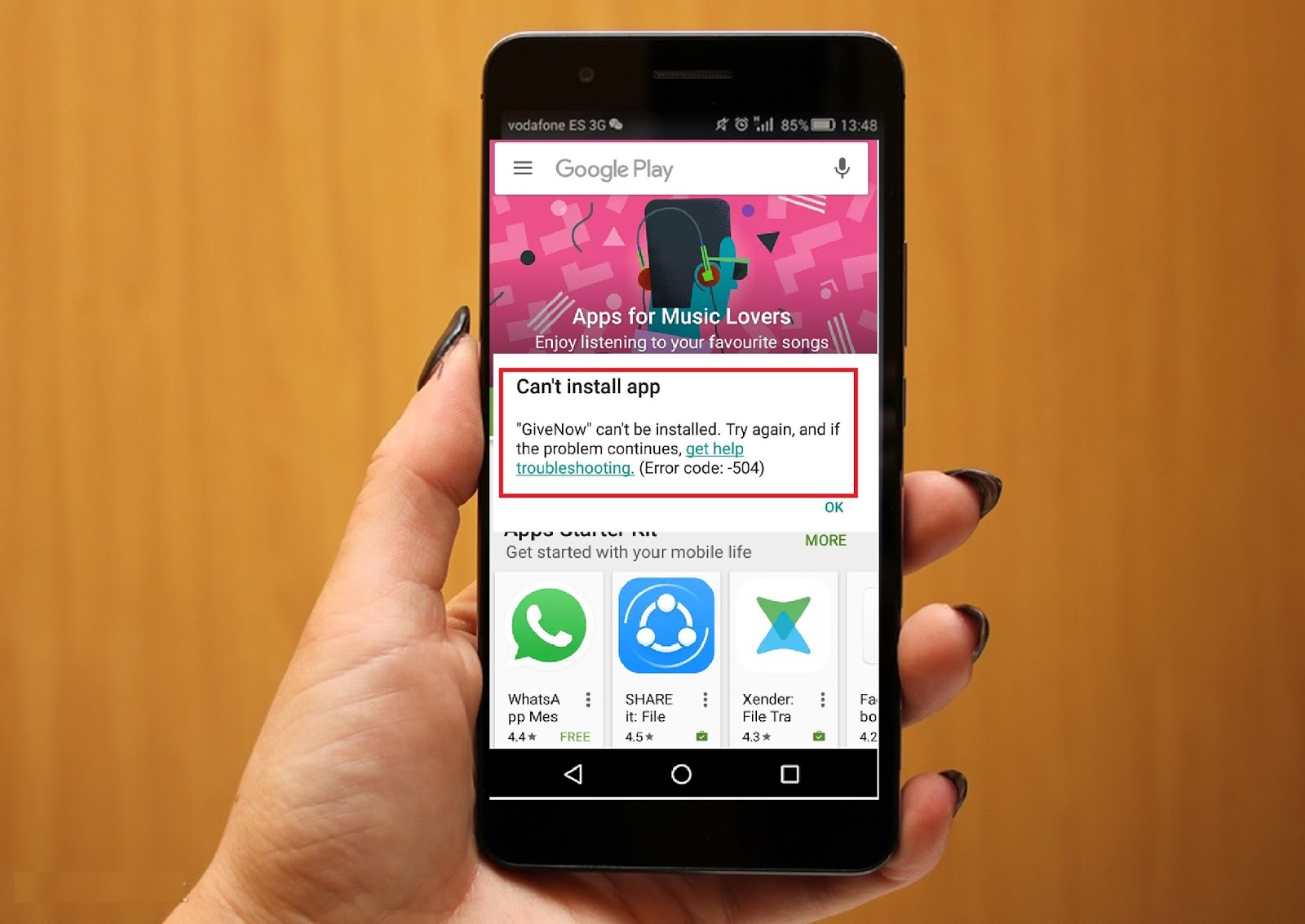
Android Errors: Troubleshooting and Fixes
Android devices, like any other technology, are not immune to errors and issues. These problems can range from simple app crashes to more complex system failures. Here, we will explore the most common Android errors, their causes, and provide detailed troubleshooting steps to resolve them.
