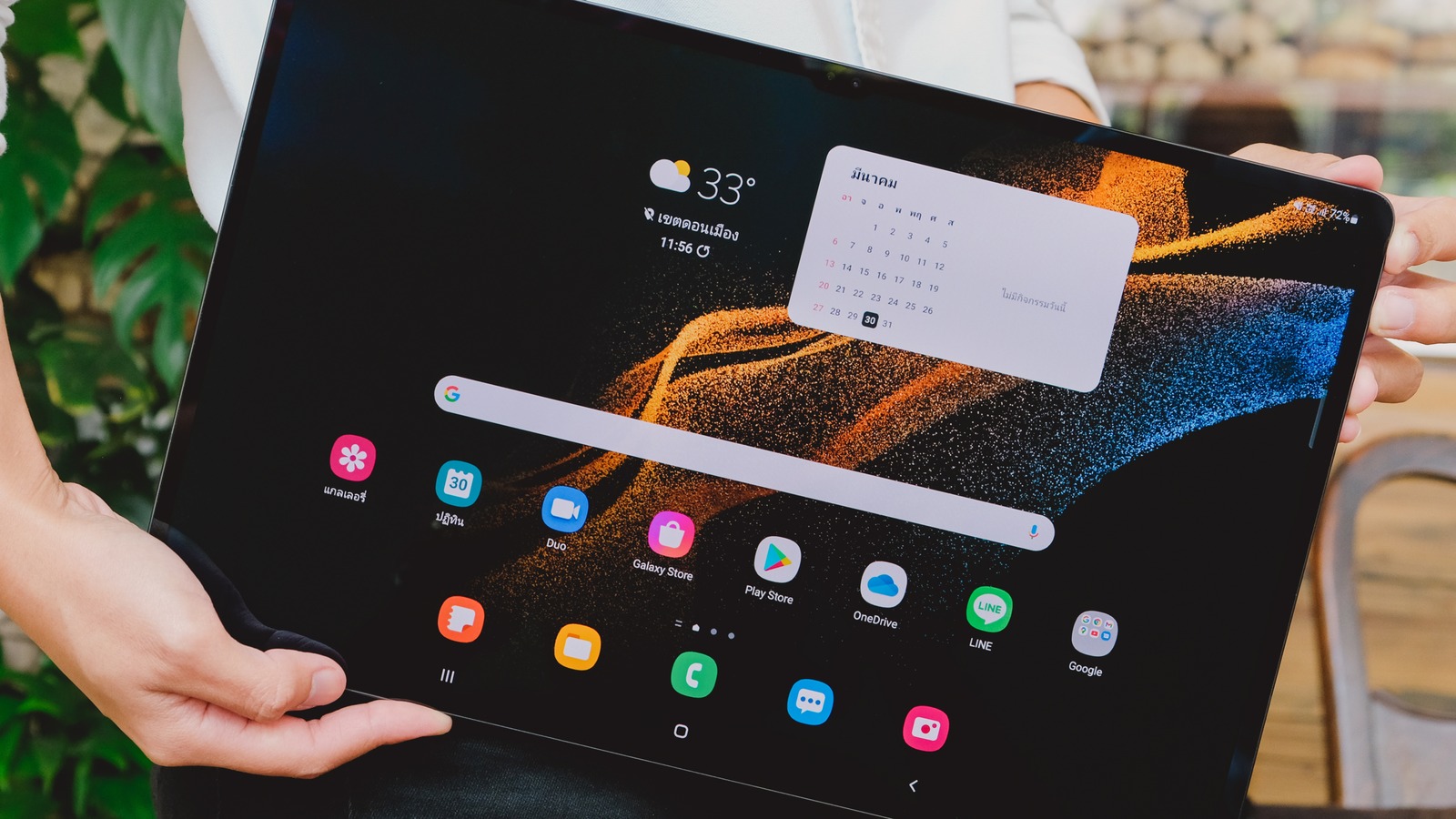
Introduction to Android Tablet App Development
Developing Android tablet apps offers an exciting and rewarding experience, especially with advancements in technology and the increasing demand for mobile applications. Whether you're a beginner or an experienced developer, this guide will walk you through the entire process of creating an Android tablet app, from planning and designing to testing and deployment.
