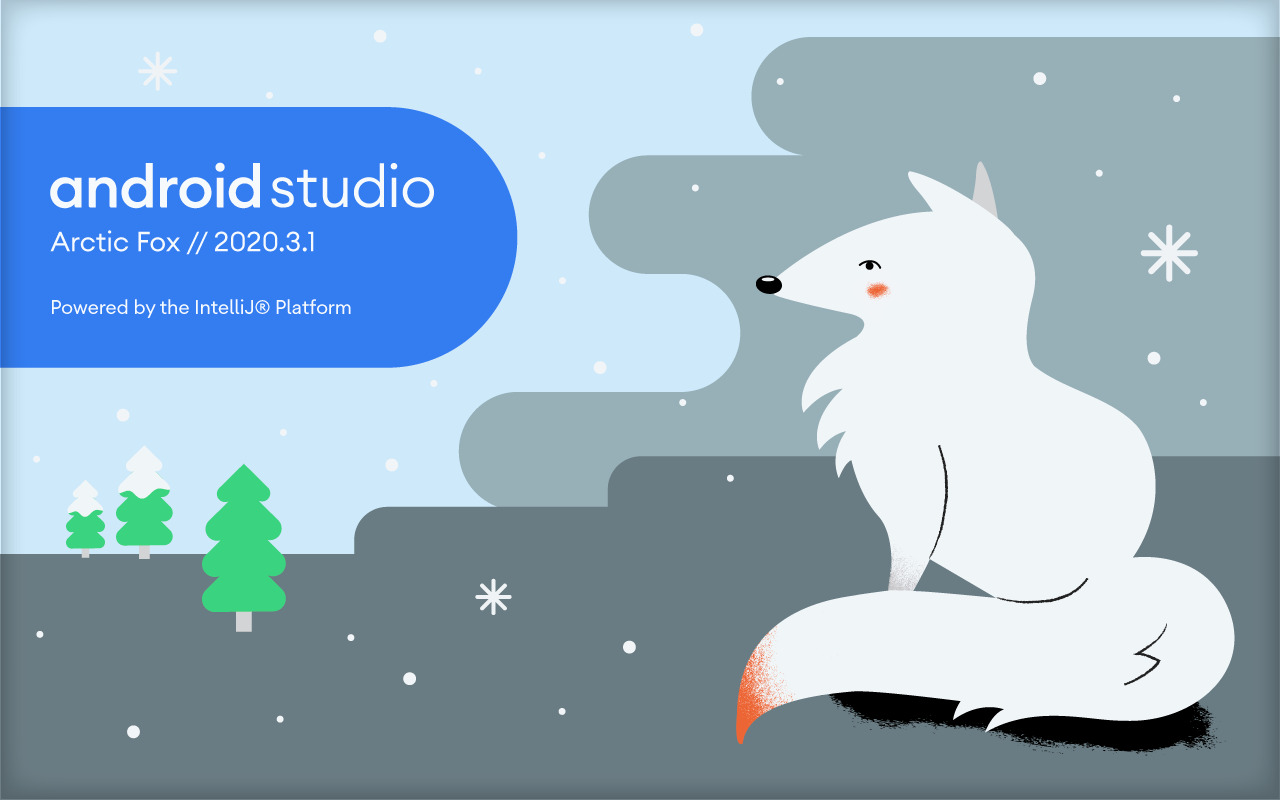
Introduction
Android Studio Arctic Fox is the latest major release of the Integrated Development Environment (IDE) for Android app development. Released in July 2021, this version brings numerous new features and improvements that enhance the overall development experience. For developers who prefer using Kotlin, this edition is particularly significant as it provides comprehensive support for Kotlin development. This article will cover the essential features and tools of Android Studio Arctic Fox, focusing on its Kotlin edition.
Setting Up an Android Studio Development Environment
Before diving into the specifics of Android Studio Arctic Fox, setting up an optimized development environment is essential. Here are the steps to download and install Android Studio on various platforms:
System Requirements
To run Android Studio Arctic Fox, your computer needs the following minimum specifications:
- Operating System: Windows 10 or later, macOS 10.14 or later, or Linux (64-bit)
- Processor: Intel Core i5 or AMD equivalent
- RAM: 8 GB or more
- Storage: At least 2 GB of free disk space
Downloading the Android Studio Package
-
Windows:
- Visit the official Android Studio download page.
- Click on the "Download" button for the recommended package (which includes the IDE and the Android Emulator).
- Select the appropriate package for your system architecture (32-bit or 64-bit).
- Once the download is complete, run the installer and follow the installation prompts.
-
macOS:
- Visit the Android Studio download page.
- Click on the "Download" button for macOS.
- Choose between the 64-bit and ARM (for M1 Macs) packages.
- After downloading, open the
.dmg
file and drag the Android Studio icon to your Applications folder.
-
Linux:
- Visit the Android Studio download page.
- Click on the "Download" button for Linux.
- Select the appropriate package for your system architecture (32-bit or 64-bit).
- Once downloaded, open a terminal and navigate to the directory where you saved the package. Run the installer script by executing
./studio-<version>.sh
.
Installing Additional Android SDK Packages
After installing Android Studio, additional SDK packages are needed to support various features and tools. Here’s how you can do it:
- Launch Android Studio.
- Open the SDK Manager by navigating to
File
>Settings
>System Settings
>Android SDK
. - Update the SDK Tools by clicking on the
Update
button. - Install Required Packages: Select the packages you need, such as the Android SDK Platform, Android SDK Build-Tools, and other necessary tools.
- Apply and OK: Apply your changes and close the SDK Manager.
