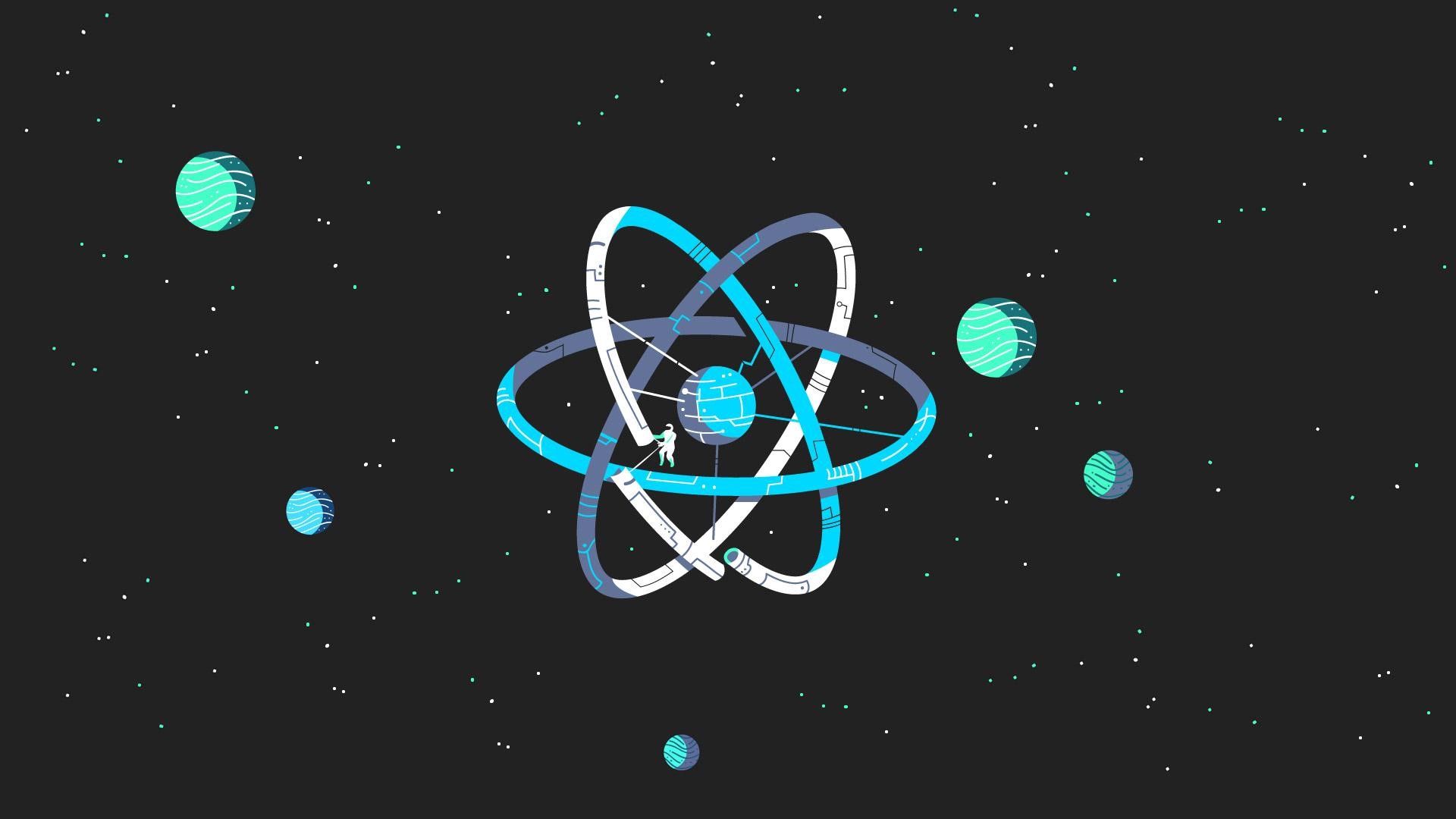
Mastering React Native Installation: A Comprehensive Guide
React Native is a popular framework for building cross-platform mobile applications using JavaScript and React. It allows developers to create apps for both Android and iOS using a single codebase, significantly reducing development time and costs. This guide covers the process of installing and setting up React Native on your machine, covering both Windows and Linux environments.
Prerequisites
Before beginning, ensure you have the following prerequisites:
- Node.js: Download from the official Node.js website.
- npm: Node Package Manager (npm) comes bundled with Node.js.
- Java Development Kit (JDK): Required for Android development.
- Android Studio: Helpful for debugging and testing your app.