Key Benefits of Developing Android Tablet Apps
- Larger Screen Real Estate: The primary advantage of developing for Android tablets is the larger screen size. This allows for more complex and visually appealing user interfaces, enhancing the overall user experience.
- Increased Productivity: With more space available, you can design apps that are more productive and efficient, such as document editors or project management tools.
- Enhanced User Experience: The larger screen provides ample room for multimedia content, making it perfect for apps like video players or educational resources.
- Cross-Platform Compatibility: Android apps can be easily ported to other Android devices, including smartphones and wearables, making it a versatile platform for development.
Setting Up Your Development Environment
Before starting the development of your Android tablet app, setting up the development environment is essential. This involves installing the necessary tools and software.
Installing Android Studio
Android Studio is the official integrated development environment (IDE) for Android app development. It provides a comprehensive set of tools to help design, develop, test, and debug apps.
- Download and Install Android Studio
- Visit the official Android Studio website and download the latest version.
- Follow the installation instructions to install Android Studio on your computer.
- Configure Your IDE
- Open Android Studio and configure it according to your preferences.
- Set up your project structure, including the project name, package name, and other essential details.
Setting Up Your Project
After configuring Android Studio, setting up your project structure is the next step. This involves creating the necessary folders and files for your app.
- Create a New Project
- In Android Studio, go to
File
>New
>New Project
. - Choose
Empty Activity
as the project type and clickNext
. - Enter your project details such as project name, package name, and language (Java or Kotlin).
- In Android Studio, go to
- Organize Your Project Structure
- Keep files organized by creating logical folders like
lib
,assets
, andtest
. - Use meaningful names for classes and methods to improve readability and maintainability.
- Keep files organized by creating logical folders like
Designing Your App
Designing your app is a crucial step in the development process. It involves creating a user interface that is both visually appealing and user-friendly.
Understanding Material Design
Material Design is Google's design language for Android apps. It provides guidelines for creating visually appealing and consistent user interfaces.
- Material Design Principles
- Color: Use a color palette that is consistent throughout the app.
- Typography: Use a clean and readable font.
- Imagery: Use high-quality images optimized for different screen sizes.
- Motion: Use animations to enhance the user experience.
- Creating a User Interface
- Use the
activity_main.xml
file to design your user interface. - Add widgets such as
TextView
,Button
, andImageView
to create a basic layout. - Use layout managers like
LinearLayout
orConstraintLayout
to arrange widgets.
- Use the
- Customizing Your UI
- Use themes and styles to customize the look and feel of your app.
- Add custom views or widgets to create a unique user interface.
Writing Code
Writing code is the core of app development. It involves creating logic that interacts with the user interface and performs tasks.
Understanding Java or Kotlin
Android apps can be developed using either Java or Kotlin. Both languages have their own strengths and weaknesses.
- Java
- Java is a mature language with a large community of developers.
- It is widely used in Android app development and provides a robust set of libraries and tools.
- Kotlin
- Kotlin is a modern language designed to be more concise and safe than Java.
- It is fully interoperable with Java and can be used alongside it in the same project.
Creating Activities
Activities are the core components of an Android app. They represent a single screen with a user interface.
- Creating an Activity
- Create a new class that extends
Activity
. - Override the
onCreate
method to set up your user interface. - Use the
setContentView
method to set the layout for your activity.
- Create a new class that extends
- Handling User Input
- Use
Button
widgets to handle user input. - Override the
onClick
method to perform actions when a button is clicked.
- Use
- Interacting with the UI
- Use
TextView
widgets to display text. - Use
ImageView
widgets to display images.
- Use
Using Fragments
Fragments are reusable pieces of code that can be used to create complex user interfaces.
- Creating a Fragment
- Create a new class that extends
Fragment
. - Override the
onCreateView
method to set up your user interface.
