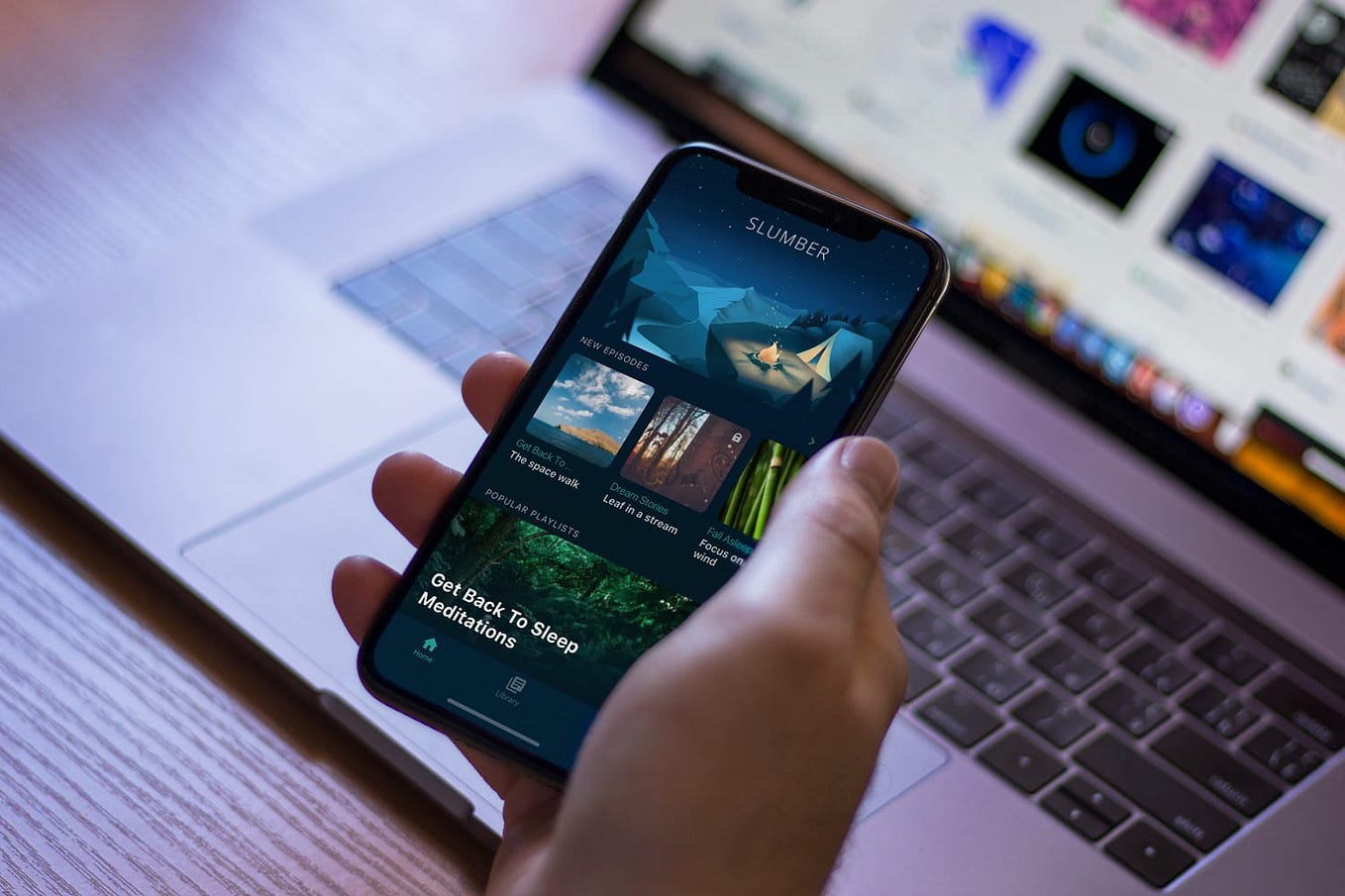
Introduction
In mobile apps, a search engine is a crucial feature that enhances user experience by allowing users to quickly find what they need. Whether it's searching for products in a shopping app or finding a specific article in a news app, a well-implemented search function can significantly improve an app's usability and overall appeal. This guide will walk you through building a search engine in Android Studio, covering prerequisites, key takeaways, and step-by-step instructions to set up your project and implement necessary functionalities.