- Text Editor/IDE: Use Visual Studio Code, IntelliJ IDEA, or Sublime Text for writing and managing code.
Step-by-Step Installation Guide
Step 1: Install Node.js and npm
- Download Node.js: Visit the official Node.js website and download the latest version for your operating system.
- Install Node.js: Follow the installation prompts. The installer will also install npm.
- Verify Installation: Open a terminal or command prompt and type
node -v
andnpm -v
to verify that Node.js and npm are installed correctly.
Step 2: Install React Native
-
Open Terminal/Command Prompt: Open your terminal or command prompt.
-
Install React Native CLI: Run the following command to install the React Native CLI globally:
sh
npm install -g react-native-cli -
Verify Installation: After installation, verify by running:
sh
react-native -v
Step 3: Set Up Your Development Environment
-
Create a New Project:
sh
npx react-native initReplace
<project-name>
with the name of your project. -
Navigate to Your Project Directory:
sh
cd -
Initialize Git Repository (Optional):
If you plan to use version control, initialize a Git repository:
sh
git add .
git commit -m "Initial commit"
Step 4: Configure Your Project
-
Open Your Project Directory:
Open your project directory in your preferred text editor or IDE. -
Understand Project Structure:
The project structure should look something like this:
plaintext
my-app/
├── android/
├── ios/
├── node_modules/
├── package.json
└── … -
Edit package.json:
Add scripts or dependencies inpackage.json
. For example, you might want to add a script for running your app:
json
"scripts": {
"start": "react-native start",
"test": "jest",
"android": "react-native run-android",
"ios": "react-native run-ios"
},
Step 5: Run Your App
-
Start Metro Bundler:
The Metro Bundler is required for development. Run:
sh
npx react-native startThis will start the Metro Bundler and make your app available at
http://localhost:8081/
. -
Run Your App on Android:
To run your app on an Android emulator or physical device, use:
sh
npx react-native run-android -
Run Your App on iOS:
To run your app on an iOS simulator or physical device, use:
sh
npx react-native run-ios
Troubleshooting Common Issues
Failed to Load JS Bundle
If you encounter an error like "Failed to load JS bundle," it might be due to issues with the Metro Bundler or network connectivity. Try restarting the Metro Bundler or checking your network connection.
Android SDK Missing
If you're getting an error related to missing Android SDK, ensure that you have JDK installed and that the path to JDK is correctly set in your environment variables.
npm Installation Issues
Sometimes, npm might encounter issues during installation. You can try cleaning the npm cache by running:
sh
npm cache clean –force
Then reinstall the package:
sh
npm install -g react-native-cli
Advanced Configuration
Customizing Build Configuration
Customize your build configuration by editing android/app/build.gradle
. For example, you might want to change the version code or add additional dependencies.
Using Third-Party Libraries
React Native supports a wide range of third-party libraries. Add them using npm or yarn. For example:
sh
npm install react-native-vector-icons
Then import them in your components:
javascript
import Icon from 'react-native-vector-icons/FontAwesome';
Mastering React Native installation is just the first step in building cross-platform mobile applications. With this guide, you should now have a solid foundation for setting up your development environment and running your first React Native app. Practice makes perfect; start building small projects and gradually move on to more complex ones as you gain experience.
Additional Resources
- Official React Native Documentation: The official documentation provides detailed guides on setting up your environment, building components, and debugging issues.
- React Native Community: The community is very active and provides numerous resources including tutorials, examples, and forums where you can ask questions.
- Stack Overflow: Stack Overflow has a dedicated section for React Native questions where you can find answers to common problems.
By following this guide and leveraging additional resources available online, you'll be well on your way to becoming proficient in React Native development. Happy coding
Understanding React Native Installation
This feature simplifies the process of installing React Native on Android devices. It automates the setup, ensuring all necessary tools and dependencies are in place. Users can quickly get started with their development projects without manual configurations. Key functionalities include automated SDK installation, environment setup, and compatibility checks. This ensures a smooth and efficient installation process, reducing potential errors and saving time.
Necessary Tools and Compatibility
To ensure your device supports React Native, check these requirements:
- Operating System: Your device must run Android 5.0 (Lollipop) or later. Older versions won't support the latest features.
- Processor: A 64-bit processor is necessary. Most modern devices have this, but double-check if using an older model.
- RAM: At least 2GB of RAM is required for smooth performance. More RAM means better multitasking and faster app execution.
- Storage: Ensure you have at least 500MB of free storage for the initial setup. Additional space will be needed for app data and updates.