- Create a new class that extends
- Using Fragments in Activities
- Add a
FragmentContainerView
to your activity layout. - Use the
FragmentManager
to add and remove fragments dynamically.
- Add a
Testing Your App
Testing is a crucial step in the development process. It involves ensuring that your app works as expected and is free from bugs.
Unit Testing
Unit testing involves testing individual units of code to ensure they work correctly.
- Creating Unit Tests
- Use the JUnit framework to create unit tests.
- Write test cases for individual methods or classes.
- Running Unit Tests
- Use the
Run
menu in Android Studio to run unit tests. - Use the
Test
tab to view the results of your unit tests.
- Use the
UI Testing
UI testing involves testing the user interface to ensure it works correctly.
- Creating UI Tests
- Use the Espresso framework to create UI tests.
- Write test cases for individual UI elements such as buttons and text views.
- Running UI Tests
- Use the
Run
menu in Android Studio to run UI tests. - Use the
Test
tab to view the results of your UI tests.
- Use the
Debugging Your App
Debugging involves identifying and fixing bugs in your app.
Using Debugging Tools
Android Studio provides a range of debugging tools to help identify and fix bugs.
- Using Logcat
- Use Logcat to view logs and identify issues.
- Add log statements to your code to track the execution flow.
- Using Breakpoints
- Use breakpoints to pause the execution of your code and inspect variables.
- Use the
Debug
tab in Android Studio to set and manage breakpoints.
- Using the Debugger
- Use the debugger to step through your code line by line.
- Use the
Debug
tab in Android Studio to manage the debugger.
Optimizing Performance
Optimizing performance involves ensuring that your app runs smoothly and efficiently.
Avoiding Memory Leaks
Memory leaks occur when objects are not properly released, causing the app to consume more memory than necessary.
- Using Weak References
- Use weak references to avoid memory leaks.
- Use the
WeakReference
class to create weak references to objects.
- Releasing Resources
- Release resources such as bitmaps and databases when they are no longer needed.
- Use the
recycle
method to recycle bitmaps and free up memory.
Using Profilers
Profiling involves analyzing the performance of your app to identify bottlenecks.
- Using the CPU Profiler
- Use the CPU profiler to analyze the CPU usage of your app.
- Identify methods that consume the most CPU time and optimize them accordingly.
- Using the Memory Profiler
- Use the memory profiler to analyze the memory usage of your app.
- Identify objects that consume the most memory and optimize them accordingly.
Deploying Your App
Deploying your app involves publishing it to the Google Play Store or other app stores.
Preparing Your App for Deployment
Before deploying your app, ensure that it meets the requirements of the app store.
- Checking Compatibility
- Check the compatibility of your app with different devices and screen sizes.
- Use the
lint
tool to check for any issues with your code.
- Optimizing Graphics
- Optimize graphics to reduce the size of your app.
- Use the
pngcrush
tool to compress PNG files.
- Testing on Different Devices
- Test your app on different devices to ensure it works correctly.
- Use the
Android Debug Bridge
(ADB) to test your app on different devices.
Publishing Your App
Once you have prepared your app for deployment, you can publish it to the Google Play Store.
- Creating a Developer Account
- Create a developer account on the Google Play Console.
- Pay the required fees to publish your app.
- Uploading Your App
- Upload your app to the Google Play Console.
- Fill in the required details such as title, description, and screenshots.
- Setting Up Pricing and Distribution
- Set up pricing and distribution options for your app.
- Choose the countries where you want to distribute your app.
- Publishing Your App
- Once you have filled in all the required details, click the
Publish
button to publish your app.
- Once you have filled in all the required details, click the
Developing Android tablet apps is a rewarding experience that requires careful planning, designing, testing, and deployment. By following this guide, you can create high-quality apps that run smoothly and efficiently on Android tablets. Stay updated with the latest tools and technologies, and always test your app thoroughly before publishing it to ensure it meets the expectations of your users. Happy coding!