Key Takeaways:
- Android game development is exciting and accessible, with popular game engines like Unity, Unreal, and Godot making it easier to create fun games for millions of players.
- Setting up tools like Android Studio and testing on real devices ensures your game runs smoothly, while publishing on Google Play Store lets you share your creation with the world.
Prerequisites
Before diving into building a search engine in Android Studio, ensure you have the following:
- Android Studio Installed: Download and install Android Studio from the official Android Developers website.
- Programming Skills: Basic understanding of Java or Kotlin is essential for building Android apps. Familiarity with XML is necessary for designing the user interface.
- Knowledge of Android Apps: Understanding how Android apps work is crucial for integrating the search engine into your app.
Key Takeaways
- Enhancing User Experience: Building a search engine in Android Studio makes your app more user-friendly by helping users find what they need quickly and easily.
- Adding Cool Features: Integrating features like voice search and caching can make your app faster and more enjoyable to use.
- Testing and Debugging: Thorough testing and debugging ensure everything works perfectly. This includes testing the search functionality under various conditions and debugging any issues that arise.
Setting Up Your Project
Creating a New Project
To start building your search engine, create a new project in Android Studio:
- Open Android Studio: Launch Android Studio on your computer.
- Start a New Project: Click on "Start a new Android Studio project."
- Choose a Template: Select an appropriate template that fits your needs. For this example, choose "Empty Activity."
- Name Your Project: Give your project a name, such as "SearchEngineApp."
- Choose Save Location: Choose a save location for your project.
- Set Language: Select the language you prefer (Java or Kotlin). For this example, we'll use Kotlin.
- Finish: Click "Finish," and Android Studio will set up your project.
Adding Necessary Dependencies
Once your project is set up, add some libraries to help with search functionality:
- Open build.gradle File: Open the
build.gradle
file in theapp
module. - Add Dependencies: Add the following dependencies to enable search functionality:
gradle
dependencies {
implementation 'androidx.appcompat:appcompat:1.2.0'
implementation 'androidx.recyclerview:recyclerview:1.1.0'
implementation 'androidx.cardview:cardview:1.0.0'
}
- Sync Project: Sync your project to download these libraries.
Designing the Layout
Next, design the layout for your search engine:
- Open activity_main.xml: Open
activity_main.xml
in the layout folder. - Add SearchView: Add a
SearchView
to allow users to input keywords. Example:
xml
<SearchView
android:id="@+id/searchView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:queryHint="Search..." />
<androidx.recyclerview.widget.RecyclerView
android:id="@+id/recyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
- Add RecyclerView: Add a
RecyclerView
to display the search results. In this example, we'll use it to display items.
Creating Data Model
Define a class for your data items:
- Create Data Model Class: Define a class for your data items, such as
Item
. Example:
kotlin
data class Item(val id: Int, val title: String, val description: String)
Implementing Search Functionality
With the layout and data model set up, implement the search functionality:
- Get SearchView Instance: Get an instance of the
SearchView
in your activity.
kotlin
private lateinit var searchView: SearchView
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
searchView = findViewById(R.id.searchView)
}
- Set OnQueryTextListener: Set an
OnQueryTextListener
to handle search queries.
kotlin
searchView.setOnQueryTextListener(object : SearchView.OnQueryTextListener {
override fun onQueryTextSubmit(query: String): Boolean {
// Handle search query submission
search(query)
return true
}
override fun onQueryTextChange(newText: String): Boolean {
// Handle search query change
return true
}
})
- Search Functionality: Implement the search functionality to retrieve relevant results based on the query.