- Screen Resolution: A minimum resolution of 720p (1280x720) is recommended. Higher resolutions offer better visual experiences.
- Development Environment: For development, use a computer with Node.js installed. Ensure you have Android Studio for emulation and testing.
- USB Debugging: Enable USB Debugging in your device's Developer Options. This allows your computer to communicate with the device for testing.
- Google Play Services: Ensure Google Play Services are up-to-date. Many apps rely on these for various functionalities.
Meeting these requirements ensures your device can handle React Native apps efficiently. If any criteria aren't met, consider upgrading your hardware or software.
Getting Started with React Native
Install Node.js: Download from the official Node.js website. Follow the installation prompts.
Install React Native CLI: Open your terminal. Type
npm install -g react-native-cli
and press Enter.Set Up Android Studio: Download Android Studio. Install it using the default settings. Open Android Studio, then go to Configure > SDK Manager. Ensure Android SDK, Android SDK Platform, and Android Virtual Device are checked.
Set Up Environment Variables:
- For Windows: Open System Properties > Environment Variables. Add a new variable named
ANDROID_HOME
with the path to your Android SDK. Add%ANDROID_HOME%\tools
and%ANDROID_HOME%\platform-tools
to the Path variable. - For Mac: Open your terminal. Type
nano ~/.bash_profile
. Addexport ANDROID_HOME=$HOME/Library/Android/sdk
andexport PATH=$PATH:$ANDROID_HOME/tools:$ANDROID_HOME/platform-tools
. Save and exit.
- For Windows: Open System Properties > Environment Variables. Add a new variable named
Create a New React Native Project: In your terminal, type
react-native init ProjectName
and press Enter.Run Your Project: Navigate to your project directory using
cd ProjectName
. Typereact-native run-android
to start your project on an Android emulator or connected device.Start Coding: Open your project in your favorite code editor. Begin building your app!
Maximizing React Native Usage
React Native makes building mobile apps a breeze. Here’s how to make the most of it:
- Start Small: Begin with simple components. Break down your app into smaller parts. This helps in managing code better.
- Use Expo: For quick prototyping, Expo is your friend. It simplifies setup and testing.
- State Management: Use Redux or Context API for handling state. This keeps your app organized.
- Styling: Stick to StyleSheet for consistent styling. Avoid inline styles for better performance.
- Testing: Implement Jest for unit tests. This ensures your components work as expected.
- Navigation: Use React Navigation for smooth transitions between screens.
- Performance: Optimize images and use FlatList for rendering large lists efficiently.
- Debugging: Use Reactotron or Flipper for debugging. They provide powerful tools to track down issues.
- Native Modules: When you need native functionality, create Native Modules. This bridges the gap between JavaScript and native code.
- Community: Leverage the React Native community. Many libraries and tools are available to solve common problems.
Keep learning and experimenting. React Native evolves quickly, so staying updated is key.
Troubleshooting React Native Problems
Problem: React Native installation fails on Windows.
Solution:
- Ensure Node.js is installed. Download from the official site.
- Install Chocolatey, a package manager for Windows.
- Use Chocolatey to install Python and JDK:
- Open Command Prompt as Administrator.
- Run
choco install -y python2 jdk8
.
- Install Android Studio. During setup, check "Android Virtual Device" and "Android SDK."
- Set up environment variables:
- Open System Properties > Environment Variables.
- Add
ANDROID_HOME
with the path to the Android SDK (e.g.,C:\Users\YourName\AppData\Local\Android\Sdk
). - Append
;%ANDROID_HOME%\tools;%ANDROID_HOME%\platform-tools
to thePath
variable.
- Install React Native CLI:
- Run
npm install -g react-native-cli
.
- Run
- Create a new project:
- Run
react-native init ProjectName
.
- Run
- Start the project:
- Navigate to the project folder.
- Run
react-native run-android
.
Problem: Emulator not starting.
Solution:
- Open Android Studio.
- Go to AVD Manager.
- Create a new virtual device.
- Select a device definition and system image.
- Ensure hardware acceleration is enabled:
- Open BIOS settings.
- Enable Intel VT-x or AMD-V.
- Start the emulator from AVD Manager.
Problem: Metro Bundler not running.
Solution:
- Ensure the project directory is correct.
- Open a new terminal.
- Navigate to the project folder.
- Run
npx react-native start
.
Problem: App crashes on launch.
Solution:
- Check for errors in the terminal.
- Ensure all dependencies are installed:
- Run
npm install
.
- Run
- Clear cache:
- Run
npm start --reset-cache
.