1. ActivityNotFoundException
One of the most common errors encountered in Android development is the ActivityNotFoundException
. This exception occurs when a call to startActivity
fails because the specified activity cannot be found. This usually happens because the activity has not been declared in the AndroidManifest.xml file.
Cause
- Missing Activity Declaration: The activity is not declared in the AndroidManifest.xml file.
- Incorrect Activity Name: The activity name in the code does not match the one declared in the manifest.
Solution
-
Check AndroidManifest.xml: Ensure that the activity is declared in the AndroidManifest.xml file.
xml -
Correct Activity Name: Verify that the activity name in the code matches the one declared in the manifest.
-
Restart the App: After making changes to the manifest, restart the app to ensure the changes take effect.
2. ClassCastException
A ClassCastException
occurs when you try to cast an object to a subclass that it is not an instance of. This error is often caused by incorrect casting in your code.
Cause
- Incorrect Casting: You are trying to cast an object to a subclass that it is not an instance of.
- Missing Type Checks: You are not performing necessary type checks before casting.
Solution
-
Check Casting: Navigate to the section of your code indicated in the error message and check what object(s) are being cast.
java
Object obj = …;
if (obj instanceof YourClass) {
YourClass castObj = (YourClass) obj;
// Use castObj here
} -
Perform Type Checks: Always perform type checks before casting to avoid ClassCastException.
3. Error Converting Byte to Dex
The error "Error converting byte to dex" typically occurs during the build process when the Android SDK is unable to convert the .class files into .dex files. This can be caused by various factors including mismatched package names or incorrect build configurations.
Cause
- Mismatched Package Names: The package names in your classes do not match the package name in your project's Manifest.
- Incorrect Build Configurations: The build configurations are not set correctly, leading to issues during the build process.
Solution
-
Clean and Rebuild Project:
- Go to Build > Clean Project.
- Then, go to Build > Rebuild Project.
-
Check Package Names:
- Ensure that the package names in your classes match the package name in your project's Manifest.
-
Check Build Configurations:
- Verify that the build configurations are set correctly in your build.gradle file.
4. INSTALL_FAILED_INSUFFICIENT_STORAGE
This error occurs when the device does not have enough storage space to install the app. This can happen when using an Android Virtual Device (AVD) or a physical device with limited storage.
Cause
- Insufficient Storage Space: The device does not have enough free space to install the app.
Solution
-
Increase AVD Storage:
- If using an AVD, open the AVD Manager, find the AVD you want to use, click the Edit icon, and increase the storage space in the Memory and Storage section.
-
Free Up Device Storage:
- If using a physical device, free up some space by uninstalling unused apps or clearing out media files.
5. NullPointerException
A NullPointerException
occurs when you use a reference that points to no location in memory (null) as though you are referencing an object. This can happen when you try to call methods or access fields of a null object.
Cause
- Null Object Reference: You are trying to call methods or access fields of a null object.
Solution
-
Check for Null References:
- Ensure that the object is not null before calling methods or accessing fields.
java
if (obj != null) {
obj.method();
} else {
// Handle null case
}
- Ensure that the object is not null before calling methods or accessing fields.
-
Initialize Objects Properly:
- Initialize objects properly before using them.
6. Activity Has Leaked Window
This error occurs when an activity exits before a dialogue has been dismissed. This can cause memory leaks and other issues.
Cause
- Undismissed Dialogues: The activity exits without dismissing any dialogues.
Solution
-
Dismiss Dialogues Properly:
- Ensure that any dialogues are dismissed before exiting the activity.
java
@Override
protected void onDestroy() {
super.onDestroy();
if (dialog != null) {
dialog.dismiss();
}
}
- Ensure that any dialogues are dismissed before exiting the activity.
