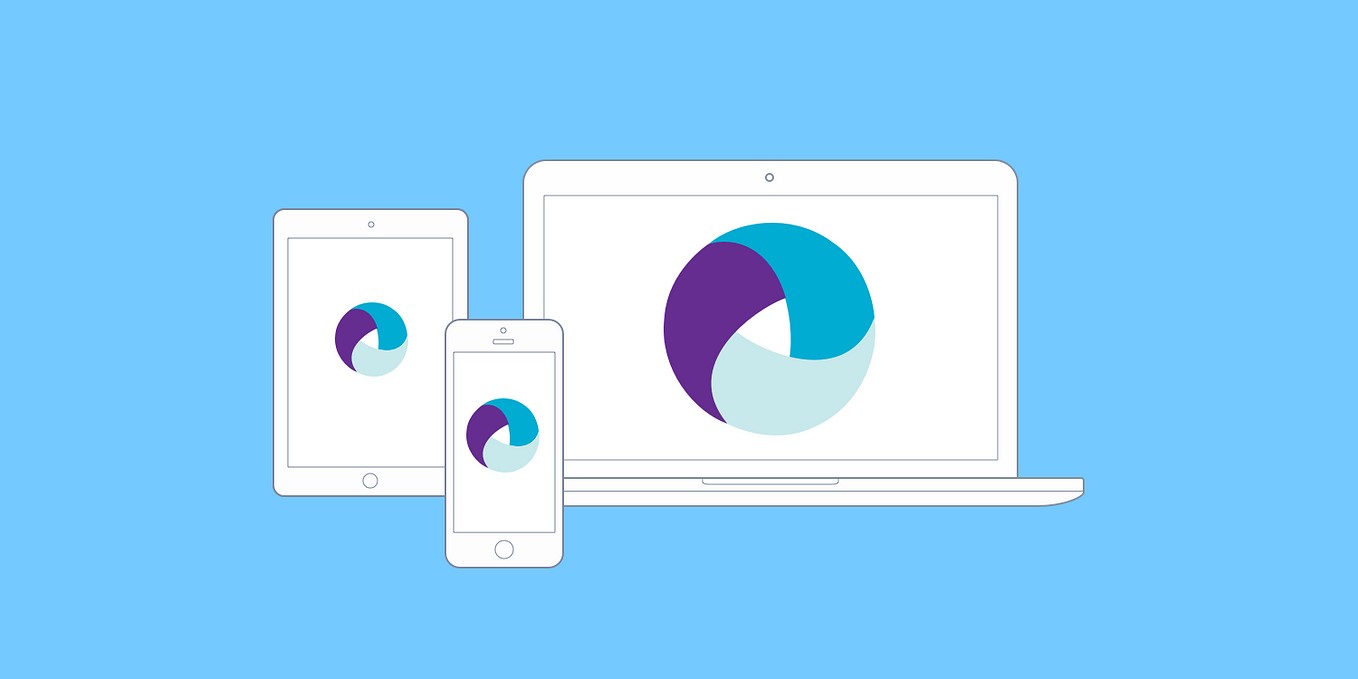
Introduction
In mobile automation testing, Appium has emerged as a powerful tool for automating both Android and iOS applications. While Appium primarily supports Android, it also provides the capability to test iOS applications using a simulator. This guide will walk you through setting up an iOS simulator with Appium, understanding key concepts, and providing a detailed step-by-step tutorial on automating an iOS application using Appium.
What is Appium?
Appium is an open-source tool for automating native, mobile web, and hybrid applications on iOS and Android platforms. It uses the WebDriver protocol, widely used in web automation testing, to interact with mobile applications. Appium supports both real devices and simulators, making it a versatile tool for mobile testing.
Setting Up the Environment
Before diving into the automation process, you need to set up your environment. Here are the steps to get started:
Install Node.js and npm
- Node.js is required to run Appium. Download it from the official Node.js website.
- npm (Node Package Manager) comes bundled with Node.js and is used to install dependencies.
Install Appium
-
Install Appium using npm by running the following command in your terminal:
sh
npm install -g appium -
Alternatively, download the Appium desktop application from the official website and follow the installation instructions.
Install Xcode (for iOS)
- To test iOS applications, you need Xcode installed on your Mac. Download it from the Mac App Store.
- Ensure that you have the Command Line Tools installed within Xcode.
Install iOS Simulator
- The iOS Simulator comes bundled with Xcode. Find it in the Applications folder after installing Xcode.
- Install additional simulators for different iOS versions by opening Xcode and navigating to
Window > Devices
.
Set Up Appium Server
-
Once you have Appium installed, start the server by running the following command in your terminal:
sh
appium -
This will start the Appium server on port 4723 by default.