- Run
- Rebuild the project:
- Run
react-native run-android
.
- Run
Problem: Slow performance on emulator.
Solution:
- Allocate more RAM to the emulator:
- Open AVD Manager.
- Edit the virtual device.
- Increase RAM allocation.
- Use a physical device for testing:
- Enable Developer Options on the device.
- Turn on USB Debugging.
- Connect the device via USB.
- Run
react-native run-android
.
Problem: Unable to connect to development server.
Solution:
- Ensure the device and computer are on the same network.
- Check the IP address of the computer:
- Open Command Prompt.
- Run
ipconfig
.
- Update the IP address in the app:
- Open
App.js
. - Replace
localhost
with the computer's IP address.
- Open
- Restart the Metro Bundler:
- Run
npx react-native start
.
- Run
Security Tips for React Native
When using React Native on an Android website, security and privacy are crucial. User data should be encrypted both in transit and at rest. Always use HTTPS to protect data during transmission. Implement authentication and authorization to ensure only authorized users access sensitive information. Regularly update your dependencies to patch any vulnerabilities.
For maintaining privacy, avoid collecting unnecessary personal information. Use anonymous identifiers instead of real names or emails. Inform users about data collection practices through a clear privacy policy. Enable two-factor authentication for an added layer of security.
Permissions should be requested only when absolutely necessary. Regularly audit your codebase for potential security flaws. Use secure storage solutions for sensitive data. Educate users on the importance of strong passwords and safe browsing habits.
Comparing Other Frameworks
Pros of React Native:
- Cross-Platform Development: Write code once, run on both Android and iOS.
- Hot Reloading: See changes instantly without restarting the app.
- Large Community: Plenty of resources, libraries, and support available.
- Performance: Near-native performance for most applications.
Cons of React Native:
- Complex Native Modules: Sometimes need to write native code for specific functionalities.
- Performance Issues: Not as fast as fully native apps for complex tasks.
- Large App Size: Can result in larger app sizes compared to native apps.
Alternatives:
- Flutter: Also supports cross-platform development with a single codebase. Uses Dart language, offers fast performance, and has a rich set of widgets.
- Xamarin: Allows sharing code between Android, iOS, and Windows. Uses C#, integrates well with Microsoft tools.
- Ionic: Uses web technologies like HTML, CSS, and JavaScript. Good for web developers transitioning to mobile app development.
Comparison:
- Flutter vs. React Native: Flutter offers better performance and more customizable widgets but has a smaller community.
- Xamarin vs. React Native: Xamarin provides better integration with Microsoft services but may have a steeper learning curve.
- Ionic vs. React Native: Ionic is easier for web developers but may not offer the same performance as React Native.
Mastering React Native Installation
Mastering React Native installation on an Android website isn't as tough as it seems. Start by installing Node.js and npm. Then, install React Native CLI. Next, set up Android Studio and configure the Android SDK. Make sure to add the necessary environment variables. After that, create a new React Native project using the CLI. Connect your Android device or start an emulator. Finally, run the project using the react-native run-android
command.
Following these steps ensures a smooth setup process. You'll be ready to develop and test your React Native apps on Android. Remember, practice makes perfect. The more you work with these tools, the more comfortable you'll become. Happy coding!
How do I install React Native on my Android device?
First, make sure you have Node.js installed. Then, install React Native CLI by running npm install -g react-native-cli
. After that, set up Android Studio and its SDK. Finally, create a new project with react-native init ProjectName
.
What are the system requirements for installing React Native?
You'll need a computer with Windows, macOS, or Linux. Also, ensure you have Node.js, Watchman (for macOS), Java Development Kit (JDK), and Android Studio installed.
Do I need an Android device to test my React Native app?
Nope! You can use the Android Emulator that comes with Android Studio. Just set up a virtual device and run your app on it.
How do I connect my Android device to my computer for testing?
Enable Developer Options on your Android device, then turn on USB Debugging. Connect your device to your computer via USB, and run adb devices
to ensure it's recognized.
What should I do if my React Native app doesn't run on the emulator?
First, check if the emulator is running properly. Make sure the Android SDK is correctly installed. If problems persist, try running react-native run-android
from your project directory.
Can I use Expo instead of React Native CLI for Android development?
Yes, Expo is a great alternative. It simplifies the setup process. Install Expo CLI with npm install -g expo-cli
, then create a new project using expo init ProjectName
.
How do I update React Native to the latest version?
Update your project by running npm install --save react-native@latest
. Also, update your Android dependencies in android/build.gradle
and android/app/build.gradle
files.