kotlin
private fun search(query: String) {
// Simulate data retrieval (replace with actual data retrieval logic)
val items = listOf(
Item(1, "Item 1", "Description 1"),
Item(2, "Item 2", "Description 2"),
Item(3, "Item 3", "Description 3")
)
val filteredItems = items.filter { it.title.contains(query, ignoreCase = true) }
// Update RecyclerView with filtered items
recyclerView.adapter = ItemAdapter(filteredItems)
}
- ItemAdapter Class: Create an adapter class to display items in the RecyclerView.
kotlin
class ItemAdapter(private val items: List
override fun onCreateViewHolder(parent: ViewGroup, viewType: Int): ViewHolder {
val view = LayoutInflater.from(parent.context).inflate(R.layout.item_layout, parent, false)
return ViewHolder(view)
}
override fun onBindViewHolder(holder: ViewHolder, position: Int) {
holder.bind(items[position])
}
override fun getItemCount(): Int {
return items.size
}
inner class ViewHolder(itemView: View) : RecyclerView.ViewHolder(itemView) {
private val titleTextView = itemView.findViewById<TextView>(R.id.titleTextView)
private val descriptionTextView = itemView.findViewById<TextView>(R.id.descriptionTextView)
fun bind(item: Item) {
titleTextView.text = item.title
descriptionTextView.text = item.description
}
}
}
- Item Layout: Create an item layout to display each item in the RecyclerView.
xml
<TextView
android:id="@+id/titleTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="16sp"
android:textStyle="bold" />
<TextView
android:id="@+id/descriptionTextView"
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:textSize="14sp" />
Integrating Voice Search
To make your app more interactive, integrate voice search functionality using the SpeechRecognizer
class:
- Add SpeechRecognizer Dependency: Add the
SpeechRecognizer
dependency to yourbuild.gradle
file.
gradle
implementation 'com.google.android.gms:play-services-speech:20.0.0'
- Initialize SpeechRecognizer: Initialize the
SpeechRecognizer
in your activity.
kotlin
private lateinit var speechRecognizer: SpeechRecognizer
override fun onCreate(savedInstanceState: Bundle?) {
super.onCreate(savedInstanceState)
setContentView(R.layout.activity_main)
speechRecognizer = SpeechRecognizer.createSpeechRecognizer(this)
}
- Set OnActivityResultListener: Set an
OnActivityResultListener
to handle speech recognition results.
kotlin
speechRecognizer.setRecognitionListener(object : RecognitionListener() {
override fun onReadyForSpeech(params: Bundle?) {
// Speech recognition is ready to receive audio input.
}
override fun onBeginningOfSpeech() {
// Speech recognition has begun processing audio input.
}
override fun onRmsChanged(rmsdB: Float) {
// The RMS (Root Mean Square) value of the audio input.
}
override fun onEndOfSpeech() {
// Speech recognition has finished processing audio input.
}
override fun onError(errorCode: Int) {
// Error occurred while recognizing speech.
}
override fun onResults(results: Bundle?) {
// Speech recognition results are available.
val speechText = results?.getStringArrayList(SpeechRecognizer.RESULTS_RECOGNITION)
if (speechText != null) {
search(speechText)
}
}
override fun onPartialResults(partialResults: Bundle?) {
// Partial speech recognition results are available.
}
override fun onEvent(eventType: Int, params: Bundle?) {
// Event occurred while recognizing speech.