-
Use Dialogs Correctly:
- Use dialogues correctly and dismiss them when they are no longer needed.
Troubleshooting Steps for Common Issues
Restarting Your Device
One of the simplest yet most effective troubleshooting steps is restarting your device. This clears the memory and closes background apps, which can often resolve issues caused by bugs or temporary glitches.
- Press Power Button: Press and hold the power button until your phone restarts.
- Force Restart: If your phone is unresponsive, press and hold the power button and volume down button simultaneously for about seven seconds until it turns off and then back on.
Force Stopping Apps
Force stopping an app can help resolve issues related to unresponsive or malfunctioning apps.
- Open Settings App: Go to Settings > Apps.
- Search for App: Search for the unresponsive app.
- Force Stop: Tap the "Force Stop" button.
Clearing App Cache and Data
Clearing an app's cache and data can help resolve issues related to slow performance or app crashes.
- Open Settings App: Go to Settings > Apps.
- Search for App: Search for the problematic app.
- Clear Cache and Data: Tap "Clear Cache" and then "Clear Data."
Checking for System Updates
Regularly updating your Android device is crucial for ensuring it runs smoothly and securely.
- Open Settings App: Go to Settings > System > Advanced > System Update.
- Check for Updates: Tap "Check Now" or "Download and Install" if an update is available.
Managing App Permissions
Ensuring that apps have the necessary permissions can help resolve issues related to app functionality.
- Open Settings App: Go to Settings > Apps.
- Search for App: Search for the problematic app.
- App Info: Tap "App Info."
- Permissions: Check and adjust permissions as needed.
Resetting Network Settings
Resetting network settings can help resolve connectivity issues related to Wi-Fi, Bluetooth, or cellular networks.
- Open Settings App: Go to Settings > General Management > Reset > Reset Network Settings.
- Confirm Reset: Tap "Reset Settings."
Advanced Troubleshooting Techniques
Using Google Issue Tracker
If you encounter an issue that you believe is a bug or a feature request, you can report it using the Google Issue Tracker.
- Search for Existing Issues: Use the Issue Tracker's search field to see if anyone has already reported your issue or feature.
- Star Important Issues: If you find your issue and it's important to you, star it. The number of stars on an issue helps Google determine its priority.
- Create New Bug Report: If no one has reported your issue, create a new bug report.
- Fill Out Template: Fill out the provided template, including steps to reproduce the bug or explicit details on the proposed feature.
- Submit Report: Click Create to submit your report.
Common Android Errors Infographic
Here is an infographic summarizing some of the most common Android errors and their fixes:
-
ActivityNotFoundException:
- Cause: Missing activity declaration in AndroidManifest.xml.
- Solution: Declare activity in AndroidManifest.xml.
-
ClassCastException:
- Cause: Incorrect casting in code.
- Solution: Perform type checks before casting.
-
Error Converting Byte to Dex:
- Cause: Mismatched package names or incorrect build configurations.
- Solution: Clean and rebuild project, check package names.
-
INSTALL_FAILED_INSUFFICIENT_STORAGE:
- Cause: Insufficient storage space on device.
- Solution: Increase AVD storage or free up device storage.
-
NullPointerException:
- Cause: Null object reference in code.
- Solution: Check for null references before calling methods or accessing fields.
-
Activity Has Leaked Window:
- Cause: Undismissed dialogues when activity exits.
- Solution: Dismiss dialogues properly before exiting activity.
Android devices are powerful tools that can be prone to various errors and issues. By understanding the causes of these errors and following the detailed troubleshooting steps outlined in this article, you can effectively resolve common problems and ensure your device runs smoothly. Whether it's a simple app crash or a more complex system failure, knowing how to troubleshoot and fix these issues is crucial for any Android user or developer.