Understanding Key Concepts
Before diving into automation, it's essential to understand some key concepts related to Appium and iOS simulators:
Appium Capabilities
Capabilities configure the behavior of Appium. For example, you can specify the platform name, platform version, and device name. Here is an example of how you might set up capabilities for an iOS simulator:
json
{
"platformName": "iOS",
"platformVersion": "14.2",
"deviceName": "iPhone 12",
"app": "/path/to/your/app.ipa"
}
Appium Drivers
Appium uses WebDriver drivers to interact with mobile applications. For iOS, use the XCUITest
driver. The XCUITest
driver is specific to iOS and allows you to interact with elements using XPath or other locators.
Simulator Management
Manage simulators using Xcode or through command-line tools like xcrun
. For example, list all available simulators using:
sh
xcrun simctl list
Automating an iOS Application with Appium
Now that you have your environment set up and understand the key concepts, let's dive into automating an iOS application using Appium.
Step 1: Create a New Project
First, create a new project using your preferred IDE (Integrated Development Environment). For this guide, we'll use Visual Studio Code (VS Code).
Install Required Extensions
- In VS Code, install the
Node.js
andnpm
extensions from the Extensions marketplace. - Install the
ESLint
extension for better code quality.
Create a New Folder
- Create a new folder for your project and navigate into it in your terminal.
Initialize Node.js Project
- Initialize a new Node.js project by running:
sh
npm init -y
Install Appium Client
- Install the Appium client using npm:
sh
npm install appium
Create Test File
- Create a new file named
test.js
in your project directory.
Step 2: Write Your First Test
Now it's time to write your first test using Appium. Here’s an example of how you might write a simple test to open an app and verify its title:
javascript
const { Builder, Capabilities } = require('selenium-webdriver');
const { By } = require('selenium-webdriver');
(async () => {
// Set up capabilities for iOS simulator
const capabilities = {
platformName: 'iOS',
platformVersion: '14.2',
deviceName: 'iPhone 12',
app: '/path/to/your/app.ipa'
};
// Create a new instance of the WebDriver
const driver = await new Builder().forBrowser('XCUITest').withCapabilities(capabilities).build();
try {
// Navigate to the app
await driver.getCapabilities();
// Wait for the app to launch and verify its title
await driver.wait(until.titleContains('Your App Title'), 10000);
console.log('App launched successfully!');
} catch (error) {
console.error('Error launching app:', error);
} finally {
// Close the driver instance
await driver.quit();
}
})();
Step 3: Run Your Test
To run your test, execute the following command in your terminal:
sh
node test.js
This will start the Appium server if it's not already running and execute your test script. You should see output indicating whether your test passed or failed.
Advanced Topics
Once you've mastered the basics, you can explore more advanced topics such as:
Element Identification
- Learn how to identify elements using various locators like XPath, CSS selectors, or UIAutomator.
- Use methods like
driver.findElement(By.xpath('your_xpath'))
ordriver.findElement(By.cssSelector('your_css_selector'))
.
Actions and Interactions
- Perform actions like tapping buttons, scrolling lists, or entering text into fields.
- Use methods like
driver.findElement(By.id('your_id')).click()
ordriver.findElement(By.name('your_name')).sendKeys('your_text')
.
Assertions
- Verify that elements are present or that certain conditions are met.
- Use assertions like
expect(driver.findElement(By.id('your_id')).getText()).toBe('your_expected_text')
.
Handling Alerts and Pop-ups
- Learn how to handle alerts and pop-ups that appear during your test execution.
- Use methods like
driver.switchTo().alert().accept()
ordriver.switchTo().alert().dismiss()
.
Handling Network Issues
- Understand how to handle network issues like slow internet connections or server timeouts.
- Use methods like
driver.manage().timeouts().implicitlyWait(10000)
to set an implicit wait time.
Parallel Testing
- Learn how to run multiple tests in parallel using tools like
appium-doctor
orappium-parallel
. - This can significantly speed up your test execution time.
Reporting and Logging
- Understand how to generate reports and logs for your tests using tools like Allure or JUnit.
- This helps in tracking test results and identifying failures more efficiently.
Additional Resources
For further learning, here are some additional resources you might find useful:
- Appium Documentation: The official Appium documentation provides detailed guides on setting up environments, writing test scripts, and troubleshooting common issues.
- Appium GitHub Repository: The Appium GitHub repository contains all the source code and issues related to Appium.
- Appium Community Forum: The Appium community forum is an excellent place to ask questions or share knowledge with other users.
- Mobile Automation Testing Books: Several books are available on mobile automation testing that cover topics like Appium, Selenium, and other tools in detail.
By leveraging these resources along with this guide, you'll be well-equipped to handle complex automation tasks involving iOS simulators using Appium.