}
})
- Start SpeechRecognition: Start speech recognition when the user clicks a button.
kotlin
searchView.setOnQueryTextListener(object : SearchView.OnQueryTextListener {
override fun onQueryTextSubmit(query: String): Boolean {
// Handle search query submission
search(query)
return true
}
override fun onQueryTextChange(newText: String): Boolean {
// Handle search query change
return true
}
})
// Start speech recognition when the user clicks a button.
searchButton.setOnClickListener {
speechRecognizer.startListening(intent)
}
Caching Search Results
To improve performance and reduce network latency, cache search results using a caching library like OkHttp
:
- Add OkHttp Dependency: Add the
OkHttp
dependency to yourbuild.gradle
file.
gradle
implementation 'com.squareup.okhttp3:okhttp:4.9.3'
implementation 'com.squareup.okhttp3:logging-interceptor:4.9.3'
- Create Cache: Create a cache instance using
OkHttp
.
kotlin
private val cache = Cache(File(cacheDir, "http-cache"), 10 * 1024 * 1024)
- Create OkHttpClient: Create an instance of
OkHttpClient
with the cache.
kotlin
private val client = OkHttpClient.Builder()
.cache(cache)
.build()
- Use OkHttpClient: Use the
OkHttpClient
instance to make HTTP requests and cache responses.
kotlin
private fun search(query: String) {
val url = "https://example.com/search?q=$query"
val request = Request.Builder().url(url).build()
client.newCall(request).enqueue(object : Callback {
override fun onFailure(call: Call, e: IOException) {
// Handle failure
}
override fun onResponse(call: Call, response: Response) {
val body = response.body?.string()
if (body != null) {
val items = parseJson(body)
recyclerView.adapter = ItemAdapter(items)
}
}
})
}
Testing and Debugging
Thorough testing and debugging are essential to ensure that your search engine works perfectly under various conditions:
- Unit Testing: Write unit tests to test individual components of your search engine, such as the data model and adapter classes.
- UI Testing: Write UI tests to test the entire flow of the search engine, including user interactions and search results display.
- Debugging Tools: Use debugging tools like Logcat and Android Studio's built-in debugger to identify and fix issues.
Building a search engine in Android Studio is a fantastic way to improve your app's usability. From setting up the project to handling queries and optimizing performance, each step plays a pivotal role. With the right dependencies, XML configurations, and efficient data handling, your app can deliver swift and accurate search results. Integrating features like voice search and caching can make your app even better. Thorough testing and debugging ensure everything runs smoothly. Dive into the code and watch your app transform into a powerful tool that users will love.
Feature Overview
This feature simplifies game development on Android. It offers a user-friendly interface, pre-built templates, and drag-and-drop tools. Developers can integrate graphics, sound, and physics engines seamlessly. It supports multiplayer capabilities, cloud saving, and cross-platform compatibility. Real-time testing and debugging streamline the process. Performance optimization tools ensure games run smoothly.
Compatibility and Requirements
To ensure your device supports this feature, check the following requirements:
Operating System: Your device must run Android 8.0 (Oreo) or later. Older versions won't support the feature.
Processor: A 64-bit processor is necessary. Devices with 32-bit processors won't be compatible.
RAM: At least 4GB of RAM is required. Less memory might cause performance issues or prevent the feature from working.
Storage: Ensure you have at least 2GB of free storage space. This space is needed for installation and smooth operation.
Graphics: A GPU supporting OpenGL ES 3.1 or higher is essential. Lower versions won't render graphics properly.
Screen Resolution: A minimum screen resolution of 1280x720 pixels is needed. Lower resolutions might not display the feature correctly.
Battery: A device with a 3000mAh battery or higher is recommended. This ensures longer usage without frequent charging.
Internet Connection: A stable Wi-Fi or 4G connection is required for online features. Slower connections might cause lag or disconnections.
Permissions: Ensure your device allows access to storage, location, and camera. These permissions are crucial for full functionality.
Updates: Keep your device updated with the latest security patches and firmware. Outdated software might cause compatibility issues.
Check these details to confirm your device supports the feature.
How to Set Up
- Download the game engine from the official website.
- Install the software by running the downloaded file.
- Open the game engine after installation completes.
- Create a new project by selecting "New Project" from the main menu.
- Name your project and choose a location to save it.
- Select the template that fits your game type.
- Configure the project settings, such as resolution and platform.
