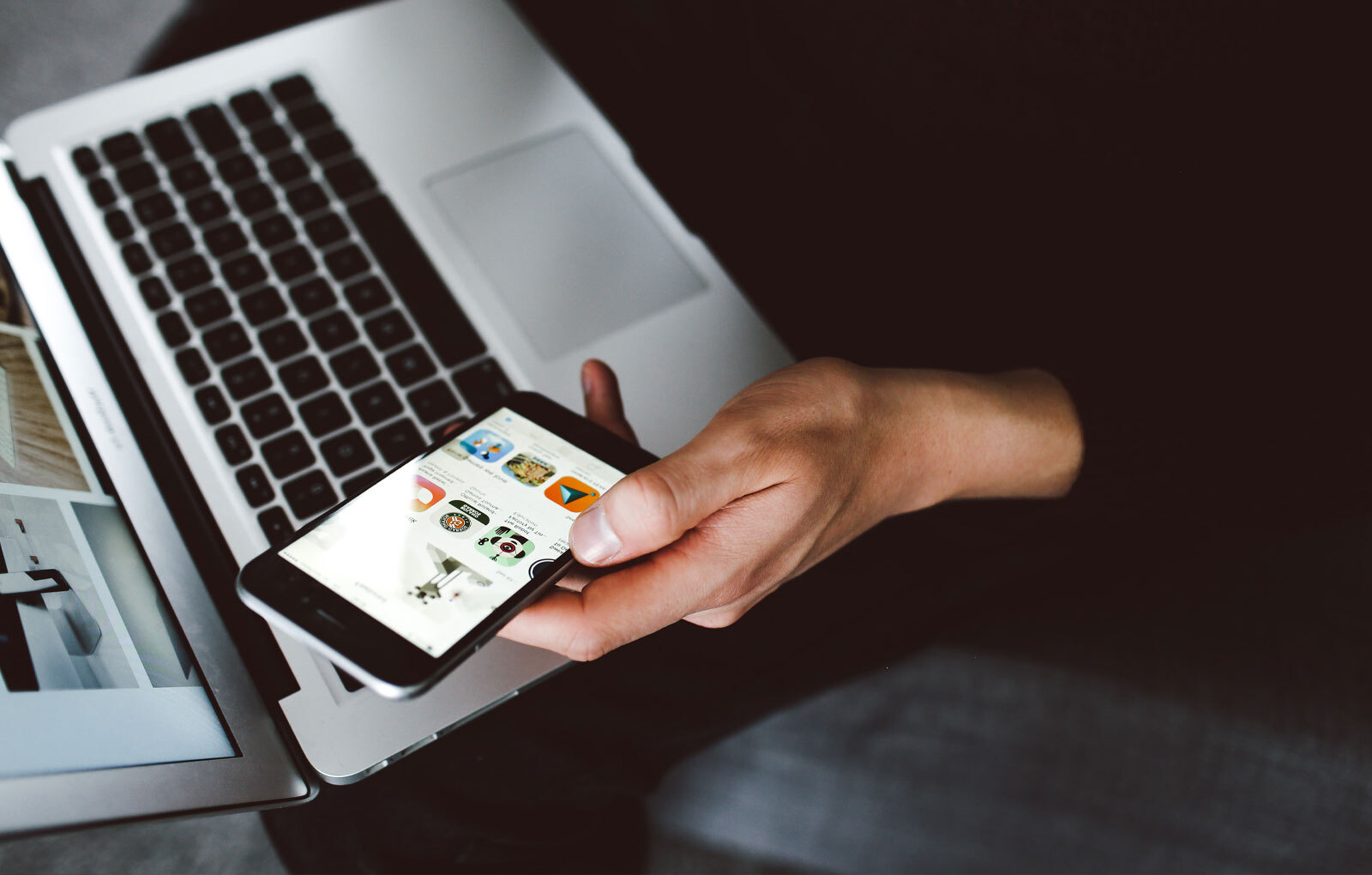
Overview of Android App Development
Android app development has grown significantly, with the global market for mobile applications expanding rapidly. The demand for skilled Android developers is higher than ever. This guide covers everything from the basics to advanced topics, including the best tools and technologies to use.