Essential Features of Android Studio Arctic Fox
Android Studio Arctic Fox is packed with numerous features that enhance the development experience. Here are some of the key features:
1. Themed App Icon Preview
Preview how themed app icons respond to dynamic background wallpaper changes. This feature allows developers to see how their app icons will look across different devices and backgrounds, ensuring a consistent and visually appealing experience.
2. Gemini AI Assistant
Gemini is an AI assistant integrated into Android Studio that helps developers generate code, fix code issues, and answer questions about Android app development. Available in Android Studio Jellyfish, Gemini significantly reduces the time and effort required for coding tasks, making it easier for developers to focus on more complex aspects of their projects.
3. Compose Design Tools
Advanced design tools for Jetpack Compose are included, which is a modern UI toolkit for building natively rendered Android UIs. These tools provide better support for previewing and testing apps that use Jetpack Compose, making it easier to create visually appealing and interactive user interfaces.
4. Intelligent Code Editor
The code editor in Android Studio Arctic Fox has been significantly improved with features like code completion, refactoring, and debugging tools. The editor also supports multiple programming languages including Kotlin and Java, ensuring that developers can work seamlessly with their preferred language.
5. Flexible Build System
Android Studio’s build system is powered by Gradle, allowing developers to customize their build configurations to generate multiple build variants for different Android devices from a single project. The Build Analyzer tool helps analyze the performance of builds and identifies potential build issues, ensuring that developers can optimize their build processes efficiently.
6. Emulation Capabilities
The Android Emulator in Android Studio Arctic Fox allows developers to test their applications on a variety of Android devices. This includes responsive layouts that adapt to fit phones, tablets, foldables, Wear OS, TV, and ChromeOS devices. The emulator provides a realistic testing environment, helping developers identify and fix issues early in the development cycle.
Configuring Your Project
Once the development environment is set up and the necessary tools are installed, it’s time to configure your project settings in Android Studio Arctic Fox. Here’s how you can do it:
Project Structure
- Create a New Project: Launch Android Studio and create a new project by selecting
Start a new Android Studio project
. - Choose Your Project Template: Select the template that best fits your needs (e.g., Empty Activity, Navigation Drawer Activity).
- Set Up Your Project Structure:
- Package Name: Enter a unique package name for your app.
- Language: Choose Kotlin as your preferred language.
- Minimum SDK: Set the minimum SDK version required by your app.
- Target SDK: Set the target SDK version for your app.
- Version Code: Enter a unique version code for your app.
- Version Name: Enter a descriptive version name for your app.
Gradle Configuration
Gradle is the build tool used by Android Studio Arctic Fox. Here’s how you can configure your Gradle settings:
-
Open Your Gradle File:
- Navigate to
File
>Open...
and select your project’sbuild.gradle
file.
- Navigate to
-
Configure Dependencies:
- Add dependencies required by your project. For example:
groovy
implementation "org.jetbrains.kotlin:kotlin-stdlib-jdk8:$kotlin_version"
implementation 'androidx.core:core-ktx:1.6.0'
implementation 'androidx.appcompat:appcompat:1.3.1'
implementation "androidx.fragment:fragment-ktx:1.3.6"
implementation 'com.google.android.material:material:1.4.0'
- Add dependencies required by your project. For example:
-
Configure Build Features:
- Enable data binding or view binding as needed:
groovy
buildFeatures {
dataBinding true // or viewBinding true
}
- Enable data binding or view binding as needed:
-
Configure Kotlin Options:
- Set the JVM target for your Kotlin code:
groovy
kotlinOptions {
jvmTarget = '1.8'
}
- Set the JVM target for your Kotlin code:
-
Configure Build Types:
- Define build types (e.g., debug, release):
groovy
buildTypes {
release {
minifyEnabled false
proguardFiles getDefaultProguardFile('proguard-android-optimize.txt'), 'proguard-rules.pro'
}
}
- Define build types (e.g., debug, release):
Handling Kotlin Configuration Issues
Sometimes, even with proper configuration, issues related to Kotlin configuration might arise. Here’s how you can troubleshoot and resolve common issues:
"Kotlin Not Configured" Error
If a "Kotlin not configured" error occurs after upgrading to Android Studio Arctic Fox, it might be due to missing dependencies or incorrect configuration. Here’s how you can resolve it:
-
Check Dependencies:
- Ensure that the necessary Kotlin dependencies are added in your
build.gradle
file. For example:
groovy
implementation "org.jetbrains.kotlin:kotlin-android-extensions-runtime:$kotlin_version"
- Ensure that the necessary Kotlin dependencies are added in your
-
Verify Gradle File:
- Review your top-level
build.gradle
file to ensure that the Kotlin version is correctly set:
groovy
ext {
kotlin_version = "1.5.21"
version_navigation = "2.3.5"
version_lifecycle_extensions = "2.2.0"
version_lifecycle = "2.3.1"
version_room = "2.4.0-alpha04"
version_coroutine = "1.5.0"
}
- Review your top-level
-
Clean and Rebuild Project:
- Sometimes, cleaning and rebuilding the project can resolve configuration issues:
sh
./gradlew clean build
- Sometimes, cleaning and rebuilding the project can resolve configuration issues:
Additional Resources
For further learning and troubleshooting, here are some additional resources:
- Official Documentation: The official Android Developers website provides extensive documentation on using Android Studio Arctic Fox, including tutorials and guides.
- Community Support: Joining online communities like Stack Overflow or Reddit can provide valuable insights and solutions from other developers who have encountered similar issues.
- Books and Tutorials: There are numerous books and tutorials available that cover the basics and advanced topics of Android development using Kotlin in Android Studio Arctic Fox.
By leveraging these resources and mastering the features of Android Studio Arctic Fox, you can take your Android development skills to the next level and create innovative applications that meet the demands of modern users.