- Import assets like images, sounds, and scripts into the project.
- Design your game levels using the built-in editor.
- Add game objects and set their properties.
- Script the game logic using the engine's scripting language.
- Test your game by clicking the "Play" button.
- Debug any issues that arise during testing.
- Build the final version of your game for Android.
- Export the APK file to your device.
- Install the APK on your Android device.
- Play your game and enjoy!
Effective Usage Tips
Optimize Performance: Ensure your game runs smoothly by reducing unnecessary background processes. Lower graphics settings if needed.
Test Frequently: Regularly test your game on different devices. This helps catch bugs early and ensures compatibility.
Use Tutorials: Many game engines offer tutorials. Follow them to understand features better and save time.
Community Forums: Join forums related to your game engine. Share experiences, ask questions, and learn from others.
Backup Regularly: Always backup your project files. This prevents loss of work due to unexpected issues.
Stay Updated: Keep your game engine and plugins updated. Updates often come with new features and bug fixes.
Asset Management: Organize your assets (images, sounds, etc.) in folders. This makes it easier to find and manage them.
Code Comments: Comment your code. This helps you and others understand what each part does, making future edits easier.
Prototype First: Create a prototype before developing the full game. This helps test ideas quickly without investing too much time.
User Feedback: Gather feedback from players. This provides insights into what works and what needs improvement.
Documentation: Read the documentation provided by the game engine. It often contains valuable information and tips.
Stay Consistent: Use consistent naming conventions for files and variables. This keeps your project organized and easy to navigate.
Performance Metrics: Monitor performance metrics like FPS (frames per second) to ensure your game runs efficiently.
Sound Design: Pay attention to sound. Good audio enhances the gaming experience significantly.
Plan Ahead: Outline your game’s design and features before starting development. This provides a clear roadmap to follow.
Troubleshooting Common Problems
Problem: Game Crashes Frequently
- Check for Updates: Ensure both the game and your device's software are up to date.
- Clear Cache: Go to Settings > Apps > [Game Name] > Storage > Clear Cache.
- Free Up Space: Delete unused apps or files to free up storage.
- Restart Device: Sometimes a simple restart can fix many issues.
- Reinstall Game: Uninstall and then reinstall the game.
Problem: Game Lags or Runs Slowly
- Close Background Apps: Shut down apps running in the background.
- Lower Graphics Settings: Adjust in-game settings to lower graphics quality.
- Free Up RAM: Use a device cleaner app to free up RAM.
- Check Internet Connection: Ensure a stable and fast internet connection.
- Restart Device: Restarting can help improve performance.
Problem: Game Won't Load
- Check Internet Connection: Make sure you have a stable connection.
- Clear Cache: Go to Settings > Apps > [Game Name] > Storage > Clear Cache.
- Check Server Status: Sometimes game servers are down; check the game's official website or social media.
- Update Game: Ensure the game is updated to the latest version.
- Restart Device: Restarting can sometimes resolve loading issues.
Problem: Game Audio Issues
- Check Volume Settings: Ensure your device volume is turned up and not muted.
- Check In-Game Settings: Verify audio settings within the game.
- Restart Game: Close and reopen the game.
- Update Game: Make sure the game is updated.
- Restart Device: Restarting can resolve audio glitches.
Problem: Game Controls Not Responding
- Check Touchscreen: Ensure your screen is clean and free of debris.
- Restart Game: Close and reopen the game.
- Update Game: Ensure the game is up to date.
- Check for Device Issues: Test the touchscreen with other apps to see if the issue persists.
- Reinstall Game: Uninstall and reinstall the game.
Problem: Game Not Connecting to Server
- Check Internet Connection: Ensure a stable and fast connection.
- Restart Router: Sometimes restarting your router can help.
- Check Server Status: Verify if the game servers are down.
- Update Game: Ensure the game is updated.
- Restart Device: Restarting can sometimes resolve connection issues.