Key Components of Android App Development
Android SDK
The Android Software Development Kit (SDK) is essential for any developer. It provides libraries, tools, and documentation to build, test, and debug applications. The SDK includes the Android API, allowing access to various device features like the camera and GPS.
Java and Kotlin
Java and Kotlin are the primary programming languages for Android development. Java has been the traditional choice, but Kotlin has gained popularity due to its concise syntax and interoperability with Java. Both languages are supported by the Android SDK.
Android Studio
Android Studio is the official Integrated Development Environment (IDE) for Android development. It offers a comprehensive set of tools and features for building, testing, and debugging applications. Features include code completion, debugging tools, and project management capabilities.
Choosing the Right Tools
Selecting the right tools is crucial for productivity and efficiency. Here are some popular tools used by developers:
Android Studio
Android Studio is the official IDE for Android development, offering a wide range of features:
- Code Completion: Provides suggestions as you type, reducing errors and improving productivity.
- Debugging Tools: Includes advanced tools for stepping through code, setting breakpoints, and inspecting variables.
- Project Management: Offers robust capabilities, including version control integration with tools like Git.
IntelliJ IDEA
IntelliJ IDEA is another popular IDE among developers. It offers many of the same features as Android Studio, with additional capabilities such as support for multiple programming languages and a more extensive set of plugins.
Figma
Figma is a powerful design tool gaining popularity among Android developers. It allows collaborative creation of user interfaces (UI) and user experiences (UX) designs in real-time. Figma integrates well with Android Studio, making it easier to translate designs into code.
Designing Your App
Creating a visually appealing and user-friendly interface involves several steps:
User Research
Conduct user research to understand your target audience, their needs, and preferences. Tools like Google Analytics can help gather data about users.
Wireframing
Wireframing involves creating low-fidelity sketches of your app's UI, helping visualize the layout and flow. Tools like Figma or Sketch are ideal for wireframing.
Prototyping
Create interactive versions of your app's UI to test usability and functionality. Prototyping helps in refining the user experience.
Designing Screens
Design individual screens for your app, creating high-fidelity designs that include buttons, text fields, and images.
Building Your App
Writing code in Java or Kotlin involves several steps:
Setting Up Your Project
Set up a new project in Android Studio or IntelliJ IDEA. Choose the project template, set up necessary dependencies, and configure build settings.
Creating Activities
An activity represents a single screen in your app. Create activities for each screen using XML layouts or programmatically with Java or Kotlin.
Handling User Input
Create event listeners for buttons, text fields, and other UI elements to handle user input effectively.
Networking
Fetch data from servers or send data to servers using networking libraries like OkHttp and Retrofit.
Testing Your App
Testing is an essential part of the development process:
Unit Testing
Test individual components of your code in isolation using frameworks like JUnit and Espresso.
Instrumented Testing
Test your app's UI and behavior on an actual device or emulator using tools like Espresso and UI Automator.
Performance Testing
Test how well your app performs under various conditions using tools like Android Debug Bridge (ADB).
Deploying Your App
Make your app available to users through the Google Play Store or other app stores:
Creating a Keystore
Create a keystore for signing your app. The keystore contains a private key and a certificate used for signing.
Generating a Signed APK
Generate a signed APK ready for deployment by configuring build settings in Android Studio or IntelliJ IDEA.
Publishing on Google Play Store
Create a developer account and follow Google's guidelines to publish your app. Upload your signed APK and fill out necessary details like screenshots and descriptions.
Advanced Topics
Every Android developer should know these advanced topics:
Kotlin Coroutines
Kotlin Coroutines provide a way to write asynchronous code that is easier to read and maintain than traditional callbacks or RxJava.
Room Persistence Library
The Room Persistence Library is a SQLite object-relational mapping library that simplifies interactions with databases in Android apps.
LiveData
LiveData is a lifecycle-aware data holder class that allows data to be observed only when the observer is in the correct lifecycle state.
Best Practices
Follow these best practices to ensure high-quality Android apps:
Follow Android Design Guidelines
Ensure your app looks and feels like a native Android app by using Material Design components and adhering to UI design best practices.
Optimize Performance
Reduce memory usage and improve app responsiveness by using efficient data structures, reducing unnecessary computations, and employing caching mechanisms.
Test Thoroughly
Write comprehensive tests for all components of your app, including unit tests, instrumented tests, and performance tests.