Privacy and Security Tips
When using this feature, user data is often collected to enhance the experience. However, it's crucial to understand how this data is handled. Typically, data is encrypted during transmission to prevent unauthorized access. Stored data should also be encrypted and protected by strong passwords.
To maintain privacy, follow these tips:
- Regularly update your device and apps to patch security vulnerabilities.
- Use strong, unique passwords for your accounts.
- Enable two-factor authentication whenever possible.
- Review app permissions and only grant access to necessary features.
- Avoid public Wi-Fi for sensitive activities; use a VPN if needed.
- Clear cache and cookies regularly to remove stored data.
- Be cautious of phishing attempts and suspicious links.
By following these steps, you can safeguard your data and enjoy a more secure experience.
Comparing Alternatives
Pros:
- Customization: Android allows extensive customization. iOS offers limited customization.
- App Variety: Google Play Store has a wide range of apps. Apple App Store has a more curated selection.
- Hardware Choices: Many manufacturers produce Android devices. Apple only offers iPhones.
- Price Range: Android phones come in various price ranges. iPhones are generally more expensive.
- Expandable Storage: Many Android phones support microSD cards. iPhones do not have expandable storage.
Cons:
- Updates: Android updates can be slow due to manufacturer delays. iOS updates are timely and consistent.
- Security: Android is more susceptible to malware. iOS has a more secure ecosystem.
- App Quality: Some Android apps may be less optimized. iOS apps often have higher quality and better performance.
- Bloatware: Many Android phones come with pre-installed apps. iPhones have minimal pre-installed apps.
- Fragmentation: Android has many versions in use, causing inconsistency. iOS has fewer versions, leading to a more uniform experience.
Alternatives:
- Windows Phone: Offers a unique interface and integration with Microsoft services. Limited app availability.
- BlackBerry OS: Known for security features. Limited app support and fewer device options.
- KaiOS: Suitable for feature phones with basic smart capabilities. Limited to essential apps and services.
Android Game Engine Hub
Android game engines offer a wide range of tools for developers. Unity stands out for its versatility and large community. Unreal Engine provides stunning graphics but has a steeper learning curve. Godot is open-source and great for 2D games. Cocos2d-x is lightweight and perfect for mobile games. GameMaker Studio simplifies game development with its drag-and-drop interface. Each engine has its strengths, so the choice depends on your project's needs. Whether you're a beginner or an experienced developer, there's an engine that fits your skill level and goals. Dive in, experiment, and find the one that brings your game ideas to life.
What game engine can you use on Android?
Any game engine that supports cross-platform development can be used to develop Android games. Some of the best options include Unity, Unreal Engine, Godot Engine, Buildbox, and Solar2D.
Can I use Unreal Engine on Android?
Yes, you can use Unreal Engine on Android. To create and deploy an Android project, you'll need to install several Android development prerequisites included with Unreal Engine and ensure your device is ready for testing.
Is Unity available for Android?
Absolutely! To create a Unity game for Android, follow these steps: Download and install the Unity Hub, start the Unity Hub, and on the Installs tab, add a version of the Unity Editor that supports 64-bit apps.
What makes Godot Engine a good choice for Android game development?
Godot Engine is a great choice because it's open-source, lightweight, and has a user-friendly interface. It supports both 2D and 3D game development, making it versatile for various game types.
How does Buildbox simplify Android game development?
Buildbox simplifies Android game development by offering a drag-and-drop interface. You don't need to write any code, which makes it perfect for beginners or those who want to focus on game design rather than programming.
Can I develop Android games using Solar2D?
Yes, Solar2D (formerly known as Corona SDK) is a popular choice for developing Android games. It's a free, open-source framework that uses the Lua scripting language, making it easy to learn and use.
What are the benefits of using a cross-platform game engine for Android development?
Using a cross-platform game engine allows you to develop games for multiple platforms, not just Android. This means you can reach a wider audience without having to rewrite your game for each platform, saving time and resources.